27 Javascript Watch Property Change
In data property, the kilometers and meters are initialized to 0. There is a watch object created with two functions kilometers and meters. In both the functions, the conversion from kilometers to meters and from meters to kilometers is done. As we enter values inside any of the texboxes, whichever is changed, Watch takes care of updating both ... 17/11/2009 · A solution for most browsers (and IE6+) is available that uses the onpropertychange event and the newer spec defineProperty. The slight catch is that you'll need to make your variable a dom object. Full details: http://johndyer.name/native-browser-get-set-properties-in-javascript/. Share.
Watch For Object Changes With Javascript
Vue.js - The Progressive JavaScript Framework. How Changes Are Tracked. When you pass a plain JavaScript object to a Vue instance as its data option, Vue will walk through all of its properties and convert them to getter/setters using Object.defineProperty.This is an ES5-only and un-shimmable feature, which is why Vue doesn't support IE8 and below.
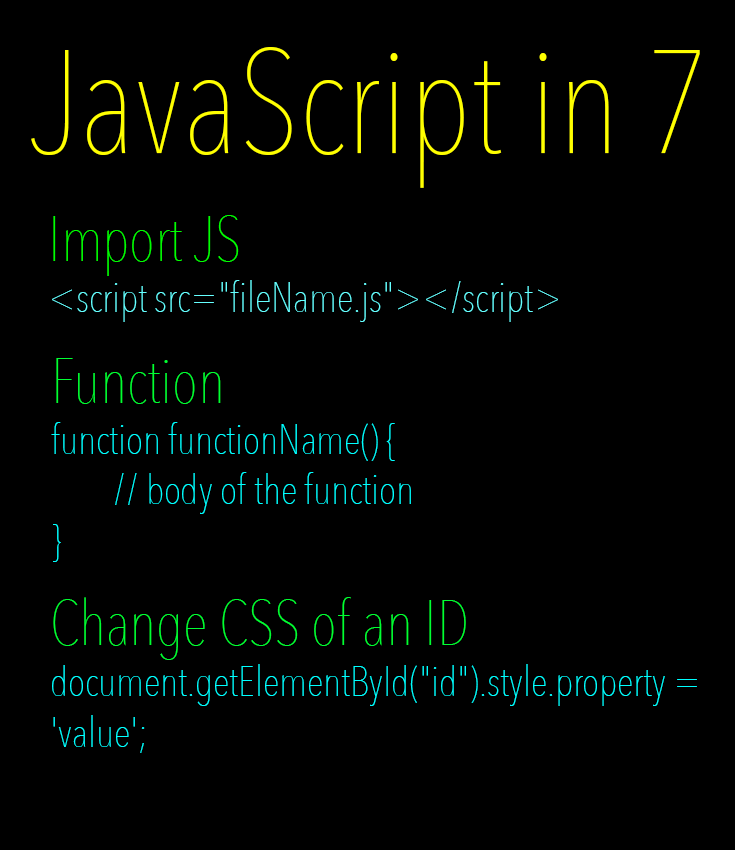
Javascript watch property change. JavaScript Properties. Properties are the values associated with a JavaScript object. A JavaScript object is a collection of unordered properties. Properties can usually be changed, added, and deleted, but some are read only. JavaScript is designed on a simple object-based paradigm. An object is a collection of properties, and a property is an association between a name (or key) and a value. A property's value can be a function, in which case the property is known as a method. In addition to objects that are predefined in the browser, you can define your own objects. This chapter describes how to use objects ... The watch function watches any property of an object for changes. This also includes the first assignment of value to the property. The watch function includes a handler which has access to the old...
When the property already exists, Object.defineProperty() attempts to modify the property according to the values in the descriptor and the object's current configuration. If the old descriptor had its configurable attribute set to false the property is said to be "non-configurable". It is not possible to change any attribute of a non-configurable accessor property. Rollup is a module bundler for JavaScript which compiles small pieces of code into something larger and more complex, such as a library or application. It uses the new standardized format for code modules included in the ES6 revision of JavaScript, instead of previous idiosyncratic solutions such as CommonJS and AMD. What we want is our fetchDogs method to listen or watch for a change in the breed value and rerun when a change happens. [1:50] Alpine JS provides the $watch property, which lets you watch a component property and fire a callback whenever that property changes. Let's change our x-init to instead of calling fetchDogs, call a new method called init.
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Note: Watchers can change only one property at a time. If multiple component values have to be changed, one can use computed properties. Syntax: watch: { // We can add our functions here } Vue.js Watchers can be demonstrated using the following example: Example: We will first create a simple Vue.js app without using any Watcher. # View and edit local, closure, and global properties. While paused on a line of code, use the Scope pane to view and edit the values of properties and variables in the local, closure, and global scopes. Double-click a property value to change it. Non-enumerable properties are greyed out. Figure 9. The Scope pane, outlined in blue
Object.observe () lets you add a listener to any JavaScript object that gets called whenever that object, or its properties, change. You can try it out now in Chrome Canary version 25. To... 13/12/2018 · You want to notify your web app visitor that some change has occurred on the page he’s currently on. You’re working on a new fancy JavaScript framework that loads dynamically JavaScript modules based on how the DOM changes. You might be working on a WYSIWYG editor, trying to implement undo/redo functionality. 11/11/2019 · Watchers are Vue.js feature that allows you to track a component property and run a function whenever its value changes. The following example shows how to watch for a property in Vue.js. Each time the input value changes, the watcher function track the property linked to the input and assigns the new value to the “result” property.
A few years back I wrote about a jquery-watch plugin I wrote that can monitor CSS property and Attribute changes and let you get notified if one of the monitored properties are changed. Unfortunately the plugin broke a while back as browser and jQuery dropped some older APIs. In this post I describe an update to the jquery-watch plugin using the newer and more widely supported MutationObserver ... The change () method triggers the change event, or attaches a function to run when a change event occurs. Note: For select menus, the change event occurs when an option is selected. For text fields or text areas, the change event occurs when the field loses focus, after the content has been changed. Variable values may change as an application continues to operate. The watch list is not a live view of the variables unless you are stepping through execution. When you are stepping through execution using breakpoints, the watched values will update automatically.
in this video, we will learn how to change dynamic css variable with javascriptSubscribe to SensiDev Channel HERE : https://www.youtube /channel/UCzVL5cWb... 20/12/2017 · Watch for Object Changes with JavaScript. By David Walsh on December 20, 2017. 5. Watching for changes to an object's property has always been a much sought after task; many shims have been used over the years to listen to object changes. These days we have better methods for listening to object changes: the Proxy API. Because any little change made to the controller will fire this watcher whether you intend it or not. And that is not a very performance-friendly idea. Summary: In this article, we learned about the "controller-as" syntax and a simple trick using which you can watch any changes made to the controller properties. Oh, and a final note ...
When you have some data that needs to change based on some other data, it is tempting to overuse watch - especially if you are coming from an AngularJS background. However, it is often a better idea to use a computed property rather than an imperative watch callback. Consider this example: < div id = "demo" > {{ fullName }} </ div > Change CSS Property With getElementById in JavaScript If we have a unique id assigned to an HTML element, we can query it and change its style with the getElementById () function of the Document interface. It is the most widely used method by web developers. on-change. Watch an object or array for changes. It works recursively, so it will even detect if you modify a deep property like obj.a.b[0].c = true.. Uses the Proxy API.. Install
The change event occurs when the element has completed changing. To attach an event handler to the change event of an element, you can either call the addEventListener () method: element.addEventListener ( 'change', function() { // handle change }); Code language: JavaScript (javascript) or use the onchange attribute of the element. For example: Access to magic properties in Alpine.js templates. There's no official Alpine.js "template" definition but I'll call all the elements inside of the root element (the one that has the x-data attribute), the "template".. In your Alpine.js template, you can access the magic properties directly. For objects, the deal doesn't change: if objectEquality is false, you just watch for any reassignment to that scope variable, while if it's true, every time an element in the object is changed ...
As the.change () method is just a shorthand for.on ("change", handler), detaching is possible using.off ("change"). I recently came across a jQuery plugin that claims to emulate the functionality of Object.prototype.watch, a method inherent in all objects that enables you to watch for a property change and run a function upon that change. Of course, this isn't available in all implementations. A watcher is a special Vue.js feature that allows you to spy on one property of the component state, and run a function when that property value changes.
Please use syntax highlighting for your code.. This won't work because data won't update through the prop after initial instantiation. Normally you would use a computed property, but as you want to deeply watch the object you should watch the prop and then assign it to your data property. Changing CSS Custom Properties With Javascript. Where things get really interesting is when we use Javascript to change the values of custom properties. There are two things we need to be able to do to use Javascript with custom properties. We need to know how to get the value of a custom property and also how to set a new value on a custom ... USE AT YOUR OWN RISK. */ // object.watch if (!Object.prototype.watch) Object.prototype.watch = function (prop, handler) { var oldval = this[prop], newval = oldval, getter = function { return newval; }, setter = function (val) { oldval = newval; return newval = handler.call(this, prop, oldval, val); }; if (delete this[prop]) { // can't watch constants if (Object.defineProperty) // ECMAScript 5 Object.defineProperty(this, prop, { get: getter, set…
Respond To Change With Object Observe Web Google Developers
Use The Debugger Features Microsoft Edge Development
Solved Js Api Vue Watch For Data Store Changes Esri Community
How Javascript Works Tracking Changes In The Dom Using
Javascript Global Object Contentful
Debug Node Js Apps Using Visual Studio Code
Is It Possible To Change Javascript Variable Values While
Typescript Documentation Overview
Checking For Modified Or Changed Fields In Script
Top 18 Most Common Angularjs Mistakes That Developers Make
Clock In Javascript Displaying Current Date And Time With
How To Watch For Changes To The Dom Attribute Text Or Children Mutationobserver In Javascript
The 16 Javascript Debugging Tips You Probably Didn T Know
Method Vs Computed In Vue Stack Overflow
Watchkit Apple Developer Documentation
I Need The Javascript Code Of This File Read The I Chegg Com
Watch An Object For Changes In Vanilla Javascript By
Watching A Directory For Changes The Java Tutorials
The Mysterious Realm Of Javascriptcore
How To Create Interactive Websites With Javascript By
Use The Debugger Features Microsoft Edge Development
Guide Scopes Angular Js 1 8 W3cubdocs
Data Binding Revolutions With Object Observe Html5 Rocks
Javascript Changing Object Properties Stack Overflow
Webpack 5 Federation A Game Changer To Javascript
How To Declare A Watch On The This Object In A Factory Method
0 Response to "27 Javascript Watch Property Change"
Post a Comment