28 Javascript Reduce Example Es6
Checking whether an integer number is in the safe range, i.e., it is correctly represented by JavaScript (where all numbers, including integer numbers, are technically floating point number). ECMAScript 6 — syntactic sugar: reduced | traditional The reduce () method executes the callbackFn once for each assigned value present in the array, taking four arguments: accumulator. currentValue. currentIndex. array. The first time the callback is called, accumulator and currentValue can be one of two values. If initialValue is provided in the call to reduce () , then accumulator will be equal ...
Learn Map Filter And Reduce In Javascript By Joao Miguel
const total = arr.reduce ( (acc, item) => acc+= item, 0); // return a total as 15. Reduce works on an array but can return anything you want it to return. As the name speaks for itself, it can be reduced to anything and can behave like map, find, filter or any other JavaScript array function. The above code snippet works on an array and reduces ...
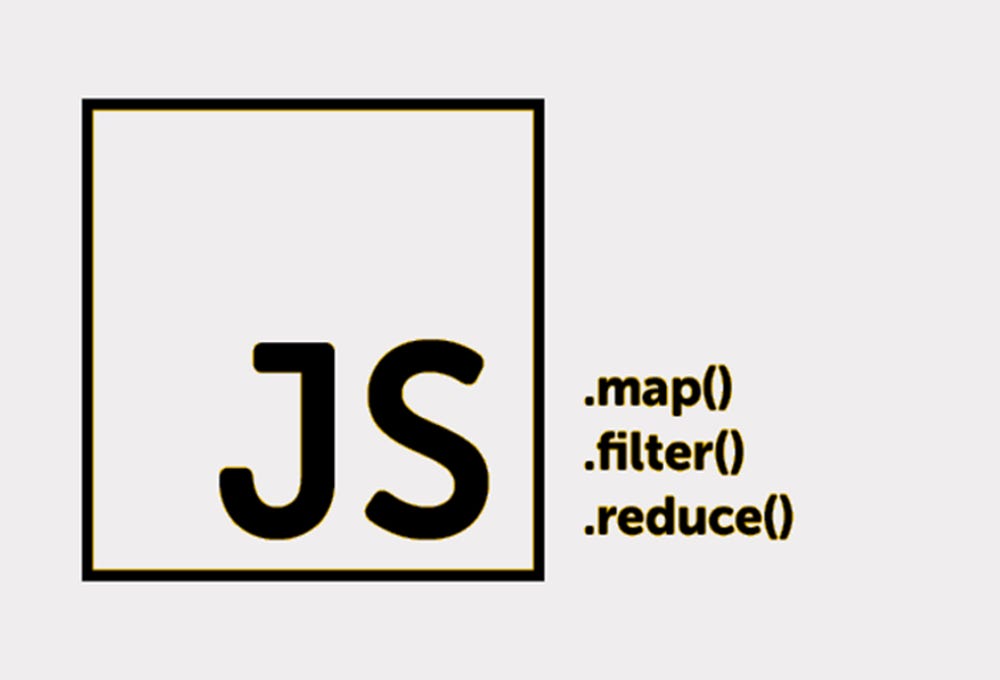
Javascript reduce example es6. An object can be turned into an array with: Object.entries(), Object.keys(), Object.values(), and then be reduced as array.But you can also reduce an object without creating the intermediate array. I've created a little helper library odict for working with objects.. npm install --save odict reduce() method applies a function simultaneously against two values of the array (from left-to-right) as to reduce it to a single value. Syntax array.reduce(callback[, initialValue]); Parameter Details. callback − Function to execute on each value in the array. initialValue − Object to use as the first argument to the first call of the callback. Feb 20, 2021 - The reduce() method executes a user-supplied “reducer” callback function on each element of the array, passing in the return value from the calculation on the preceding element. The final result of running the reducer across all elements of the array is a single value.
The call, apply and bind methods are NOT suitable for Arrow functions -- as they were designed to allow methods to execute within different scopes -- because Arrow functions establish "this" based on the scope the Arrow function is defined within. For example call, apply and bind work as expected with Traditional functions, because we establish the scope for each of the methods: All the JavaScript (ES6+) Array methods you need. George Roubie. Jul 16 · 11 min read. I will explain to you with examples the methods: filter, map, reduce, find, findIndex, includes, some, every, flat and flatMap. Get ready because this article is huge, but it will be a reference for you in the future. 31/8/2015 · I also did the same example using _.each() for comparison, (update) and using Array.prototype.reduce and ES6 w/o Underscore. Example using Underscore's reduce ¶ var myArr = [ { rating : 5 }, { price : 200 }, { distance : 10 } ]; var myObj = _ . reduce ( myArr , function ( memo , item ) { return _ . extend ( memo , item ); }, {} ); console . log ( myObj );
JavaScript has created a few iterators that are based off the list objects or arrays. These iterators are helpful in providing efficient ways to do things you normally would do with a for-loop. All of my examples are written using es6 syntax, and so if you don't know about es6, you should check out this article. Map/Reduce. Map actually means to compute things with the original array without doing structural changes to the output. For example, when map receives an array, you can make sure the output will be another array, and the only difference is that the elements inside it may be transformed from the original value/type to another value/type. So we can say the doMap function from the above example ... let sum = arr.reduce((acc, val) => {return acc + val;}, 100); As you can see, the code above is almost identical to the previous example. All that's changed is we've added a second argument after our callback. I've passed in the number 100 as our starting point. Now, when we run the function, sum will equal 110. Reduce & ES6
23/7/2020 · Javascript answers related to “reduce array of objects es6 into number” array reduce and count based on proeperty js; async reduce javascript; creating array of objects usinng reduce js; es6 reduce return promise; how to update specific key of an object in reducer; javascript array patch object in array; javascript es6 filter sum distinct Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. by Josh Pitzalis. How JavaScript's Reduce method works, when to use it, and some of the cool things it can do Image credit to Karthik Srinivas.Thank you Karthik.. JavaScript's reduce method is one of the cornerstones of functional programming. Let's explore how it works, when you should use it, and some of the cool things it can do.
In this video, I cover the JavaScript array function reduce().🎥 Next Video: https://youtu.be/qmnH5MT_luk🔗 MDN's Array Documentation: https://developer.mozi... JavaScript fundamental (ES6 Syntax): Exercise-124 with Solution. Write a JavaScript program to reduce a given Array-like into a value hash (keyed data store). Note: Given an Iterable or Array-like structure, call Array.prototype.reduce.call () on the provided object to step over it and return an Object, keyed by the reference value. 5 days ago - Javascript array reduce() is an inbuilt method that is used to apply a function to each element in the array to reduce the array to a single value.
JavaScript ES6 Map and Reduce Patterns. ... An important thing to note is that the imperative example mixes the flattening and filtering operations in the same 'stage' of the computation. In contrast, the functional paradigm using map has more of a 'pipeline' approach, ... 29/6/2019 · ES6 comes with many new features. One of which are a few very handy `Array` functions. The most popular for doing daily tasks are `.filter()`, `.map`, `.reduce()`. In this post, I'll cover each one with examples of first the "old" way, then with these new functions. Dec 04, 2019 - The other day when i was writing an article for the Rest Operator one friend told me than one of my examples can be simplify by a function call reduce().
filter, map and reduce Example. map and reduce and filter operators will not support a Set object using javascript and typescript. But we can apply with some mechanism in javascript and typescript. First, you need to convert to an array using Es6 Spread operator, then we can apply these methods. Introduction To map(), reduce(), and filter() function in javascript. This tutorial explains how to use map(), reduce(), and filter() function in javascript programming language. Nowadays these function are widely used during react and react native application development.map returns an array with the same length, filter as the name implies, it returns an array with less items than the ... Map, reduce, and filter are all array methods in JavaScript. Each one will iterate over an array and perform a transformation or computation. Each will return a new array based on the result of the function. In this article, you will learn why and how to use each one. Here is a fun summary by Steven Luscher: Map/filter/reduce in a tweet:
JavaScript arrow functions arrived with the release of ECMAScript 2015, also known as ES6. Their concise syntax and handling of the this keyword have made JavaScript arrow functions an ES6 ... As you can see in the example, the reduce method uses the first two mandatory arguments, the total and the current value. It iterates on each number of the array like it would in a for-loop. When the loop starts the total value is the number on the far left (29) and the current value is the one next to it (46). Jan 16, 2019 -
Understand JavaScript Reduce With 5 Examples. The reduce () method on JavaScript arrays executes a "reducer" function on every element of the array in order, passing the return value from the previous reducer call to the next reducer call. The reduce () function is a common cause of confusion, but it can also make your code much more readable ... Jan 16, 2020 - In this article, I will try to introduce the most useful features of ES6 in a succinct way. Oct 03, 2017 - ES6 for beginners. The post covers let, const, arrow functions, for..of, map, sets and spread attributes.
Javascript ES6 Previous Next ... The example above creates a class named "Car". The class has two initial properties: "name" and "year". A JavaScript class is not an object. It is a template for JavaScript objects. Using a Class. When you have a class, you can use the class to create objects: If you're starting in JavaScript, maybe you haven't heard of .map(), .reduce(), and .filter().For me, it took a while as I had to support Internet Explorer 8 until a couple years ago. Sep 05, 2020 - The reduce array method in JavaScript can seem complicated to grasp at first glance. This post should finally help anchor exactly how reduce works.
This article explains how the reduce() method works with code samples. The reduce() method operates on the items of an array and returns a single value. Let's go straight to the examples to see how it works. Syntax reduce (callback) reduce (callback, initialValue) initialValue parameter is optional. If you don't specify an initial value, it will be the first item of the array. Javascript ES6 reduce() method and how to use it July 27, 2018 by Azadeh, 3 min. We will talk about Javascript ES6 reduce() method and how to use it in this article. Among new ES6 features, reduce() method is one of the good and sometimes confusing ones. First we need to understand its syntax. The ES6 reduce() syntax So you know what Array reduce means. Isn't that awesome? It only took you one minute. You can now head on to the Mozilla Docs for Array.prototype.reduce for a more in depth overview. Map Reduce. When you hear people talking about "Map Reduce" they are just talking about a "pattern": Mapping over a collection and then reducing it. Yeah. Really ...
The arr.reduce () method in JavaScript is used to reduce the array to a single value and executes a provided function for each value of the array (from left-to-right) and the return value of the function is stored in an accumulator. Syntax: array.reduce ( function (total, currentValue, currentIndex, arr), initialValue ) The reduce function is called on the array of functions provided in the call to pipe ( dbl and x => x + 42 in the example shown). The reduce function itself takes two parameters - a "reducer" function that is executed for each of the items in the array being reduced over (i.e. for each of the functions), and a starting value. Definition and Usage. The reduce() method executes a reducer function for each value of an array.. reduce() returns a single value which is the function's accumulated result. reduce() does not execute the function for empty array elements. reduce() does not change the original array.
Jul 19, 2019 - As the name speaks for itself it can be reduce to anything and can behave like map, find, filter or any other javascript function. The above code snippet works on an array and reduce to compute the total value of item of array. Explanation of example above : On reduce first run, acc is assigned ... Javascript has its problems, but syntax is not one of them. The ternary operator, the es6 map/reduce and the spread operator are powerful constructs. Apart from readability and precision, these constructs also help enforce loose immutability because the original data structure is never modified. If you are not familiar with the .forEach() or .reduce() array methods I suggest you check out Why and when to use forEach, map, filter, reduce, and find in JavaScript. Using .every() s similar to…
Dec 29, 2019 - Javascript is a language that gives the freedom of writing code in any style, from imperative to declarative. Most programmers use imperative because either they are coming from OOPs background… Apr 24, 2020 - JavaScript ES6 brings new syntax and new awesome features to make your code more modern and more readable. It allows you to write less code and do more. ES6 introduces us to many great features like arrow functions, template strings, class destruction, Modules… and more. Let’s take a look.
Javascript Count Duplicates In An Array Of Objects Example Code
How To Reduce Javascript Arrays With Array Reduce Codeburst
Powerful Js Reduce Function You Can Use It To Solve Any Data
A Simple Introduction To Javascript Method Reducer
Reduce Method In React Js Code Example
Main Difference Between Map And Reduce Stack Overflow
Functional Programming Try Reduce In Javascript And In Abap
Map Reducing The Pain Of Dealing With Arrays Rangle Io
Javascript Fundamental Es6 Syntax Reduce A Given Array
A Better Javascript With Map Filter Reduce By Al Amin
The Ultimate Guide To Javascript Array Methods Reduce
Javascript Array Reduce Amp Reduceright Reducing An Array Into
Javascript Filter Map Reduce Get Started With Functional
Functional Programming Try Reduce In Javascript And In Abap
Powerful Js Reduce Function You Can Use It To Solve Any Data
The Javascript Array Handbook Js Array Methods Explained
Javascript Array S Reduce Method
Most Useful Javascript Array Functions Vegibit
Es6 Amp Es7 Tips And Tricks Hacks In Javascript Tkssharma
Javascript Es6 Map Reduce Filter Find
A Definitive Guide To Map Filter And Reduce In
Javascript Reduce Superjavascript
How To Explain Javascript Filter Map And Reduce To
Javascript Array Reduce Method
Powerful Js Reduce Function You Can Use It To Solve Any Data
Javascript React Js Es6 Es5 Vue Js Js Array Reduce Method In
0 Response to "28 Javascript Reduce Example Es6"
Post a Comment