26 Javascript Split String In Array
If the limit is zero, the split() returns an empty array. If the limit is 1, the split() returns an array that contains the string. Note that the result array may have fewer entries than the limit in case the split() reaches the end of the string before the limit. JavaScript split() examples. Let's take some examples of using the split() method. Split Method in Javascript The Split method is used to split a string into an array of strings and breaking at a specified delimiter string or regular expression.
Javascript Fundamental Es6 Syntax Split A Multiline String
Definition and Usage. The join () method returns an array as a string. The elements will be separated by a specified separator. The default separator is comma (,). join () does not change the original array.
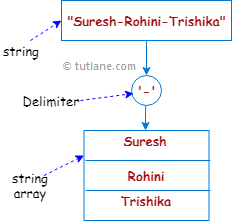
Javascript split string in array. Dec 14, 2020 - See the Pen JavaScript Split a string and convert it into an array of words - string-ex-3 by w3resource (@w3resource) on CodePen. Contribute your code and comments through Disqus. Previous: Write a JavaScript function to check whether a string is blank or not. Apr 28, 2021 - Since we used the period (.) as ... output array do not contain the period in them – the output separated strings do not include the input separator itself. You can operate on strings directly, without storing them as variables: "Hello... I am another string... keep on learning!".split("..."); // ... The split() method in javascript accepts two parameters: a separator and a limit. The separator specifies the character to use for splitting the string. If you don't specify a separator, the entire string is returned, non-separated. But, if you specify the empty string as a separator, the string is split between each character. Therefore: s.split('')
The String in JavaScript can be converted to Array in 5 different ways. We will make use of split, Array.from, spread, Object.assign and for loop. Let's discuss all the methods in brief. 1. The split() method. This method is used to split a string based on a separator provided and returns an Array of substring. Jul 20, 2021 - Warning: When the empty string ("") is used as a separator, the string is not split by user-perceived characters (grapheme clusters) or unicode characters (codepoints), but by UTF-16 codeunits. This destroys surrogate pairs. See “How do you get a string to a character array in JavaScript?” on ... The split () method is used to split a string on the basis of a separator. This separator could be defined as a comma to separate the string whenever a comma is encountered. This method returns an array of strings that are separated.
4 days ago - Javascript string split() is a built-in function that is used to split the string into an array of the substring and returns a new collection. JavaScript String - split() Method, This method splits a String object into an array of strings by separating the string into substrings. Type of Array in JavaScript with Example. There are two types of string array like integer array or float array. 1. Traditional Array. This is a normal array. In this, we declare an array in such a way the indexing will start from 0 itself. 0 will be followed by 1, 2, 3, ….n.
Browse other questions tagged javascript arrays string split integer or ask your own question. The Overflow Blog Diagnose engineering process failures with data visualization. Podcast 370: Changing of the guards: one co-host departs, and a new one enters. Featured on Meta ... Creating arrays by using split method in a string We have seen how to create string using elements of an array. Now we will see how to create an array from a string by using some delimiter. For this we will use split() method which works on a string and create array out of it. This is one of the method used to create an array. Javascript Split String in Array to Objects in Array I have this Array ["2.900000F02A_1313_01","2.600000F02A_1315_03","2.900000F02A_1354_01"] And I want to split it like this:
JavaScript code recipe: split string into array. Tutorial on how to split a string into an array in JavaScript with code examples. Posted on January 27, 2021. Sometimes, you need to create an array that represents your string for your JavaScript program. You can create an array that represents your string data by using the split method, which ... 24/6/2020 · split () method just creates an array from an input string and a delimiter string The join () method returns a string containing the values of all the elements in the array glued together using the string parameter passed to join () You can use split () and join () to emulate replace () method, but It will make your code less clear to understand. PHP Array Functions PHP String Functions PHP File System Functions PHP Date/Time Functions PHP Calendar Functions PHP MySQLi Functions PHP Filters PHP Error Levels ... You can use the JavaScript split() method to split a string using a specific separator such as comma (,), space, etc.
This JavaScript tutorial explains how to use the string method called split() with syntax and examples. In JavaScript, split() is a string method that is used to split a string into an array of strings using a specified delimiter. The modern / proper way to split a UTF8 string is using Array.from (str) instead of str.split ('') The JavaScript split () method is used to split a string using a specific separator string, for example, comma (, ), space, etc. However, if the separator is an empty string ( "" ), the string will be converted to an array of characters, as demonstrated in the following example:
Dec 05, 2019 - I have this string · var str = "January,February,March,April,May,June,July,August,September,October,November,December"; now I want to split this by the comma, and then store it in an array. ... Just wanted to comment that this does not require jquery this is part of javascript itself. Make a program that filters a list of strings and returns a list with only your friends name in it.javascript ... Sets can be used to store __________. in js ... Encountered two children with the same key, `undefined`. flatlist · filter the falsy values out of an array in a very simple way! String.split () Method The String.split () method converts a string into an array of substrings by using a separator and returns a new array. It splits the string every time it matches against the separator you pass in as an argument. You can also optionally pass in an integer as a second parameter to specify the number of splits.
JavaScript's string split method returns an array of substrings obtained by splitting a string on a separator you specify. The separator can be a string or regular expression. Invoke the split method on the string you want to split into array elements. Pass the separator you want to use to ... split() method in Javascript allows to split a string using a specific delimiter/separator and returns an array of substrings. syntax: string.split(delimiter,limit) delimiter - A character/characters used to separate the string. limit - Limits the number of elements returned to the array.( optional) Given a statement which contains the string and separators, the task is to split the string into substring. String split() Method: The str.split() function is used to split the given string into array of strings by separating it into substrings using a specified separator provided in the argument.. Syntax: str.split(separator, limit)
Converting string to array using split: In this method, we use split () to convert a string to array in JavaScript. After the split is used, the substring is returned in an array. Using the following syntax we can convert strings to arrays in JavaScript. The split () methods take in a delimiter as a parameter and based on the specifying value ... This function splits a javascript string into a two dimensional array. The string must have two delimiters embedded in the string. The first delimiter is for the first array, and the second delimiter is for the second array. function stringTo2dArray(string, d1, d2) { return string.split(d1).map(function(x){return x.split(d2)}); } Jul 26, 2021 - If the separator is unspecified ... single array element. The same also happens when the separator is not present in the string. If the separator is an empty string (“”) then every character of the string is separated. limit: Defines the upper limit on the number of splits to be found ...
Definition and Usage. The split () method splits a string into an array of substrings, and returns the new array. If an empty string ("") is used as the separator, the string is split between each character. The split () method does not change the original string. 4 weeks ago - The join() method creates and returns a new string by concatenating all of the elements in an array (or an array-like object), separated by commas or a specified separator string. If the array has only one item, then that item will be returned without using the separator. String.split The split method splits a string into an array of strings by separating it into substrings. This tool is extremely useful. As an example, we'll take apart the query string parameters of a URL.
16/6/2021 · The split () Method in JavaScript The split () method splits (divides) a string into two or more substrings depending on a splitter (or divider). The splitter can be a single character, another string, or a regular expression. After splitting the string into multiple substrings, the split () method puts them in an array and returns it. < h2 > JavaScript Strings </ h2 > < p > The split() method splits a string into an array of substrings, and returns the array. </ p > ... Split ( ) Slice ( ) and splice ( ) methods are for arrays. The split ( ) method is used for strings. It divides a string into substrings and returns them as an array. It takes 2 parameters, and both are optional. string.split (separator, limit); Separator: Defines how to split a string… by a comma, character etc.
# 4 Ways to Convert String to Character Array in JavaScript. Here are 4 ways to split a word into an array of characters. "Split" is the most common and more robust way. But with the addition of ES6, there are more tools in the JS arsenal to play with 🧰 22/6/2020 · JavaScript split() syntax. array = string.split(separator,limit); string – input value of the actual string. separator – delimiter is used to split the string. This is optional and can be any letter/regular expression/special character. limit – the number of words that need to be accessed from the array. This is an optional parameter. Nov 09, 2020 - The morse code is split into an array with a ' ' as the separator. (Without ' ' as an argument you would end up with an array that has separate entries for each . and -.) The morse code array is mapped/transformed to a text array · A string is created from the array with '' as the separator.
STRING.split(SEPARATOR) Convert the string to an array. Elements will be separated by the defined SEPARATOR. Click Here: Array.from(STRING, FUNCTION) Convert the given string, to an array. The mapping FUNCTION is optional. Click Here: Object.assign(TARGET, SOURCE) Assigns the SOURCE to the TARGET. Click Here: JSON.parse(STRING) Parse a JSON ...
How To Split Strings By Spaces Help Uipath Community Forum
Javascript Split Examples Dot Net Perls
Java String Array String Array In Java With Examples Edureka
Split String Into Array Of Characters Javascript Code Example
How To Manipulate A Part Of String Split Trim Substring
Convert Comma Separated String To Array Using Javascript
Javascript String Split Tutorial
Using Powershell To Split A String Into An Array
Javascript Capitalize First Letter Of Each Word In A String
The String Split Function In Sql Server
How To Get Character Array From String In Javascript
Learn Javascript How To Split String Into An Array
Convert Comma Separated String To Array Using Javascript
How To Convert Strings To An Array Using Javascript Split
Split Method To Create Array By Breaking String Using
Splice Slice Amp Split What S The Difference By Maria
4 Examples To Understand Java String Split Method With Regex
Javascript String Split Tutorial
Split Method To Create Array By Breaking String Using
4 Ways To Convert String To Character Array In Javascript
Javascript String Split How To Split String In Javascript
Javascript Split A String And Convert It Into An Array Of
How To Use The Javascript Split Method To Split Strings And
0 Response to "26 Javascript Split String In Array"
Post a Comment