27 Javascript String With Variables
Template literals are enclosed by the backtick (` `) (grave accent) character instead of double or single quotes.Template literals can contain placeholders. These are indicated by the dollar sign and curly braces (${expression}).The expressions in the placeholders and the text between the backticks (` `) get passed to a function. Apr 28, 2021 - As you can see, string interpolation ... things with strings. ... The addition of template literals in ES6 allows us to write better, shorter, and clearer strings. It also gives us the ability to inject variables and expressions into any string. Essentially, whatever you write inside the curly brackets (${}) is treated as JavaScript...
Valid Javascript Variable Names In Es5 Mathias Bynens
JavaScript Data Types. JavaScript variables can hold numbers like 100 and text values like "John Doe". In programming, text values are called text strings. JavaScript can handle many types of data, but for now, just think of numbers and strings. Strings are written inside double or single quotes. Numbers are written without quotes.
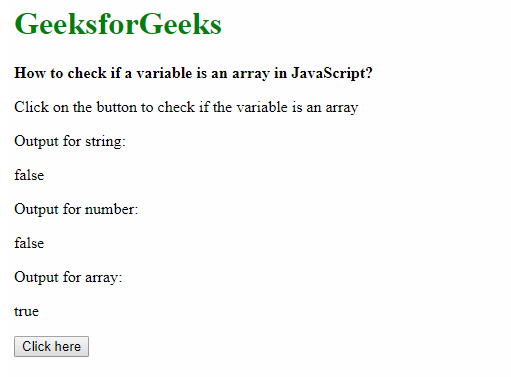
Javascript string with variables. Another way to include a variable to a string is through String Interpolation. In JavaScript, you can insert or interpolate variables into strings using template literals. Here is an example of a... How to Do String Interpolation in JavaScript. Replacement of placeholders with values inside of a string literal is called string interpolation. In JavaScript, the template literals (also template string) wrapped in backticks (`) that supports the string interpolation and $ {expression} as placeholder perform the string interpolation like this: Well, it is possible, and here in this simple tutorial, I am going to show you how to convert any string into a variable in JavaScript. To do this task, we will use the JavaScript eval () function. Well, this is the function that will play the main role to create variable name from our string. Now see the example below:
Backticks are generally used when you need to include variables or expressions into a string. This is done by wrapping variables or expressions with $ {variable or expression} as shown above. You can also write a quote inside another quote. Aug 31, 2020 - The string interpolation is helpful in many situations. But when the template string becomes large, with complex placeholder expressions, you might look for other solutions. The following component builds the CSS class based on 2 variables: Storing a String in a Variable. Variables in JavaScript are named containers that store a value, using the keywords var, const or let. We can assign the value of a string to a named variable. const newString = "This is a string assigned to a variable."; Now that the newString variable contains our string, we can reference it and print it to the ...
It means a variable must be declared with the data type that specifies the type of data a variable will store. JavaScript is a loosely typed language. It means it does not require a data type to be declared. You can assign any literal values to a variable, e.g., string, integer, float, boolean, etc. String Substitution One of their first real benefits is string substitution. Substitution allows us to take any valid JavaScript expression (including say, the addition of variables) and inside a... Assignment operators assign values to JavaScript variables. The addition assignment operator (+=) adds a value to a variable.
Separators make Array#join() a very flexible way to concatenate strings. If you want to join together a variable number of strings, you should generally use join() rather than a for loop with +. String#concat() JavaScript strings have a built-in concat() method. The concat() function takes one or more parameters, and returns the modified string. Dec 11, 2019 - In JavaScript, we can assign strings to a variable and use concatenation to combine the variable to another string. When comparing a string with a number, JavaScript will convert the string to a number when doing the comparison. An empty string converts to 0. A non-numeric string converts to NaN which is always false. When comparing two strings, "2" will be greater than "12", because (alphabetically) 1 is less than 2.
JavaScript is a "dynamically typed language", which means that, unlike some other languages, you don't need to specify what data type a variable will contain (numbers, strings, arrays, etc). For example, if you declare a variable and give it a value enclosed in quotes, the browser treats the variable as a string: Strings Can be Objects. Normally, JavaScript strings are primitive values, created from literals: let firstName = "John"; But strings can also be defined as objects with the keyword new: let firstName = new String ("John"); Example. let x = "John"; let y = new String ("John"); // typeof x will return string. There is no more need to open and close multiple string pieces — the whole lot can just be wrapped in a single pair of backticks. When you want to include a variable or expression inside the string, you include it inside a ${ } construct, which is called a placeholder. You can include complex expressions inside template literals, for example:
Apr 26, 2019 - Build a strong foundation in web development by learning and practicing JavaScript, one of the major programming languages on the web. You'll even create a simple application! The eval() function evaluates any JavaScript code in the form of a string. For example: eval("myNum = 88"); The above example will create a variable myNum and store value 88 in it. Now below is the example of creating an adynamic variable using the eval() function: What's the best way to do insert variables in a string in JavaScript? I'm guessing it's not this: var coordinates = "x: " + x + ", y: " + y; In Java, Strings are immutable and doing something like the above would unnecessarily create and throw away Strings. Ruby is similar and has a nice way of doing the above: coordinates = "x: #{x}, y: #{y}"
Use Basic Formatting to Insert Variable Into String in JavaScript. Another way of neatly inserting the variable values into our string is by using the basic formatting supported in JavaScript. Using the Javascript console.log (), we can avoid concatenation and add placeholders in the targetted string. The unary + operator: value = +value will coerce the string to a number using the JavaScript engine's standard rules for that. The number can have a fractional portion (e.g., +"1.50" is 1.5). Any non-digits in the string (other than the e for scientific notation) make the result NaN. Also, +"" is 0, which may not be intuitive. The addition of template literals in ECMAScript 6 (ES6) allows us to interpolate strings in JavaScript. In simpler words, we can use placeholders to inject variables into a string. You can see an example of string interpolation using template literals in the snippet below: const age = 4.5; const earthAge
Jan 16, 2018 - JavaScript is untyped language. This means that a JavaScript variable can hold a value of any data type. To declare variables in JavaScript, you need to use the var keyword. Whether it is a string or a number, use the var keyword for declaration. Sep 02, 2020 - javascript create a function that counts the number of syllables a word has. each syllable is separated with a dash -. ... JavaScript function that generates all combinations of a string. Nov 07, 2017 - This is a string in the console. Another simple way to output a value is to send an alert popup to the browser with alert(): ... alert() is a less common method of testing and viewing output, as it can quickly become tedious to close the alerts. ... Variables in JavaScript are named containers ...
JavaScript's String type is used to represent textual data. It is a set of "elements" of 16-bit unsigned integer values (UTF-16 code units). Each element in the String occupies a position in the String. The first element is at index 0, the next at index 1, and so on. 16/10/2011 · If you want to have something similar, you could create a function: function parse (str) { var args = [].slice.call (arguments, 1), i = 0; return str.replace (/%s/g, () => args [i++]); } Usage: s = parse ('hello %s, how are you doing', my_name); This is only a simple example and does not take into account different kinds of data types (like %i, ... slice () extracts a part of a string and returns the extracted part in a new string. The method takes 2 parameters: the start position, and the end position (end not included). This example slices out a portion of a string from position 7 to position 12 (13-1): Remember: JavaScript counts positions from zero. First position is 0.
5 Ways to Pass Variables Between Pages In Javascript By W.S. Toh / Tips & Tutorials - Javascript / June 15, 2021 June 24, 2021 Welcome to a tutorial on how to pass variables between pages in Javascript. JavaScript automatically converts primitives to String objects, so that it's possible to use String object methods for primitive strings. In contexts where a method is to be invoked on a primitive string or a property lookup occurs, JavaScript will automatically wrap the string primitive and call the method or perform the property lookup. How to declare String Variables in JavaScript? Javascript Web Development Front End Technology. JavaScript is untyped language. This means that a JavaScript variable can hold a value of any data type. To declare variables in JavaScript, you need to use the var keyword. Whether it is a string or a number, use the var keyword for declaration.
Let's check out the different ways of converting a value to a string in JavaScript. The preferred way from Airbnb's style guide is… Apr 11, 2021 - Unlike the mere string concatenation, with template literals, we can include expressions with more than one variable, similar to coding in JavaScript with the template literals. If we need to do certain operations like summing, getting substrings, including dates, etc., all these functionalities ... Returns -1 if not found. localeCompare (string,position) Compares two strings in the current locale. match (RegExp) Search a string for a match using specified regular expression. Returns a matching array. replace (searchValue, replaceValue) Search specified string value and replace with specified replace Value string and return new string.
JavaScript variables can hold numbers like 100 and text values like "John Doe". In programming, text values are called text strings. JavaScript can handle many types of data, but for now, just think of numbers and strings. Strings are written inside double or single quotes. Numbers are written without ...
How To Work With Strings In Jmeter Blazemeter
String Variables To Html Elements Super Simple Javascript Jquery Tutorials
Javascript Concatenate Int With String Code Example
Javascript Console Log With Examples Geeksforgeeks
Apache Jmeter User S Manual Functions And Variables
Javascript Variable Types And Syntax Js Note 3 Data Visioner
How To Check If A Variable Is An Array In Javascript
Best Way To Check Null Undefined Empty Variables In
Javascript Complete Basics Tutorial Variables Constants
How To Work With Strings In Jmeter Blazemeter
Introduction To Javascript Part1 Online Presentation
Learning Javascript Variables Strings String
How To Work With Strings In Javascript Digitalocean
Search A String Variable With Javascript Methods
Javascript Strings Properties And Methods
Text Styling With Global Variable Values Axure Rp 9 Axure
How To Call Function From It Name Stored In A String Using
How To Use Test Case Variables In Javascript Web Testing
Storing The Information You Need Variables Learn Web
How To Insert Variables In Javascript String Example Code
Quick Tip How To Declare Variables In Javascript Sitepoint
Understanding Js Primitives Javascript Is A Loosely Typed
Cannot Create Date Variable Using Javascript In Script Task
5 Ways To Pass Variables Between Pages In Javascript
0 Response to "27 Javascript String With Variables"
Post a Comment