24 Javascript Check If Value Is In Array
Check whether an object is an array: ... Return Value: A Boolean. Returns true if the object is an array, otherwise it returns false: JavaScript Version: ECMAScript 5: Related Pages. Array Tutorial. Array Const. Array Methods. Sorting Arrays. Array Iterations Previous JavaScript Array Reference Next ... // program to check if an array contains a specified value const array = ['you', 'will', 'learn', 'javascript']; const hasValue = array.indexOf('javascript') !== -1; // check the condition if(hasValue) { console.log('Array contains a value.'); } else { console.log('Array does not contain a value.'); } Output. Array contains a …
1 week ago - The indexOf() method returns the first index at which a given element can be found in the array, or -1 if it is not present.
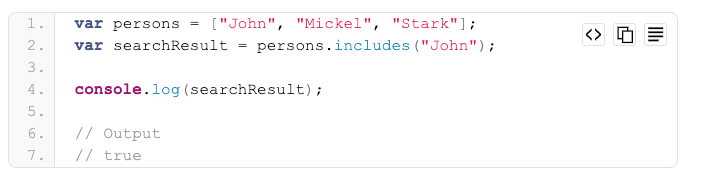
Javascript check if value is in array. In case no element is less than or equal zero, the value of the result variable remains true. This code is simple and straight forward. However, it is quite verbose. JavaScript Array type provides the every () method that allows you to check if every element of an array pass a test in a shorter and cleaner way. The $.inArray () method is similar to JavaScript's native .indexOf () method in that it returns -1 when it doesn't find a match. If the first element within the array matches value, $.inArray () returns 0. Because JavaScript treats 0 as loosely equal to false (i.e. 0 == false, but 0 !== false), to check for the presence of value within array ... In javascript we can check whether a variable is array or not by using three methods. 1) isArray () method The Array.isArray () method checks whether the passed variable is array or not. If the variable is an array it displays true else displays false.
JavaScript contains a few built-in methods to check whether an array has a specific value, or object. In this article, we'll take a look at how to check if an array includes/contains a value or element in JavaScript. Check Array of Primitive Values Includes a Value indexOf () compares searchElement to elements of the Array using strict equality (the same method used by the === or triple-equals operator). $ ("#add-employeeId").val () is returning string value and you are comparing it to int values. Your function should be: function isInArray (array, search) { return array.indexOf (parseInt (search)) >= 0; } Sep 02, 2020 - Today, we’re going to learn how to check if an item is an array with vanilla JS. Let’s dig in. The problem In JavaScript, the typeof operator returns a string indicating the type of an object. Or, at least, it’s supposed to. But it doesn’t always return the result you would expect for ...
To check if an array contains a primitive value, you can use the array method like array.includes() The following example uses the array.includes()method to check if the colorsarray contains 'red': constcolors = ['red', 'green', 'blue']; indexOf () Method The simplest and fastest way to check if an item is present in an array is by using the Array.indexOf () method. This method searches the array for the given item and returns its index. If no item is found, it returns -1. You can use the indexOf () method to check whether a given value or element exists in an array or not. The indexOf () method returns the index of the element inside the array if it is found, and returns -1 if it not found. Let's take a look at the following example:
The every () method returns true if all elements in an array pass a test (provided as a function). The method executes the function once for each element present in the array: If it finds an array element where the function returns a false value, every () returns false (and does not check the remaining values) callbackFn is invoked only for array indexes which have assigned values. It is not invoked for indexes which have been deleted, or which have never been assigned values. callbackFn is invoked with three arguments: the value of the element, the index of the element, and the Array object being traversed.. If a thisArg parameter is provided to every, it will be used as callback's this value. Jul 20, 2021 - The value to be checked. ... If the value is an Array, true is returned; otherwise, false is. See the article “Determining with absolute accuracy whether or not a JavaScript object is an array” for more details. Given a TypedArray instance, false is always returned.
Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. 3.Check value exists in array using Javascript Array filter There is one more alternative way to check whether a particular value exists in an array of objects using the javascript array filter. it returns another array of objects if the value exists and you can use the length() method to check the value exists or not. See below code example - <script type = 'text/javascript' > //code to check if a value exists in an array using javascript for loop var fruits_arr = ['Apple', 'Mango', 'Grapes', 'Orange', 'Fig', 'Cherry']; function checkValue(value, arr) { var status = 'Not exist'; for (var i = 0; i < arr.length; i++) { var name = arr[i]; if (name == value) { status = 'Exist'; break; } } return status; } console.log('status : ' + checkValue('Mango', fruits_arr)); console.log('status : …
You can use the JavaScript Array.isArray() method to check whether an object (or a variable) is an array or not. This method returns true if the value is an array; otherwise returns false. A Boolean which is true if the value searchElement is found within the array (or the part of the array indicated by the index fromIndex, if specified).. Values of zero are all considered to be equal, regardless of sign. (That is, -0 is considered to be equal to both 0 and +0), but false is not considered to be the same as 0. Unfortunately, that isn't really helpful for us who just want to check if the value is an array or not 🙊 ... : Primitive is data type that is not an object and has no methods. All primitives are immutable (ie. they can't be altered). They are stored by value · In JavaScript, there are 6 ...
Code to check if an array is empty using javascript We will quickly go over the code and its demonstration to check if an array is empty or not and also see why these specific functions are used. //To check if an array is empty using javascript function arrayIsEmpty(array) { //If it's not an array, return FALSE. A primitive value in JavaScript is a string, number, boolean, symbol, and special value undefined. The easiest way to determine if an array contains a primitive value is to use array.includes () ES2015 array method: const hasValue = array.includes(value[, fromIndex]); The first argument value is the value to search in the array. From line 3 of the code, you can see that we have an array of fruits with the name fruits_arr. This contains 6 elements namely Apple, Mango, Grapes, Orange, Fig and Cherry. The function checkValue takes 2 parameters as input, the value that needs to be searched and the array in which the value needs to be searched.
Apr 28, 2021 - This post will discuss how to check whether an array contains a certain value in JavaScript... In modern browsers, you can use the `includes()` method to check the presence of an element in an array, returning true or false as appropriate. 2 weeks ago - What is the most concise and efficient way to find out if a JavaScript array contains a value? This is the only way I know to do it: function contains(a, obj) { for (var i = 0; i Most of the scripting language has an in_array method to check an item exists inside an array or not. In JavaScript, it is a little different compare to other scripting languages. Until ECMAScript 7, there was no standard method to check this. The modern JavaScript ECMAScript 7 introduces Array#includes for checking that. Before introducing Array#includes, JavaScript quite a few ways to do in ...
Jun 04, 2021 - In this tutorial, I show How you can check whether an Array already contains a specific value or not. This requires within the program in some cases like - Stop new value from insert if it already exists in an Array, execute script when the Array contains the particular value, etc.. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. There are multiple methods available to check if an array contains duplicate values in JavaScript. You can use the indexOf () method, the Set object, or iteration to identify repeated items in an array.
indexof () method The indexof () method in Javascript is one of the most convenient ways to find out whether a value exists in an array or not. The indexof () method works on the phenomenon of index numbers. This method returns the index of the array if found and returns -1 otherwise. Check if a value exists in array in Javascript with code snippet. In this chapter, you will learn about how to check if a value exists in an array in Javascript. When you have an array of the elements, and you need to check whether the certain value exists or not. Solution 1: The includes () method returns true if an array contains a specified element, otherwise false. includes () is case sensitive.
Definition and Usage. The some() method checks if any of the elements in an array pass a test (provided as a function).. some() executes the function once for each element in the array: If it finds an array element where the function returns a true value, some() returns true (and does not check the remaining values); Otherwise it returns false; some() does not execute the function for empty ... In modern browsers which follow the ECMAScript 2016 (ES7) standard, you can use the function Array.prototype.includes, which makes it way more easier to check if an item is present in an array: const array = [1, 2, 3]; const value = 1; const isInArray = … Using the .find () Function to Find Element in an Array in Javascript. Another way to check if an element is present in an array is by using the .find () function. As compared to the .indexOf () and .includes () functions, .find () takes a function as a parameter and executes it on each element of the array. It will return the first value which ...
This is the common way through which one can check whether the value exists in an array in javascript or not. let res10 = false for (const item of array){ if(item === value) res10 = true } console.log(res10) The some () method takes a callback function, which gets executed once for every element in the array until it does not return a true value. The some () method returns true if the user is present in the array else it returns false. You can use the some () method to check if an object is in the array. Code Recipe to check if an array includes a value in JavaScript using ES6
Now in this example,You can use indexOf() function for check the value exists in an array or no. it is function returns the true and false value. see below example of indexOf().
Javarevisited 3 Ways To Find Duplicate Elements In An Array
Check If A Value Exists In Array In Javascript Learn Simpli
How To Check If A Javascript Array Contains A Specific Value
How To Check If Array Contains Duplicate Values Javascript
Check If Value Exists Jquery In Array Jquery Inarray
Javascript How To Check If An Array Has Duplicate Values
How To Check If A Value Exists In An Array In Php Code Examples
How To Check If A String Contains At Least One Number Using
How To Check Array Contains A Value In Javascript Scratch Code
How To Check If A Javascript Array Contains A Specific Value
Check If All Values In Array Are True Javascript Code Example
Should You Use Includes Or Filter To Check If An Array
Check If An Array Includes A Value Using Javascript
How To Filter Out Only Numbers In An Array Using Javascript
Javascript Inarray Check If Value Exists Pakainfo
2 Ways To Check If Value Exists In Javascript Object
How To Check If A Variable Is An Array In Javascript
Javascript Array Splice Delete Insert And Replace
Test If Object Array Or String Issue 3457 Facebook
Check For Value Exist In Array Modeler Camunda Platform Forum
Here Are The New Built In Methods And Functions In Javascript
How To Check If Value Exists In A Javascript Array Xpertphp
0 Response to "24 Javascript Check If Value Is In Array"
Post a Comment