33 Palindrome Logic In Javascript
This is a full description of checking palindrome number (theory +program), Most of the time this question is asked ,fresher should learn before appear in in... Jul 01, 2019 - This article is based on Free Code Camp Basic Algorithm Scripting “Check for Palindromes”. A palindrome is a word, phrase, number, or other sequence of characters which reads the same backward or forward. The word “palindrome” was first coined by the English playwright Ben Jonson in ...
Palindrome In Python How To Check Palindrome Number In Python
Jul 17, 2017 - Palindromes are words or phrases and even numbers that read the same forwards and backwards. Short palindromes are quite common such as: civic, kayak, level, madam, noon, radar, rotor, and solos…
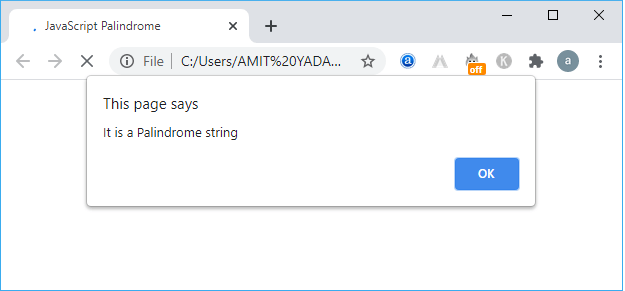
Palindrome logic in javascript. In this solution, we will take care of some of the simple cases before writing out logic. Once those are taken care of, we will follow the two-pointer method to check if the number is a palindrome. The idea is, we will take one digit from the start, and another from the last. Check if both are equal if not, the number is not a palindrome. Given an integer, write a function that returns true if the given number is palindrome, else false. For example, 12321 is palindrome, but 1451 is not palindrome. Recommended: Please solve it on " PRACTICE " first, before moving on to the solution. Let the given number be num. Approach: Take two pointers i pointing to the start of the string and j pointing to the end of the string. Keep incrementing i and decrementing j while i < j and at every step check whether the characters at these pointers are same or not. If not then the string is not a palindrome else it is. Below is the implementation of the above approach:
Java program to check if a string is a palindrome or not, it's a palindrome if it remains the same on reversal. If given array length is even, it can't be a palindrome Go through half the array, and find out whether array element is equal to the corresponding element on the "other side". In other words, check whether first element is equal to last element, the second element is equal to the last but one element, and so on. When a number doesn't change it' s value or meaning even after rotation from left to right or vice a versa, then the number is palindrome number. For example: 12321 after rotation 12321number is still same. JavaScript code to check palindrome number
Palindrome Number Program in JavaScript - Here we write program to check number is palindrome or not in javascript. HOME C C++ DS Java AWT Collection Jdbc JSP Servlet SQL PL/SQL C-Code C++-Code Java-Code Project Word Excel. Sitesbay - Easy to Learn . Html Html5 CSS JavaScript Ajax JQuery AngularJS JSON GMaps Adsense Blogger Earning Email Domain ... palindrome program in java with examples of fibonacci series, armstrong number, prime number, palindrome number, factorial number, bubble sort, selection sort, insertion sort, swapping numbers etc. Input Palindrome JavaScript - posted in HTML, CSS and Javascript: A little info:A Palindrome is a word or phrase when spelled backwards is exactly the same work. For example: bobbob is an example of a PERFECT palindrome.A Standard palindrome is a word or phrase similar to the perfect palindrome except with punctuation marks or spaces.Example: Madam, Im Adam What I need to do is have an input ...
Question :- Write a javascript function to check whether a word or a sentence is palindrome or not irrespective of case and spaces. Name the HTML file as palin.html. Give appropriate alerts on click of a button name is "palinbtn". Also provide a text box named "palin" which accepts the word / sentence. Important Note : Dec 31, 2019 - Write a function that — given a string — returns true if the string is a palindrome or false otherwise. This is a question that often shows up in job interviews and code challenges, JavaScript being… The logic to detect wheter a word is palindrome or not in programming is very simple, you need to remove the special characters from the string and reverse the result. If the strings are equal (the filtered and the reversed filtered string) then the string is palindrome, pretty easy right?
js, loop, palindrome in javascript, palindrome in js using function, palindrome number A number is a palindrome if it is written the same way after inverting it. Aug 01, 2018 - A detailed guide on implementing a palindrome checker in vanilla javascript. Tagged with javascript, showdev, explainlikeimfive, performance. To check given input is Palindrome with JavaScript The function named getPalindrome accepts input text as the parameter but here acting as the default parameter. We will handle even if the user doesn't pass the value will simply reject it.
JavaScript Function and Function Expressions A string is a palindrome if it is read the same from forward or backward. For example, dad reads the same either from forward or backward. So the word dad is a palindrome. Dec 14, 2020 - JavaScript exercises, practice and solution: Write a JavaScript function that checks whether a passed string is palindrome or not? \$\begingroup\$ As an improvement, 0-9 can be written as \d, with no side effects. 'use strict'; is being used twice. You can put it on the top and it takes effect everywhere. You should store document.getElementById("inputPalindrome") and document.getElementById('notification') in variables outside the click handler. Also, you have mixed quote styles. I recommend using double-quotes (") for ...
Similarly, a number that is equal to the reverse of that same number is called a palindrome number. For example, 3553, 12321, etc. To check a Palindrome in Java, we first reverse the string or number and compare the reversed string or number with the original value. Example 1: Java Program to Check Palindrome String Mar 22, 2017 - I wonder how to write palindrome in javascript, where I input different words and program shows if word is palindrome or not. For example word noon is palindrome, while bad is not. Thank you in ad... Program 1: String program to check palindrome using in Java. In the String Palindrome program, we can use logic to reverse a String, and then we will check the reversed String is similar to the given String or not. If reversed String will be equal to given String, then given String will be Palindrome, otherwise not.
Nov 11, 2018 - Three Ways to Check for Palindromes in JavaScript with performance measurement. Tagged with javascript, algorithms, recursion, programming. Write a program to check whether given number is Palindrome or not using do-while loop. Skip to content +91 97223 47635 ... Jun 11, 2021 - Palindrome in Java is nothing but any number or a string which remains same when reversed. Read up this blog to check palindrome program in multiple ways.
Whether you use Python, C++, or JavaScript, use one of these algorithms to find out. A string is said to be a palindrome if the original string and its reverse are the same. In this article, you'll learn about the algorithm to determine if the given string is a palindrome or not. Oct 07, 2019 - This article covers palindrome problem in Javascript, to check if a string is palindrome or not in JavaScript with 3 different code solution in Javascript. Aug 22, 2020 - The easiest way to deal with such ... case. After that, we can use the logic provided in the first example as follows: ... There is also a bit more advanced test we can make — compare two strings and output whether they are palindromes to each other or not....
You can't confirm if a string is a palindrome by just checking if one pair of chars are the same (which is what your modification does). But you can confirm a palindrome by checking if the chars in one pair are not the same. If it can't find non-matching chars, then you can say that the string is indeed a palindrome. In this tutorial, we will write a simple function called isPalindrome(chars) that takes a sequence of characters as input and returns true if the sequence is a palindrome, and false if it isn't. We will implement the algorithm for this function in JavaScript using recursion, but it can also be implemented in any other language of your choosing. Javascript Palindrome Logic. 0. Palindrome Checker - issue with for loop-2. how to i write my function in recursive way? See more linked questions. Related. 7626. How do JavaScript closures work? 8164. How do I check if an element is hidden in jQuery? 6730. How do I remove a property from a JavaScript object?
Jul 28, 2019 - Learn to check if a string is palindrome string with simple java programs using stack, queue, for and while loops. A palindrome is equal to its reverse. Create a javascript program to perform a palindrome test. Before understanding the logic of palindrome in javascript we need to understand what is a palindrome. Below is the definition of palindrome with examples. JavaScript Program to find Palindrome pwb, palindrome javascript by programmingwithbasics, Javascript Palindrome Check. What is ... Palindrome in JavaScript In this topic, we will learn about Palindrome and validate that the given numbers or strings are Palindrome or not in JavaScript. A palindrome is used to verify a sequence of numbers, strings, or letters that are read left to right and right to left to match the same characters or return the same sequence of characters.
Introduction to Palindrome in JavaScript In general sense, Palindrome is a word such as that when we read that word character by character from forward, it matches exactly with a word formed when the same word is read from backward. For Example: "level", "madam" etc. A Palindrome Number is a number that remains the same number when it is reversed. For example, 131. When its digits are reversed, it remains the same number. Palindrome number has reflection symmetry at the vertical axis. Javascript Palindrome Logic. Ask Question Asked 6 years, 5 months ago. Active 6 years, 5 months ago. Viewed 381 times 2 I have checked this thread: Palindrome check in Javascript But I'm more so looking to fix my own algorithm. I am just programming online right now so I do not have access to a good debugger.
C Examples C Program To Check A Palindrome String
Palindrome In Php How To Check Palindrome Number Or String
Python Program To Check A Number Or String Is Palindrome
Palindrome Number Program In Javascript T4tutorials Com
Check If A String Is Palindrome In Javascript Code Example
Js Coding Interview Question Build A Function That Tests To
Write A Palindrome Check Function In Javascript Using String And Array Methods
11 Ways To Check For Palindromes In Javascript By Simon
Three Different Ways To Check Palindrome In Javascript Using
Javascript Palindrome Code Example
Palindrome Number Program In Javascript
Palindrome In Javascript Javatpoint
Function To Check If A Singly Linked List Is Palindrome
Palindrome In Javascript Logical Explanation With Examples
Palindrome In Javascript Javatpoint
Check If A String Is A Palindrome With Javascript Tutorial
Is This A Palindrome Translating Between Ruby And Javascript
How To Make Palindrome Program Javascript
Check Number Is Palindrome Or Not In Javascript
Palindrome In Javascript Logical Explanation With Examples
Javascript Coding Challenge 3 Palindrome Check Freecodecamp
Javascript Function Check Whether A Passed String Is
C Program To Check If A Given String Is Palindrome
Check For Palindrome In Javascript 3 Different Ways
11 Ways To Check For Palindromes In Javascript By Simon
A Few Succinct Ways To Solve The Palindrome Problem In
C Creating Palindrome Program In C Checking String
Palindrome Check In Javascript Quiz For Exam
Leetcode Valid Palindrome With Javascript Dev Community
Check For Palindromes In Javascript By Sonya Moisset
0 Response to "33 Palindrome Logic In Javascript"
Post a Comment