20 Javascript Read Text Input
There is a built-in Module or in-built library in NodeJs which handles all the reading operations called fs (File-System). It is basically a JavaScript program (fs.js) where function for reading operations is written. Import fs-module in the program and use functions to read text from the files in the system. How to read/write data from/to .properties file in Java? Read/Write Class Objects from/to File in C++; Write a Python program to read an Excel data from file and read all rows of first and last columns; How to create a file, write data into it and read data from it on iOS? How to read a cookie using JavaScript? Read and write WAV files using ...
Css Stylish Custom Checkbox And Radio Input By Mahmoud
8/9/2017 · The JavaScript input text property is used to set or return the value of a text input field. The value property contains either the default value that is present upon loading the element, the value entered by the user, or the value assigned by the script.
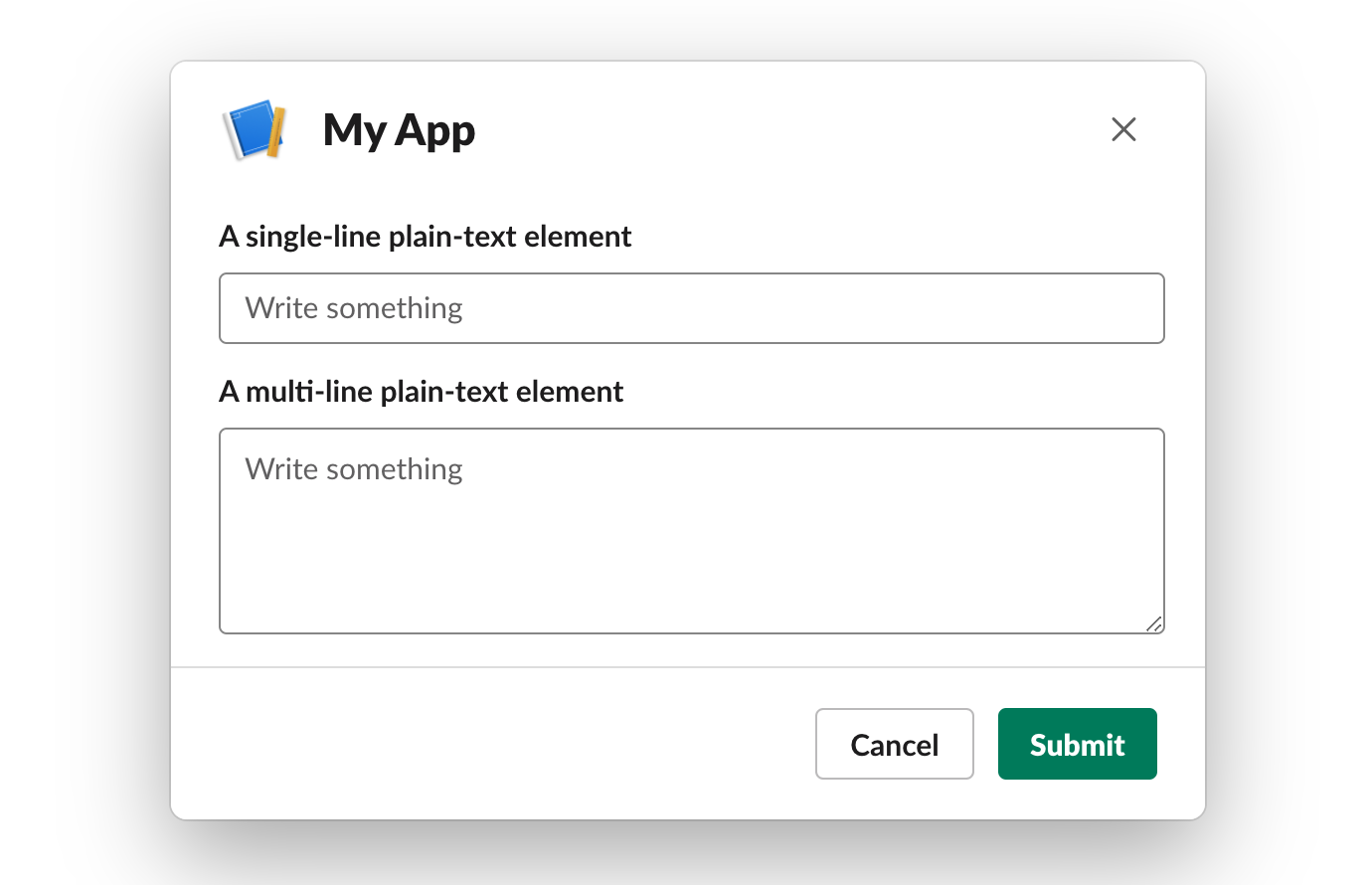
Javascript read text input. JavaScript does not have direct access to the local files due to security and privacy. We can offer the user the possibility to select files via a file input element that we can then process. The file input has a files property with the selected file(s). FileReader.readAsText (): Reads the contents of the specified input file. The result attribute contains the contents of the file as a text string. This method can take encoding version as the second argument (if required). The default encoding is UTF-8. In this case we are using FileReader.readAsText () method to read local .txt file. This code ... 21/12/2020 · Talking Ghost Chat Input. A little concept of a ghost reading your input text and "hiding" when you are typing out your message. Compatible browsers: Chrome, Edge, Firefox, Opera, Safari. Responsive: no. Dependencies: -
Methods of JavaScript FileReader. Learn the Methods of javaScript filereader. FileReader.abort(): This method aborts or stops the read operation and makes the readyState value to DONE. FileReader.readAsArrayBuffer(): Its returns result attribute contains the ArrayBuffer object once finished which contains all the contents read from the Blob which is started for reading. Summary. File objects inherit from Blob.. In addition to Blob methods and properties, File objects also have name and lastModified properties, plus the internal ability to read from filesystem. We usually get File objects from user input, like <input> or Drag'n'Drop events (ondragend).. FileReader objects can read from a file or a blob, in one of three formats: Text files (TXT, CSV, JSON, HTML etc) can be read using the readAsText () method. Binary files (EXE, PNG, MP4 etc) can be read using the readAsArrayBuffer () method. Data url string can be read using the readAsDataURL () method.
15/4/2019 · We can get the value of text input field using various methods in script. There is a text value property which can set and return the value of the value attribute of a text field. Also we can use jquery val() method inside script to get or set the value of text input field. Using text value property: Syntax: The readOnly property sets or returns whether a text field is read-only, or not. A read-only field cannot be modified. However, a user can tab to it, highlight it, and copy the text from it. Tip: To prevent the user from interacting with the field, use the disabled property instead. In this video, you will learn how to get and set input text value in javascript. In this video, you will learn how to get and set input text value in javascript.
Answer: Use the value Property. You can simply use the value property of the DOM input element to get the value of text input field. The following example will display the entered text in the input field on button click using JavaScript. The JavaScript FileList and File Objects Whether you include the multiple attribute or not, the file input always returns a FileList object, which is a simple array of individually selected files from the underlying system. Like any array, it is zero based, so files gets the first one. To read any file in javascript, you have to make use of FileReader object. You can read all kind of file types such jpeg, txt, json, png, etc. For images, you can make use of readAsDataURL () function to get base64 encoded string and use it directly as source in image tag. Please check out base64 to image converter online tool to see it in action.
In this video tutorial, you will learn how to read text file in javascript line by line.Source Code:https://www.fwait /how-to-read-text-file-in-javascript... The JavaScript Confirmation Message Box The window.prompt method is one way to read user input, but JavaScript also provides a way to get confirmation from the user. For instance, you might want to confirm that the user has entered the right information and wants to continue with payment. getElementsByClassName class selector to read input value. This is an method in document object to select the elements with classname. getElementsByClassName returns the all the elements with classname matched.. It is class select, return HTMLCollection. Input is defined with class value.
In the JavaScript code we have a function called say_hi. It used the getElementById we have already seen to locate the DOM element representing the input element with the id first_name. The object returned has a method value that will return the text the user has typed in that field. We use this technique to retrieve the content of both input ... PDF - Download JavaScript for free Previous Next This modified text is an extract of the original Stack Overflow Documentation created by following contributors and released under CC BY-SA 3.0 Right, but with little fantasy it could be modified to read :-) Using jQuery is more elegant, please see my answer. :-)--SA
Input native events read values. By default html input elements provides native events, Angular also supports this events to read input text elements in controller. Following events we can use. onBlur event: client leaves from input element. onChange: Fired, when input element value changed. input event: fired when user typed the data To extract the information which a user enters into the text fields using Javascript, the Javascript code to do would be: The line document.getElementById ("firstname").value accesses the text box with an id of firstname and retrieves the value which the user has entered into this text box. On the server, Node has a filesystem package [code ]fs[/code] you can use to read file. On the browser, you would normally use HTML form element as such for file ...
In your web developer console, you will see the file contents read out using.text (),.stream (),.buffer (), and.slice (). This approach uses ReadableStream, TextDecoder (), and Uint8Array (). Applying FileReader Lifecycle and Methods There are 6 main events attached to FileReader: There are several methods are used to get an input textbox value without wrapping the input element inside a form element. Let’s show you each of them separately and point the differences. The first method uses document.getElementById('textboxId').value to get the value of the box: The maximum number of characters (as UTF-16 code units) the user can enter into the text input. This must be an integer value 0 or higher. If no maxlength is specified, or an invalid value is specified, the text input has no maximum length. This value must also be greater than or equal to the value of minlength.. The input will fail constraint validation if the length of the text value of the ...
You can see this in action in the input-type-file Glitch demo. Read a file's content # To read a file, use FileReader, which enables you to read the content of a File object into memory. You can instruct FileReader to read a file as an array buffer, a data URL, or text. Input Text Object Properties. Property. Description. autocomplete. Sets or returns the value of the autocomplete attribute of a text field. autofocus. Sets or returns whether a text field should automatically get focus when the page loads. defaultValue. Sets or returns the default value of a text field. In this lesson we'll take a look at how to write programs in JavaScript which accept user input. The Code. Programs which get input from the user need to be written in a specific way. The program will essentially prompt the user for information, ...
Fallback required.'); return false; } } /** * read text input */ function readText(filePath) { var output = ""; //placeholder for text output if(filePath.files && filePath.files[0]) { reader.onload = function (e) { output = e.target.result; displayContents(output); };//end onload() reader.readAsText(filePath.files[0]); }//end if html5 filelist support else if(ActiveXObject && filePath) { //fallback to IE 6-8 support via ActiveX try { … The reader emits a 'load' event (similar to images for example) that tells our code that the reading is finished. The reader keeps the file content in its result property. The data in this property depends on which method we used to read the file. In our example we read the file with the readAsText method, so the result will be a string of text.
How To Manage Text Input And Output With Javascript For Html5
Read Text Files Directly Into Thunkable Thunkable Tutorials
Javascript Algorithm Funny String By Erica N Javascript
How To Read A Local File Using Javascript Txt Json Etc
Custom Filterable Select Input In Pure Javascript Css Script
Html Clearing The Input Field Geeksforgeeks
How To Auto Calculate The Sum Of Input Values Using Jquery Or
Question 6 1 Pts Which Is Not True Of The Password Chegg Com
How Can I Read A File From An Html Input With Js Xlsx
New Feature Customize Your Clickdimensions Forms With Css
How To Copy Page Url And Paste Input Text In Javascript Code
Readonly Inputs Don T Look Like Inputs Issue 1129
Change Values In Read Only Fields Javascript Executor
How To Get Values From Html Input Array Using Javascript
How To Enable Disable An Element Using Jquery And Javascript
How The Fetch The Value Of Textbox Using Javascript In
Copy Text To Clipboard With Javascript Coders Zine
Reference Block Elements Slack
0 Response to "20 Javascript Read Text Input"
Post a Comment