24 Add Element Attribute Javascript
May 26, 2020 - This JSX tag's 'children' prop expects a single child of type 'Element', but multiple children were provided. ... If para1 is the DOM object for a paragraph, what is the correct syntax to change the text within the paragraph? ... Showing results for div id javascript id selector combine with ... Nov 08, 2011 - I'm trying to find a way that will add / update attribute using JavaScript. I know I can do it with setAttribute() function but that doesn't work in IE.
Visual Editor Change Types Optimize Resource Hub
The Element.attributes property returns a live collection of all attribute nodes registered to the specified node. It is a NamedNodeMap, not an Array, so it has no Array methods and the Attr nodes' indexes may differ among browsers. To be more specific, attributes is a key/value pair of strings that represents any information regarding that attribute.
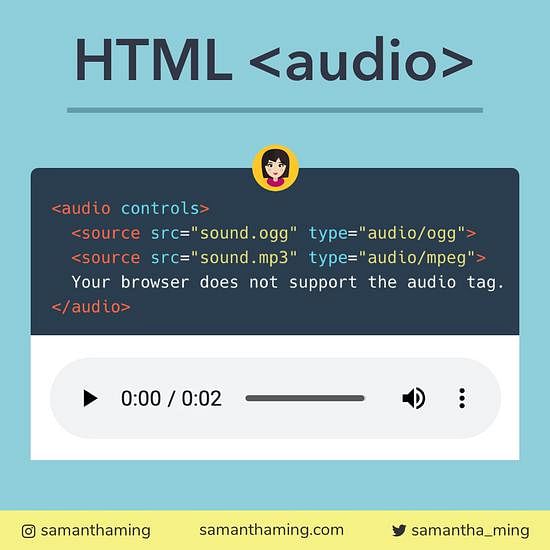
Add element attribute javascript. 13/12/2014 · First create the attribute and set the value. Then add it to the element.. var attr = document.createAttribute('step'); attr.value="any"; document.getElementById("id_price").setAttributeNode(attr); Then we set the attributes of our newly created element by setting the properties of the newly created object. Finally, we attach the element to the element that we want our element to be in as a child element by calling the appendChild method of the element that want our element to stay inside of with our newly created element as the argument.. For instance, if we create a new input element ... Definition and Usage The getAttribute () method returns the value of the attribute with the specified name, of an element. Tip: Use the getAttributeNode () method if you want to return the attribute as an Attr object.
Changing Element Attributes. 2:56 with Guil Hernandez and Joel Kraft. Attributes, like the href attribute on a link, or the action attribute on a form, exist as properties of an element object. You get or set them in a similar way we saw with text in the last video. In this video, we'll look at index.html and find an attribute to access. Sometimes, you may want to load a JavaScript file dynamically. To do this, you can use the document.createElement() to create the script element and add it to the document. The following example illustrates how to create a new script element and loads the /lib.js file to the document: JavaScript With this neat code snippet you can add an ID attribute to any existing HTML element by using JavaScript.
JavaScript setAttribute () The setAttribute () method is used to set or add an attribute to a particular element and provides a value to it. If the attribute already exists, it only set or changes the value of the attribute. So, we can also use the setAttribute () method to update the existing attribute's value. Apr 13, 2016 - These answers aren't really addressing the large confusion with between properties and attributes. Also, depending on the Javascript prototype, sometimes you can use a an element's property to access an attributes and sometimes you can't. The attributes collection is iterable and has all the attributes of the element (standard and non-standard) as objects with name and value properties. Property-attribute synchronization. When a standard attribute changes, the corresponding property is auto-updated, and (with some exceptions) vice versa.
In jQuery, you can use the .css () method for setting one or more CSS properties on an element. It works by modifying the value of the style property of the element. The above version of the .css () method takes the property name and value as separate parameters. To add multiple CSS attributes in a single line, you can pass a single object of ... Sep 09, 2020 - Use the setAttribute() to set the value of an attribute on a specified element. Setting the value of a Boolean attribute to whatever value, that value will be considered to be true. ... The JavaScript Tutorial website helps you learn JavaScript programming from scratch quickly and effectively. This JSX tag's 'children' prop expects a single child of type 'Element', but multiple children were provided. ... If para1 is the DOM object for a paragraph, what is the correct syntax to change the text within the paragraph? ... Showing results for div id javascript id selector combine with ...
In JavaScript, there are some approaches to add a class to an element. We can use the.className property or the.add () method to add a class name to the particular element. Now, let's discuss the approaches to add a class to an element. Add disabled attribute JavaScript | Disable HTML elements Example. Posted January 24, 2021 August 20, 2021 by Rohit. HTML webpages needed to disable/enable the button programmatically using JavaScript. Not only buttons are sometimes needed for input, checkbox, radio button, etc. ... Use id if you want to Add a disabled attribute on a single ... In this tutorial you will learn how to get, set and remove attributes from HTML elements in JavaScript. ... The attributes are special words used inside the start tag of an HTML element to control the tag's behavior or provides additional information about the tag.
26/9/2019 · Syntax: var elementVar = document.getElementById ("element_id"); elementVar.setAttribute ("attribute", "value"); So what basically we are doing is initializing the element in JavaScript by getting its id and then using setAttribute () to modify its attribute. Example: Below is the implementation of above approach. <!DOCTYPE html>. Tip: Use the element. setAttributeNode () method to add the newly created attribute to an element. Tip: Often, you will want to use the element .setAttribute () method instead of the createAttribute () method. Answer: Use the jQuery attr () method You can use the jQuery attr () method to add attributes to an HTML element. In the following example when you click on the "Select Checkbox" button it will add the checked attribute to the checkbox dynamically using jQuery.
This setAttribute JavaScript method is used to add a specified attribute to an element, giving the attribute a certain value. Take a look at the example below. It shows how you can modify an input field to an input button: Example. document .getElementsByTagName ( "input" ) [ 0 ].setAttribute ( "type", "button" ); Try it Live. Mar 02, 2020 - This JSX tag's 'children' prop expects a single child of type 'Element', but multiple children were provided. ... If para1 is the DOM object for a paragraph, what is the correct syntax to change the text within the paragraph? ... Showing results for div id javascript id selector combine with ... This JSX tag's 'children' prop expects a single child of type 'Element', but multiple children were provided. ... If para1 is the DOM object for a paragraph, what is the correct syntax to change the text within the paragraph? ... Showing results for div id javascript id selector combine with ...
We call setAttribute with 'style' and 'color: red' to add the style attribute with the value in the 2nd argument. Use Set the Property of the Element. We can also set the property with the attribute name to add an attribute. For instance, we can write: This JSX tag's 'children' prop expects a single child of type 'Element', but multiple children were provided. ... If para1 is the DOM object for a paragraph, what is the correct syntax to change the text within the paragraph? ... Showing results for div id javascript id selector combine with ... Use setAttribute () Method to add the readonly attribute to the form input field using JavaScript. setAttribute () Method: This method adds the defined attribute to an element, and gives it the defined value. If the specified attribute already present, then the value is being set or changed.
Mar 14, 2016 - Concerning boolean attributes, consider a DOM element defined by the HTML markup <input type="checkbox" checked="checked" />, and assume it is in a JavaScript variable named elem: According to the W3C forms specification, the checked attribute is a boolean attribute, which means the corresponding ... In vanilla JavaScript setting a data attribute of an element is done with the generic setAttribute () method. This is the equivalent of jQuery's $.data () method. Here's an example for setting and retrieving the attribute "data-foo": var el = document. querySelector ('div'); 12/3/2020 · Fortunately, you can just create an attribute and attach it to your button element by using JavaScript. We need to do 3 things: Create an attribute of the type id; Give it a value (name) Add the new attribute+value to the button element; HTML markup. First, the HTML button, which sadly has no ID attribute (buhu), yet: <
Now, we need to select the above elements by data attribute in JavaScript. Selecting the Single element. To select the single element, we need to use document.querySelector() method by passing a [data-attribute = 'value'] as an argument. Example: Nov 19, 2020 - Attributes can help us assign a style to a tag; disable a button; change the tag’s type, and more. Manipulating an element’s attributes in JavaScript is straightforward. First, a specific element is required. Some DOM queries return an array of elements (e.g. The JavaScript. DOM elements have an attributes property which contains all attribute names and values: Using Array.prototype.slice.call, which is also helpful in converting NodeLists to Arrays, you can convert the result to a true array which you can iterate over: The attributes property of an element is incredibly handy when you're looking to ...
Aug 25, 2020 - This JSX tag's 'children' prop expects a single child of type 'Element', but multiple children were provided. ... If para1 is the DOM object for a paragraph, what is the correct syntax to change the text within the paragraph? ... Showing results for div id javascript id selector combine with ... Steps to follow 1) First, create a div section and add some text to it using <p> tags. 2) Create an element <p> using document.createElement ("p"). 3) Create a text, using document.createTextNode (), so as to insert it in the above-created element ("p"). The setAttribute () method adds the specified attribute to an element, and gives it the specified value. If the specified attribute already exists, only the value is set/changed. Note: Although it is possible to add the style attribute with a value to an element with this method, it is recommended that you use properties of the Style object instead ...
const attribute = document. createAttribute ('id') attribute. value = `remove-${item. name} ` button. setAttributeNode (attribute) If the element does not exist yet, you have to first create it, then create the attribute, then add the attribute to the element, and finally add the element to the DOM: to get attribute you must first get the <td>value. Then you must get it's inputchildren using td.childrenand set the inputchildren attributevalue using input.setAttirbute('dummy', 'value'). Then retrieve it using getAttribute('dummy'). Element.setAttribute () Sets the value of an attribute on the specified element. If the attribute already exists, the value is updated; otherwise a new attribute is added with the specified name and value. To get the current value of an attribute, use getAttribute (); to remove an attribute, call removeAttribute ().
Most of the time you can simply address it as a property: want to set a title on an element? element.title = "foo" will do it. For your own custom JS attributes the DOM is naturally extensible (aka expando=true), the simple upshot of which is that you can do element.myCustomFlag = foo and subsequently read it without issue. Note: classList is iterable, i.e., we can list all the class names with a single loop as we do for an array. Time to master the concept of JavaScript Array. JavaScript Element Style. The property element.style corresponds to the JavaScript style attribute in HTML, meaning that the following two statements work in the same way.. h1.style.color = "green";
Semalt Element Attributes Everything You Need To Know Flip
A Complete Guide To Data Attributes Css Tricks
How To Add Update An Attribute To An Html Element Using
How To Dynamically Create New Elements In Javascript
Must Be Car Themed My First Js Dom Color Red Text Node
Custom Css For Shortpoint Design Elements Shortpoint Support
Dynamically Add Button Textbox Input Radio Elements In Html
Html Lt Audio Gt Tag Samanthaming Com
How Do I Add An Attribute To A Dynamically Created Element In
Add Data Attribute To Script Tag Dynamically Code Example
The Css Attr Function Got Nothin On Custom Properties
How To Build A Random Quote Generator With Javascript And
Week 1 Building A Solid Foundation With Html By Zac Heisey
Methods For Accessing Elements In The Dom File With
How To Work With Iframe On Selenium Graphic Guide Parsing
Html Div Tag Usage Attributes Examples
Webflow Alpine Js A Match From Heaven By Jarek Lipski
Javascript Set Input Value By Class Name Change Value
Retrieve Attributes From Json Message
Dynamically Add And Remove Html Elements Using Jquery
How To Add Update An Attribute To An Html Element Using
Manipulate Html Attributes Using Jquery
0 Response to "24 Add Element Attribute Javascript"
Post a Comment