32 Javascript Get Name Of Function Inside Function
This post is meant as second part of Understanding "This" in javascript.. We will go through the same examples, but we will use arrow functions instead to compare the outputs. The motivation of this second post about the scope is, despite arrow functions are a powerful addition to ES6, they must not be misused or abused. 2/5/2007 · Is there any way to obtain the name of the function, inside the function which was called. Ex: function something() { alert( "The name of the function you invoke is " .....should says 'something' ); } Sort of.. function functionName(fn) {var name=/\W*function\s+([\w\$]+)\(/.exec(fn); if(!name)return 'No name'; return name[1];}
Mastering This In Javascript Callbacks And Bind Apply
Sep 10, 2015 - Function names are always assigned during creation and never changed later on. That is, JavaScript engines detect the previously mentioned patterns and create functions that start their lives with the correct names. The following code demonstrates that the name of the function created by ...
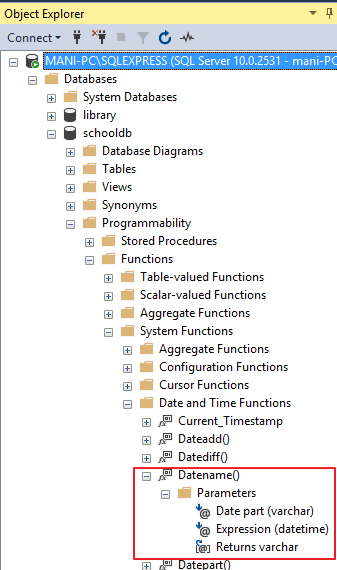
Javascript get name of function inside function. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Learn JavaScript - Named Functions. Named functions are hoisted. When using an anonymous function, the function can only be called after the line of declaration, whereas a named function can be called before declaration. Object methods, "this". Objects are usually created to represent entities of the real world, like users, orders and so on: let user = { name: "John", age: 30 }; And, in the real world, a user can act: select something from the shopping cart, login, logout etc. Actions are represented in JavaScript by functions in properties.
30/5/2019 · Given a function and the task is to get the name of the function from inside the function using JavaScript. JavaScript String substr() Method: This method gets parts of a string, starting at the character at the defined position, and returns the specified number of characters. Syntax: string.substr(start, length) Parameters: This is called a JavaScript closure. It makes it possible for a function to have " private " variables. The counter is protected by the scope of the anonymous function, and can only be changed using the add function. A closure is a function having access to the parent scope, even after the parent function has closed. It's rare, but occasionally it's helpful to discover a JavaScript function's name from inside of itself. Even with years of JavaScript experience, I find myself having to hunt this technique down, and then the examples are never precisely what I'm after, so they need to be tweaked...it's all ...
Jul 25, 2019 - function alertTheCallersName(){ var callingFunctionName=alertTheCallersName.caller.name; //get the callers name here alert(callingFunctionName); } function someOtherFunction(){ alertTheCallersName(); } someOtherFunction(); A function created with a function declaration is a Function object and has all the properties, methods and behavior of Function objects. See Function for detailed information on functions. A function can also be created using an expression (see function expression).. By default, functions return undefined.To return any other value, the function must have a return statement that specifies the ... 15/4/2019 · To find out the caller function name, we will use the Function object’s caller property. Here, the Function object is replaced by the name of the function of which we want to know the parent function name. When the above code is executed, we can see the name of the parent function …
8/4/2006 · Recommended for you. RE: get current function name. BillyRayPreachersSon (Programmer) 27 Mar 06 16:39. This works for me, although I suspect there are other ways. You should check on the compatibility of "arguments.callee" before using it for any crucial purpose, of course. Given a function and the task is to get the name of function that is currently running using JavaScript. Approach 1: Using arguments.callee method: It refers to the currently executing function inside the function body of that function. In this method, we use arguments.callee to refer to the name of the function. In JavaScript, a constructor function is used to create objects. For example, // constructor function function Person () { this.name = 'John', this.age = 23 } // create an object const person = new Person (); In the above example, function Person () is an object constructor function. To create an object from a constructor function, we use the ...
The JavaScript getElementByName () is a dom method to allows you to select an element by its name. The following syntax to represents the getElementsByName () method: 1. let elements = document.getElementsByName (name); The getElementsByName () accepts a name which is the value of the name attribute of elements and returns it value. In most implementations of JavaScript, once you have your constructor's reference in scope, you can get its string name from its name property (e.g. Function.name, or Object.constructor.name You could use Function.callee : 2. (good) create the 2 functions where the variables are outside the scope of both. This is a closure. (Basically the answer here.) Sadly, the functions are redefined for each new use of the variables. 3. (bad) don't use functions, just have one big long method doing both things. - robrich Jun 28 '18 at 16:37
Local Scope: Variables which are declared inside a function is called local variables and these are accessed only inside the function. Local variables are deleted when the function is completed. Global Scope: Global variables can be accessed from inside and outside the function. They are deleted when the browser window is closed but is ... Aug 07, 2018 - get a javascript function name. GitHub Gist: instantly share code, notes, and snippets. JavaScript provides functions similar to most of the scripting and programming languages. In JavaScript, a function allows you to define a block of code, give it a name and then execute it as many times as you want. A JavaScript function can be defined using function keyword.
The this Keyword. In a function definition, this refers to the "owner" of the function. In the example above, this is the person object that "owns" the fullName function. In other words, this.firstName means the firstName property of this object. Read more about the this keyword at JS this Keyword. Invoking a JavaScript Function The code inside a function is not executed when the function is defined. The code inside a function is executed when the function is invoked. It is common to use the term " call a function " instead of " invoke a function ". The first example uses a regular function, and the second example uses an arrow function. The result shows that the first example returns two different objects (window and button), and the second example returns the window object twice, because the window object is the "owner" of the function.
Jul 25, 2019 - function alertTheCallersName(){ var callingFunctionName=alertTheCallersName.caller.name; //get the callers name here alert(callingFunctionName); } function someOtherFunction(){ alertTheCallersName(); } someOtherFunction(); setTimeout(function() { console.log("This message is shown after 3 seconds"); }, 3000); As we can see, the callback function here has no name and a function definition without a name in JavaScript is called as an "anonymous function". This does exactly the same task as the example above. Callback as an Arrow Function Feb 26, 2020 - JavaScript exercises, practice and solution: Write a JavaScript function to get the function name.
The function keyword goes first, then goes the name of the function, then a list of parameters between the parentheses (comma-separated, empty in the example above, we'll see examples later) and finally the code of the function, also named "the function body", between curly braces. Functions within another function are called "Nested function". A function can have one or more inner functions. These nested functions (inner functions) are under the scope of outer functions. Javascript Nested Functions in this, the outer function can be called as Parent function and inner function can be called as Child function. 4/7/2010 · It includes code to get the name of functions, which works in most browsers. Obviously, there is no standard way that always works, so you should always provide a name that can be used if no other name is found. var nameFromToStringRegex = /^function\s? ([^\s (]*)/; /** * Gets the classname of an object or function if it can.
Does anyone know if there is a way to get JavaScript function name. For example I got a function like ... I have it in my head section. Then I create an object obj1 and put my function there ... When I call a method in obj1 object, do I have any way to get my function name (test1) inside of this ... They have a name (see The Name of a Function). However, JavaScript engines are getting better at inferring the names of anonymous function expressions. Object.prototype.constructor. The constructor property returns a reference to the Object constructor function that created the instance object. Note that the value of this property is a reference to the function itself, not a string containing the function's name. The value is only read-only for primitive values such as 1, true, and "test".
Nov 03, 2019 - Learn how to get the name of the surrounding function in a javascript context. That being said you can use arguments.callee.toString () inside the function (this is a string representation of the entire function) and regex out the value of the function name. Here is a function that will spit out its own name: function foo () { re = /^function\s+ ([^ (]+)/ alert (re.exec (arguments.callee.toString ())); } A function as a statement can be created as shown the following code example: function Add(num1,num2){ let sum = num1+ num2; return sum; } let res = Add(7,8); console.log(res); JavaScript. A function statement starts with the function keyword. It can return a primitive type value, object, or another function.
The returned value. The context this when the function is invoked. Named or an anonymous function. The variable that holds the function object. arguments object (or missing in an arrow function) This post teaches you six approaches to declare JavaScript functions: the syntax, examples and common pitfalls. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Function.name. A Function object's read-only name property indicates the function's name as specified when it was created, or it may be either anonymous or '' (an empty string) for functions created anonymously. Note: In non-standard, pre-ES2015 implementations the configurable attribute was false as well.
If the function f was invoked by the top level code, the value of f.caller is null, otherwise it's the function that called f.It's also null for strict, async function and generator function callers.. This property replaces the obsolete arguments.caller property of the arguments object.. The special property __caller__, which returned the activation object of the caller thus allowing to ... Variable scope, closure. JavaScript is a very function-oriented language. It gives us a lot of freedom. A function can be created at any moment, passed as an argument to another function, and then called from a totally different place of code later. We already know that a function can access variables outside of it ("outer" variables). All Functions are Methods. In JavaScript all functions are object methods. If a function is not a method of a JavaScript object, it is a function of the global object (see previous chapter). The example below creates an object with 3 properties, firstName, lastName, fullName.
Learn JavaScript - Get the name of a function object A function definition (also called a function declaration, or function statement) consists of the function keyword, followed by: The name of the function. A list of parameters to the function, enclosed in parentheses and separated by commas. The JavaScript statements that define the function, enclosed in curly brackets, {...}. The sumNumbers function is inside of the simpleObject object and is owned by it. Therefore, if we call sumNumbers function, it will return the value of this.The value will turn out to be object: sumNumbers function is owned by an object, which is simpleObject:
Write one function inside another function. Make a call to the inner function in the return statement of the outer function. Call it fun (a) (b) where a is parameter to outer and b is to the inner function. Finally return the combined output from the nested function.
A Simple Explanation Of Javascript Closures
How To Get The Function Name From Within That Function Using
Functions In Javascript Tektutorialshub
How To Access This Inside A Javascript Callback Function
How To Get Currently Running Function Name Using Javascript
Javascript Quiz Is The Calling Function Inside Function Are
Put A Function Method Inside An Object Javascript Code Example
Calling A Function Defined Inside Another Function In
How To Use Sql Server Built In Functions And Create User
Tutorial Mastering This In Javascript The New Stack
Define Function Inside Class Javascript Code Example
Python Using Variable Outside And Inside The Class And
Module Exports Javascript Function Inside Add Api Code Example
Javascript How To Access This Object Inside Event
Part 6 Javascript Functions Returning Functions From
Learn How To Utilize Closures In Javascript Dev Community
Javascript Execute Function By String Name Code Example
Why Function Inside Function Doesn T Need Parentheses
Javascript Map How To Use The Js Map Function Array Method
Tutorial Mastering This In Javascript The New Stack
A Simple Explanation Of Javascript Closures
What Went Wrong Troubleshooting Javascript Learn Web
Call A Javascript Function Within Dynamically Created Html
Javascript Functions Concept To Ease Your Web Development
How To Pass And Use Arguments To Code With Javascript Dummies
Javascript Variable Inside Function Is Not Accessible Why
Console Log Inside A Function Javascript Codecademy Forums
Part 6 Javascript Functions Returning Functions From
0 Response to "32 Javascript Get Name Of Function Inside Function"
Post a Comment