30 Javascript How To Add Event Listener
Copy and Paste the JavaScript code in your console to see how it looks like! Remove Event Listener. Another way to remove the event is the .removeEventListener function. Important: the .removeEventListener needs to have the same arguments of the .addEventListener that we are trying to remove. That includes the callback function, which needs to ... The addEventListener () method is used to attach an event handler to a particular element. It does not override the existing event handlers. Events are said to be an essential part of the JavaScript. A web page responds according to the event that occurred.
Event Binding Addeventlistener In Go
An event listener is a procedure in JavaScript that waits for an event to occur. The simple example of an event is a user clicking the mouse or pressing a key on the keyboard. The addEventListener () is an inbuilt function in JavaScript which takes the event to listen for, and a second argument to be called whenever the described event gets fired.
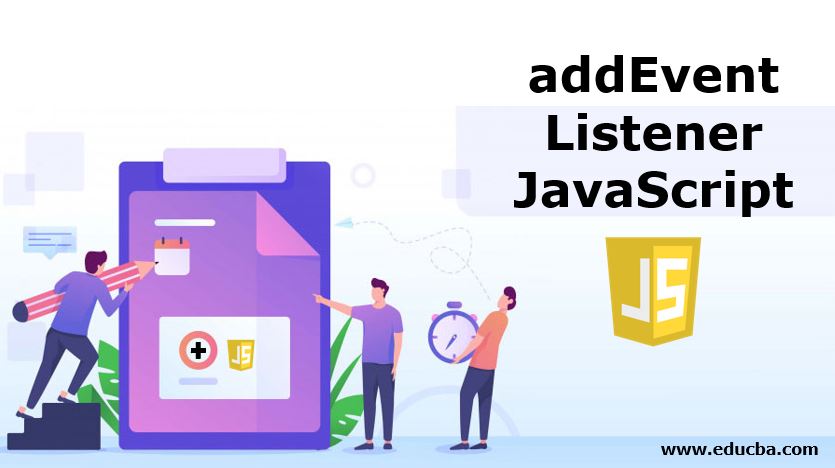
Javascript how to add event listener. As querySelectorAll () returns a NodeList instead of a single element, we need to loop through the nodes to add a click event listener to each button. For instance, if we have three buttons on the page, the code above will create three click event listeners. Note that you can only listen to one event with addEventListener (). The event listener can be specified as either a callback function or an object that implements EventListener, whose handleEvent()method serves as the callback function. The callback function itself has the same parameters and return value as the handleEvent()method; that is, the callback accepts a single parameter: an 8/7/2019 · In JavaScript you add an event listener to a single element using this syntax: document.querySelector('.my-element').addEventListener('click', event => { //handle click }) But how can you attach the same event to multiple elements? In other words, how to call addEventListener () on multiple elements at the same time?
window.onkeyup = function (event) { let key = event.key.toUpperCase (); if (key == 'W') { // 'W' key is pressed } else if (key == 'D') { // 'D' key is pressed } } Each key has it's own code, get it out by outputting value of "key" variable (eg for arrow up key it will be 'ARROWUP' - (casted to uppercase)) In the previous example we called addEventListener on the window object to register a handler for the whole window. Such a method can also be found on DOM elements and some other types of objects. Event listeners are called only when the event happens in the context of the object they are ... Feb 27, 2019 - Carefully review the code examples provided to grasp the concepts. ... When you add a JavaScript event listener, the function to be executed upon an event can be either anonymous or named. In the example below, you can see the anonymous function used. In this case, you need to define the code ...
Apr 19, 2020 - This tutorial shows you how to add an event handler to an event of an element in JavaScript. The Node.js event model relies on listeners to listen for events and emitters to emit events periodically — it doesn't sound that different, but the code is quite different, making use of functions like on() to register an event listener, and once() to register an event listener that unregisters after it has run once. 27/7/2020 · JavaScript provides an event handler in the form of the addEventListener() method. This handler can be attached to a specific HTML element you wish to monitor events for, and the element can have more than one handler attached. addEventListener() Syntax. Here's the syntax: target.addEventListener(event, function, useCapture) target: the HTML element you wish to add your
7/4/2020 · Adding event listener to multiple elements To add the event listener to the multiple elements, first we need to access the multiple elements with the same class name or id using document.querySelectorAll () method then we need to loop through each element using the forEach () method and add an event listener to it. 6/2/2018 · You can then use event.target to refer to the specific element which triggered the event: document.body.addEventListener("click", function (event) { if (event.target.classList.contains("delete")) { var title = event.target.getAttribute("title"); if (!confirm("sure u want to delete " + title)) { event.preventDefault(); } } }); How to add "enter" event listener aside from clicking the button. I am trying to apply what I learned from the Javascript and the DOM topic by building this simple to do list. My question is how do I add and event that when the user clicks "enter" key it will have the same function as clicking the button?
Add event listener for loop problem in JavaScript March 06, 2018. In web development class today, many of our students faced a problem adding event listeners to an array of HTML elements (ok, DOM nodes to be specific). Imagine you have a grid of 9 buttons forming a tic tac toe board in your HTML page. Sep 29, 2014 - Where does var _this = this; in the context of my code go? Do I need to add onClickBound(e) to the prototype? – Darthg8r Aug 27 '09 at 3:05 · In render, right before attaching event listener. You can pretty much just replace addEventListener line from original example with this snippet. In the above example when the user selects a file the event is handled by the addImage() function, which in this case simply gets the name of the file. How to Remove an Event Listener. To remove an event listener, use the removeEventListener() method. The first argument is the type of event to remove and the second is the name of the handler ...
Definition and Usage. The document.addEventListener() method attaches an event handler to the document. Tip: Use the document.removeEventListener() method to remove an event handler that has been attached with the addEventListener() method. Tip: Use the element.addEventListener() method to attach an event handler to a specified element. Add an Event Listener to Multiple Elements with JavaScript. by Louis Lazaris; May 21, 2020; code snippets; You might have a number of similar elements on a page and you want to add event listeners to all of them and in some cases you might want to add the same event listener to all the elements. React events do not work exactly the same as native events. See the SyntheticEvent reference guide to learn more. When using React, you generally don’t need to call addEventListener to add listeners to a DOM element after it is created. Instead, just provide a listener when the element is ...
May 14, 2019 - However, mixing the JavaScript code with the HTML directly is generally a poor practice for the same reason that you don't integrate style commands into HTML but rather reference the styles in a separate CSS file. A more common way to integrate the event listeners is by identifying the element and adding ... Dec 14, 2020 - The EventListener interface represents an object that can handle an event dispatched by an EventTarget object. Can I add an eventListener in JavaScript to a checkbox or do I have to use the attribute in html onclick = someFunc() to trigger a function. I set a up a fiddle where I tried to add an eventListener, but that does not give me the expected output.
In this video tutorial, you will learn how to add and remove event listener in javascript. We are going to use mouseup event for demonstration. We will simply add and remove this event on button click. Adding an event handler to the window object The addEventListener () method allows you to add event listeners to any DOM object like HTML elements, the HTML document, and the window object. For example, here is an event listener that fires when the user scrolls the document: But what about plain ol' JavaScript event listeners? document.addEventListener(); Would it be easier, and more beneficial to use that instead of React's onClick inline? Maybe. Let's start with the basics. What is a JS Event Listener. According to Mozilla JS docs, an event listener is a function that gets called when a specific event occurs.
For the window object, the load event is fired when the whole webpage (HTML) has loaded fully, including all dependent resources such as JavaScript files, CSS files, and images. To handle the load event, you register an event listener using the addEventListener () method: To log in and use all the features of Khan Academy, please enable JavaScript in your browser. ... This is the currently selected item. ... Our mission is to provide a free, world-class education to anyone, anywhere. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Nov 11, 2014 - Due to using inline event listeners or assigning event listeners to .onevent properties of DOM elements, lots of inexperienced JavaScript programmers thinks that the event name is for example onclick or onload. on is not a part of event name. Correct event names are click and load, and that's how event names are passed to .addEventListener... To add an event listener to an element, we use the addEventListener () method on the element we want to target an event for. The method takes up to 3 parameters: The event we want to listen for, like click or keypress . The function we want to invoke when the event fires. This may be a named, anonymous or arrow function. How to Add Event Listeners to Dynamic Content with Event Delegation. Have you ever added a button to a page with JavaScript expecting it to function fully, but when you clicked it nothing happened? You've double checked your code - you're creating the event listeners, the selectors are correct, but it's still not working!
Adding event handlers. We added an event listener of click, and called a showMore function. In the showMore function, we selected the class moreInfo. If you check on our css you find out that the text was set to display none. So now we are saying that onclick of the button, let the display none change to display block. We have created a dog ... You use it like this: let onMyEvent = simpleEvent();let listener = (test) => { console.log("triggered", test); };onMyEvent.addListener(listener);onMyEvent.trigger("hello");onMyEvent.removeListener(listener); Or in classes like this. class Example { public onMyEvent = simpleEvent(this);} When using the addEventListener () method, the JavaScript is separated from the HTML markup, for better readability and allows you to add event listeners even when you do not control the HTML markup. You can easily remove an event listener by using the removeEventListener () method.
Nov 27, 2019 - The addEventListener() is an inbuilt JavaScript function which takes the event to listen for, and a second argument to be called whenever the described event gets fired. Any number of event handlers can be added to a single element without overwriting existing event handlers. In this tutorial you will learn about DOM event listeners in JavaScript. ... The event listeners are just like event handlers, except that you can assign as many event listeners as you like to a particular event on particular element. In this video tutorial, you will learn how to add and remove event listener in javascript. We are going to use mouseup event for demonstration. We will si...
Feb 20, 2021 - This article demonstrates how to create and dispatch DOM events. Such events are commonly called synthetic events, as opposed to the events fired by the browser itself. The better ways to handle the scroll events. Many scroll events fire while you are scrolling a page or an element. If you attach an event listener to the scroll event, the code in the event handler needs to take time to execute.. This will cause an issue which is known as the scroll jank. The scroll jank effect causes a delay that the page doesn't feel anchored to your finger. The Function.prototype.bind () method lets you specify the value that should be used as this for all calls to a given function. Using bind () has an additional benefit in that you can add multiple event listeners for the same event name.
event.ctrlKey: the Ctrl key; event.altKey: the Alt key; event.metaKey: the "meta" key differs based on the OS.For example, on Mac OSX it's the ⌘ Command key; event.shiftkey: the Shift key; Until recently the conventional way to listen to the keyboard was via event.keyCode instead of event.key.Many forums/blogs like StackOverflow still heavily feature keyCode however beware...
Add An Event Listener With Addeventlistener
Js How To Use Anonymous Function Bind In Addeventlistener
Javascript Events Explore Different Concepts And Ways Of
Javascript Fundamental Es6 Syntax Add An Event Listener To
08 31 Javascript Event Basics Binding Events Unbinding Event
Javascript Events Add Event Handlers Programmer Sought
How To Make A Website Interactive With Javascript Events
How To Find Event Listeners On A Dom Node In Javascript Or In
Vanilla Js Addeventlistener Queryselector And Closest By
Addeventlistener Javascript 4 Examples Of Addeventlistener
Dom Events In Javascript Here Is How S Your Dom Structured
Event Listener Click And Mouseover Stack Overflow
How To Handle Dom And Window Events With React Digitalocean
Add Event Listener For Loop Problem In Javascript Nick Ang
4 Multiple Event Handlers Two S Company Head First Ajax
The Javascript Copy Event Fun With Invision Freehand Shapes
4 Multiple Event Handlers Two S Company Head First Ajax
Adding Or Attaching Eventlistener Hits Javascript Tutorial
Inline Onclick Vs Addeventlistener
Javascript Events Explore Different Concepts And Ways Of
How To Handle Event Handling In Javascript Examples And All
Javascript How To List All Active Event Listeners On A Web Page
Javascript Attachevents Programmatically Using Addeventlistener Or Attachevent
Finding That Pesky Listener That S Hijacking Your Event
Gtmtips Simple Custom Event Listeners With Google Tag
Javascript Tutorial Events In Javascript And
How To Call A Function Which Is Called Method
Javascript Addeventlistener Javatpoint
Understanding Events In Javascript By Gemma Stiles Medium
0 Response to "30 Javascript How To Add Event Listener"
Post a Comment