29 Javascript Send Data To Server
The XMLHttpRequest object is used to request data from a server. Send a Request To a Server To send a request to a server, we use the open () and send () methods of the XMLHttpRequest object: xhttp. open ("GET", "ajax_info.txt", true); XMLHttpRequest.send () The XMLHttpRequest method send () sends the request to the server. If the request is asynchronous (which is the default), this method returns as soon as the request is sent and the result is delivered using events. If the request is synchronous, this method doesn't return until the response has arrived.
Fetching Data From The Server Learn Web Development Mdn
Pass data from Javascript to Servlet through AJAX Another case is when you explicitly call a servlet method through javascript, the scenarios for this case are very common in every real application: e.g. filling a data table/grid with dynamic data retrieved from the database, handling click events.. and many more scenarios.
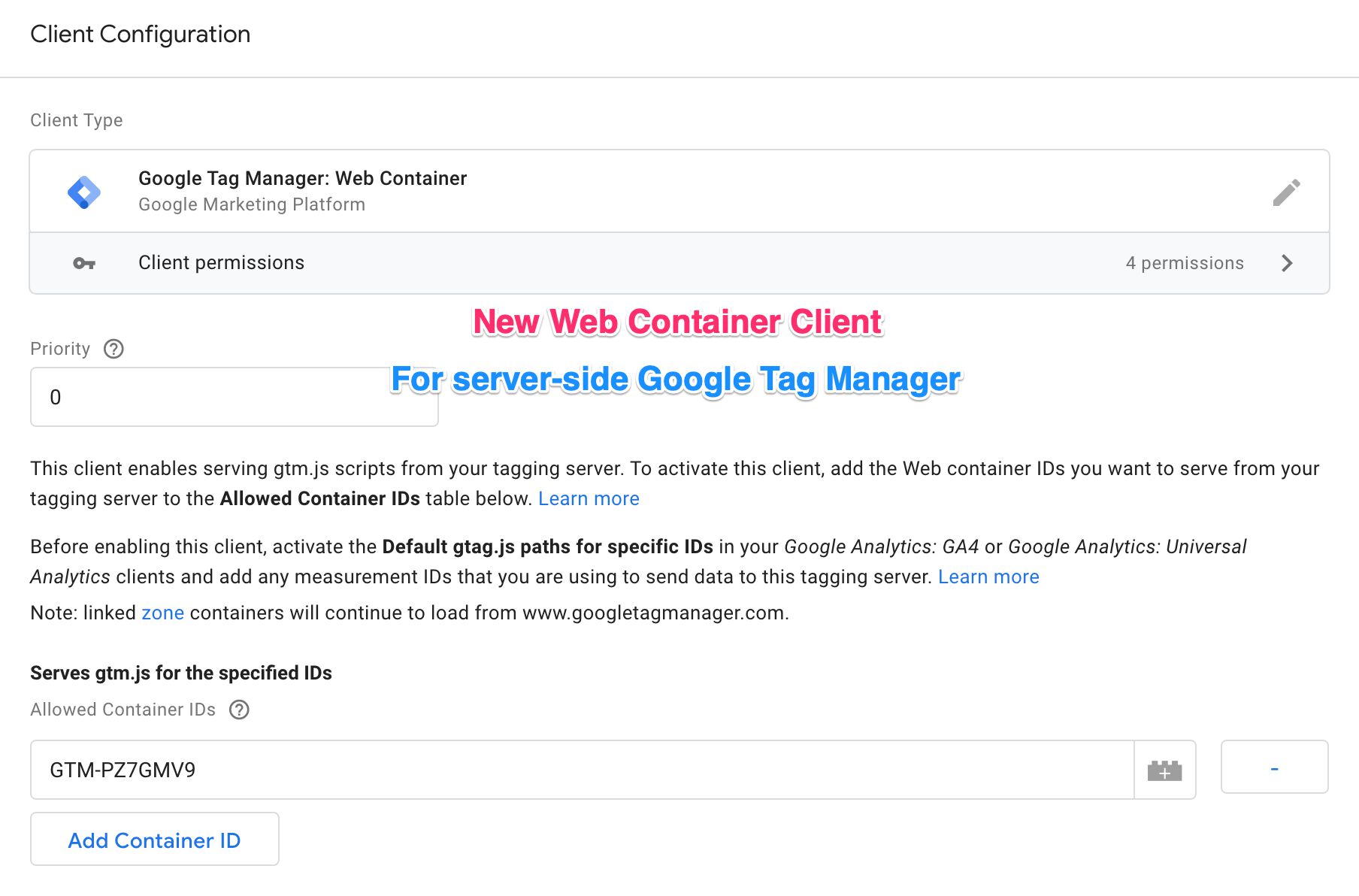
Javascript send data to server. In this article, we will learn how to send mail using Simple Mail Transfer Protocol which is free JavaScript library. It is basically used to send emails, so it only works for outgoing emails. To be able to send emails, you need to provide the correct SMTP server when you set up your email client. This can be done by Ajax request, we are sending data to our node server, and it also gives back data in response to our Ajax request. Step 1: Initialize the node modules and create the package.json file using the following command. npm init. Step 2: Install express module locally into your system by using the following command. JavaScript: When sending data to a web server, the data has to be a string. So we are using JSON.stringify () function to convert data to string and send it via XHR request to the server. Below is the sample code.
Create a JavaScript object using the standard or literal syntax. Use JSON.stringify () to convert the JavaScript object into a JSON string. Send the URL-encoded JSON string to the server as part of the HTTP Request. This can be done using the HEAD, GET, or POST method by assigning the JSON string to a variable. Your server must handle any expected data format (to extract the fields properly). URL encoding is useful, but later you will see JSON or perhaps protobufs . It really doesn't matter which format you use as long as both the client and server agree. JavaScript has great modules and methods to make HTTP requests that can be used to send or receive data from a server side resource. In this article, we are going to look at a few popular ways to make HTTP requests in JavaScript. Ajax. Ajax is the traditional way to make an asynchronous HTTP request.
ESP32 Arduino web server: Sending data to JavaScript client via websocket. ESP32 / 14 Comments. In this tutorial we will check how to setup a HTTP web server on the ESP32, which will have a websocket endpoint and will serve a HTML page. The HTML page will run a simple JavaScript application that will connect to the server using websockets and ... WebSocket .send() method can send either text or binary data. A call socket.send(body) allows body in string or a binary format, including Blob, ArrayBuffer, etc. No settings required: just send it out in any format. When we receive the data, text always comes as string. And for binary data, we can choose between Blob and ArrayBuffer formats. SmtpJS - true email sending from JavaScript. SmtpJS is a free library you can use for sending emails from JavaScript. All you need is an SMTP server and a few manipulations to get things done. We'll use Mailtrap as the server because it is an actionable solution for email testing. Below is the flow you should follow:
The send method of the XMLHttpRequest has been extended to enable easy transmission of binary data by accepting an ArrayBuffer, Blob, or File object. The following example creates a text file on-the-fly and uses the POST method to send the "file" to the server. This example uses plain text, but you can imagine the data being a binary file instead. This JavaScript function is very simple, first, we search for the three inputs using ' getElementById ', once we have data from these three inputs, we post data to the server, to a handler called ' MailHandler.ashx ', the format used is JSON. Inside this article we will see how to encrypt data in javascript before sending to server. We will use Crypto js plugin. Submitting data to server in encrypted format is good practise to secure data at client side. Here, we will provide a zipped folder in which you will get Encryption.js and Encryption.php file. This package will be used to ...
JavaScript can send network requests to the server and load JSON. JS does this using something called AJAX. AJAX stands for Asynchronous JavaScript and XML. JS has an API, fetch, to GET(receive) and POST(send) information to the server. You can use fetch to GET JSON data in the following way −. Example Sending a form with Blob data. As we've seen in the chapter Fetch, it's easy to send dynamically generated binary data e.g. an image, as Blob.We can supply it directly as fetch parameter body.. In practice though, it's often convenient to send an image not separately, but as a part of the form, with additional fields, such as "name" and other metadata. Server-Side Code Example. For the example above to work, you need a server capable of sending data updates (like PHP or ASP). The server-side event stream syntax is simple. Set the "Content-Type" header to "text/event-stream". Now you can start sending event streams. Code in PHP (demo_sse.php):
It's the method the browser uses to talk to the server when asking for a response that takes into account the data provided in the body of the HTTP request: "Hey server, take a look at this data and send me back an appropriate result." If a form is sent using this method, the data is appended to the body of the HTTP request. 28/4/2018 · Send the data from an HTML form to a PHP script with vanilla JavaScript. Process the data in the PHP script and move the local files to an uploads/ directory on a server. Setup. As mentioned in the prerequisites, you must have a basic knowledge of PHP and local server environments. Special Consideration for Mobile Devices. There is one thing that you should keep in mind while sending data to server on page unload. unload event is not guaranteed to be called in a mobile device.For example, if a browser page is in the background state, and if the mobile OS decides to terminate the browser process, unload event will not be fired. But visibilitychange event is guaranteed to ...
JavaScript gives us a way to contact the web server (without reloading the page). This style of programming a page is typically called AJAX. AJAX technically stands for Asynchronous Javascript and XML, but don't worry about the name. Just think of it as a way to send or receive data from the server (without reloading the page). The XMLHttpRequest object is used to exchange data with a server. Send a Request To a Server To send a request to a server, we use the open () and send () methods of the XMLHttpRequest object: xhttp. open ("GET", "ajax_info.txt", true); When the user tries to send the data, the application takes control and transmits the data asynchronously in the background, updating only the parts of the UI that require changes. Sending arbitrary data asynchronously is generally called AJAX, which stands for "Asynchronous JavaScript And XML". How is it different?
3/7/2016 · You need to use AJAX to send the data to the server. Something like this: jQuery.ajax({ method: "POST", url: "save.php", data: { image: canvasToDataURLString } }) Then you will need some serverside code to save the image. Here is a PHP version modified from this answer: How to save a PNG image server-side, from a base64 data string 25/3/2018 · AJAX is a fancy term for the technique by which a web browser sends either an HTTP GET or an HTTP POST request to a server via Javascript. The server-side code would be no different from the examples above. This technique is useful when you don't want the browser to abandon the current page and load an entirely new page load in the browser. How to send data to a node.js server using client side javascript. 1043. July 23, 2017, at 5:12 PM ... From your original question, it seems to me you just want to send data back to your page's originating server (ignoring the different port requirement). That is the most common case. Otherwise,
The XMLHttpRequest is a raw browser object that is used to communicate with the server in pure JavaScript. You can send data to the server or receive data from the server using the XMLHttpRequest object without reloading the entire web page. The XMLHttpRequest is mainly used in AJAX programming. Here is a sneak peek. We have some JavaScript that makes an HTTP request to a service (ipinfo.io) that returns a whole bunch of data about your connection. Using JavaScript, we process all that returned data and surgically pinpoint the IP address that we so proudly display here. Clients get the date on connection and that's it! const server = net.createServer((socket) => { socket.end(`${new Date()}\n`); }); server.listen(59090); Things to note: Node allows you to send either a string or a Buffer object. If you send a string, Node will use the socket's current encoding setting to encode the string into bytes.
If you'll need to send data to a server, XMLHttpRequest is always a friend. Here is the server.js of our Server Sent Event, we will be sending out data to the client every 5 seconds with an ... 2/4/2020 · Now that our request is ready, it's time to send it. Remember, we want to send our data object to the API, so we'll be passing it as an argument to the request's send() method. However, we need to stringify it first for it to be properly handled by the server. Add this final line to our Promise body: request.send(JSON.stringify(data)); . These technologies allow web pages to directly handle making HTTP requests for specific resources available on a server and formatting the resulting data as needed before it is displayed. Note : In the early days, this general technique was known as Asynchronous JavaScript and XML ( Ajax ), because it tended to use XMLHttpRequest to request XML ...
How To Send A Json Object To A Server Using Javascript
An Introduction To Server Side Tagging
Javascript Fundamentals Fetching Data From A Server By
Here Are The Most Popular Ways To Make An Http Request In
How To Send Data From Website To Server Side Container
4 Reasons To Learn Machine Learning With Javascript Techtalks
How To Get Set The Value Of A Session Variable Using
Real Time Online Activity Monitor Example With Node Js And
Debugging In Visual Studio Code
Passing Net Server Side Data To Javascript Marius Schulz
Create Http Web Server In Node Js Complete Tutorial
Send Draft Js Data To A Server And Fetch It With React Hooks
Javascript Network Http Requests By Amitha Mahesh Medium
How To Make Ajax Request Javascript Code Example
Here Are The Most Popular Ways To Make An Http Request In
How To Send A Json Object To A Server Using Javascript
Javascript Fundamentals Fetching Data From A Server By
An Introduction To Server Side Tagging
Making Http Web Requests In Javascript
Sending Data From A Ruby On Rails Server To A Node Js Server
Building A Node Js Static File Server Files Over Http Using
Fetching Data From Node Backend In Mean Stack Javatpoint
How To Create Communication With Node Js Server To Client
Here Are The Most Popular Ways To Make An Http Request In
New Google Tag Manager Web Container Client For Server Side
0 Response to "29 Javascript Send Data To Server"
Post a Comment