27 Foreach Method In Javascript
The forEach() method executes a provided function once for each array element. forEach() calls a provided callbackFn function once for each element in an array in ascending index order. It is not invoked for index properties that have been deleted or are uninitialized. Sep 12, 2017 - I use lodash in almost all my projects. In case you don’t know what lodash is, it’s basically a utility library in JavaScript that lets you do a lot of common things. lodash has a lot of helpful iteration methods, such as forEach and map. These also work on objects, which is really neat.
Javascript Under The Hood Building Our Own Foreach And Map
The arr.forEach method allows to run a function for every element of the array. forEach(func) - calls func for every element, does not return anything. To transform the array Create a function copySorted(arr) that returns such a copy. let arr = ["HTML", "JavaScript", "CSS"]
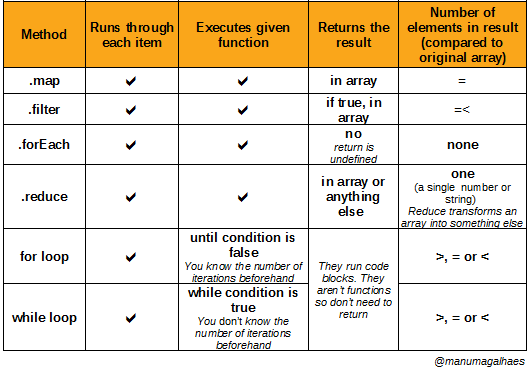
Foreach method in javascript. Definition and Usage. The forEach () method calls a function once for each element in an array, in order. forEach () is not executed for array elements without values. Javascript on AWS Lambda. A Practical Guide to AWS IAM. Asynchronous Programming Patterns in Javascript. Getting an "already subscribed" error? The forEach function is similar to the map, but instead of transforming the values and using the results, it runs the function for each element and... Javascript array forEach() method calls a function for each element in the array. ... Returns the created array.. ... This method is a JavaScript extension to the ECMA-262 standard; as such it may not be present in other implementations of the standard. To make it work, you need to add following ...
23/6/2020 · Below example illustrate the Array forEach() method in JavaScript: Example: In this example the method forEach() calculates the square of every element of the array. const items = [1, 29, 47]; const copy = []; items.forEach(function(item){ copy.push(item*item); }); print(copy); Output: 1,841,2209. Code for the above method is provided below: How to add JavaScript to html How ... in JavaScript How to open JSON file Random image generator in JavaScript How to add object in array using JavaScript JavaScript Window open method JavaScript Window close method How to check a radio button using JavaScript JavaScript Const ... The forEach method in Javascript iterates over the elements of an array and calls the provided function for each element in order. array: the array on which the specified function is called. forEach: the method called for the array with the required parameters.
JavaScript's `forEach()` function is one of several ways to iterate through an array in JavaScript. Here's what you need to know about `forEach()`. Getting Started. The forEach() method takes a parameter callback, which is a function that JavaScript will execute on every element in the array. The forEach() method calls a provided function once for each element in an array Apr 03, 2019 - How can I loop through all the entries in an array using JavaScript? I thought it was something like this: forEach(instance in theArray) Where theArray is my array, but this seems to be incorrect.
Javascript forEach() is an Array method that we can use to execute a function on each item in an array. Javascript for in loop is used to iterate over the enumerable properties of objects. Every property in an object will have an Enumerable value — if that value is set to true, then the property is... 18/2/2020 · The forEach () loop was introduced in ES6 (ECMAScript 2015) and it executes the given function once for each element in an array in ascending order. It doesn't execute the callback function for empty array elements. You can use this method to iterate through arrays and NodeLists in JavaScript. JavaScript is the language that allows web pages to be interactive. Though it is a comparatively new technology, it is found today on millions of web pages and In this tutorial, we're going to take a look at the ForEach method in JavaScript. While we have written this tutorial for beginners, you do need to...
forEach() method in JavaScript is mostly associated with some type of ordered data structures like array, maps and sets. forEach() method is used with reference to the Array references segment and is mostly used with callback function and synchronization of the elements while accessing and... Nov 30, 2017 - Hey everyone! This is going to be a quick introduction to foreach, and for...in in JavaScript. This article was written to introduce you to new methods that you can you can use instead of always using for loops. Nov 19, 2019 - The ins, outs and common pitfalls of the forEach array method in JavaScript.
26 Jul 2021 — The forEach() method executes a provided function once per each key/value pair in the Map object, in insertion order. The forEach() method calls a function once for each element in an array, in order. forEach() is not executed for array elements without values. Browser Support. forEach() is fully supported in all modern browsers Tutorial on JavaScript foreach methods: practice using JavaScript array foreach with JavaScript foreach examples JavaScript forEach() applies a specified function for every item in a particular array individually. This method only executes the specified function in an array item which has a value.
JavaScript forEach. The syntax of the forEach () method is: array.forEach (function(currentValue, index, arr)) Here, function (currentValue, index, arr) - a function to be run for each element of an array. currentValue - the value of an array. index (optional) - the index of the current element. arr (optional) - the array of the current elements. forEach() in JavaScript calls the provided function on each array item with 3 arguments: item, index, the array itself. Learn how to use forEach(). array.forEach(callback) method is an efficient way to iterate over all array items. Its first argument is the callback function, which is invoked for every item in... 20 Jul 2021 — The forEach() method executes a provided function once for each value in the Set object, in insertion order.
More briefly, .forEach is a prototype for Array in JavaScript which takes an anonymous function as input with ArrayElement, Index and Array itself as parameters so that you can perform anything with the individual elements of the Array. Let's consider an example 1 day ago - This makes sense if you understand how convenience methods like forEach are created. Newer, ES6 vintage syntax and convenience methods like forEach are added to the JavaScript engine (V8, Chakra, etc.) using a 'polyfil' that abstracts the lower level way of achieving the same thing, the for loop in ... JavaScript forEach loops are most useful when you need to do something with every item in an array in JavaScript, not just a few. This means that the forEach method it has browser support across most of the main browsers. And there you have it: JavaScript forEach loops in a nutshell!
The forEach() method executes a function once for each item in the array. The method is called on the array object that you wish to manipulate, and JavaScript provides a number of iteration methods - forEach(), map(), filter(), and reduce(). There are a couple critical differences between forEach() and... JavaScript Array provides the forEach() method that allows you to run a function on every element. The following code uses the forEach() method that is equivalent to the code above The JavaScript forEach method is one of the several ways to loop through arrays. Each method has different features, and it is up to you, depending on what you're doing, to decide which one to use. In this post, we are going to take a closer look at the JavaScript forEach method.
The JavaScript array forEach() method iterates over the array elements and executes a function for each element. Syntax is Terminating / Skipping the forEach loop. Unlike for loop, we cannot use break or continue in forEach() method. To terminate forEach() we need to through an exception... 6 days ago - Therefore, Javascript forEach only ... method to iterate the array’s items in JavaScript. Okay, first, we will see the syntax of the forEach() method. ... It is required, and it is a function to be run for each element in the array.... But additionally, JavaScript engines optimize those calls away (in performance-critical code) when dealing with native iterators for things like arrays. In ECMAScript 5 there will be a forEach method on the array prototype, but it is not supported in legacy browsers.
Foreach In Javascript Archives Learn To Code Together
Javascript Foreach A Return Will Not Exit The Calling
Understanding The Foreach Method For Arrays In Javascript
Javascript Foreach Array Function Developer Helps
Javascript Foreach How To Iterate Array In Javascript
Javascript Foreach Example Amp Demo
Javascript Array Foreach Method To Loop Through An Array
Exploring The Javascript Foreach Method For Looping Over Arrays
Using A For Each Loop On An Empty Array In Javascript Stack
Work With Javascript For Loop Foreach And Jquery Each
Foreach Vs For Loops In Javascript What S The Difference
Javascript Foreach Array Using The Foreach Method To
6 Ways To Loop Through An Array In Javascript Codespot
Foreach Loop Javascript Code Example
Use Array Foreach Method To Run A Function On Every Item In
Everything You Need To Know About Foreach In Javascript
How To Use Javascript Foreach Method Tecadmin
Array Foreach Method In Javascript Array Prototype Foreach
Comparing Js Iteration Methods Map Filter Foreach Reduce
For Each Over An Array In Javascript Stack Overflow
Javascript Array Foreach Method Complete Guide
Foreach L P In Javascript Javascript Provides N Number Ways
How To Use Foreach Loop In Javascript
Start Using Foreach And Map With Dom Selections By Dave
Foreach Method In Javascript And How To Use It Code Blog
Functions Of Javascript End Of The For Loop In Javascript
0 Response to "27 Foreach Method In Javascript"
Post a Comment