21 Javascript Import Class Es6
Summary: in this tutorial, you will learn about ES6 modules and how to export variables, functions, classes from a module, and reuse them in other modules. An ES6 module is a JavaScript file that executes in strict mode only. It means that any variables or functions declared in the module won't be added automatically to the global scope. 1 Answer1. Active Oldest Votes. 2. module.exports. syntax is from Modules (which are largely used in NodeJs - the counterpart of it is require rather than import). If you want to use import, you need to use export clause, which is from es6 modules. export default PageLoader. you could also do named exports.
Javascript Es6 Classes Objects In Programming Languages
How to import and export javascript ES6 classes. 921. August 19, 2017, at 08:29 AM. I am new to javascript and nodejs and I'm using this project to develop my skill and learn the new technologies. Currently my project uses multiple classes that depend on one another. The class files are in different directories and I am currently trying to use ...
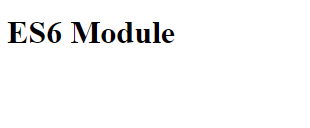
Javascript import class es6. Introduction to ES6 import: The import statement is used to import modules that are exported by some other module. A module is a file that contains a piece of reusable code. The import modules are in strict mode whether it is declared or not. Syntax of import: import name from 'module-name' Importing can be done in various ways: I am experimenting with JavaScript ES6 import and export and wanted to achieve the following: Use the import / export style of including JavaScript files. Import a class file and create an instance. Import a class file that extends another class. Expose functions to the index.html scope - call from an inline onclick event. Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics.
JavaScript ES6: Classes. Objects in programming languages provide us with an easy way to model data. Let's say we have an object called user. The user object has properties: values that contain ... Apr 16, 2018 - es6 export class In this tutorial we are going to learn about modules and exports and what does this mean in your application and how will this change the way we think about javascript applications. This is the first time we have modules natively in javascript and it has been one of the most awaited features in the new release of EcmaScript 6.
When exporting a module using export ... used to import the module. ... Depending on the module target specified during compilation, the compiler will generate appropriate code for Node.js (CommonJS), require.js (AMD), UMD, SystemJS, or ECMAScript 2015 native modules (ES6) module-loading ... Create a directory, navigate and initialize your project using NPM by issuing the below command. $ mkdir es6-app. $ cd es6-app. $ npm init. Fill in your details. Refer the below image for more info. Package.json configuration. You can leave fields empty and hit enter when you don't have values to fill. This is just a reference and let's ... In this section, we introduced JavaScript classes and ES6 modules. We discussed the prototype-based inheritance structure and demonstrated the basics of class creation and JavaScript class inheritance. When discussing modules, we first showed how to create a module and export the functions and variables stored within them.
Sep 05, 2020 - With ES2015 (ES6), with get built-in support for modules in JavaScript. Like with CommonJS, each file is its own module. To make objects, functions, classes or variables available to the outside world it’s as simple as exporting them and then importing them where needed in other files. Basically the import and export syntax is used everywhere where we write JavaScript and then transcompile and bundle it to "old-school" javascript. But the time when it can only be used in conjunction with compilers like Babel is over. Meanwhile Node.js also supports the so-called ES6 modules, and in the browser we can use them if we want to. Jul 31, 2020 - Entenda como funciona a feature mais desejada do JavaScript
Jul 19, 2017 - There are 4 types of exports: · 1— Named exports (several per module) 2— Default exports (one per module) 3 — Mixed named & default exports 4— Cyclical Dependencies A functional component is just a regular JavaScript function. A class component is a class that extends the class Component from the react library. Hooks allow functional components to use states and lifecycle methods. Functional components can use states using the setState hook. ECMAScript 6 introduces the concept of class available in traditional object-oriented languages. In ECMAScript 6, the class syntax is syntactical sugar on top of the existing prototype-based inheritance model. It does not add a new object-oriented inheritance model to JavaScript.
The For/Of Loop. The JavaScript for/of statement loops through the values of an iterable objects. for/of lets you loop over data structures that are iterable such as Arrays, Strings, Maps, NodeLists, and more. The for/of loop has the following syntax: for ( variable of iterable) {. // code block to be executed. The static import statement is used to import read only live bindings which are exported by another module.. Imported modules are in strict mode whether you declare them as such or not. The import statement cannot be used in embedded scripts unless such script has a type="module".Bindings imported are called live bindings because they are updated by the module that exported the binding. The export statement is used when creating JavaScript modules to export live bindings to functions, objects, or primitive values from the module so they can be used by other programs with the import statement. The value of an imported binding is subject to change in the module that exports it. When a module updates the value of a binding that it exports, the update will be visible in its ...
You can export functions, var, let, const, and — as we'll see later — classes.They need to be top-level items; you can't use export inside a function, for example.. A more convenient way of exporting all the items you want to export is to use a single export statement at the end of your module file, followed by a comma-separated list of the features you want to export wrapped in curly braces. Mar 06, 2020 - I’ll start out going through ... same thing using ES6 ‘import’ statements. ... Using ES5 syntax in Node means that the sharing of code between files is done with the ‘require’ and ‘module.exports’ statements. A ‘module’ in Javascript can be thought of as ... This video introduces Object-Oriented Programming in JavaScript with ES6 classes and the p5.js library.Next Video: https://youtu.be/rHiSsgFRgx4Support this c...
ES6 Class Syntax. Here is how you would create the exact same class in JavaScript using ES6 syntax: //class declaration class Person {//a Constructor method //this is called when the class is instantiated (with `new`) //and has the job of initializing the attributes constructor (newName, newAge) {//assign parameters to the attributes this. firstName = newName; this. age = newAge;} //return ... Using JavaScript ES6 import/export modules in Chrome Extensions. ... JavaScript in ECMAScript 6 supports modules which you may now use in Google Chrome since version 61. How to use ES6 modules in your browser. You can include a module in your HTML by adding a script with type="module": If you watched [episode 2][javascript_es6] of our JavaScript series, then you know that ECMAScript is the official name of the JavaScript language standard and that ECMAScript version 6 - or ES6 - introduced the idea of *modules*. Modules are what we've been taking advantage of this *entire* tu...
Note that there is no semicolon ... or a class (which are anonymous declarations). ... Even though JavaScript never had built-in modules, the community has converged on a simple style of modules, which is supported by libraries in ES5 and earlier. This style has also been adopted by ES6... Mar 31, 2017 - Join Stack Overflow to learn, share knowledge, and build your career · Find centralized, trusted content and collaborate around the technologies you use most Mar 02, 2019 - The recent updates to the JavaScript programming language, in the form of ES6, specify changes to the language, adding things like new class syntax and a module system. This module system is different from Node.js modules. A module in ES6 looks like the following:
Jun 22, 2016 - Our next three lines, we instantiate our classes, call our function, and log our constant. ... We have successfully mastered imports and exports with ES6! Learning OOP JavaScript-ES6 will significantly help you become a better developer. Concepts such as the class declaration, constructors, getter and setter, methods, static properties, static method, and inheritance can definitely help you leverage those concepts when working with other JavaScript frameworks or libraries. The ES6 is a JavaScript standard. With the help of ES6, we can create modules in JavaScript. In a module, there can be classes, functions, variables, and objects as well. To make all these available in another file, we can use export and import. The export and import are the keywords used for exporting and importing one or more members in a module.
Export default. In practice, there are mainly two kinds of modules. Modules that contain a library, pack of functions, like say.js above.; Modules that declare a single entity, e.g. a module user.js exports only class User.; Mostly, the second approach is preferred, so that every "thing" resides in its own module. ES6 入门教程. 《ECMAScript 6 入门教程》是一本开源的 JavaScript 语言教程,全面介绍 ECMAScript 6 新引入的语法特性。. 本书覆盖 ES6 与上一个版本 ES5 的所有不同之处,对涉及的语法知识给予详细介绍,并给出大量简洁易懂的示例代码。. 本书为中级难度,适合已经 ... Jan 28, 2020 - One of the major challenges when building a web-app is how quickly you can scale and respond to the market needs. When the demand (requirements) increases, the capabilities (features) increase too. It is therefore important to have a solid architectural structure so that the app grows organically.
I am experimenting with JavaScript ES6 import and export and wanted to achieve the following: Use the import / export style of including JavaScript files. Import a class file and create an instance; Import a class file that extends another class; Expose functions to the index.html scope - call from an inline onclick event 11/1/2016 · export default class Data {} If that's the case, then the importer can call the variables whatever they like: import Data1 from 'component/Data.js'; import Data2 from 'actions/Data.js'; If they are named exports: export class Data {} Then you need to use braces along with as to specify the source and target names: Access over 7,500 Programming & Development eBooks and videos to advance your IT skills. Enjoy unlimited access to over 100 new titles every month on the latest technologies and trends
ES6 - export & import, Để dễ làm việc, quản lý các chức năng file javascript, chúng ta thường tách các phần riêng biệt ra riêng từng file khác nhau, khi đó nhu cầu lồng ghép các function của file này vào file kia để sử dụng các function đã có là khó tránh khỏi. Re: How to import a javascript class and extend it in another script unit As a kind of fun example of what all this looks like in TestComplete, I've attached a little "story". Put all 5 files in a JavaScript project in TestComplete and run the "battle" function in BridgeOfKhazadum.js. En Javascript se utiliza una sintaxis muy similar a otros lenguajes como, por ejemplo, Java. Declarar una clase es tan sencillo como escribir lo siguiente: // Declaración de una clase class Animal {} // Crear o instanciar un objeto const pato = new Animal (); El nombre elegido debería hacer referencia a la información que va a contener dicha ...
import { myFunction } from './path/to/test.js'; All of this said, one thing you need to keep in mind is native browsers do not have the ability to import JavaScript modules yet. So to get around this problem, we need to use something like Webpack to provide the ability to import and export modules using ES6. https://webpack.github.io/. The import and export statements are one of the most crucial features of JavaScript ES6. It allows you to import and export JavaScript classes, functions, components, constants, any other variables between one JavaScript file and another. This feature allows you to organize your JavaScript code into small, bite-size files.
Es6 Adventures Imports Are Singleton Instances
Visual Studio 2015 Javascript Es6 Not Working Stack Overflow
Es6 Import Atmosphere Packages Not Working Help
Javascript Programming With Visual Studio Code
A Practical Guide To Es6 Modules
Modern Object Oriented Javascript With Es6 Capgemini Worldwide
Es6 Modules With Traceur Js Vegibit
Module Cheatsheet Samanthaming Com
Shipping Es6 In Browsers Without Polyfill By Arnav Yagnik
Javascript Es8 Asynchronous Await Service Es6 Modules By
Comparing React With Es5 Vs React With Es6 Dzone Web Dev
Es6 Modules And How They Work Dev Community
Javascript Es6 Classes Objects In Programming Languages
A Comprehensive Look At Es6 Modules
Import A Class From One Js To Another Js File Code Example
Import And Export Statements In Javascript And How To Use Them
Javascript Modules With Import Export Syntax Es6
0 Response to "21 Javascript Import Class Es6"
Post a Comment