27 Copy Variable To Clipboard Javascript
in variables and I want to be able to create a link that uses Javascript to copy the address to the clipboard. I know there is the javascript:window.clipboardData.getData(), but I can't get to parse the ASP variable through. Does anyone know how I can do this. Thanks in advance. We have seen what copy to Clipboard is in JavaScript. Its method execCommand () used to copy the input from the text area using 'select ()', selects all the input data from the text area. We have seen 4 examples above, out of which one we have implemented using tooltip.
Commanding The Javascript Console
One thing you must now understand is that you can't copy / paste from the clipboard without the user's permission. The permission is different if you're reading or writing to the clipboard. In case you are writing, all you need is the user's intent: you need to put the clipboard action in a response to a user action, like a click.
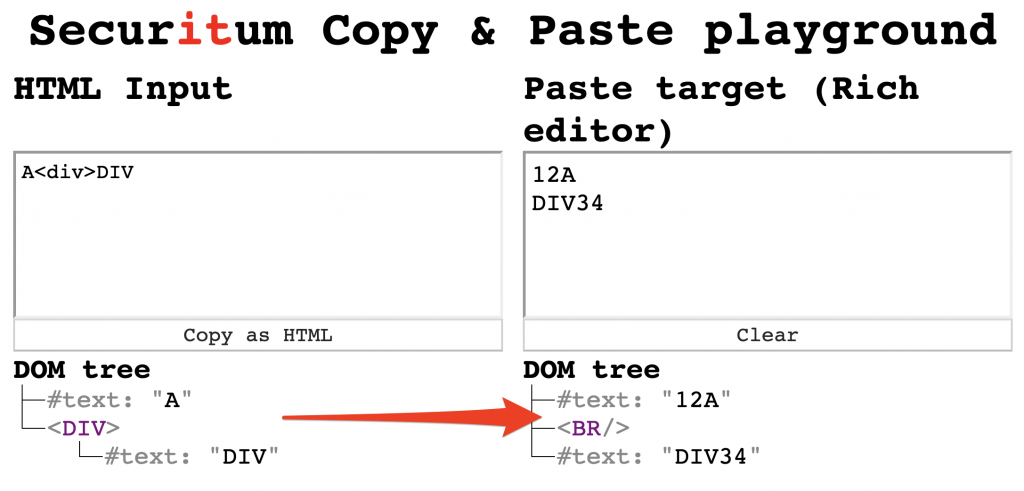
Copy variable to clipboard javascript. Javascript can easily do this in five short steps: Create a <textarea> element to be appended to the document. Set its value to the string that we want to copy to the clipboard. Append said <textarea> element to the current HTML document. It's never been a easier job to copy the text programmatically with in the browser. We use to rely on flash to get it done. Good news, now we have more than couple of ways to interact with clipboard using JavaScript. Clipboard is a data buffer which is used to store the data for a short-period of time to perform operations like cut, copy and paste. Then, we create a function name copyCodeToClipboard that will handle our Copy to Clipboard functionality. Inside that function, we hold the <textarea> element in an el variable and select its value with the el.select () method. And with the value selected, we use the document.execCommand ("copy") method to copy the value to the user's clipboard.
Copy text to the clipboard with JavaScript in 10 lines of code. By Alberto Montalesi. Published in Tutorial. February 25, 2020. 2 min read. Check out my Github for my free-to-read JavaScript Ebook that covers all the new features from ES6 to 2021. 8/10/2014 · Hi! I'm trying to copy a Javascript variable to the clipboard. I'm trying to copy a link to the clipboard when clicking on the link. I'm using the execCommand("copy"), but it won't work. Clipboard.js is one of those beautiful libraries that with a couple of lines will help you to achieve your goal. Clipboard.js is a modern approach to copy text to clipboard without Flash, it has no dependencies and is lightweight. To initialize clipboard.js, use the following code:
Javascript copy variable to clipboard. Copy output of a JavaScript variable to the clipboard, function copyToClipboard(text) { var dummy = document.createElement("textarea "); // to avoid breaking orgain page when copying more words // cant copy when I doubt you can copy a raw JS object to the clipboard. .execCommand('copy') copies a selection on a page (if allowed in user preferences). You can use the document.execCommand () to trigger the "cut" and "copy" actions, which replaces the clipboard's current contents with the currently selected data. The other option is to use the Clipboard API's Clipboard.writeText () or Clipboard.write () method to replace the clipboard's contents with specific data. a hard-coded string — such as data-copy="copy this to the clipboard". a CSS selector — such as data-copy="#mysection" . The text content of the first matching element is then copied.
Alright, so how can we add a way to copy those values to the clipboard? I'm going to use a simple button that looks like so: <button v-if="canCopy" @click="copy(something)">Copy</button> The v-if portion will handle hiding or showing the button based on if the browser supports the API. The click handler will pass the value to be copied. I can ... 26/7/2021 · Copy Plain Text using execCommand () In this example, we’re going to perform several steps. Create a temporary input. Assign the input a value. Append it to the document body. Highlight the contents with select () Copy the contents with execCommand () Remove the input from the document body. const copyToClipboard = (elementId) => { const temp ... Copy variable contents to the user clipboard. Raw. javascript-copy-paste.js. copyText (textToCopy) {. this.copied = false. // Create textarea element. const textarea = document.createElement('textarea')
1/8/2019 · execCommand () ¶. The document.execCommand () can be used to trigger the "cut" and "copy" actions, which replaces the current contents of the clipboard with the currently selected data. Have a look at the following piece of code: Here you create a fully transparent textarea and attach it to the document's body. Contrary to the previous answer, you CAN actually copy a variable string to the clipboard, as shown in my example. The user MUST EXPLICITLY take an action which causes the copy function to be called. If it is called automatically, the copy will be denied. This is most likely the cause of your problem. Here is my example. In order to copy a string to the system clipboard in the browser without using any dependency like clipboard.js, use this function: copying-strings-to-the-clipboard-using-pure-javascript.js 📋 Copy to clipboard ⇓ Download function copyStringToClipboard (str) { // Create new element
function copyToClipboard (text) { var dummy = document.createElement ("textarea"); // to avoid breaking orgain page when copying more words // cant copy when adding below this code // dummy.style.display = 'none' document.body.appendChild (dummy); //Be careful if you use texarea. setAttribute ('value', value), which works with "input" does ... How to copy value from variable to clipboard 1 minute read In javascript, copying a value from variable to clipboard is not straightforward as there is no direct command. There is this one command: document.execCommand('copy') which copies selected content to clipboard. We can use this command to copy content from a variable with a bit of ... 29/11/2020 · Nowadays all modern browsers allow copying content to the clipboard with JavaScript. But before this, it is not supported in most browsers because of security reasons. You need to ask the user to press CTRL+C to copy the currently selected text. The ZeroClipboard library uses invisible Adobe Flash movie for copying content to Clipboard.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... Copy to clipboard Copy text Copy Text to Clipboard Step 1) Add HTML: Example <!-- The text field --> Copy Text to Clipboard Using Document.execCommand () Method in JavaScript This is the most popularly used method of interacting with the clipboard. Using this method, you can perform the below 3 operations. Copy the text to the clipboard using Document.execCommand ('copy') Home » Javascript » javascript - Copy text to clipboard when a Chrome extension's browser action is clicked. javascript - Copy text to clipboard when a Chrome extension's browser action is clicked ... Angular 2+ Access/Change Variable from Lazy-Loaded component . February 26, 2020 Javascript Leave a comment. Questions: I want after ...
Copy ASP Variable To Clipboard Using Javascript. I have a search function that is reading companies from a database via ASP and spitting out the results. I have various fields of each company in variables and I want to be able to create a link that uses Javascript to copy the address to the clipboard. select () the method selects all the text in a <textarea> element or in an <input> element that includes a text field. document.execCommand ("copy") The method interacts with the clipboard and copies the text inside <textarea> or <input>. You can also use - document.execCommand ("cut") or document.execCommand ("paste") for cut and paste option. The clipboard object in Internet Explorer doesn't expose text/html via JavaScript. It does, however, support copying and pasting HTML into contenteditable elements. We can leverage this if we let the browser perform its default copy and paste, but 'hijack' the events to get/put the HTML data we want.
Is there an easy way to pass a variable or string to the Windows clipboard using photoshop javascript without having to write out a textfile and then use clip.exe to copy the contents of that file to windows clipboard? Or is it easily done in Visual Basic (in which case I would nee to alter my scrip... 21/11/2015 · Very useful. I modified it to copy a JavaScript variable value to clipboard: When you need to copy a variable to the clipboard in the Chrome dev console, you can simply use the copy () command. https://developers.google /web/tools/chrome-devtools/console/command-line-reference#copyobject. Copying to the clipboard is a tricky task to do in Javascript in terms of browser compatibility. The best way to do it is using a small flash. It will work on every browser. You can check it in this article. Here's how to do it for Internet Explorer: function copy (str) { //for IE ONLY! window.clipboardData.setData('Text',str); }
How To Copy The Text To The Clipboard In Javascript
Js Copy Span Text To Clipboard Code Example
Easy Copy To Clipboard Button In Javascript With Code
The Curious Case Of Copy Amp Paste On Risks Of Pasting
Caution When Using Variables In Dax And Power Bi Radacad
Easy Copy To Clipboard Button In Javascript With Code
Quiver Copy Selection To New Quiver Note Macro Library
Java Blog Documentum D2 Custom Action Plugins
Copy To Clipboard Example In Javascript Jquery How To Copy
Error Object Reference Not Set To An Instance Of An Object
Copying Text To Clipboard With Javascript By Angelos
How To Deep Clone An Array In Javascript Dev Community
Clipboard Basics Cut Copy Paste Delphi Code
Copy String To Clipboard Javascript Code Example
Storing The Information You Need Variables Learn Web
How To Deep Clone An Array In Javascript Dev Community
How To Copy Value From Variable To Clipboard Prakash
The Curious Case Of Copy Amp Paste On Risks Of Pasting
Copy Text To Clipboard Using Javascript Skptricks
Use Chrome Devtools Like A Senior Frontend Developer By
Javascript Copy To Clipboard For Dynamically Created Controls
Copy Text To Clipboard Using Vue Clipboard2 Vue Js
How To Copy Value From Variable To Clipboard Prakash
Need Help Adjusting Applescript Automation For Converting
Fast Text Copy Javascript Library Copyclipboard Css Script
Copy Text Amp Input Value To The Clipboard Using Pure
0 Response to "27 Copy Variable To Clipboard Javascript"
Post a Comment