31 How To Check Event Listeners Javascript
How to listen to hover event in JavaScript, EventTarget.addEventListener modern JavaScript answer on Code to go Definition and Usage. The removeEventListener() method removes an event handler that has been attached with the addEventListener() method.. Note: To remove event handlers, the function specified with the addEventListener() method must be an external function, like in the example above (myFunction). Anonymous functions, like "element.removeEventListener("event", function(){ myScript});" will ...
How To Make A Website Interactive With Javascript Events
The format for adding events using this method is as follows: document.addEventListener('click', myfunction, false); In the above format, you start by adding the method to the Document object. In the parentheses, list the event listener but without the on. (In this example, the event listener is onclick, which is shortened to click .)
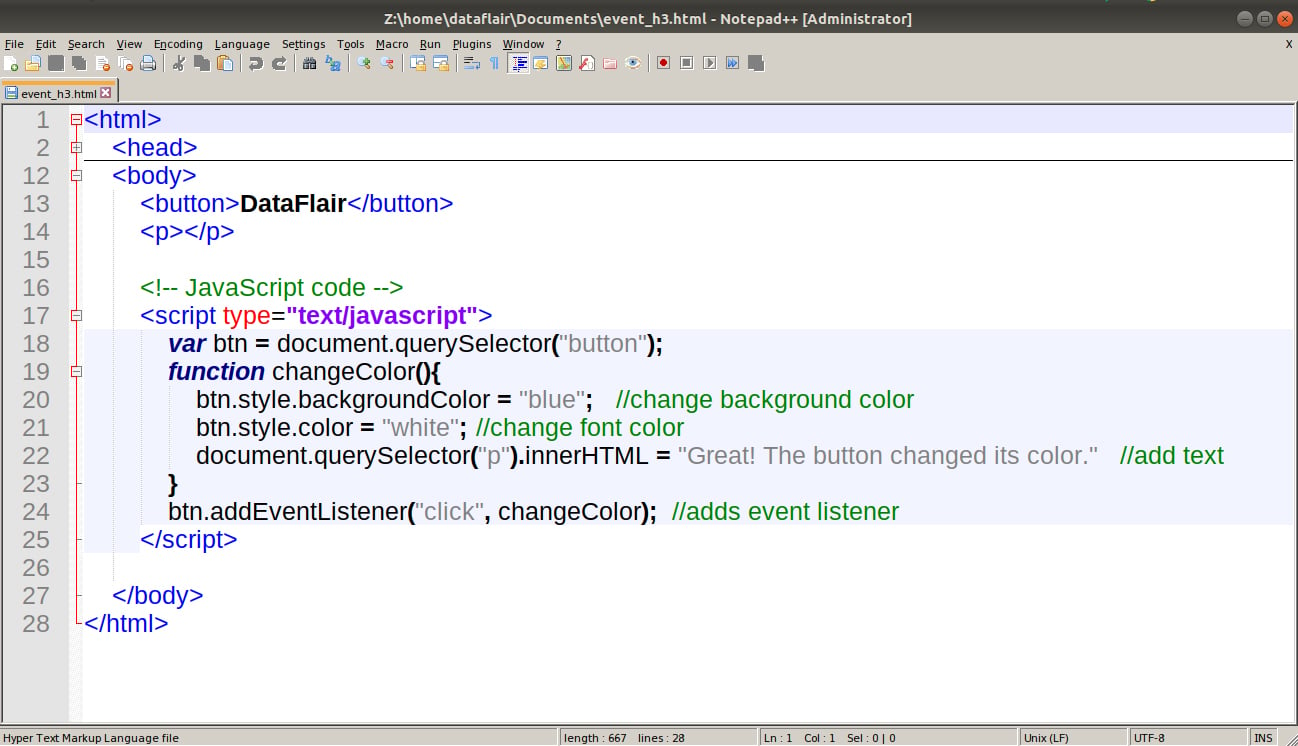
How to check event listeners javascript. An event handler is also known as an event listener. It listens to the event and responds accordingly to the event fires. An event listener is a function with an explicit name if it is resuable or an anonymous function in case it is used one time. An event can be handled by one or multiple event handlers. If an event has multiple event handlers ... javascript event listener checkbox Code Example. //jsvar checkbox = document.querySelector("input[name=checkbox]");checkbox.addEventListener( 'change', function() { if(this.checked) { // Checkbox is checked.. } else { // Checkbox is not checked.. As querySelectorAll () returns a NodeList instead of a single element, we need to loop through the nodes to add a click event listener to each button. For instance, if we have three buttons on the page, the code above will create three click event listeners. Note that you can only listen to one event with addEventListener ().
In this blog, I will try to make clear the fundamentals of the event handling mechanism in JavaScript, without the help of any external library like Jquery/React/Vue. I will be explaining the following topics in this article: The document and window objects, and adding Event Listeners to them. The Event.preventDefault() method and it's usage. Testing event listeners in JavaScript. Testing JavaScript code that's asynchronous can prevent its own set of challenges that you have to ensure you deal with when writing your tests. I recently came across a test that fell foul to the asynchronous code it was trying to test and in this post I'll show you how to look out for these tests and how ... EventListener. The EventListener interface represents an object that can handle an event dispatched by an EventTarget object. Note: Due to the need for compatibility with legacy content, EventListener accepts both a function and an object with a handleEvent () property function. This is shown in the example below.
It is possible to list all event listeners in JavaScript: It's not that hard; we just have to hack the prototype's method of the HTML elements (before adding the listeners). Instead of adding event listeners to specific elements, you listen to all events on a parent element (often the document or window). Events within that element "bubble up," and you can check to see if the element that triggered the event (the event.target) matches the selector you really care about. You can use getEventListeners (node) in the Console Panel to retrieve registered event listeners on the passed in DOM node. In addition to that, the video clip shows debug (fn) invoking the...
The window's load event For the window object, the load event is fired when the whole webpage (HTML) has loaded fully, including all dependent resources such as JavaScript files, CSS files, and images. To handle the load event, you register an event listener using the addEventListener () method: JavaScript removeEventListener () method with examples. Javascript Web Development Object Oriented Programming. The JavaScript removeEventListener () method is used to remove an event listener from an element that has been previously attached with the addEventListener () method. Following is the code for removeEventListener () method −. 19/7/2020 · Navigate to the Event Listeners Pane in the Elements Panel Browse the events registered on any node. You can view the source of an event listener by selecting Show Function Definition in the context menu
First, register an event listener to the button with id btn. Second, use the querySelectorAll() method to select the radio button group with the name choice. Third, iterate over the radio button groups and get the value of the selected radio button. Summary. Use the <input> element with the type radio to create a radio button. According to DOM 3 Events, when you used addEventListener there should be an event listener list (EventListenerList DOM 3 Events), but it has yet to be implemented by any browser (Sept, 2012). Until then there is no easy getEventListener method. If you want to programmatically triggering events, keep a copy of a list globally or use a library. But this method is only available in developer tools. Overriding addEventListener prototype. If we want this method to be available in scripts, we have to override the addEventListener prototype. The override consists in adding an object eventListenerList that will store added event listeners. The method that will retrieve the event listeners will return this object.
event Event name, e.g. "click". handler The handler function. options An additional optional object with properties: once: if true, then the listener is automatically removed after it triggers.; capture: the phase where to handle the event, to be covered later in the chapter Bubbling and capturing.For historical reasons, options can also be false/true, that's the same as {capture: false/true}. JavaScript provides an event handler in the form of the addEventListener () method. This handler can be attached to a specific HTML element you wish to monitor events for, and the element can have more than one handler attached. A tab or window closing in a browser can be detected by using the beforeunload event. This can be used to alert the user in case some data is unsaved on the page, or the user has mistakenly navigated away from the current page by closing the tab or the browser. The addEventListener () method is used to set up a function whenever a certain event ...
The addEventListener () method allows you to add event listeners to any HTML DOM elements, the document object, the window object, or any other object that support events, e.g, XMLHttpRequest object. Here's an example that attaches an event listener to the window "resize" event: I think there is no standard way in javascript to get the existing event handlers. At best you could surcharge the addEventListener function of Node to intercept and store the listeners but I don't recommend it... 16/10/2019 · Finally we can check for multiple keys with simple statements. document.addEventListener ('keydown', (event) => { keysPressed [event.key] = true; if (keysPressed ['Control'] && event.key == 'a') { alert (event.key); } }); document.addEventListener ('keyup', (event) => { delete keysPressed [event.key]; });
There is no JavaScript function to achieve this. However, you could set a boolean value to true when you add the listener, and false when you remove it. Then check against this boolean before potentially adding a duplicate event listener. Possible duplicate: How to check whether dynamically attached event listener exists or not? The Elements section of Chrome's Developer Tools: select an element and look for "Event Listeners" on the bottom right (similar in Firefox) If we want to use the events in … 8/6/2020 · Once the dev tools are open, switch to the “Event Listeners” tab and you will see all the event listeners bound to the element. You can expand any event listener by clicking the right-pointing arrowhead. When you expand an event listener and hover over the element, the “remove” button will appear.
An event listener is a procedure in JavaScript that waits for an event to occur. The simple example of an event is a user clicking the mouse or pressing a key on the keyboard. The addEventListener () is an inbuilt function in JavaScript which takes the event to listen for, and a second argument to be called whenever the described event gets fired. So in Vanilla JavaScript, there are multiple ways of adding event listeners to elements. So let's look at some Vanilla JS examples for the addEventListener method and apply it to HTML elements. Add event listener to one single element permalink. So ofcourse we can use add event listener directly on an HTML element using for example the ... Use Chrome's Developer Tools to check for event listeners. I don't know how long this feature has been in the Dev Tools but I know it's something that I could have used a few times over the years. When you inspect an element hidden down in the corner underneath the "Styles", "Properties", "DOM Breakpoints" among others, is the ...
When debugging, if you want to just see if there's an event, I recommend using Visual Event or the Elements" section of Chrome's Developer Tools: select an element and look for "Event Listeners on the bottom right. In your code, if you are using jQuery before version 1.8, you can use: $(selector).data("events") to get the events. element.addEventListener (event, function, useCapture); The first parameter is the type of the event (like " click " or " mousedown " or any other HTML DOM Event.) The second parameter is the function we want to call when the event occurs. The third parameter is a boolean value specifying whether to use event bubbling or event …
Javascript Event Listener Checkbox Code Example
Javascript Event Types 8 Essential Types To Shape Your Js
Get Started With Debugging Javascript In Microsoft Edge
Use Chrome S Developer Tools To Check For Event Listeners
How To Find Event Listeners On A Dom Node When Debugging
Debug Javascript Chrome Developers
Event And Listener Concepts Matlab Amp Simulink
Debug Javascript Chrome Developers
Event Delegation In Javascript Geeksforgeeks
Learn Javascript Event Listeners In 18 Minutes
Javascript Events Explore Different Concepts And Ways Of
Inspect Attached Event Handlers For Any Dom Element Stack
Debug Javascript Chrome Developers
Listen To Multiple Events In A Single Class In Laravel Amit
View Event Listeners On Elements Chrome Devtools Dev Tips
Pause Your Code With Breakpoints Chrome Developers
How To Make A Website Interactive With Javascript Events
How To Handle Event Handling In Javascript Examples And All
Javascript Events Explore Different Concepts And Ways Of
Get And Debug Event Listeners Web Google Developers
Chrome Devtools On Twitter Tip If Your Event Listeners Are
An In Depth Guide To Event Listeners Aurelio De Rosa Blog
Finding That Pesky Listener That S Hijacking Your Event
Javascript How To List All Active Event Listeners On A Web Page
Remove Unused Javascript Event Listeners Javascript Dev Tips
Event Handlers Vs Event Listeners In Javascript By Artturi
How To Find Event Listeners On A Dom Node When Debugging
Blocking Event Listeners Techpowerup Forums
Javascript Addeventlistener With Examples Geeksforgeeks
0 Response to "31 How To Check Event Listeners Javascript"
Post a Comment