35 How To Build Json In Javascript
JSON Object Literals. JSON object literals are surrounded by curly braces {}. JSON object literals contains key/value pairs. Keys and values are separated by a colon. Each key/value pair is separated by a comma. It is a common mistake to call a JSON object literal "a JSON object". JSON cannot be an object. To start a schema definition, let's begin with a basic JSON schema. We start with four properties called keywords which are expressed as JSON keys. Yes. the standard uses a JSON data document to describe data documents, most often that are also JSON data documents but could be in any number of other content types like text/xml.
Build A Simple Json Prettier Viewer Or Editor In Browser
Sep 26, 2015 - In this short tutorial we'll take you through dynamically generating a JSON object within JavaScript... Let's say, for the sake of illustration, that we want to loop through a list of HTML elements and retrieve product data from which we will then use to create a JSON object for posting via AJAX.
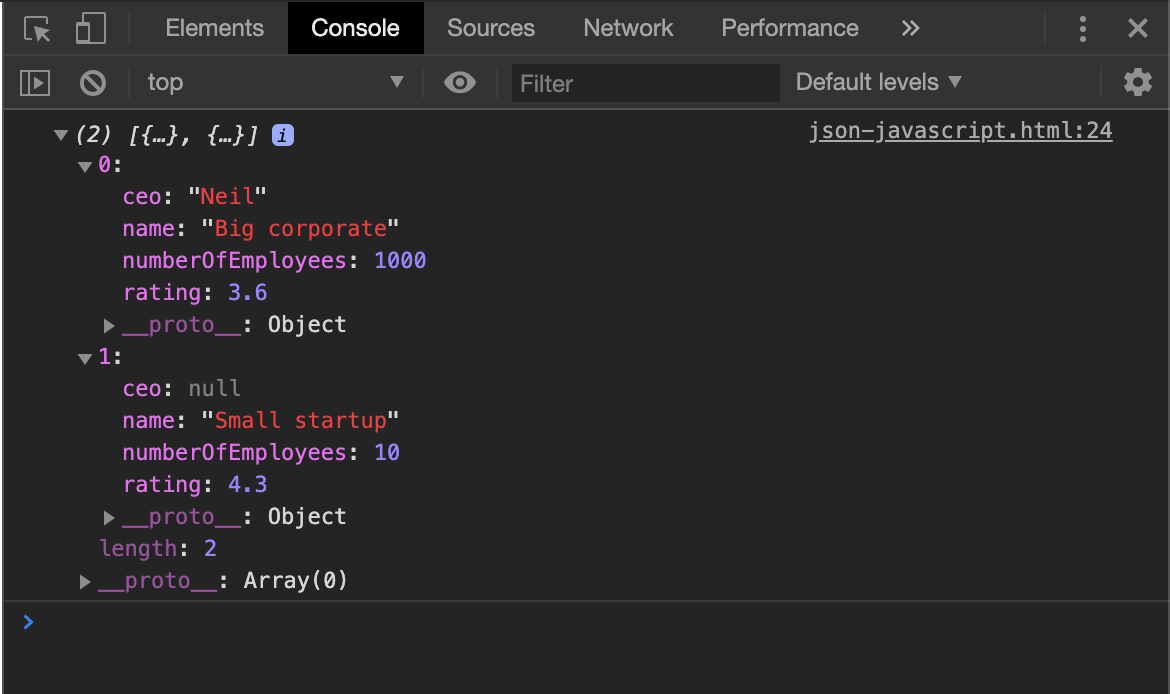
How to build json in javascript. JSON objects can be created with JavaScript. Let us see the various ways of creating JSON objects using JavaScript −. Creation of an empty Object −. var JSONObj = {}; Creation of a new Object −. var JSONObj = new Object (); Creation of an object with attribute bookname with value in string, attribute price with numeric value. Javascript Web Development Object Oriented Programming. To create an array from JSON data, use the concept of map () from JavaScript. Let's say the following is our data −. const studentDetails = [ { name : "John" }, { name : "David" }, { name : "Bob" } ]; Following is the code to create an array from the above data −. Nov 30, 2017 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers.
Because JSON is derived from the JavaScript programming language, it is a natural choice to use as a data format in JavaScript. JSON, short for JavaScript Object Notation, is usually pronounced like the name "Jason." To learn more about JSON in general terms, read the "An Introduction to JSON" tutorial. May 24, 2016 - Working with data from an art museum ... files into flat CSV files. ... Donate today! Great Open Access tutorials cost money to produce. Join the growing number of people supporting The Programming Historian so we can continue to share knowledge free of charge. ... JSON vs. JSON Lines ... JSON (JavaScript Object Notation) ... How to create a JSON object using Javascript? [closed] Ask Question Asked 9 months ago. Active 9 months ago. Viewed 2k times -1 Closed. This question needs details ... In Javascript, JSON objects are, well, Javascript objects.. You could simply write something like this:
Dec 08, 2015 - We’ll also use our newfound skills with JSON at the end of this project to build a quick app that loads photos from Flickr without requiring a page refresh. [demolink]See the Demo | Download the Source[/demolink] ... JSON is short for JavaScript Object Notation, and is a way to store information ... The JSON format is syntactically similar to the code for creating JavaScript objects. Because of this, a JavaScript program can easily convert JSON data into JavaScript objects. Since the format is text only, JSON data can easily be sent between computers, and used by any programming language. To format HSON string in JavaScript, use JSON.stringify() with some parameters. Following is the code −Examplevar details = { studentId: 101, studen ...
Jun 09, 2021 - JSON, or JavaScript Object Notation, is all around us. If you've ever used a web app, there's a very good chance that it used JSON to structure, store, and transmit data between its servers and your device. In this article, we'll briefly go over the differences between JSON and JavaScript, JSON is the most commonly used format for transmitting data (data interchange) from a server to a client and vice-versa. JSON data are very easy to parse and use. It is fast to access and manipulate JSON data as they only contain texts. JSON is language independent. You can create and use JSON in other programming languages too. Inside the JSON string there is a JSON array literal: ["Ford", "BMW", "Fiat"] Arrays in JSON are almost the same as arrays in JavaScript. In JSON, array values must be of type string, number, object, array, boolean or null. In JavaScript, array values can be all of the above, plus any other valid JavaScript expression, including functions ...
As we can see, the replacer of JSON.stringify() visits values top-down (root first, leaves last). The rationale for going in that direction is that we are converting JavaScript values to JSON values. And a single JavaScript object may be expanded into a tree of JSON-compatible values. The function JSON.stringify will turn your json object into a string: var jsonAsString = JSON.stringify(obj); In case the browser does not implement it (IE6/IE7), use the JSON2.js script. It's safe as it uses the native implementation if it exists. May 18, 2020 - In this tutorial, we’re going to learn about JSON. We will cover JSON structure, different data types and how to use JSON inside JavaScript.JSON is...
JavaScript Object Notation (JSON) is a standard text-based format for representing structured data based on JavaScript object syntax. It is commonly used for transmitting data in web applications (e.g., sending some data from the server to the client, so it can be displayed on a web page, or vice versa). 6/7/2019 · This is one of most common scenarios and you’ll see two ways of creating JSON array dynamically. The first method will use for loop for creating JSON array from an input array. let output = []; let input = [ " John " , " Hari " , " James " ] let tmp ; for ( let i = 0 ; i < input . length ; i ++ ){ tmp = { " name " : input [ i ]}; output . push ( tmp ); } console . log ( output ) To create an HTML list from JSON data: Parse the JSON data into an array first (assuming it is a flat array) - var data = JSON.parse (DATA); Loop through the array and create the HTML list. Lastly, append the list to where you want - document.getElementById (ID).appendChild (list); That should cover the basics, but read on for more examples!
JSON forEach tutorial shows how to loop over a JSON array in JavaScript. In this tutorial we use JSON server to handle test data. The json-server is a JavaScript library to create testing REST API. First, we create a project directory an install the json-server module. The JSON server module is installed globally with npm . Feb 07, 2013 - I am trying to construct JSON object in js. There are couple of posts already about this topic in stack overflow itself. Referred to How do i build JSON dynamically in javascript?. Have to construc... If I want to create JavaScript Object from string generated by for loop then I would JSON to Object approach. I would generate JSON string by iterating for loop and then use any popular JavaScript Framework to evaluate JSON to Object. I have used Prototype JavaScript Framework. I have two array with keys and values.
Use the JavaScript function JSON.parse() to convert text into a JavaScript object: const obj = JSON.parse('{"name":"John", "age":30, "city":"New York"}'); Make sure the text is in JSON format, or else you will get a syntax error. 3/12/2016 · "JSON" stands for "JavaScript Object Notation" - it's just a specification for representing some data in a string that explicitly mimics JavaScript object (and array, string, number and boolean) literals. You're trying to build up a JavaScript object dynamically - so the word you're looking for is "object". If you have defined tools needed for your build as development dependencies in your project's package.json or package-lock.json file, install these tools along with the rest of your project dependencies through npm. This will install the exact version of the tools defined in the project, isolated from other versions that exist on the build agent.
Jun 07, 2020 - Get code examples like "how to build json object in javascript" instantly right from your google search results with the Grepper Chrome Extension. Sep 26, 2015 - In this short tutorial we'll take you through dynamically generating a JSON object within JavaScript... Let's say, for the sake of illustration, that we want to loop through a list of HTML elements and retrieve product data from which we will then use to create a JSON object for posting via AJAX. Convert the request into an object, using the PHP function json_decode(). Access the database, and fill an array with the requested data. Add the array to an object, and return the object as JSON using the json_encode() function.
In JavaScript, JSON objects can contain other JSON objects, nested arrays, arrays, arrays of the JSON object, and so on. In mention below example, you will learn how to extract all the values and parse a nested JSON in JavaScript. 18/8/2020 · We can use any of the two ways to access the json file −. Using require module. Code to access employees.json using require module −. const data = require('./employees.json'); console.log(data); Using fetch function. Code to access employees.json using fetch function − Converting a JSON Text to a JavaScript Object. A common use of JSON is to read data from a web server, and display the data in a web page. For simplicity, this can be demonstrated using a string as input. First, create a JavaScript string containing JSON syntax:
Parsing Options. The JSON.parse() method accepts a function that is called on each key-value pair.. The function receives two arguments, the key and the value, and needs to return a value. If the function returns undefined, then the key is removed from the result; if it returns any other value, that value is inserted into the result. May 23, 2017 - Not the answer you're looking for? Browse other questions tagged javascript jquery json or ask your own question. ... Two Germanys and two Koreas but only one China. Why wasn't a compromise to internationally recognise both Chinas reached? This is vanilla JavaScript and this is how we did front-end development in the "old" days :). Step 2 - Loop through every object in our JSON object. Next step is to create a simple loop. We can then get every object in our list of JSON object and append it into our main div.
Sep 11, 2020 - JSON (JavaScript Object Notation) is a lightweight format for sharing data. Although it’s derived from JavaScript — it may be used with many programming languages. In this article however, we’ll be… Aug 12, 2016 - This questions does not make sense ... from and in what format. Are you just adding to existing data or creating all of it from scratch. Maybe you can create a jsfiddle to demonstrate what the problem you are having is.Is your question really just, how to access data within an array/ or javascript object? And lets be clear about the data, JSON or Javascript ... JSON - Overview. JSON or JavaScript Object Notation is a lightweight text-based open standard designed for human-readable data interchange. Conventions used by JSON are known to programmers, which include C, C++, Java, Python, Perl, etc. JSON stands for JavaScript Object Notation. The format was specified by Douglas Crockford.
var obj = JSON.parse(data); obj.notes.push(addition); var json = JSON.stringify(obj, null, 2); In the following code, we declare a variable named obj that parses the data from the code.json file. We then "push" the object addition into the notes array. Next, in the same above code, we stringify i.e. create an object into a JSON string. JSON is lightweight and language independent and that is why its commonly used with jQuery Ajax for transferring data. Here, in this article I’ll show you how to convert JSON data to an HTML table dynamically using JavaScript. In addition, you will learn how you can dynamically create a table in JavaScript using createElement() Method. The function JSON.stringify will turn your json object into a string: var jsonAsString = JSON.stringify(obj); In case the browser does not implement it (IE6/IE7), use the JSON2.js script. It's safe as it uses the native implementation if it exists.
10/10/2013 · To access the JSON object in JavaScript, parse it with JSON.parse (), and access it via “.” or “ []”. JavaScript. <script> var data = ' {"name": "mkyong","age": 30,"address": {"streetAddress": "88 8nd Street","city": "New York"},"phoneNumber": [ {"type": "home","number": "111 111-1111"}, {"type": "fax","number": "222 222-2222"}]}' ; var json = JSON ... Dec 08, 2015 - I got a situation where I would like to read some data off a JSON format through PHP, however I am having some issues understanding how I should construct the Javascript object to create the JSON f... Transforming JSON Data in JavaScript. The JSON format is syntactically similar to the way we create JavaScript objects. Therefore, it is easier to convert JSON data into JavaScript native objects. JavaScript built-in JSON object provides two important methods for encoding and decoding JSON data: parse() and stringify().
Top 5 Online Json Courses According To Reviews Feb 2021
How To Create A Json File Learning Container
Cannot Find Module Build Info Json Discord
Github Josdejong Jsoneditor A Web Based Tool To View Edit
Json Editing In Visual Studio Code
How To Write Json Object To File In Java Crunchify
How To Read And Write Json File Using Node Js Geeksforgeeks
How To Build Json With Javascript Vr Orchestrator Nerd On
Json Creation How To Create Json Objects Using C Code
How To Extract Data From Json In Javascript Geeksread
Build Json Array From Thing And Referenced List Of Things And
Convert Json Data Dynamically To Html Table Using Javascript
How To Generate Webpack Stats Json File Get Help
What Is Json A Better Format For Data Exchange Infoworld
Parse Json And Store Json Data Using Node Js Codez Up
Reshaping Json With Jq Programming Historian
How To Read Local Json File In React Js By Rajdeep Singh
Creating Node Js Modules Npm Docs
Json Tutorial Learn How To Use Json With Javascript
Json Array In Javascript Revisited Codehandbook
Reactjs A Quick Tutorial To Build Dynamic Json Based Form
Creating A Simple Csv To Json Editing Workflow Via Js By
Json Form Javascript Library To Build Forms From Json
How To Read And Write A Json Object To A File In Node Js
Java Api For Json Processing Tutorial 04 Generate Json W The Object Model Api
All Possible Ways Of Making An Api Call In Javascript By
Dynamically Building Nested List From Json Data And Tree View
Download Json In Action Build Json Based Applications
Load And Render Json Data Into React Components Pluralsight
Using Json To Build Efficient Applications Lansa
Convert Java Object To Json String Using Jackson Api
Build A Json File Validator Linter And Formatter Online Tool
0 Response to "35 How To Build Json In Javascript"
Post a Comment