31 Javascript Get Html Content
Some of the definitions are given below: getIframeContent (frameId): It is used to get the object reference of an iframe. contentWindow: It is a property which returns the window object of the iframe. contentWindow.document: It returns the document object of iframe window. contentWindow.document.body.innerHTML: It returns the HTML content of ... First, put on element that wraps the div in question, put an id attribute on the element and then use getElementById on it: once you've got the lement, just do 'e.innerHTML` to retrieve the HTML. <div><span>This is in bold</span></div> => <div id="wrap"><div><span>This is in bold</span></div></div>
Vanilla Javascript To Get Url Parameter Values Query String
Feb 20, 2021 - The textContent property of the Node interface represents the text content of the node and its descendants.
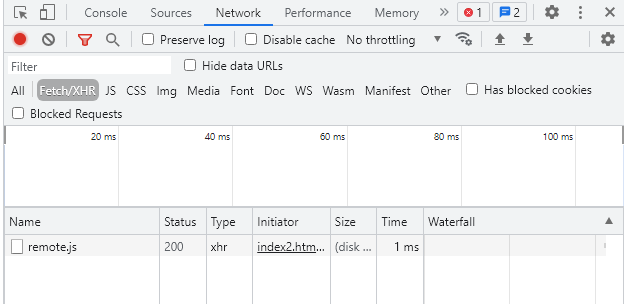
Javascript get html content. The <template> HTML element is a mechanism for holding HTML that is not to be rendered immediately when a page is loaded but may be instantiated subsequently during runtime using JavaScript. Think of a template as a content fragment that is being stored for subsequent use in the document. While the parser does process the contents of the ... 15/4/2018 · In particular I need it’s title and whole body. NilkasG(Niklas Gollenstede) April 15, 2018, 11:07pm #2. const html = (await (await fetch(url)).text()); // html as textconst doc = new DOMParser().parseFromString(html, 'text/html');doc.title; doc.body; freaktechnik(Martin Giger) If you need to know the actual size of the content, regardless of how much of it is currently visible, you need to use the Element.scrollWidth and Element.scrollHeight properties. These return the width and height of the entire content of an element, even if only part of it is presently visible due to the use of scroll bars.
1. Create a temporary DOM element and retrieve the text. This is the preferred (and recommended) way to strip the HTML from a string with Javascript. The content of a temporary div element, will be the providen HTML string to strip, then from the div element return the innerText property: /** * Returns the text from a HTML string * * @param ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Getting HTML with fetch() in vanilla JS Yesterday, we looked at how to use the Fetch API with vanilla JS. The article focused on making API calls and working with JSON data. Today, I want to show you how to use fetch() to get HTML instead. The Fetch API returns a stream. To recap, the response we get back from fetch() is a ReadableStream.
Using data attributes. HTML5 is designed with extensibility in mind for data that should be associated with a particular element but need not have any defined meaning. data-* attributes allow us to store extra information on standard, semantic HTML elements without other hacks such as non-standard attributes, extra properties on DOM, or Node ... 2) Define the main page, for example "main.html" (in the same folder on the server), in which write the code to include the IFrame and the JavaScript that will retrieve and modify the content of the IFrame. The element property innerHTML is used for getting and setting the HTML content of en element in vanilla JavaScript:
The basic steps to create the table in sample1.html are: Get the body object (first item of the document object). Create all the elements. Finally, append each child according to the table structure (as in the above figure). The following source code is a commented version for the sample1.html. Finding HTML Elements by CSS Selectors If you want to find all HTML elements that match a specified CSS selector (id, class names, types, attributes, values of attributes, etc), use the querySelectorAll () method. This example returns a list of all <p> elements with class="intro". Using the API, you will get ten users and display them on the page using Vanilla JavaScript. The idea is to get all the data from the Random User API and display it in list items inside the author's list. Begin by creating an HTML file and adding a heading and unordered list with the id of authors:
The HTML DOM allows JavaScript to change the content of HTML elements. 🎓 We need a browser environment for executing Javascript code and rendering content — HTML. It sounds like an easy and fun problem to solve! In the below 👇 section I will show 2 ways how to solve the above-mentioned problem using: Get the HTML contents of the first element in the set of matched elements or set the HTML contents of every matched element. ... Description: Get the HTML contents of the first element in the set of matched elements.
The HTML DOM allows JavaScript to change the content of HTML elements. Changing HTML Content. The easiest way to modify the content of an HTML element is by using the innerHTML property. To change the content of an HTML element, use this syntax: document.getElementById(id).innerHTML = new HTML. The innerHTML property sets or returns the HTML content (inner HTML) of an element. Introduction to JavaScript Get Element by Class. Whenever we want to access and get an element present in the DOM of HTML in javascript, we can get the element either based on its id, class or name. There are predefined methods and functions provided to access HTML elements in javascript that are mentioned in the Document interface.
Approach: First make the necessary JavaScript file, HTML file and CSS file. Then store the API URL in a variable (here api_url). Define a async function (here getapi ()) and pass api_url in that function. Define a constant response and store the fetched data by await fetch () method. Define a constant data and store the data in JSON form by ... 1 week ago - The Element property innerHTML gets or sets the HTML or XML markup contained within the element. Vanilla JavaScript provides two really easy ways to get and set content in the DOM—one to manipulate markup, and the other just for text. Manipulating HTML You can use the innerHTML to get and set HTML content in an element. var elem = document.querySelector('#some-elem'); // Get HTML content var html = elem.innerHTML; // Set HTML content elem.innerHTML = 'We can dynamically change the HTML.
Apr 13, 2021 - Get access to ad-free content, doubt assistance and more! ... Understanding basic JavaScript codes. ... Given an HTML document, the task is to get the entire document as a string using JavaScript. Here few methods are discussed: Nov 17, 2017 - Vanilla JavaScript provides two really easy ways to get and set content in the DOM—one to manipulate markup, and the other just for text. ... You can use the innerHTML to get and set HTML content in an element. For an external (cross-site) solution, you can use: Get contents of a link tag with JavaScript - not CSS It uses $.ajax () function, so it includes jquery.
If we want to get data from another HTML page and insert it into the displayed page, this can be achieved easily. ... It will be filled when the other HTML page is loaded. JavaScript functions. ... A very simple demo will display below the content of another HTML page anotherpage.html. Using DOM's method. In the last DOM article, you learned that the DOM represents the contents of a Web page as a "tree" of JavaScript objects.By accessing the parts of the tree, called the nodes, you can read existing page content, alter content, and even add new content from scratch.. In this article you'll learn how to locate and retrieve the elements of a Web page using JavaScript and the DOM. Description: Get the combined text contents of each element in the set of matched elements, including their descendants. ... This method does not accept any arguments. Unlike the .html() method, .text() can be used in both XML and HTML documents. The result of the .text() method is a string ...
5 Ways To Display Dynamic HTML Content In Javascript. By W.S. Toh / Tips & Tutorials - HTML & CSS, Tips & Tutorials - Javascript / July 24, 2021 July 24, 2021. Welcome to a tutorial on how to display dynamic HTML content in Javascript. Yes, the Stone Age of the Internet is long over, and we now live in an age of non-static HTML pages. The HTMLCollection in the HTML DOM is live, meaning when the document is changed it will be automatically updated. NodeList objects are collections of nodes returned by properties such as Node. There are two types of NodeList: live and static. It is static when any change in the DOM does not affect the content of the collection. 25/1/2015 · Typically, you can make a script using [Insert Server Side Language here] to download the requested page. Then your javascript can make a request to this page. There is also 'JSONP', but this is typically used on sites that provide specific JSONP access, which most random URL's do not. Share.
Definition and Usage. The textContent property sets or returns the text content of the specified node, and all its descendants. If you set the textContent property, any child nodes are removed and replaced by a single Text node containing the specified string.. Note: This property is similar to the innerText property, however there are some differences: ... In this article, you will learn how JavaScript interacts with HTML. CFP is Open Now: C# Corner Software Architecture Virtual Conference . Why ... My suggestion is that content elements content (inner text) can be get/set with the help of INNERTEXT, INNERHTML. FORM control element value can be get/set with help of VALUE. Dec 31, 2014 - On a recent project, I needed to load the form on a contact page in a modal window on a different page. That’s typically something you’d turn to jQuery for, but I want to show you can achieve the same effect with native JavaScript. Asynchronous HTML This approach uses XMLHttpRequest web API.
6/6/2019 · It specifies the tagname of the elements to get. HTML DOM innerHTML Property This property set/return the HTML content (inner HTML) of an element. Syntax: Return the innerHTML property: HTMLElementObject.innerHTML. Set the innerHTML property: HTMLElementObject.innerHTML = text. text: It specifies the HTML content of an element. If we want to get data from another HTML page and insert it into the displayed page, this can be achieved easily. The responseXML attribute holds an XML document DOM can access, and to provide the equivalent for HTML we need just for a <div> tag and a JavaScript function that extends Ajax or ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
innerHTML is a JavaScript property that can be used to get the HTML content added into a HTML element in web page, and also it can be used to replace the content into a HTML element with other content. 1. Syntax, get content with innerHTML : var content = element.innerHTML;
How To Get Only Html Content Containing No Js Code With
Html Javascript Change Div Content Stack Overflow
Javascript Getelementbyid How Getelementbyid Work With
Kendojs Htmltopdf Htmltoimage Htmltosvg Overview Outsystems
How To Get Attributes Of Html Element Element In Javascript
Saggezza Next Js A Development Framework For Scalability
How To Link Javascript To Html
How Can Get Html Content Include Content Of Javascript
How To Get Html Content Of An Iframe Using Javascript
Safe Ways To Inject Html Through Javascript Ta Digital Labs
Get Html Tag Values With Javascript
An Introduction To The Javascript Dom
Download A Html Content As Pdf Using Javascript Jspdf
Web Scraping Javascript Rendered Content Html Goodies
Learn How To Get Current Date Amp Time In Javascript
Migrate Sharepoint Javascript Customizations To Sharepoint
When Using Clientless Access Cvpn Some Urls Referenced In
How To Get Client Ip Address In Php And In Javascript
Javascript 2 Pdf Javascript Can Change Html Content U2028
Html To Pdf In Javascript Convert Web Page To Pdf File
How To Get Current Date Amp Time In Javascript Pakainfo
Jsreport Javascript Based Reporting Platform
Html Css And Javascript Influence On Zendesk 729 Solutions
Javascript Set Input Value By Class Name Change Value
Pdf Innerhtml And Outerhtml To Get And Replace Html Content
Dynamically Loading A Javascript File Codeproject
How To Scrape Html Content From A Website Built With
Working With Javascript Across Web Files Digitalocean
Features Of Javascript 13 Vital Javascript Features You
How To Get Html Text Of An Element In Javascript
0 Response to "31 Javascript Get Html Content"
Post a Comment