32 How To Write A Simple Javascript Program
It helps programmers in writing modular codes. Functions allow a programmer to divide a big program into a number of small and manageable functions. Like any other advanced programming language, JavaScript also supports all the features necessary to write modular code using functions. You must have seen functions like alert () and write () in ... To write a "Hello, World!" program, open up a command line text editor such as nano and create a new file: With the text editor opened, enter the following code: The console object in Node.js provides simple methods to write to stdout, stderr, or to any other Node.js stream, which in most cases is the command line.
How To Run Javascript Program In Notepad
Creating your first JavaScript Program. In the last lesson you learned how to create simple java script program. To run the program open the html file in any browser and click on the "Run Java Script First Program". The will display the message box. Here is the screen shot of the browser. Test JavaScript program from here. Here is the code of ...
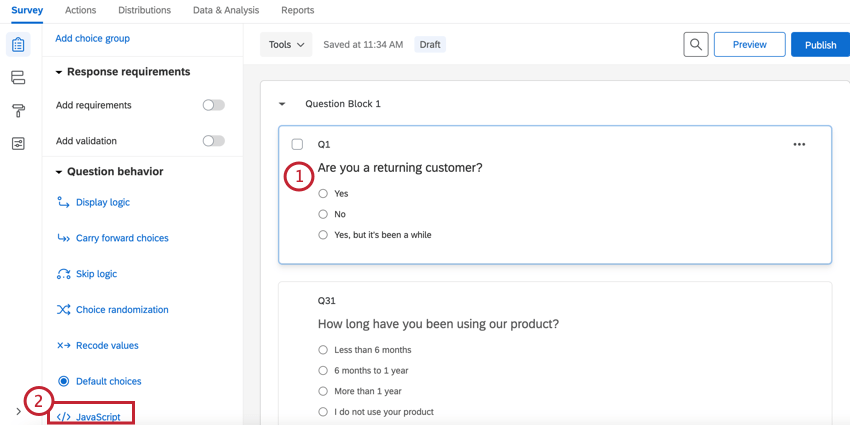
How to write a simple javascript program. JavaScript treats this as a single-line comment, just as it does the // comment. The HTML comment closing sequence --> is not recognized by JavaScript so it should be written as //-->. Example. The following example shows how to use comments in JavaScript. <script language = "javascript" type = "text/javascript"> <!-- // This is a comment. JavaScript drawing [6 exercises with solution] [An editor is available at the bottom of the page to write and execute the scripts.] 1. Write a JavaScript program to draw the following rectangular shape. Go to the editor Expected Output: Click me to see the solution. 2. Write a JavaScript program to draw a circle. Go to the editor Expected Output: The Script takes 3 attributes: language: this attribute indicates which scripting language we are using in the program and its value will be javascript. type: this attribute indicates the scripting language is getting used and its value should be "text/javascript". src: it specifies the URL of an external JavaScript file.This attribute overrides any existing JavaScript place between the ...
The HTML <script> tag is used to define a client-side script (JavaScript). The <script> element either contains script statements, or it points to an external script file through the src attribute. Common uses for JavaScript are image manipulation, form validation, and dynamic changes of content. All Examples Introduction Control Flow Functions Arrays and Objects Strings Miscellaneous. JavaScript Program To Print Hello World. JavaScript Program to Add Two Numbers. JavaScript Program to Find the Square Root. JavaScript Program to Calculate the Area of a Triangle. JavaScript Program to Swap Two Variables. For genuine 'for each'-type loops in javascript, you can use one of the several popular javascript libraries; for instance, jQuery defines an 'each' iterator that gets you the elements of an array one by one. Javascript Array Sort: Sorting Arrays in Javascript. To sort an array in javascript, use the sort() function. You can only use sort() by ...
How To Connect to an API (with JavaScript) Now we know the basic elements of working with API in JavaScript, and we can create a step-by-step guide to creating a JavaScript app with API integration: 1. Get an API key The document-relative path to that file will look one way for the home page– scripts/site.js –but for a page located inside a folder named about, the path to the same file would be different; ../scripts/site.js –the ../ means climb up out of the about folder, while the /scripts/site.js means go to the scripts folder and get the file site.js. 11/10/2013 · We can now go ahead and write the JavaScript program. Add the following code after the <h1> tag and save the file: <script> <alert(“Hello World!”) <script> That’s it! You’ve now successfully crated a JavaScript program. Now use your web browser to open test.html. This is what you should see: Easy, right? All JavaScript code is written between <script></script> tags.
A very easy tutorial for JavaScript beginners. var x= is a variable declaration. If you want to create a variable, you must declare the variable using the var statement.x will get the result, namely, true or false.Then we use a condition statement if else to give the script the ability to choose between two paths, depending on this result (condition for the following action). Step 3 - Creating a simple Web JavaScript Project. In the rest of the article, we will create a simple web page that uses JavaScript for its functionality. It is as basic as it can be, but it might be a useful starting point for those not that familiar with JavaScript. forerunners of JavaScript. •On the first page of their book, the authors suggest that the first step in learning any language is to write a simple program that prints the words "hello, world" on the display. That advice remains sound today. •In Monday's class, you learned how to execute JavaScript functions in the console window.
Debug JavaScript Program in notepadHow to Write JavaScript Program in notepad. Debug JavaScript Program in notepadHow to Write JavaScript Program in notepad. The Java Connection. Understandably, JavaScript's connection with Java is regularly misunderstood. They are not the same thing. » Java, created by » Sun Microsystems, is a full computer programming language like C++, suitable for writing complete, large-scale programs.; JavaScript, on the other hand, was created by » Netscape.It was based to some degree on Java — the syntax of the code is ... Well, unfortunately, it's not that simple. So, this blog is for those users who need the exact information in short and simple format. Fibonacci Series JavaScript Program (for beginners)
3. Write a JavaScript program to get the current date. Go to the editor. Expected Output : mm-dd-yyyy, mm/dd/yyyy or dd-mm-yyyy, dd/mm/yyyy. Click me to see the solution. 4. Write a JavaScript program to find the area of a triangle where lengths of the three of its sides are 5, 6, 7. Go to the editor. Although the game seems simple, you need to figure out how to create the logic that follows the game rules in JavaScript. Hence, before you start writing any code, break down the flow of the game into logical steps first. For a simple game like Tic Tac Toe, I find it helpful to draw a small flow chart to visualize the different outcomes of the ... Using console.log () console.log () is used in debugging the code. Hello, World! Here, the first line is a comment. console.log ('Hello, World!'); prints the 'Hello, World!' string to the console. 2. Using alert () The alert () method displays an alert box over the current window with the specified message.
JavaScript in Visual Studio Code. Visual Studio Code includes built-in JavaScript IntelliSense, debugging, formatting, code navigation, refactorings, and many other advanced language features. Most of these features just work out of the box, while some may require basic configuration to get the best experience. Yaphi and James walk you through making your own simple JavaScript quiz. This is a fun exercise and a great way to learn a variety of coding techniques. 2/8/2017 · To write the “Hello, World!” program, first open up your preferred web browser’s JavaScript Console. There are two primary ways that we can go about creating the “Hello, World!” program in JavaScript, with the alert() method and with the console.log() method.
JavaScript String Concatenation. Adding two strings together using the concatenating (+) operator Adding two strings together with a space in the first string Adding two strings together with a space in between Adding two strings together using using the += operator Adding strings and numbers. Concatenation Explained. Example 3: Dynamic JavaScript Calculator using the HTML, CSS and JavaScript. // Use insert () function to insert the number in textview. // Use equal () function to return the result based on passed values. /* Here, we create a backspace () function to remove the number at the end of the numeric series in textview. */. This was the first simple JavaScript unit test from start to end. If you installed the Visual Studio Code extension, it will run tests automatically once you save a file. Let's try it by extending the test with this line: expect (getAboutUsLink ("cs-CZ")).toBe ("/o-nas");
Javascript example is easy to code. JavaScript provides 3 places to put the JavaScript code: within body tag, within head tag and external JavaScript file. Let's create the first JavaScript example. <script type="text/javascript">. document.write ("JavaScript is a simple language for javatpoint learners"); </script>.
How And When Should I Start Creating Programs In Javascript
How To Write A Hello World Program In Javascript Digitalocean
Part 1 Task 1 Practice How To Use Functions And Chegg Com
Search Bar Using Html Css And Javascript Geeksforgeeks
Write A Program In Javascript To Take Input From User And
10 Best Javascript Courses Online Recommended By Js Developers
Prcatical No 09 Write A Simple Javascript Program Using
Responsive Website Basics Code With Html Css And
Let S Learn Javascript Lesson 3 Beginning Javascript
Run Javascript In Visual Studio Code Stack Overflow
Javascript Java Script Object Oriented Programming
How To Write A Hello World Program In Javascript Digitalocean
What S The Difference Between Python And Javascript Skillcrush
The Basic Story Of Javascript Past To Present Dolovers Blog
Simple Programs In Javascript Html Instalzonejunkie
Learning Javascript Essentials And Guidelines Smashing
1 Writing Your First Javascript Program Javascript
Programmers Sample Guide Dynamically Generate Html Table
How To Run Javascript Code Quora
Run Javascript In Visual Studio Code Stack Overflow
Learn Javascript Tutorials For Beginners Intermediate And
Learning Javascript Design Patterns
Life Runs On Code A Simple Paint Program Using Html5 And
Educational Products Easy And Professional Tools To Learn
How To Call Javascript Function In Html Javatpoint
How To Write A Javascript Program Dev Community
Your First Program In Javascript You Need 5 Minutes And A
Javascript Recursion With Examples
0 Response to "32 How To Write A Simple Javascript Program"
Post a Comment