29 Javascript Read Local Json File Example
Dec 02, 2019 - Learn to read & write JSON files, both asynchronously and synchronously, using the Node.js native fs module. And in the same directory (folder), we have a JavaScript file index.js. Our task is to access the content of the json file through the JavaScript file. Method 1: Using require module (NodeJS environment only)
How To Load A Json Model From Manifest File And Send Json
A common use of JSON is to exchange data to/from a web server. When receiving data from a web server, the data is always a string. Parse the data with JSON.parse(), and the data becomes a JavaScript object.
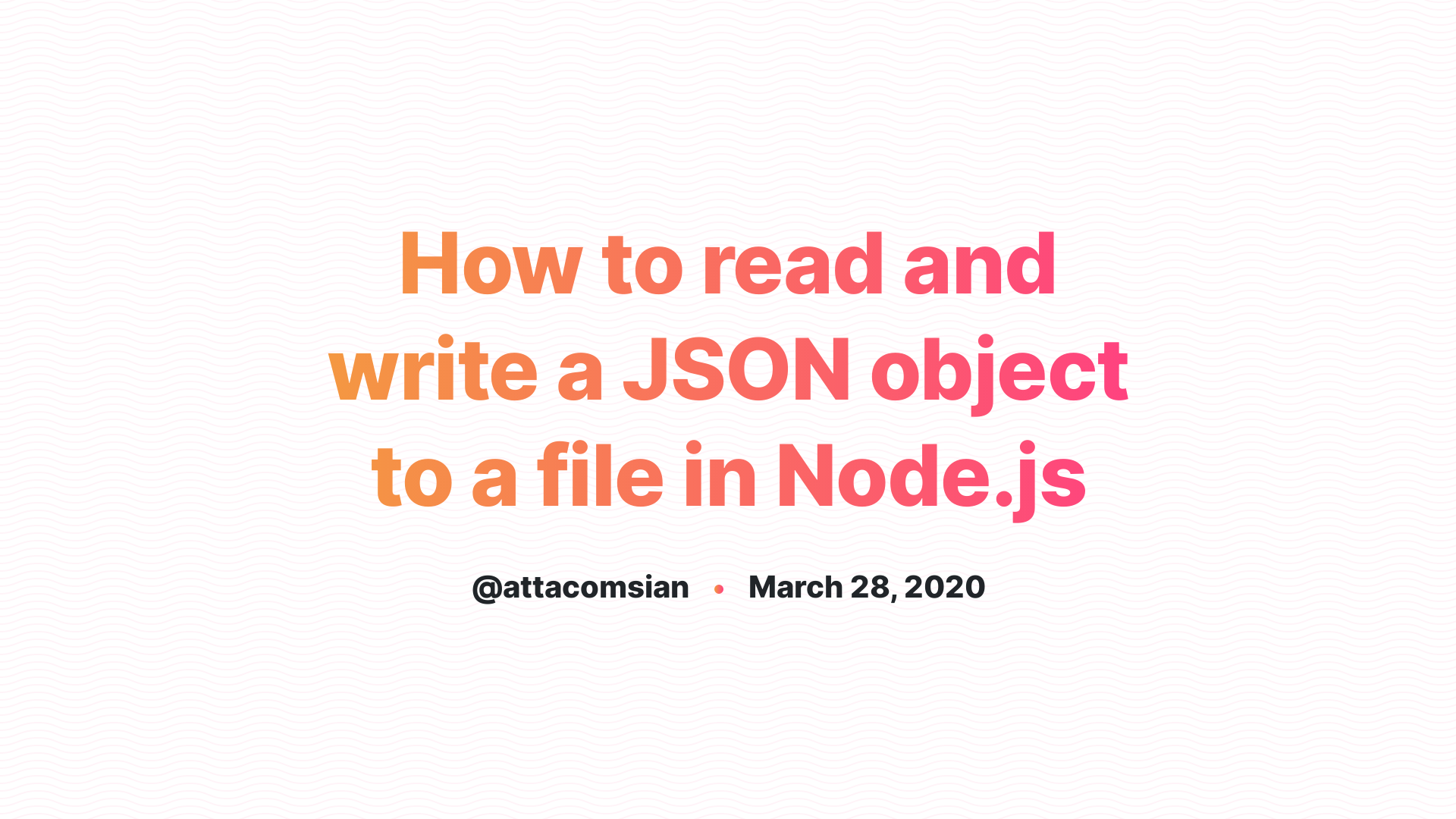
Javascript read local json file example. A common use of JSON is to read data from a web server, and display the data in a web page. This chapter will teach you, in 4 easy steps, how to read JSON data, using XMLHttp. JSON Example What you see as output in the above example is the raw data extracted from the JSON file. Now , there are lot of things you can do with the extracted data, like populate a SELECT dropdown list with the JSON data or simply create an HTML table . example.json, which is the example JSON file index.html , which is the HTML page to call the JavaScript and display the data From the command prompt we can simply invoke live-server within the new ...
Nov 18, 2020 - // pure javascript let object; let httpRequest = new XMLHttpRequest(); // asynchronous request httpRequest.open("GET", "local/path/file.json", true); httpRequest.send(); httpRequest.addEventListener("readystatechange", function() { if (this.readyState === this.DONE) { // when the request has ... Mar 25, 2018 - p5.js is a client-side JS platform that empowers artists, designers, students, and anyone to learn to code and express themselves creatively on the web. It is based on the core principles of Processing. http://twitter /p5xjs — - Loading external files: AJAX, XML, JSON · processing/p5.js Wiki How to read an external local JSON file in JavaScript 0 votes I have saved a JSON file in my local system and created a JavaScript file in order to read the JSON file and print data out.
Example 1. For reading the external Local JSON file (data.json) using java script. data = ' [ {"name" : "Ashwin", "age" : "20"}, {"name" : "Abhinandan", "age" : "20"}]'; // Mention the path of the json file in the script source along with the javascript file. <script type="text/javascript" src="data.json></script> <script type="text/javascript " ... // pure javascript let object; let httpRequest = new XMLHttpRequest(); // asynchronous request httpRequest.open("GET", "local/path/file.json", true); httpRequest.send(); httpRequest.addEventListener("readystatechange", function() { if (this.readyState === this.DONE) { // when the request has ... 2) The correct method. 2.1) - Create A New XMLHttpRequest. 3) Usage. 3.1) - Parse JSON string into object. In this tutorial, we will see how to Load JSON file locally using pure Javascript. the proper way to include or load the JSON file in a web application, Instead of using JQuery we'll The correct method using XMLHttpRequest.
JSON is a text-based data format that is used to store and transfer data. For example, // JSON syntax { "name": "John", "age": 22, "gender": "male", } In JSON, the data are in key/value pairs separated by a comma ,. JSON was derived from JavaScript. So, the JSON syntax resembles JavaScript object literal … Load Local JSON File This example demonstrates how to load package a json file with your application and then load it at runtime. The application includes 2 buttons: 1) a load button that will fetch t Step 3 — Read File Contents using FileReader Object. The contents of the selected File object is read using the FileReader object. Reading is performed asynchronously, and both text and binary file formats can be read. Text files (TXT, CSV, JSON, HTML etc) can be read using the readAsText() method.
Mar 30, 2021 - How to read an external local JSON file in JavaScript? How to read an external local JSON file in JavaScript? Three ways of accessing the local JSON file. How to read JSON file using react API call (fetch, axios). Inside src (No Need Axios or Fetch for api call). then import and read inside any component like below. State -Try to assign and handle through the state because state is dynamic, it enables a component to keep track of changing information ... 11. If you have couple of json files: import data from 'sample.json'; If you were to dynamically load one of the many json file, you might have to use a fetch instead: fetch (`$ {fileName}.json`) .then (response => response.json ()) .then (data => console.log (data)) Share. edited Dec 4 '19 at 16:23. answered Dec 4 '19 at 15:06.
Normally, the idea is to go to the location of the file (or download it if you prefer). To access a local file on a server, you could simply write the path of your JSON in you web browser. Now, doing this redirects you to the URL specified and sho... function readJSON (path) {. var xhr. Continue Reading. Normally, the idea is to go to the location of the file (or download it if you prefer). To access a local file on a server, you could simply write the path of your JSON in you web browser. Now, doing this redirects you to the URL specified and show the file. Using a local JSON file in the React app is a common approach when you want to render some static data, maintain server config, etc. Rendering JSON Objects from an API Call You have seen the example where a local JSON file is used, but at the same time you may need to access JSON data from the server.
Nov 07, 2020 - This guide will demonstrate how to correctly fetch data from a JSON file in your React app and consume it on the frontend. Topic: JavaScript / jQuery Prev|Next. Answer: Use the jQuery $.getJSON() Method. You can simply use the $.getJSON() method to load local JSON file from the server using a GET HTTP request. If the JSON file contains a syntax error, the request will usually fail silently. Let's try out the following example to understand how it basically works: For reading the external Local JSON file (data.json) using javascript, first create your data.json file: data = '[{"name" : "Ashwin", "age" : "20"},{"name" : "Abhinandan", "age" : "20"}]'; Mention the path of the json file in the script source along with the javascript file.
Generate project. Create new angular project using the following angular cli command. ng new angular-read-local-json-file and then you'll be asked to choose the stylesheet format, select your preferred format and hit enter.. Then Angular CLI will create necessary file and folders and will install the necessary npm packages. 26/10/2019 · try {// create a reader Reader reader = Files. newBufferedReader (Paths. get ("customer.json")); //create ObjectMapper instance ObjectMapper objectMapper = new ObjectMapper (); //read customer.json file into tree model JsonNode parser = objectMapper. readTree (reader); // read customer details System. out. println (parser. path ("id"). asLong ()); System. out. println (parser. path … Nov 18, 2020 - // pure javascript let object; let httpRequest = new XMLHttpRequest(); // asynchronous request httpRequest.open("GET", "local/path/file.json", true); httpRequest.send(); httpRequest.addEventListener("readystatechange", function() { if (this.readyState === this.DONE) { // when the request has ...
JSON. Function Files. A common use of JSON is to read data from a web server, and display the data in a web page. This chapter will teach you, in 4 easy steps, how to read JSON data, using function files. Feb 07, 2021 - I made this simple project to ... with a local JSON file. Some Fast & Furious Bytes about JSON. ... JSON is a complete platform and language independent. JSON is a text based format file, So it's easy to read and understand even for non-coders. JSON mostly used for asynchronous transactions. We can convert any Javascript Object into ... Nov 18, 2020 - // pure javascript let object; let httpRequest = new XMLHttpRequest(); // asynchronous request httpRequest.open("GET", "local/path/file.json", true); httpRequest.send(); httpRequest.addEventListener("readystatechange", function() { if (this.readyState === this.DONE) { // when the request has ...
The fs.readFile () and fs.writeFile () methods can be used to read and write data to JSON files asynchronously. To synchronously interact with the filesystem, there are fs.readFileSync () and fs.writeFileSync () methods available. You can also use the global require () method to synchronously read and parse a JSON file at startup. One good place to start is reading the jquery doc link $.getJSON and follow that up with the MDN Working with JSON. If you have a local *amp stack dev environment, here's some code to get started (basically from the jquery doc example) HTML - index.html - you'll need to download jquery or change the reference to use the CDN Nov 18, 2020 - Get code examples like "load local json file javascript" instantly right from your google search results with the Grepper Chrome Extension.
JSON stands for J ava S cript O bject N otation. JSON is a lightweight data interchange format. JSON is language independent *. JSON is "self-describing" and easy to understand. * The JSON syntax is derived from JavaScript object notation syntax, but the JSON format is text only. Code for reading and generating JSON data can be written in any ... FileReader.readAsText (): Reads the contents of the specified input file. The result attribute contains the contents of the file as a text string. This method can take encoding version as the second argument (if required). The default encoding is UTF-8. In this case we are using FileReader.readAsText () method to read local .txt file. This code ... The first parameter is the url of .json file and second parameter is a callback function which will be executed once .json file is loaded. It passes parsed data object as a parameter to callback function. Let's look at an example. Create a sample file "users.json" in the data folder of your project's root folder and paste the following JSON in it.
Sep 11, 2020 - JSON (JavaScript Object Notation) is a lightweight format for sharing data. Although it’s derived from JavaScript — it may be used with many programming languages. In this article however, we’ll be… how to import and use json document typescript. ts type json file. create json data file in typescript and import. import json file in d.ts. check type typescript in json file. typescript load local json file. import json from json file into ts file. angular reading json file in ts. 18/8/2020 · Code to access employees.json using fetch function − fetch("./employees.json") .then(response => { return response.json(); }) .then(data => console.log(data)); Note − While the first function is better suited for node environment, the second function only works in the web environment because the fetch API is only accessible in the web environment.
Here we are fetching our people.json file. After the file has been read from disk, we run the then function with the response as a parameter. To get the JSON data from the response, we execute the json() function. The json() function also returns a promise. This is why we just return it and chain another then function. JavaScript Object Notation (JSON) is a standard text-based format for representing structured data based on JavaScript object syntax. It is commonly used for transmitting data in web applications (e.g., sending some data from the server to the client, so it can be displayed on a web page, or vice versa). Method 3: Reading Local JSON Files in Offline Angular Apps Using ES6+ import Statement. If your Angular application goes offline, reading the JSON file with HttpClient will fail. In this case, we have one more method to import local JSON files using the ES6+ import statement which supports importing JSON files.
How To Read And Write Json File Using Node Js Geeksforgeeks
Working With Json Learn Web Development Mdn
How To Write To A Json File From A React App Quora
Json Server Json Server Journaldev
Read Local Json Files In Angular 12 Application Positronx Io
Importing Data From Json Files And Power Bi Rest Apis Into
How To Load Local Json File Issue 1110 Swagger Api
Read Locally Json File Use Fetch Method In React Js By
Vuejs Get Data From Json File Get Data From Url Example
How To Parse Json From Local File In React Native Codevscolor
Read An External Json File In Angular 8 Vr Softcoder
How To Set Multiple Json Data To A One Time Request Using
How To Read And Write A Json Object To A File In Node Js
Your Json Data Is Ready For Analysis In Tableau 10 1
How To Read Local Json File In React Js By Rajdeep Singh
Using R To Download And Parse Json An Example Using Data
How To Read Angular Json And Angular Json Pipe Edupala
How To Read Local Json File In React Js By Rajdeep Singh
How To Read An External Local Json File In Javascript
Using Json Server To Build Local Data Interface Develop Paper
Ionic Json Getting Local Json Data Edupala
How To Write Json Object To File In Java Crunchify
D3 Js Tutorial 10 Loading External Data
Echarts Loading Json Data Solution Take Broken Line Diagram
Read Write Json Files In Protractor Typescript
Load Local Json File Javascript Code Example
0 Response to "29 Javascript Read Local Json File Example"
Post a Comment