32 Javascript Callback Function With Parameters
Oct 18, 2019 - JavaScript callback functions; another important concept you must need to understand to become a successful JavaScript developer. But I am sure that after reading this article thoroughly you will be able to overcome any obstacles you previously had with callbacks. Dec 31, 2016 - In Javascript every function is a first class object, which means that every function is an Object and can be used like any other object(String, Number etc.). This allows the use of a function as a parameter in another function which is the fundamental idea of callback functions.
What S The Proper Way To Document Callbacks With Jsdoc
The event listener can be specified as either a callback function or an object that implements EventListener, whose handleEvent() method serves as the callback function. The callback function itself has the same parameters and return value as the handleEvent() method; that is, the callback accepts a single parameter: an object based on Event ...
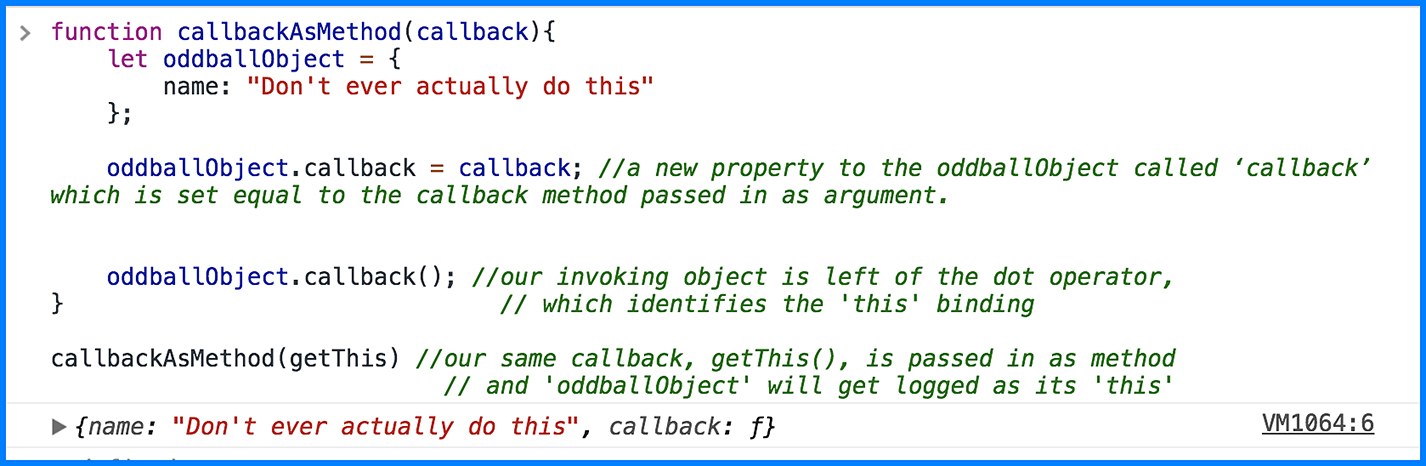
Javascript callback function with parameters. This will let us explore topics ... can use callback functions to manipulate the data after D3 receives it and parses it for us. ... Anonymous Function JavaScript tutorial with examples. Create a JavaScript anonymous function easily and quickly. ... How pass a Function as a Parameter of another ... Working with ReactJS and having trouble understanding how callback functions work with ReactJS. I have a parent component titled TodoFormComponent, which initializes my list of todo items. I've created a callback function on the TodoItemsComonent, but it doesn't trigger the updateItem method and display the selected item. JavaScript Callback function are the most special and important function of JavaScript whose main aim is to pass another function as a parameter where the callback function runs which means one function when infused into another function with the parameters is again called as per the requirement.
If you are not sure how many params are you going to be passed into callback functions. Using apply. function tryMe (param1, param2) { alert (param1 + " and " + param2); } function callbackTester(callback,params){ callback.apply(this,params); } callbackTester(tryMe,['hello','goodbye']); Now you have a strong type callback instead of just passing around function as we usually do in JavaScript. Generalize the callback type. We could also use generic to generalize the use case of callback with one parameter as below. By doing so, you don't have to define a new interface with a new name each time you need to use a callback with ... Callback functions are actually straightforward, and here is a classic example. Remember from the above introduction that 2 things happen in a callback function? We pass a function as a parameter into another function. Here, we pass foo() into setTimeout() as a parameter. The function gets called inside.
In JavaScript, a callback is a function passed into another function as an argument to be executed later. Suppose that you the following numbers array: let numbers = [ 1, 2, 4, 7, 3, 5, 6 ]; Code language: JavaScript (javascript) To find all the odd numbers in the array, you can use the filter () method of the Array object. Callback functions are one of the chief technique made from a base of functional programming. At the basic level, functional programming specifies functions as arguments. And it is much more used in modern-day by the master of programming. It is a simple technique yet seen in advanced JavaScript techniques. What is Callback function? What is a callback function? Simply put, a callback function is a function that passed as an argument of another function.Later on, it will be involved inside the outer function to complete some kind of action. A higher-order function is a function that takes a function as its argument, or returns a function as a result.. In JavaScript, functions are first-class objects which means functions ...
Dec 06, 2017 - Learn and understand the basics of callbacks in just 6 minutes with easy examples. 19/7/2016 · Here is an example with variable amount of parameters. function someObject(){ this.callbacks=new Array(); this.addCallback=function(cb){ this.callbacks[this.callbacks.length]=cb } this.callCallbacks=function(){ //var arr=arguments; this does not seem to work //arr[arr.length]="param2"; var arr = new Array(); for(i in arguments){ arr[i]=arguments[i]; } arr[arr.length]="another param"; i=0; for(i in … Dec 02, 2014 - This has nothing to do with said function being taken as parameter to another function. This confuses me a bit and I’d appreciate your thoughts. Thanks! ... Pingback: JavaScript Callback | J.-H. Kwon
31/5/2019 · Passing a function to another function or passing a function inside another function is known as a Callback Function. In other words, a callback is an already defined function which is passed as an argument to the other code. Syntax: function geekOne(z) { alert(z); } function geekTwo(a, callback) { … 10/3/2021 · You can pass some parameter to a function used as callback in JavaScript. These two options would look like this: function firstfunction(callback) { // code here callback(arg1, arg2); } firstfunction(callbackfunction); Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Jul 02, 2020 - This article gives a brief introduction to the concept and usage of callback functions in the JavaScript programming language. Functions are Objects The first thing we need to know is that in JavaScript, functions are first-class objects. As such, we can work with them in the same way we work ... In JavaScript, you can also pass a function as an argument to a function. This function that is passed as an argument inside of another function is called a callback function. JavaScript Callbacks. A callback is a function passed as an argument to another function. Using a callback, you could call the calculator function ( myCalculator ) with a callback, and let the calculator function run the callback after the calculation is finished: Example. function myDisplayer (some) {.
When you have a callback that will be called by something other than your code with a specific number of params and you want to pass in additional params you can pass a wrapper function as the callback and inside the wrapper pass the additional param(s). A custom callback function can be created by using the callback keyword as the last parameter. It can then be invoked by calling the callback () function at the end of the function. The typeof operator is optionally used to check if the argument passed is actually a function. The callback function as the name implies is the function that gets executed after the other function has finished executing. A callback is a function that is passed as a parameter into another function to be executed later to perform some operation. The callback function is executed asynchronously. When to use callback functions in JavaScript?
Aug 04, 2019 - This article gives a brief introduction to the concept and usage of callback functions in the JavaScript programming language. Functions are Objects The first thing we need to know is that in JavaScript, functions are first-class objects. As such, we can work with them in the same way we work ... Jan 16, 2016 - By default you cannot pass arguments to a callback function. For example: A callback, as the name suggests, is a function that is to execute after another function has finished executing. As we know, in JavaScript, functions are objects. Because of this, functions can take functions as arguments, and other functions can also return it. Functions that take the additional function as a parameter are called higher-order ...
Callback functions work because in JavaScript, every function is an object. This means that we can work with them like any other object. We can assign functions to variables, or pass them as arguments, just like we would with any other value. Sep 04, 2018 - An explanation that doesn't use jQuery, setTimeout, etc. Jun 10, 2020 - In JavaScript, the way to create a callback function is to pass it as a parameter to another function, and then to call it back right after something has happened or some task is completed. Let’s see how… ... To understand what I’ve explained above, let me start with a simple example.
Feb 02, 2016 - The method of passing in functions as parameters to other functions to use them inside is used in JavaScript libraries almost everywhere · A JavaScript Callback Function is a function that is passed as a parameter to another JavaScript function, and the callback function is run inside of the ... Sep 15, 2019 - It is a simple technique yet seen in advanced JavaScript techniques. ... Callback function or higher-order function is a function that is passed to another function as a parameter and the callback function is executed inside another function. A callback function is established to solve a common ... to the element passed in. 0:48. The third function takes in an element and the callback function. 0:50. It calls the callback and passes in one argument, which is the element. 0:55. Into the callback function and executes the callback. 1:02. Let's use makeRed and makeBlue as callbacks to the addStyleToElement 1:06.
Javascript anonymous callback function with parameters. Anonymous Callback Function Clarification, by passing them into the function as arguments. Let's try to reimplement this function so that it could be called as follows: console.log( " And that is why you can pass an anonymous function as a parameter to a function, and it can execute it as a callback. With callback functions on JavaScript methods, the arguments are automatically passed in by the method itself. Using the Array.forEach () method as an example, the callback function will always receive the current item, its index, and the array itself as arguments, in that order. You can name the parameters for them anything you want, or even ... A callback function is a function that is passed as an argument to another function, to be "called back" at a later time. A function that accepts other functions as arguments is called a higher-order function, which contains the logic for when the callback function gets executed. It's the combination of these two that allow us to extend ...
Aug 14, 2016 - In the above example, we pass an anonymous function into whatTime as our callback argument. For those comfortable with JavaScript this is a common pattern but it can be hard to read. It is the same as this example, which passes a function name as the callback instead: As you see in the example above, we pass a function as a parameter to the click method. And the click method will call (or execute) the callback function we passed to it. This example illustrates a typical use of callback functions in JavaScript, and one widely used in jQuery. Examine this other classic example of callback functions in basic ... The above example is a synchronous callback, as it is executed immediately.. Note, however, that callbacks are often used to continue code execution after an asynchronous operation has completed — these are called asynchronous callbacks. A good example is the callback functions executed inside a .then() block chained onto the end of a promise after that promise fulfills or rejects.
Callback Review When a function is invoked, the JavaScript interpreter creates what is known as an execution context. This record contains information about where the function was called from (the call-stack), how the function was invoked, what parameters were passed, etc.
How To Rewrite A Callback Function In Promise Form And Async
A Guide To Test Automation With Javascript Callbacks And
Javascript Callback Functions What Are Callbacks In Js And
5 Differences Between Arrow And Regular Functions
Call Action In Crm 2015 Easily From Javascript Library
What Is Callback Function In Javascript How It Is Replaced
Javascript Callback Functions What Are Callbacks In Js And
Using Functions As Children And Render Props In React Components
What Are Callbacks In Javascript Coding Dummies
Understand Callback Functions In Javascript Through Examples
Asynchronous Javascript Async Await Tutorial Toptal
Callback Function With Parameters Javascript Example Code
Javascript Callback Function How Callback Function Work In
Javascript Function Multiple Parameters Code Example
Callback Function Javascript Code Example
Callbacks And Promises Living Together In Api Harmony
Understand Callback Function In Javascript By Satyendra
Javascript Callback Functions With Examples Dot Net Tutorials
Sequential Asynchronous Calls In Node Js Using Callbacks
Explaining Javascript Callbacks Callback Functions Are A
Everything About Callback Functions In Javascript
Callbacks The Definitive Guide Learn The Real Way To Async
Type Safe Callbacks In C Codeproject
Javascript Callback Function Simply Explained By Le Van
What Is A Callback Function In Javascript
How To Create A Custom Callback In Javascript Geeksforgeeks
A Practical Guide To Es6 Arrow Functions By Arfat Salman
Mastering This In Javascript Callbacks And Bind Apply
0 Response to "32 Javascript Callback Function With Parameters"
Post a Comment