28 Javascript Remove Undefined From Array
To remove empty, null, or undefinedelements from an array, we can use the filter()array method and pass a function to the method which returns the element currently getting looped. This method works because when an empty, nullor undefineditem is looped, it evaluates to boolean falsewhich will then be filtered out from the array. 1 week ago - The removed element from the array; undefined if the array is empty. ... The shift method removes the element at the zeroeth index and shifts the values at consecutive indexes down, then returns the removed value. If the length property is 0, undefined is returned.
Javascript Remove Object From Array If Value Exists In Other
Not quite an answer, but I would say it's better practice to try to avoid null/undefined in an array in this first place as much as you can. For instance, if your nulls come from mapping over another array with the map function returning null for certain elements, try to Array.filter out those elements prior to running the map. Makes your code more readable/self-documenting.
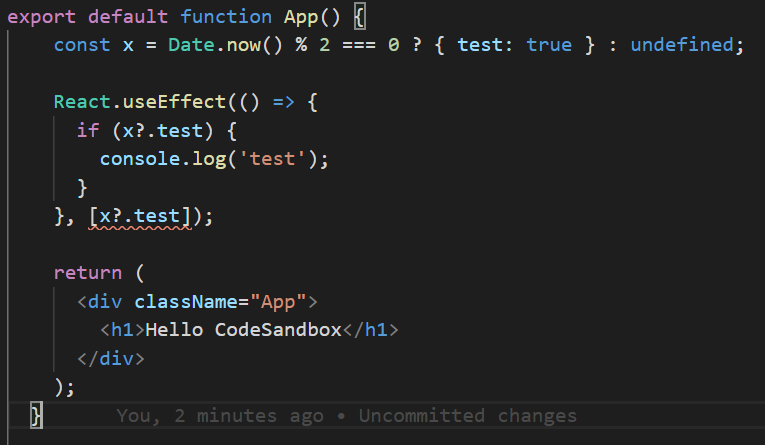
Javascript remove undefined from array. Apr 15, 2019 - Returns a copy of the array with all falsy values removed. In JavaScript, false, null, 0, "", undefined and NaN are all falsy. ... It also removes non-empty elements defined elements like 0. ... Actually Array.filter works on all browsers if you add some extra code. See below. Remove empty elements from an array in javascript - In some situations we may want to keep 0 in the array and remove anything else null, undefined and , this is one way. WRITE FOR US. Toggle sidebar. TUTORIALS TECHNOLOGY. ALL TECHNOLOGY ARTICLES FULL FORMS NEW; WEBINARS ... Feb 03, 2021 - But we can do it slighty more elegant, ... remove any falsy value, //we would call data = data.filter( Boolean ); //Since the Boolean() constructor returns true on truthy values //and false on any falsy value, this is a very neat option. ... var array = [0, 1, null, 2, "", 3, undefined, 3,,,,,, 4,, ...
The above example demonstrates that accessing: an uninitialized variable number; a non-existing object property movie.year; or a non-existing array element movies[3]; are evaluated to undefined.. The ECMAScript specification defines the type of undefined value:. Undefined type is a type whose sole value is the undefined value.. In this sense, typeof operator returns 'undefined' string for an ... But the disadvantage of this method is delete operator sets the array element to undefined. So it's better to use array slice function. Using Array Splice function. In Angular or typescript, If you want to remove array element completely use Javascript Array splice function as shown below. 26/2/2020 · Write a JavaScript function to remove. 'null', '0', '""', 'false', 'undefined' and 'NaN' values from an array. Sample array: [NaN, 0, 15, false, -22, '',undefined, 47, null] Expected result: [15, -22, 47]
To remove undefined values from a JavaScript array, you can simply use the filter () method like so: // ES5+ const arr = [1, undefined, 2, 3, undefined, 5]; const filteredArr = arr.filter(function (elem) { return elem !== undefined; }); console.log(filteredArr); // output: [1, 2, 3, 5] Or alternatively, you can use the arrow function syntax ... The array.splice () method is used to add or remove items from an array. This method takes in 3 parameters, the index where the element's id is to be inserted or removed, the number of items to be deleted and the new items which are to be inserted. This method actually deletes the element at index and shifts the remaining elements leaving no ... Since you do not return a value, the value undefined gets returned instead, so the final array just has a bunch of undefined values. MDN Web Docs Array.prototype.map() The map() method creates a new array populated with the results of calling a provided function on every element in the calling array.
13/12/2018 · Avoid null/undefined in JavaScript arrays. How to avoid TypeError: Cannot read property ___ of undefined using Array.filter (Boolean) tl;dr: Array.filter (Boolean) filters out falsy values ( null, undefined, 0, false, '') and avoids Cannot read property of undefined errors. Table of contents: To remove empty elements from a JavaScript Array, the filter () method can be used, which will return a new array with the elements passing the criteria of the callback function. The filter () method creates an array filled with all array elements that pass a test. To remove null or undefined values do the following: but if i run console.log (myObject.f ([1, 2,,,,, 3, 4, [5,,,,, ], 6,,,, 8, 3, [ [ [], 9]]])); i get [ 1, 2, 3, 4, [ 5,,,, ], 6, 8, 3, [ [ [], 9 ] ] ] and that is kinda weird result. I goes pretty well for the first layer but removes only one undefined from inner layers.
Jan 24, 2021 - How delete works I know some folks ... that a remove function is just a lot more easy to use and remember than splice, and honestly I don’t see any drawbacks with this approach; especially if we protect the global Array object, somehow. In the above code we are trying to print undefined array value of x[0] from x array so, ... The JavaScript array has a variety of ways you can delete array values. There are different methods and techniques you can use to remove elements from JavaScript arrays: pop — Removes from the ... 18/9/2020 · We are required to write a JavaScript function that takes in one such array and remove all the undefined elements from the array in place. We are only required to remove the undefined and empty values and not all the falsy values. Use a for loop to iterate over the array and Array.prototype.splice () to remove undefined elements in place.
7/9/2020 · Remove '0','undefined' and empty values from an array in JavaScript. Javascript Web Development Object Oriented Programming. To remove ‘0’. ‘undefined’ and empty values, you need to use the concept of splice (). Let’s say the following is our array −. var allValues = [10, false,100,150 ,'', undefined, 450,null] If you have jquery or javascript array with lots of undefined value and you want to remove all undefined value from array. you can remove undefined items from your array without using each loop you have to just use filter, I would like give the example how to remove undefined element from javascript array. so, let's see bellow example. Remove all false, null, undefined and empty values from array using Lodash; Check if a variable is null, empty, undefined or false Javascript; Remove items starting from last untill given condition is false Lodash; Remove items begin from start until given condition is false Lodash; Remove duplicate values from an array in Javascript
The pairs() function turns the input object into an array of key/value arrays. You do this so that it's easier to use reject() to eliminate undefined and null values. After, you're left with pairs that weren't rejected, and these are input for zipObject(), which reconstructs your object for you. 1/1/2021 · Code to remove undefined from array The below code will only remove the undefined values from array. const removeUndefinedFromArray = (arrayToClean) => { const cleanedArray = []; arrayToClean.forEach((val) => { if(typeof val !== "undefined"){ cleanedArray.push(val); } }); return cleanedArray; } var myArray = [5,6,undefined,7,8]; myArray = removeUndefinedFromArray(myArray); The JavaScript delete operator removes a property from an object; if no more references to the same property are held, it is eventually released automatically. ... If you want an array element to exist but have an undefined value, use the undefined value instead of the delete operator. In the following example, ...
JavaScript | Remove empty elements from an array. In order to remove empty elements from an array, filter () method is used. This method will return a new array with the elements that pass the condition of the callback function. array.filter () This function creates a new array from a given array consisting of those elements from the provided ... 14 hours ago - If there are no elements, or the array length is 0, the method returns undefined. Using Splice to Remove Array Elements in JavaScript · The splice method can be used to add or remove elements from an array. The first argument specifies the location at which to begin adding or removing elements. push () / pop () — add/remove elements from the end of the array unshift () / shift () — add/remove elements from the beginning of the array concat () — returns a new array comprised of this array joined with other array (s) and/or value (s) Found a problem with this page?
Using the Array.filter () method: In this approach, we are using the array.filter method. The filter method checks the array and filter out the falsy values of the array and return a new array. Use the splice () Method to Remove an Object From an Array in JavaScript. The method splice () might be the best method out there that we can use to remove the object from an array. It changes the content of an array by removing or replacing existing elements or adding new elements in place. The syntax for the splice () method is shown below. 2 weeks ago - The filter() method creates a new array with all elements that pass the test implemented by the provided function.
Jul 26, 2021 - The find() method returns the value of the first element in the provided array that satisfies the provided testing function. If no values satisfy the testing function, undefined is returned. Home › remove all undefined from array javascript › remove undefined from array javascript › remove undefined from array javascript lodash. 44 Remove Undefined From Array Javascript Written By Ryan M Collier. Saturday, August 21, 2021 Add Comment Edit. Filtering undefined elements from an array in TypeScript How TypeScript user-defined type guards can remove undefined or null types with Array.filter. November 29, 2020 · 4 min read. One feature I really appreciate about TypeScript is nullable type guards.
Our goal is to remove all the falsy values from the array then return the array. The good people at freeCodeCamp have told us that falsy values in JavaScript are false, null, 0, "", undefined, and NaN. They have also dropped a major hint for us! They suggest converting each value of the array into a boolean in order to accomplish this challenge. Jul 20, 2021 - The removed element from the array; undefined if the array is empty. ... The pop method removes the last element from an array and returns that value to the caller. 27/4/2016 · Remove empty null undefined values from JavaScript Array : There are many ways to remove the null values from the javascript arrays. Sometimes null/empty or undefined values are inserted in the array which are useless. So we need to remove the null, undefined values from array so that it remain good without garbage values.
Remove the First Element of an Array by Changing the Original Array in JavaScript. splice () method: The splice () method is used to remove or replace existing elements from the array. This method changes or modifies the original array. The splice () method also returns the elements which have been removed from the array. 18/2/2015 · Array.prototype.reduce() can be used to delete elements by condition from an array but with additional transformation of the elements if required in one iteration. Remove undefined values from array, with sub-arrays support. Apr 10, 2021 - remove undefined from array jquery,remove element from array jquery by index,javascript filter undefined from array,javascript remove undefined elements from array,jquery delete undefined values from array,jquery grep undefined items from array
How Can I Remove A Specific Item From An Array Stack Overflow
Remove Element From Array Javascript First Last Value
Sololearn On Twitter Remove Falsy Values From An Array Rt
How To Remove A Property From A Javascript Object
How To Remove Objects From Associative Array In Javascript
Bug Eslint Plugin React Hooks Cannot Use Optional Chaining
Js How To Remove Item From Array Code Example
Remove From Array If Exists Javascript Code Example
Remove Null Undefined And Empty Values From Array In Javascript
Js Array Copy Insert Remove Fails On Dynamic
Remove Null Undefined And Empty Values From Array In Javascript
Javascript Remove Undefined From Array Code Example
Remove Specific Item From Array Javascript Without Index Code
Creating Indexing Modifying Looping Through Javascript
Filtering Undefined Elements From An Array In Typescript
How To Remove Array Duplicates In Es6 Samanthaming Com
9 Ways To Remove Elements From A Javascript Array
Manipulating Javascript Arrays Removing Keys By Adrian
How To Remove An Element From A Javascript Array By Eniola
How To Filter Out Only Numbers In An Array Using Javascript
Remove Null Undefined And Empty Values From Array In Javascript
Typeerror Cannot Read Property Name Of Undefined At Array
How To Remove Empty Slots In Javascript Arrays
How To Remove All Falsy Values From An Array In Javascript
Removing Elements From An Array In Javascript Edureka
Remove Null Undefined And Empty Values From Array In Javascript
0 Response to "28 Javascript Remove Undefined From Array"
Post a Comment