26 Javascript Map Key Index
Ther e are two main differences between Maps and regular JavaScript objects. 1. Unrestricted Keys. Each key in an ordinary JavaScript object must be either a String or a Symbol . The object below ... Apr 03, 2017 - Getting value out of maps that are treated like collections is always something I have to remind myself how to do properly. In this post I look at JavaScript object iteration and picking out values from a JavaScript object by property name or index.
Policy Responses To The Coronavirus Pandemic Statistics And
Jul 27, 2021 - Object.entries(obj) – returns an array of [key, value] pairs. Please note the distinctions (compared to map for example):
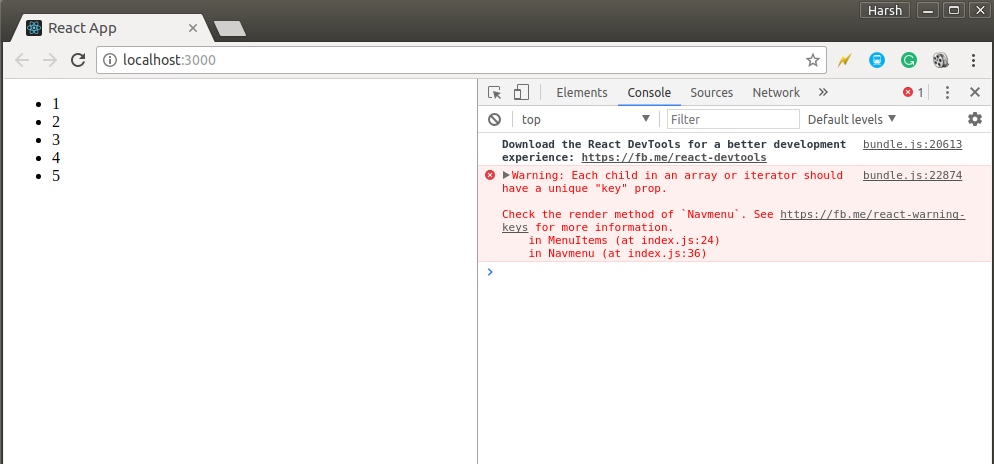
Javascript map key index. Map.prototype.get () The get () method returns a specified element from a Map object. If the value that is associated to the provided key is an object, then you will get a reference to that object and any change made to that object will effectively modify it inside the Map object. The source for this interactive example is stored in a GitHub ... Map. Map is a collection of keyed data items, just like an Object. But the main difference is that Map allows keys of any type. Methods and properties are: new Map () - creates the map. map.set (key, value) - stores the value by the key. map.get (key) - returns the value by the key, undefined if key doesn't exist in map. How do you select a particular option in a SELECT element in jQuery · Subquery returned more than 1 value. This is not permitted when the subquery follows =, !=, <, <= , >, >= or when the subquery is used as an expression · How can I select all elements without a given class in jQuery
22/11/2019 · In JavaScript, map () method handles array elements, which creates a new array with the help of results obtained from calling function for each and every array element in an array. The index is used inside map () method to state the position of each element in an array, but it doesn’t change the original array. Jun 20, 2018 - The map() method is used to apply a function on every element in an array. A new array is then returned. You can think of map() as a for loop, that is specifically for transforming values. Consider… Get first value from javascript Map. Map is a new key/value data structure in es6 (and its available in your chrome browser RIGHT NOW). But getting values out of a Map without using a key is complicated a little by having to go through a javascript iterator. > m = new Map([[1,"a"],[2,"b"]]) Map {1 => "a", 2 => "b"} > m.values()[0] undefined > m ...
When you don't have stable IDs for rendered items, you may use the item index as a key as a last resort: const todoItems = todos.map((todo, index) => <li key={index}> {todo.text} </li>); We don't recommend using indexes for keys if the order of items may change. This can negatively impact performance and may cause issues with component state. Feb 19, 2018 - This article is pointed towards beginners in Javascript or people who are just starting working with functional Javascript and never heard of map, filter and reduce. If you already have a lot of… The translation function that is provided to this method is called for each top-level element in the array or object and is passed two arguments: The element's value and its index or key within the array or object. ... Use $.map() to change the values of an array.
One Map to store key-value pairs, one Map to store key-index mappings, one array to store index-key mappings. Make each of the operations (set by key, remove by key, lookup by key, lookup by index) do the right thing to all three of those structures, based on information from the relevant one. This doesn't require language support. If you only need the keys or the values of the Map, you can use Map#keys () or Map#values (). Map#keys () returns an iterator over the map's keys, and Map#values () returns an iterator over the map's values. Make sure you convert the iterator to an array using the spread operator or Array.from () if you want to use filter () or map ()! A Map is backed by a Set of [key, value] entries, with contentEquals and contentHash overridden to only consider the key. Properties length. The number of items in this collection. Methods has(key) Returns whether an entry with the given key exists in a Map. get(key|index, default?) Gets the value for a key in a map. set(key, value) Sets the ...
Indexed Collections (Arrays) arrays is the indexed collection in javascript. Array is an ordered set of values that has a numeric index and value. For example, you could have an array called employees that contains employees' names indexed by their numerical employee number. // Map Map. prototype. forEach ((value, key, map) = => {} // Array Array. prototype. forEach ((item, index, array) = => {} This is a big advantage for Maps over Objects, as Objects need to be converted with keys() , values() , or entries() , and there is not an simple way to retrieve the properties of an Object without converting it. exchange value between 2 items in array javascript ... The value associated with each key will be an array consisting of all the elements that resulted in that return value when passed into the callback.
The Array.prototype.findIndex() method returns an index in the array if an element in the array satisfies the provided testing function; otherwise, it will return -1, which indicates that no element passed the test. It executes the callback function once for every index in the array until it finds the one where callback returns true. 25/4/2018 · Map is a data structure in JavaScript which allows storing of [key, value] pairs where any value can be either used as a key or value. The keys and values in the map collection may be of any type and if a value is added to the map collection using a key which already exists in … 27/3/2020 · Hashmaps offer the same key/value functionality and come native in JavaScript (ES6) in the form of the Map() object (not to be confused with Array.prototype.map()).
Get Key and Value by Index The last scenario is an infrequent iteration one where you may need to get a property by its index. For example, you may have a filtered list where you know there's a fixed result and you just want to grab a specific result - typically the first one. Jul 15, 2018 - This is because the spread operator is simpler than the map function. It simply loops through the array (or any iterable, really) from 0 to length and creates a new index key in the enclosing array with the value returned from the spreading array at the current index. Since JavaScript returns ... May 29, 2020 - exchange value between 2 items in array javascript ... The value associated with each key will be an array consisting of all the elements that resulted in that return value when passed into the callback.
callbackFn is invoked with three arguments: the value of the element, the index of the element, and the array object being mapped.. If a thisArg parameter is provided, it will be used as callback's this value. Otherwise, the value undefined will be used as its this value. The this value ultimately observable by callbackFn is determined according to the usual rules for determining the this seen ... The Array has a key (index) that is always a number from 0 to max value, while in a HashMap, you have control of the key, and it can be whatever you want: number, string, or symbol. Hash Function. The first step to implement a HashMap is to have a hash function. This function will map every key to its value. Dec 18, 2020 - The Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value.
By definition, a Map object holds key-value pairs where values of any type can be used as either keys or values. In addition, a Map object remembers the original insertion order of the keys. To create a new Map, you use the following syntax: let map = new Map ([iterable]); One of the most popular methods of iterating through datasets in JavaScript is the .map() method. .map() creates an array from calling a specific function on each item in the parent array. .map() is a non-mutating method that creates a new array inste map() map() to iterate over an array. Its callback function actually takes three arguments, though often only the first one is used (the other two are optional). These arguments are: currentValue - the current value processed in the array; index (optional) - the index of the current element being processed; array (optional) - the array map was ...
Map, reduce, and filter are all array methods in JavaScript. Each one will iterate over an array and perform a transformation or computation. Each will return a new array based on the result of the function. In this article, you will learn why and how to use each one. Here is a fun summary by Steven Luscher: Map/filter/reduce in a tweet: 30/7/2020 · JavaScript Map Index To find index of the current iterable inside the JavaScript map () function, you have to use the callback function’s second parameter. An index used inside the JavaScript map () method is to state the position of each item in an array, but it doesn’t modify the original array. This section shows you how to load the Maps JavaScript API into your web page, and how to write your own JavaScript that uses the API to add a map with a marker on it. The following code shows the CSS and JS inline in the HTML document.
The second argument to the callback function is the index of the item that we are currently processing. Taking our example array of [1, 2, 3], if we run [1, 2, 3].map ((value, index) => index) we'll see our callback get run with 0 the first time, 1 the second time, and 2 on the final time. The map () method in JavaScript creates an array by calling a specific function on each element present in the parent array. It is a non-mutating method. Generally map () method is used to iterate over an array and calling function on every element of array. 13/7/2016 · Other arguments of Array.prototype.map():. The third argument of the callback function exposes the array on which map was called upon; The second argument of Array.map() is a object which will be the this value for the callback function. Keep in mind that you have to use the regular function keyword in order to declare the callback since an arrow function doesn't have its own binding to the ...
Definition and Usage. The findIndex() method returns the index of the first array element that passes a test (provided by a function).. The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, findIndex() returns the index of that array element (and does not check the remaining values) I'm looking for a solution that allows me to have a collection of keys and values that are ordered & indexed... Arrays can't do keys and values and require looping to look for a certain "key". Objects aren't ordered and get jumbled up. Maps aren't indexed and require loops to get indexes. An API key is passed as the key parameter in the URL that is used to load the Maps JavaScript API. Here are a few options to check if you are using an API key: Use the Google Maps Platform API Checker Chrome extension. This allows you to determine if your website is properly implementing Google's licensed Maps APIs.
Definition and Usage. The map() method creates a new array with the results of calling a function for every array element.. The map() method calls the provided function once for each element in an array, in order.. map() does not execute the function for empty elements. map() does not change the original array. 1. The map accepts any key type. As presented above, if the object's key is not a string or symbol, JavaScript implicitly transforms it into a string. Contrary, the map accepts keys of any type: strings, numbers, boolean, symbols. Moreover, the map preserves the key type. That's the map's main benefit.
Leaflet Map With React Part I This Tutorial Is For
Google Map Using Bootstrap In Asp Net Mvc Part One
Checking If A Key Exists In A Javascript Object Stack Overflow
Using Javascript S Map Function The Map Function Simply
Javascript Map Index How To Use Index Inside Map
Javascript Array Methods How To Use Map And Reduce
Hash Table Hash Map Data Structure Interview Cake
Javascript Keyed And Indexed Collections Array Map And Set
Hacks For Creating Javascript Arrays
Javascript When You Add Or Subtract Form Items Dynamically
Javascript Map Vs Foreach What S The Difference Between
Quickstart Create A Search Index In Javascript Azure
Integrating Google Maps In Angular By Hassam Ali Medium
Javascript Hash Table Associative Array Hashing In Js
How To Remove Array Duplicates In Es6 Samanthaming Com
What Is A Hash Map Data Structure Javascript
Es6 Collections Using Map Set Weakmap Weakset Sitepoint
Javascript Map Vs Foreach What S The Difference Between
Javascript Map Vs Foreach What S The Difference Between
Understanding Map And Set Objects In Javascript Digitalocean
Mapped The State Of Press Freedom Around The World Visual
Javascript Array Map How To Map Array In Javascript
Hash Table Hash Map Data Structure Interview Cake
0 Response to "26 Javascript Map Key Index"
Post a Comment