29 Iterate Object Array In Javascript
Apr 02, 2018 - Sometimes we may need to retrieve multiple pairs of data from an object. This article will briefly describe three ways that we can accomplish this task using array looping methods. The three ways I… It is possible in JavaScript to convert an array-like object into an array. Once you do that, you could then loop over your collection simply as you would with an array using any loop.
How To Iterate A Foreach Over An Object Array Key Values From
From the classic forloop to the forEach () method, various techniques and methods are used to iterate through datasets in JavaScript.
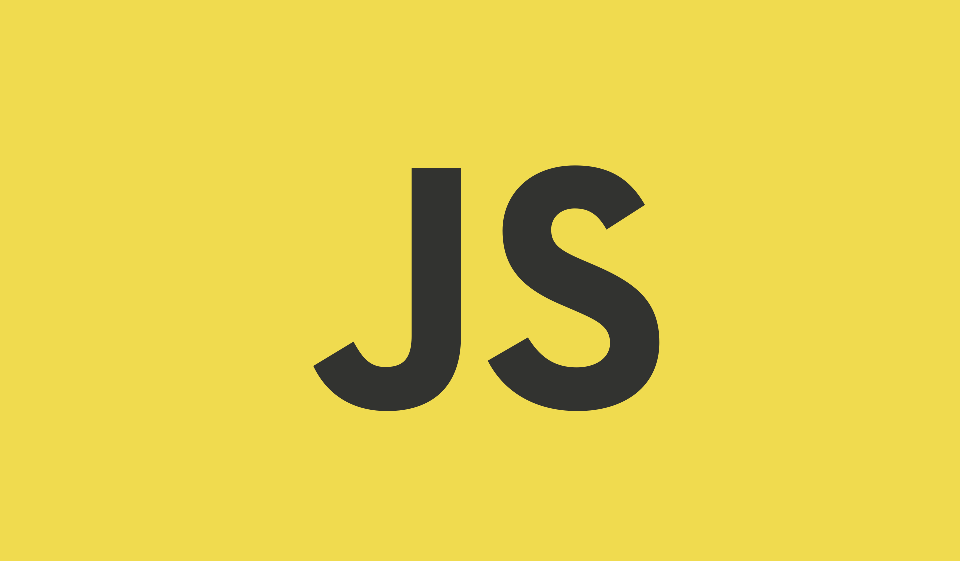
Iterate object array in javascript. forEach () The forEach () is a method of the array that uses a callback function to include any custom logic to the iteration. The forEach () will execute the provided callback function once for each array element. The callback function takes up 3 arguments: 1 week ago - Note: for...in should not be used to iterate over an Array where the index order is important. Array indexes are just enumerable properties with integer names and are otherwise identical to general object properties. There is no guarantee that for...in will return the indexes in any particular ... An object is deemed iterable if it has an implementation for the Symbol.iterator property. Some built-in types like Array, Map, Set, String, Int32Array, Uint32Array, etc. have their Symbol.iterator property already implemented. Symbol.iterator function on an object is responsible for returning ...
Arrays of objects don't stay the same all the time. We almost always need to manipulate them. So let's take a look at how we can add objects to an already existing array. Add a new object at the start - Array.unshift. To add an object at the first position, use Array.unshift. Javascript arrays are just a specific kind of object with some handy properties that help you treat them as arrays, but they still have internal object properties and you don't mean to iterate ... The filter () method creates a new array with array elements that passes a test. This example creates a new array from elements with a value larger than 18: Example. const numbers = [45, 4, 9, 16, 25]; const over18 = numbers.filter(myFunction); function myFunction (value, index, array) {. return value > 18; }
Here's a very common task: iterating over an object properties, in JavaScript Published Nov 02, 2019 , Last Updated Apr 05, 2020 If you have an object, you can't just iterate it using map() , forEach() or a for..of loop. The common ways to iterate over objects in Javascript are: The easiest way, use a for-of loop to run through the object. for (let KEY of OBJECT) { ... } Use Object.keys () to extract the keys into an array and loop through it. for (let i of keys) { ... } Use Object.values () to extract the values and loop through. Before clicking the button: After clicking the button: Method 2: Object.entries() map: The Object.entries() method is used to return an array of the object's own enumerable string-keyed property pairs. The returned array is used with the map() method to extract the key and value from the pairs. The key and values from the key-value pair can be extracted by accessing the first and second ...
There are multiple ways one can iterate over an array in Javascript. The most useful ones are mentioned below. Using for loop. Using while loop. This is again similar to other languages. using forEach method. The forEach method calls the provided function once for every array element in the order. Comparing the different ways to iterate over an array As you can see using a for loop with length caching is the fastest way to iterate over an array. However, this depends on the browser (if you are running it in a browser), your system, etc. Loop through object array with javascript. 1. javascript Iterate Object Array-2. Iterate through an array of objects in JavaScript. 0. how to iterate the below array in jquery-2. Multiline object in JavaScript. How to loop through it? See more linked questions. Related. 7626.
In JavaScript, you'll often need to iterate through an array collection and execute a callback method for each iteration. And there's a helpful method JS devs typically use to do this: the forEach () method. The forEach () method calls a specified callback function once for every element it iterates over inside an array. Object.entries() returns an array whose elements are arrays corresponding to the enumerable string-keyed property [key, value] pairs found directly upon object. The ordering of the properties is the same as that given by looping over the property values of the object manually. The Object.keys () method was introduced in ES6 to make it easier to loop over objects. It takes the object that you want to loop over as an argument and returns an array containing all properties names (or keys). After which you can use any of the array looping methods, such as forEach (), to iterate through the array and retrieve the value of ...
22/7/2021 · To iterate through an array of objects in JavaScript, you can use the forEach () method aong with the for...in loop. Here is an example that demonstrates how you can loop over an array containing objects and print each object's properties in JavaScript: May 29, 2020 - JavaScript's Array#forEach() function lets you iterate over an array, but not over an object. But you can iterate over a JavaScript object using forEach() if you transform the object into an array first, using Object.keys(), Object.values(), or Object.entries(). In this tutorial, we are going to learn different ways to loop or iterate through an array of objects in JavaScript. First way: ForEach method Let's use es6 provided forEach() method which helps us to iterate over the array of objects:
Feb 22, 2018 - Not the answer you're looking for? Browse other questions tagged javascript arrays iteration or ask your own question. ... The full data set for the 2021 Developer Survey now available! Podcast 371: Exploring the magic of instant python refactoring with Sourcery 26/10/2017 · The JavaScript Loop is used to iterate through an array of items (which can be a number array, string array, etc) or objects. There are many ways to do it and so in this tutorial we will look on them one by one. Here I have taken an array of numbers and I will do the JavaScript Loop through array by … 19 hours ago - There is a classic JavaScript for ... methods. And don't forget a JavaScript object is just a special array and you can iterate over its members as well. You will learn different ways to loop or iterate JavaScript arrays, including how to use associative arrays or objects to ...
3 weeks ago - The forEach() method executes a provided function once for each array element. JS Examples JS HTML DOM JS HTML Input JS HTML Objects JS HTML Events JS Browser JS Editor JS Exercises JS Quiz JS Certificate ... Array iteration methods operate on every array item. The Object.keys () method was introduced in ES6. It takes the object that you want to iterate over as an argument and returns an array containing all properties names (or keys). You can then use any of the array looping methods, such as forEach (), to iterate through the array and retrieve the value of each property. Here is an example:
Jul 20, 2018 - Once in a while, you may need to loop through Objects in JavaScript. The only way to do so before ES6 is with a `for...in` loop. The problem with a `for...in` loop is that it iterates through properties in the Prototype chain. When you loop through an object with the `for...in` loop, you need to An alternative to for and for/in loops isArray.prototype.forEach(). The forEach() runs a function on each indexed element in an array. Starting at index[0] a function will get called on index[0], index[1], index[2], etc… forEach() will let you loop through an array nearly the same way as a for loop: Currently, the ES6 specification has the DRAFT status, but it should introduce the JavaScript iterators. In object-oriented computer programming, an iterator is an object that enables a programmer to traverse a container, particularly lists. An iterator is an object that defines the next () method.
Array.prototype [@@iterator] () The @@iterator method is part of The iterable protocol, that defines how to synchronously iterate over a sequence of values. The initial value of the @@iterator property is the same function object as the initial value of the values () property. array.every() doesn't only make the code shorter. It is also optimal, because .every() method breaks iterating after finding the first odd number.. 8. Conclusion. array.forEach(callback) method is an efficient way to iterate over all array items. Its first argument is the callback function, which is invoked for every item in the array with 3 arguments: item, index, and the array itself. Object.keys() The Object.keys()takes an object and returns an arrayof the object's properties. By chaining the Object.keys()with the forEach()method, you can access the keys and values of an object. Note that the Object.keys()method was introduced in ES6.
In this tutorial, we are going to learn different ways to loop through an array of objects in JavaScript. First way: ForEach method. In es6 we have a forEach method which helps us to iterate over the array of objects. Iterating over objects. Combine for...in with hasOwnProperty (), in the manner described above. Combine Object.keys () or Object.getOwnPropertyNames () with forEach () array iteration. Iterate over the property (key,value) pairs of an object: Iterate over the keys, use each key to retrieve the corresponding value. Feb 14, 2020 - This data can come in the form of arrays, lists, maps, or other objects. Luckily, developers have at their disposal a variety of tools applicable to this exact problem. The JavaScript language, in particular, provides a diverse array of iteration devices. Below is a brief explanation of many ...
How to create a loop to loop through an array of objects and getting just the values without knowing property names ... Complete a function that takes in one parameter, an object. Your function should iterate over the object, and log the values to the console. In the same way, you can make a loop through an array of strings. 2. Making a loop through DOM elements. The For loop can also be used to alter colors. Consider a situation where you want to choose a particular color for all the anchors of your page. In order to do so, use the following piece of code. iterate over array of object javascript and access the properties. Andrew Ng. Code: Javascript. 2021-06-11 01:40:39. for ( let item of items) { console .log (item); // Will display contents of the object inside the array } -1.
Iterating Over Arrays The article describes ES5 and ES6 approaches to iterate over javascript arrays and array-like objects! There are three ways to iterate through an array: The Array.prototype.forEach method; The for loop; The for..in statement. Additionally, two new methods are expected with the introduction of the new ECMAScript 6 standard: The for…of statement; The …
Iterate Javascript Object With Arrays In Python Stack Overflow
Exploring The Javascript Foreach Method For Looping Over Arrays
Javascript Loop Iterate Through An Array Or An Object
How To Loop Through The Array Of Json Objects In Javascript
How To Create Array Of Objects In Java Geeksforgeeks
Looping Over Objects Javascript Tutorial
How To Properly Iterate Through Nested Arrays With Javascript
Loop And Array In Typescript With Examples Codez Up
For Each In Javascript With Example Phpcoder Tech
Different Ways To Loop Through Arrays And Objects In React
How To Iterate Over Object Properties In Javascript
A Guide To Es6 Iterators In Javascript With Examples
Traverse Array Object Using Javascript Javatpoint
Iterate Through Each Values From Object Datatype Learn
6 Ways To Loop Through An Array In Javascript Codespot
Iterating Through Object Literals In Javascript By Garrett
Looping Through Array Of Objects Typeerror Cannot Read
Javascript Loop Iterate Through An Array Or An Object
Javascript Foreach How To Iterate Array In Javascript
Pivot A Javascript Array Convert A Column To A Row Techbrij
How To Iterate Through Jsonarray In Javascript Crunchify
Js Looping And Iteration Traversing Learn Co
How To Loop Through Every Nested Objects In Array Javascript
Looping Through Object Properties In Vue
Javascript Array Of Objects Tutorial How To Create Update
How To Iterate Over A Javascript Object Geeksforgeeks
Dynamic Array In Javascript Using An Array Literal And
Javascript Map How To Use The Js Map Function Array Method
0 Response to "29 Iterate Object Array In Javascript"
Post a Comment