35 Submit Button Validation In Javascript
No I want to check all these input elements using javascript validation. I want this to performed when a button "Archive" is pressed. My problem is this "Archive button" needs to be a submit button. This does run the javascript validation but after completing the validation it submits the form. Note that we are using all client side JavaScript validation here. Once all data are validation is passed then we will display the button ( or enable it ) for the user to submit the form. We will track changes of all the elements of the form and apply data validation once any change or data is entered on the form.
How To Do Ajax Form Validation With Inline Error Messages
Dec 29, 2011 - Inside the Javascript, when validated, do form.submit() or else return false.
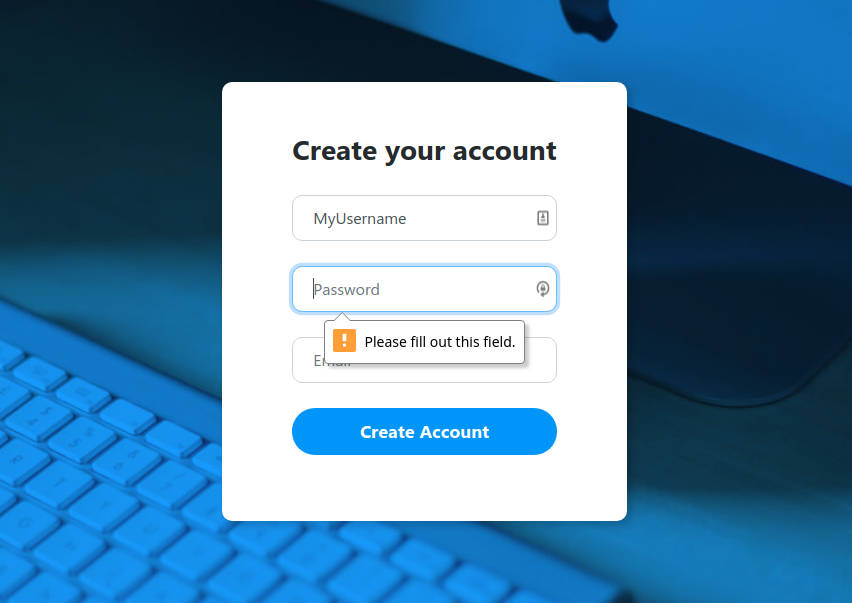
Submit button validation in javascript. Javascript answers related to "javascript onclick submit form validation" angularjs form validation on submit; buttons js before submit; form validation in javascript; How can I set the form action through JavaScript? how to create a form without a submit button javascript; javaScript disable submit button until form is fully validated When a user clicks on submit button of a form, JavaScript onsubmit event will call a function. Invoking JavaScript function on form submission: <form action="#" method="post" onsubmit="return ValidationEvent ()"> In our example, we call ValidationEvent () function on form submission. Enabling submit button only when all fields are valid. The following example will disable the Submit button as long as there is at least one invalid field. In case all of fields are valid, the button will be enabled. We can archive it by using the onStatusChanged option provided by the FieldStatus plugin.
Submit and Validate HTML Form Using JavaScript October 27, 2018 JsTutorials Team javascript This is simple JavaScript tutorial to submit html form using javascript, I am creating HTML form and validation data using javascript, after successfully validated data , We will submit form into server side to update db or send email etc. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Basic Form Validation in JavaScript In the past, form validation would occur on the server, after a person had already entered in all of their information and pressed the submit button.
JavaScript File - formvalid.js It includes various function to check validity of entered information. Like, formValidation () is the main function that runs as soon as the user clicks on submit button. Here, object is defined for each field. When present, it specifies that the form-data should not be validated on submission. This attribute overrides the form's ... The formnovalidate attribute is only used for buttons with type="submit". ... The numbers in the table specify the first browser version that fully supports the attribute. sample-registration-form-validation.js is the external JavaScript file which contains the JavaScript ocde used to validate the form. js-form-validation.css is the stylesheet containing styles for the form. Notice that for validation, the JavaScript function containing the code to validate is called on the onSubmit event of the form.
After you write the validation function, you need to tell your form to run the script when someone clicks the Submit button. To do this, you need to use the onsubmit event for the form. For more information, see onsubmit Event. (The onsubmit event occurs every time a form is submitted for ... First, you need to design the HTML page that contains the form using an HTML editor. Next, you have to create a set of input validations using JavaScript. The final step is to create a form processor script that collects the data submitted in the form, validates it again and then sends it as ... Feb 26, 2019 - Validating form input with JavaScript is easy to do and can save a lot of unnecessary calls to the server. It can prevent people from leaving fields blank, from entering too little or too much or from using invalid characters. User-submitted data should also always be verified using a secure ...
Dec 26, 2019 - Client-side form validation sometimes requires JavaScript if you want to customize styling and error messages, but it always requires you to think carefully about the user. Always remember to help your users correct the data they provide. To that end, be sure to: Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. JS Examples JS HTML DOM JS HTML Input JS HTML Objects JS HTML Events JS Browser JS Editor JS Exercises JS Quiz JS Certificate ... HTML form validation can be done by JavaScript. If a form field (fname) is empty, this function alerts a message, and returns false, to prevent the form from being submitted...
Look at the preceding source code closely; see the ids of controls that play an important role in JavaScript validation by reading the ASP.Net control values in JavaScript code. ... To call the JavaScript function on the ASP.Net button we need to call the function in the ASP.Net button's OnClientClick property. Malicious users can disable JavaScript and submit bad data to your server. In this tutorial, you're going to focus on the client-side validation only. Client-side validation options. When it comes to client-side validation, you'll have two options: JavaScript validation: you develop the validation logic using JavaScript. Client-side validation usually means: JavaScript intercepting the form before it's submitted to check for errors, possibly using regex. This saves the trip to the server but still uses a bit of code. While we should still use server-side validation, I'm going to discuss how we can make the most of client-side validation to reduce the number ...
May 14, 2017 - I'm trying to validate my form with the easiest way possible, but somehow it is not working and when I click submit it just takes me to the next page without giving the alert message: HTML: Definition and Usage. The formnovalidate attribute is a boolean attribute. When present, it specifies that the form-data should not be validated on submission. This attribute overrides the form's novalidate attribute. The formnovalidate attribute is only used for buttons with type="submit". A new JavaScript method that validates forms before submitting. People started to work on a solution to this behavior in June 2019 (the proposal is an interesting read). The HTMLFormElement now includes a new method called requestSubmit. And this method does the same as clicking a submit button. 🎉.
Finally, the form tag includes an onsubmit attribute to call our JavaScript validation function, validate_form(), when the "Send Details" button is pressed. The return allows us to return the value true or false from our function to the browser, where true means "carry on and send the form to the server", and false means "don't send ... TOP Interview Coding Problems/Challenges Run-length encoding (find/print frequency of letters in a string) Sort an array of 0's, 1's and 2's in linear time complexity JavaScript code for validating TextBox on Button Click When the Validate Button is clicked, the ValidateTextBox JavaScript function is called. Inside the function, first the TextBox is referenced using JavaScript and then its value is trimmed and compared with an Empty string.
Forms are ubiquitous in web applications. Some apps use forms to collect data to sign up users and provide an email address. Others use them to fulfill online transactions to facilitate a shopping experience. You might use some web forms to apply for a new car loan, whereas you'll use Although the Button may validate different validation groups, it always submits a definite HTML form - the one in which it is nested. To avoid mixing up validated and submitted values, we recommend that a single HTML form contain only a single validation group. You can check a radio button in the same way as a checkbox. Keep Learning // Advanced DOMs → Go! Go! Other JavaScript Articles // Basic JavaScript · Event Handlers · Writing Scripts · Functions · Objects and Properties · Form Validation · Advanced DOMs · Support Detection · Popup ...
Submit button validation in javascript. What we will cover in this article Forms are ubiquitous in web applications I have a function which checks if all fields in the form are filled before enabling the submit button, however if the previously mentioned fields are invalid (but filled), the button will still be enabled and allow submission You can validate jQuery form using button click using.valid () method, so you code will be something like this //This is used to initialize the validation on form and applying rules,conditions $ ("#ConnectionWiseForm").validate ({ // Specify validation rules rules: { // The key name on the left side is the name attribute // of an input field. JavaScript provides facility to validate the form on the client-side so data processing will be faster than server-side validation. Most of the web developers prefer JavaScript form validation. Through JavaScript, we can validate name, password, email, date, mobile numbers and more fields.
The easiest way would be to call formvalid () onkeyup for every field. That function validates every field and shows the button if they are valid. This should do the job, although it is not very efficient. It is a job in vain to check every field every time you type anything on any field. It is not as simple as calling form.submit () or clicking on the submit button. When the form is submitted, the submit event is fired right before the request is sent to the server. This is where JavaScript will come into action and validate the data entered. We can even create objects and add various attributes. buttons: Function: It is a function that accepts the current form element and returns the list of submit buttons. By default, the plugin ignores all the submit button/input which have the formnovalidate attribute.
Form validation normally used to occur at the server, after the client had entered all the necessary data and then pressed the Submit button. If the data entered by a client was incorrect or was simply missing, the server would have to send all the data back to the client and request that the form be resubmitted with correct information.
Simple Form Validation In Reactjs Example Skptricks
Changing Input Type Button To Submit Button To Validate
Form Validation Using Jquery Geeksforgeeks
Form Validation In React Client Side Validation Is The
Javascript Onsubmit Event With Form Validation Formget
Validate Js Declarative Validation With Javascript Jquery
Angularjs Form Validation On Submit Form With Example
Javascript Form Validation Script More Features Javascript
Password Validation Using Regular Expressions And Html5
Simple Form Validation In React Js Medium
Github Patwan Log In Form Validation Using Javascript
Javascript Validation In Asp Net Website Asp Net C Net Vb
Form Validation In Javascript Qa With Experts
Javascript Login Form Validation Formget
How To Submit Ajax Forms With Jquery Digitalocean
How To Create A Password Validation Form
Html 5 Form Validation With Javascript Jquery And Ajax The
How To Form Submissions With Flask And Ajax By Louis De
Validate Html Forms With Javascript And Html
Form Validation Bootstrap Studio
Javascript Form Validation Is Used For Validate The User S
How To Add Form Validation For Empty Input Fields With
Execute Javascript On Save Only When Form Is Valid Nintex
Javascript Form Validation Different Types Of Javascript
Form Validation With Php Sitepoint
Submit Form Without Page Refresh Using Ajax Jquery And Php
Form Validation Lt Javascript The Art Of Web
Javascript A Sample Registration Form Validation W3resource
Why You Should Be Using Html5 Form Validation A Tour Pageclip
Can You Trigger Custom Html5 Form Errors With Javascript
0 Response to "35 Submit Button Validation In Javascript"
Post a Comment