26 Convert String To Html Element Javascript
Use the innerHTML property to add the string as elements to the document. And you have to use the correct quotes in the string. Your string is not valid. Mar 20, 2017 - Today there's a little known (but standard) way for converting string to DOM with JavaScript: ContextualFragment. I've touched on DocumentFragment to create and store DOM nodes for performance in the past, but that post illustrated element creation via document.createElement:
How Browser Rendering Works Behind The Scenes Logrocket Blog
Say you have an HTML snippet and you would like to extract the plain text from the snippet without any of the HTML tags. This may come handy when you are sending mail through a program that doesn't support HTML Mail. The easiest way would be to strip all the HTML tags using the replace() method of JavaScript.
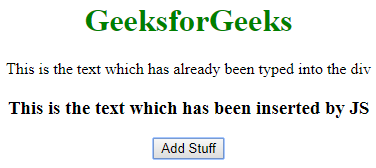
Convert string to html element javascript. There are many ways to convert Set to String with space separatro in javascript. set is a datastructure introduced in latest javascript let's declare set. var set = new Set (); set.add ('first'); set.add ('second'); set.add ('three'); if we want to return string from a set,It is not striaignforward. normally we will use below approaches to ... The replace() RegExp method replaces the specified string with another string. The method takes two parameters the first one is the string that should be replaced, and the second one is the string replacing from the first string. The second string can be given an empty string so that the text to be replaced is removed. Finding HTML Elements. Often, with JavaScript, you want to manipulate HTML elements. To do so, you have to find the elements first. There are several ways to do this: Finding HTML elements by id; Finding HTML elements by tag name ; Finding HTML elements by class name; Finding HTML elements by CSS selectors; Finding HTML elements by HTML object ...
Nov 06, 2015 - Free source code and tutorials for Software developers and Architects.; Updated: 8 Oct 2015 1. Using .replace(/<[^>]*>/g, '') This method is a simple and efficient way to remove the tags from the text. This method uses the string method .replace(old value, new value) which replaces the HTML tag values with the empty string.The /g is used for it to happen globally (every value found in the string is replaced with the specified if the /g is used). Convert HTML string to DOM element / Checkout Revealing the magic: How to properly convert HTML string to a DOM element article. I'm working on a chrome extension which accepts user text, work with it and output the result in a div. I used jQuery in the beginning, for several tasks, but later I decided to remove it and deal with pure javascript/DOM manipulations. The output div was filled by ...
Sometimes, you need to convert a JavaScript object to a plain string that is useful for storing object data in a database. In this tutorial, we will suggest two methods for converting an object to a string. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Oct 08, 2020 - Is there a way to convert HTML like: or ... DOM element so that I could pass it in a .appendChild().
The HTML DOM allows JavaScript to change the content of HTML elements. Changing HTML Content The easiest way to modify the content of an HTML element is by using the innerHTML property. Javascript strip HTML from a string. If you are running in a browser, it is best to use DOMParser: function strip (html) { let doc = new DOMParser ().parseFromString (html, 'text/html'); return doc.body.textContent || ""; } If you are running in browser to test the inline JavaScript, use the following code: The outerHTML attribute of the Element DOM interface gets the serialized HTML fragment describing the element including its descendants. It can also be set to replace the element with nodes parsed from the given string. To only obtain the HTML representation of the contents of an element, or to replace the contents of an element, use the innerHTML property instead.
The DOMParser () object creates a new DOM document from a string. To use it, you instantiate a new instance. Then you use the parseFromString () method to convert your string into a new document element. The method accepts the string as it's first argument. JAVASCRIPT,DOMPARSER,DOCUMENT.CREATEELEMENT,HTML,DOM.In some cases, one would want to convert a HTML string to the DOM elements so that JavaScript can handle them easily. This is frequently used when one get some data from third party APIs where the datPixelstech, this page is to provide vistors information of the most updated technology information around the world. convert a string to html element in js. javascript by Wandering Wolf on May 28 2020 Comment. 4. /** * Convert a template string into HTML DOM nodes * @param {String} str The template string * @return {Node} The template HTML */ var stringToHTML = function (str) { var parser = new DOMParser (); var doc = parser.parseFromString (str, 'text/html ...
Read this JavaScript tutorial and find the method that is used for creating a new DOM element from an HTML string. Get the explanation and see examples. ... This allows to convert an HTML string to DOM elements by setting the innerHTML of a <template> element, then reach into the .content property. html javascript HTML5 dom function strings. A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Given an HTML document, the task is to get the entire document as a string using JavaScript. Here few methods are discussed: getElementsByTagName() Method This method returns a set of all elements in the document with the defined tag name, as a NodeList object.
Jun 04, 2021 - Contribute your code and comments through Disqus. Return Value: Returns the converted string. HTML elements such as span, div etc. Read this JavaScript tutorial and find the method that is used for creating a new DOM element from an HTML string. element â Required. Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. 2 weeks ago - The DOMParser interface provides ... from a string into a DOM Document. You can perform the opposite operation—converting a DOM tree into XML or HTML source—using the XMLSerializer interface. In the case of an HTML document, you can also replace portions of the DOM with new DOM trees built from HTML by setting the value of the Element.innerHTML ...
Given an HTML document, the task is to get the entire document as a string using JavaScript. Here few methods are discussed: getElementsByTagName () Method. This method returns a set of all elements in the document with the defined tag name, as a NodeList object. This object represents a collection of nodes, Which are accessed by index numbers. Convert HTML string to element. Javascript Forums on Bytes. I want to use element.appendChild(child) on a string of HTML I have: html= The document.createRange().createContextualFragment function is an awesome, sane method for converting strings to DOM nodes within JavaScript. Ditch your old shims and switch to this performant, simple API! The Original Post: DOMParser. Today we have a standard way for converting string to DOM with JavaScript: DOMParser. The JavaScript
Create a temporary DOM element and retrieve the text This is the preferred (and recommended) way to strip the HTML from a string with Javascript. The content of a temporary div element, will be the providen HTML string to strip, then from the div element return the innerText property: using the xpath string. xpath is a string that specifies we match the div elements that contains the text 'bar'. Then we can use the xpath string to search for the element we want by calling the document.evaluate method with the xpath as the first argument. document is the 2nd argument which means we're search the whole document for the ... JSfiddle link I am building a string and appending it to an html element using html () rather than text () function. This will be treated as a HTML tag by browser and diplay the changes in the UI as seen in the fiddle.
Hi There! I use slim and just started to learn a basic REST API. I am a stuck on how I could transform my JSON Response into a value inside HTML Page. Here is the api endpoint example and the response. So the question is, How can I use that json data to put into my HTML element. For instance inside a or a table or whatever. Thanks and I wish someone could give a a clue on it 🙂 Another way to replace the text inside a div element with JavaScript is to set the textContent property of an element to a different value. If we have the following HTML: const div = document.querySelector ('div') div.textContent = "My new text!"; to select the div with querySelector . And then we can set the textContent to a new string. This article is going to tell and guide the users to convert a div element into an image using AngularJS. The user will be generating an image from the webpage and also be able to convert a particular part of the HTML page into the picture. Also, the user needs an HTML tag and html2canvas JavaScript library. By using this, we can create the ...
There are two ways to append HTML code to a div through JavaScript. Using the innerHTML attribute. Using the insertAdjacentHTML () method. Using the innerHTML attribute: To append using the innerHTML attribute, first select the element (div) where you want to append the code. Then, add the code enclosed as strings using the += operator on ... Jul 11, 2013 - Convert HTML string to DOM element ... properly convert HTML string to a DOM element article. I'm working on a chrome extension which accepts user text, work with it and output the result in a div. I used jQuery in the beginning, for several tasks, but later I decided to remove it and deal with pure javascript/DOM ... But now seems to be a good time in almost all browsers if you want to convert a string into DOM elements with just JavaScript code and no extra libraries. Meet the DOMParser. Yeah, DOMParser class is your guy. All you need to do is to create a new instance of this class and call the parseFromString
Mar 21, 2020 - Say you have some HTML that is a string: let string_of_html = ` Cool `; Maybe it comes from an API or you've constructed it yourself from getElementsByName () - This method is also a good choice as any element in an HTML document can be assigned a unique name value. If there are multiple elements with the same name, you can select a specific element by it's index value (order value) on the page. getElementsByTagName () - This method isn't that great of a choice unless you ... jQuery.parseHTML uses native methods to convert the string to a set of DOM nodes, which can then be inserted into the document. These methods do render all trailing or leading text (even if that's just whitespace). To prevent trailing/leading whitespace from being converted to text nodes you can pass the HTML string through jQuery.trim.
Aug 13, 2019 - Yesterday, I wrote about Reef, my lightweight alternative to Vue and React. Over the next few days, I want to take a look at how various parts of it work under-the-hood. Today, we’re going to look at how to take an HTML string and convert it into actual markup.
Convert Html Css Content To A Sleek Multiple Page Pdf File
How To Convert String To Float Number In Javascript
Translating A Json Data To Html Element Javascript The
How To Convert Comma Separated String Into An Array In
Converting Strings Into Hypertext Markup Language Html Php
Github Peternewnham React Html Parser Converts Html
Javascript Remove Html From Text Code Example
Jsoup Html Parsing Library For Java Developers
6 Ways To Convert String To Array In Javascript
Easy Way To Convert Html To Pdf Using Javascript Qa With
How To Convert A String To A Number In Javascript
How To Get The Entire Html Document As A String In Javascript
How To Append Html Code To A Div Using Javascript
Translate Css Cascading Style Sheets Mdn
How To Export Generate Convert Html Css To Pdf Using Javascript
Javascript Convert Text Case From Uppercase To Lowercase
How To Convert Html Form Field Values To A Json Object
Convert Html Inline Styles To A Style Object For React
Render Html String As Real Html In A React Component Stack
3 Different Ways To Convert Html Into Plain Text By
How To Convert String Into A Html Element In Next Js
Jquery Object To String Learn How To Convert The Object To
Js Convert Html In String Code Example
Parsing Html In Dart With Html Package By Jonathan Monga
Does React Escape Html Tags And New Line Breaks In A String
0 Response to "26 Convert String To Html Element Javascript"
Post a Comment