34 How To Catch Undefined In Javascript
At the end we see that even though null and undefined are considered equal, they are not the same identity (equal without type conversion).As discussed, this is because they are of different types behind the scenes: null being an object and undefined being an undefined type. With that out of the way we can start to understand why trying to access a property of null or undefined may fail. throw The throw statement throws a user-defined exception. Execution of the current function will stop (the statements after throw won't be executed), and control will be passed to the first catch block in the call stack. If no catch block exists among caller functions, the program will terminate.
A Mostly Complete Guide To Error Handling In Javascript
Answer: Use the equality operator ( ==) In JavaScript if a variable has been declared, but has not been assigned a value, is automatically assigned the value undefined. Therefore, if you try to display the value of such variable, the word "undefined" will be displayed. Whereas, the null is a special assignment value, which can be assigned to a ...
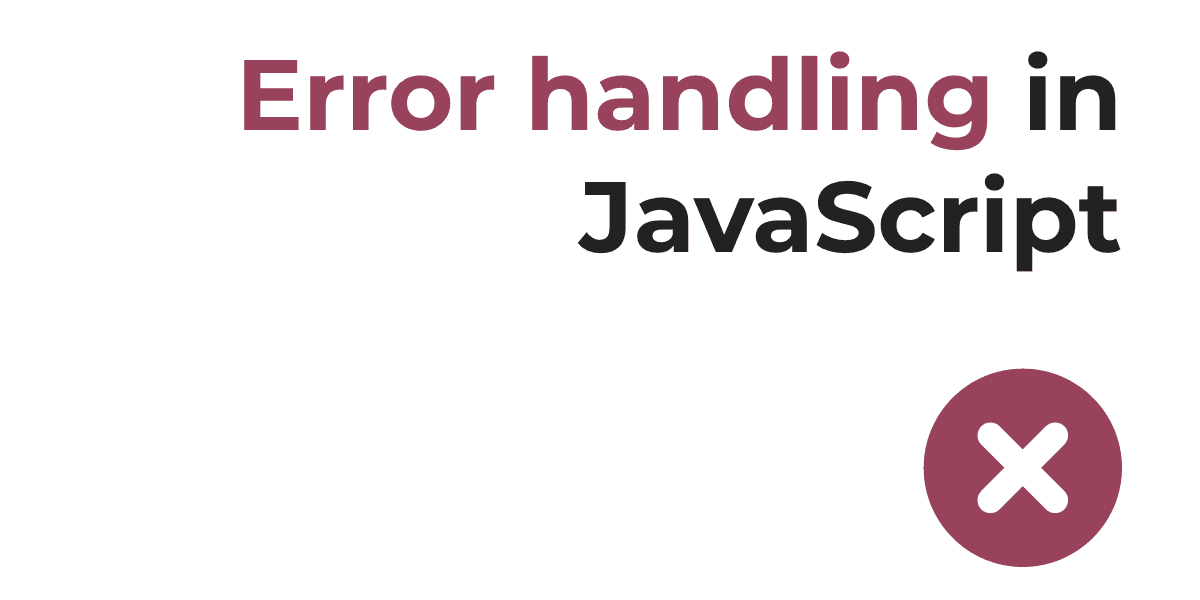
How to catch undefined in javascript. In a JavaScript program, the correct way to check if an object property is undefined is to use the `typeof` operator. See how you can use it with this simple explanation Published May 26, 2018, Last Updated May 29, 2019 In a JavaScript program, the correct way to check if an object property is undefined is to use the typeof operator. The try/catch/finally statement handles some or all of the errors that may occur in a block of code, while still running code. Errors can be coding errors made by the programmer, errors due to wrong input, and other unforeseeable things. The try statement allows you to define a block of code to be tested for errors while it is being executed. You can use the qualities of the abstract equality operatorto do this: if (variable == null){ // your code here. Because null == undefinedis true, the above code will catch both nulland undefined.
Generally, it is a good idea to catch both null and undefined values, as both represent an absence of a value. That means checking for null is one of the few times in JavaScript that using == is recommended, while otherwise === is generally recommended. Javascript Web Development Object Oriented Programming. Undeclared − It occurs when a variable which hasn't been declared using var, let or const is being tried to access. Undefined − It occurs when a variable has been declared using var, let or const but isn't given a value. Following is the code for undeclared and undefined in ... Description. undefined is a property of the global object. That is, it is a variable in global scope. The initial value of undefined is the primitive value undefined. In modern browsers (JavaScript 1.8.5 / Firefox 4+), undefined is a non-configurable, non-writable property, per the ECMAScript 5 specification.
When this occurs, JavaScript will show its displeasure by throwing a Undefined Variable error, indicating that the referenced object was not previously defined. For example, here we're making a simple statement of attempting to grab the .length property of our undefined itemvariable. We're also using a simple try-catch block and grabbing ... JavaScript catches adddlert as an error, and executes the catch code to handle it. JavaScript try and catch The try statement allows you to define a block of code to be tested for errors while it is being executed. JavaScript has a built-in asynchronous Either monad-ish data type called Promise. You can use it to do declarative error branching for undefined values: const exists = x => x != null; const...
Found a problem with this page? Edit on GitHub; Source on GitHub; Report a problem with this content on GitHub; Want to fix the problem yourself? See our Contribution guide. catch_statements. Statement that is executed if an exception is thrown in the try-block. exception_var. An optional identifier to hold an exception object for the associated catch-block. finally_statements. Statements that are executed after the try statement completes. These statements execute regardless of whether an exception was thrown or ... The short answer is that JavaScript interpreter returns undefined when accessing a variable or object property that is not yet initialized.
Using try/catch in your case is also very hard to read. You should stick to testing the values of the data object (and the dataobject itself - if data is undefined, data.url will throw indeed). - try-catch-finally Nov 28 '15 at 17:57 On the above example, we have added a try and catch block to manage exceptions. Then we declared a variable isIE and assigned a comment (/*@cc_on*/), with an exclamation (!) & false boolean value.So finally, only IE browser will consider it as var isIE = !false and return true and other browsers will return false.. We have also added OR condition (document.documentMode) in isIE variable which ... Probably a simple fix. I am expecting a value, but occassionally the data is undefined because of empty data. I would like Javascript code step to catch it instead of the zap erroring out. Any tips to use IF statement or another method to catch an undefined before it errors the code step when I use...
Test if a variable is undefined: var x; if (typeof x === "undefined") {. txt = "x is undefined"; } else {. txt = "x is defined"; } Try it Yourself ». More "Try it Yourself" examples below. It behaves the same as calling Promise.prototype.then (undefined, onRejected) (in fact, calling obj.catch (onRejected) internally calls obj.then (undefined, onRejected)). This means that you have to provide an onRejected function even if you want to fall back to an undefined result value - for example obj.catch (() => {}). The Throw Statement. The throw statement is used to generate user-defined exceptions. During runtime, when a throw statement is encountered, execution of the current function will stop and control will be passed to the first catch clause in the call stack. If there is no catch clause, the program will terminate. Check out this example showing how to use a throw statement:
25/2/2021 · In JavaScript, checking if a variable is undefined can be a bit tricky since a null variable can pass a check for undefined if not written properly. As a result, this allows for undefined values to slip through and vice versa. Make sure you use strict equality === to check if a value is equal to undefined. 30/12/2009 · As is often the case with JavaScript, there are multiple ways to do this: typeof foo !== 'undefined' window.foo !== undefined 'foo' in window The first two should be equivalent (as long as foo isn't shadowed by a local variable), whereas the last one will return true if the global varible is defined, but not initialized (or explicitly set to undefined ). The Promise `catch ()` Function in JavaScript. Promises in JavaScript are an object representation of an asynchronous operation. Promises are like a placeholder for some value that may not have been computed yet. If the async operation failed, JavaScript will reject the promise. The catch () function tells JavaScript what function to call if ...
JavaScript check undefined To check undefined in JavaScript, use (===) triple equals operator. In modern browsers, you can compare the variable directly to undefined using the triple equals operator. There are also two more ways to check if the variable is undefined or not in JavaScript, but those ways are not recommended. Compared to typeof approach, the try/catch is more precise because it determines solely if the variable is not defined, despite being initialized or uninitialized.. 4. Using window.hasOwnProperty(). Finally, to check for the existence of global variables, you can go with a simpler approach. Each global variable is stored as a property on the global object (window in a browser environment ... try { getData () // getData is not defined }catch (e) { alert (e) } This is basically how a try/catch is constructed. You put your code in the try block, and immediately if there is an error, JavaScript gives the catch statement control and it just does whatever you say. In this case, it alerts you to the error.
Example: When you try to reference an undefined variable or call a non-existent method, an exception will occur. The statements that you want to monitor for exceptions are contained within a try block. If an exception occurs within the try block then it is thrown. You can catch this thrown exception using the catch block that handles the ... TutorialsTeacher is optimized for learning web technologies step by step. Examples might be simplified to improve reading and basic understanding.
Isnullorundefined Javascript Code Example
Javascript Runtime Error Excel Is Undefined
Uncaught Typeerror Cannot Read Property Name Of Undefined
Top 10 Javascript Errors From 1000 Projects And How To
Failed Joining Lobby Undefined Urgent Help Install
React Native Encrypted Storage Githubmemory
Uncaught In Promise Typeerror Cannot Read Property Of
The 10 Most Common Mistakes Javascript Developers Make Toptal
Typeerror Cannot Read Property Delete Of Undefined Vue
Can Javascript Object Keys Have Spaces By Dr Derek Austin
Typeerror Cannot Read Property Orderitems Of Undefined
Check If Undefined Javascript Es6 Code Example
Javascript 06 Error Handling Programmer Sought
The Ultimate Guide To Exception Handling With Javascript Try
Python 网页crawler Javascript One Line Of Js Code Identifies
Tools Qa What Is Error Handling In Javascript And How To Do
Undefined 39 Is Null Or Not An Object Javascript Error When
7 Tips To Handle Undefined In Javascript
Handling Null And Undefined In Javascript By Eric Elliott
How To Quickly Locate The Code Error In Application Which
Javascript Errors Anatomy Of The Error Bugsnag Blog
How To Try Catch Statements That Return Promise Objects In Js
Webdatamenu Does Not Show Javascript Error Instead
Javascript Undefined How Does Undefined Work In Javascript
Uncaught Typeerror Cannot Read Property Of Undefined In
7 Tips To Handle Undefined In Javascript
Javascript Promise Consumer Then Catch Finally
7 Tips To Handle Undefined In Javascript
Adversary Playbook Javascript Rat Looking For That
Handling Javascript Errors In React With Error Boundaries
Cannot Read Property Status Of Undefined Issue 2248
Broadcast Plugin Cannot Read Property Channel Of Undefined
Undefined Old Voicestate For Left Or Banned Members Discord Js
0 Response to "34 How To Catch Undefined In Javascript"
Post a Comment