27 How To Order An Array In Javascript
Introduction to JavaScript- This tutorial introduces to you the very basics of JavaScript JavaScript Array sort(): Sort in Reverse Order. There is also a built-in JavaScript function that allows you to sort an array in reverse order: reverse(). The reverse() method reverses an array so that the first element becomes the last, and the last element becomes the first. Thus, this array does not order an array in alphabetical descending ...
The sort () method sorts the elements of an array in place and returns the sorted array. The default sort order is ascending, built upon converting the elements into strings, then comparing their sequences of UTF-16 code units values. The time and space complexity of the sort cannot be guaranteed as it depends on the implementation.
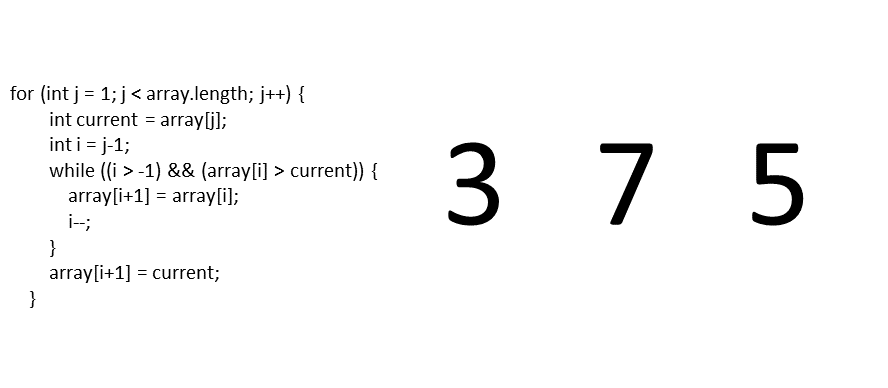
How to order an array in javascript. Jul 25, 2020 - Then the order of a and b remains unchanged. Return value: This method returns the reference of the sorted original array. Below examples illustrate the JavaScript Array sort() method: Shuffling logic: pick up a random index, then add the corresponding element to the result array and delete it from the source array copy. Repeat this action until the source array gets empty. And if you really want it short, here's how far I could get: When we return a positive value, the function communicates to sort() that the object b takes precedence in sorting over the object a.Returning a negative value will do the opposite. The sort() method returns a new sorted array, but it also sorts the original array in place. Thus, both the sortedActivities and activities arrays are now sorted. One option to protect the original array from being ...
How it works: First, declare an array rivers that consists of the famous river names.; Second, sort the rivers array by the length of its element using the sort() method. We output the elements of the rivers array to the web console whenever the sort() method invokes the comparison function .; As shown in the output above, each element has been evaluated multiple times e.g., Amazon 4 times ... const selfName = 'Arnav'; We are required to write a function that sorts the scorecard array in alphabetical order and makes sure the object with name same as selfName appears at top (at index 0). Therefore, let's write the code for this problem −. We can sort a multidimensional array ascending and descending order using JavaScript and modern JavaScript.In both ways we use.sort method.
May 19, 2017 - I had to do this for a JSON payload I receive from an API, but it wasn't in the order I wanted it. Array to be the reference array, the one you want the second array sorted by: Aug 17, 2016 - Sorting arrays in JavaScript is done via the method array.sort(), a method that's probably as much misunderstood as it is underestimated. While calling sort() by itself simply sorts the array in lexicographical (aka alphabetical) order, the sky's really the limit once you go beyond the surface. ... Sorting an ... Specifies a function that defines the sort order. If omitted, the array is sorted according to each character's Unicode code point value, according to the string conversion of each element. ... Javascript arrays // reordering an array starting at a specified index while keeping the original order. 3.
Sep 05, 2020 - To do this, the sort method calls the String() casting method on every array element and then compares the equivalent strings to determine the correct order. If you want your images to be drawn in a specific order - e.g. the order it's listed in the array - you need to request a new one after the previous finished loading. Here's an example: Arrays in JavaScript enables multiple values to be stored in a single variable. It stores the same kind of element collection sequential fixed-size. Arrays in JavaScript are used to store an information set, but it is often more helpful for storing a set of variables of the same type.
May 01, 2014 - How do I create a function to sort the objects by the price property in ascending or descending order using JavaScript only? ... the quickest way is to use the isomorphic sort-array module which works natively in both browser and node, supporting any type of input, computed fields and custom ... The reverse () method. If you want to sort your array in descending order, you can use the reverse method. myAlhabeticArray.reverse (); // output is ['d', 'c', 'b', 'a'] In JavaScript Arrays are mutable and also, the sort () and reverse () methods are implemented in a mutable way. That's is why by running these methods, the array content will ... Order Array Of Objects By Property Value In JavaScript May 22, 2020 by Andreas Wik Imagine that you have an array of objects and want to order them depending on the value of a specific property in the objects. With sort () we can do exactly this.
May 20, 2020 - Connect and share knowledge within a single location that is structured and easy to search. ... Assuming a JavaScript array is posted in a form (using ajax/JQuery) is the order in the array guaranteed? Javascript Slice: Selecting Part of an Array You can obtain an array consisting of part of another array in javascript using slice(START, END). START is the index of the first item to include in your selection. END is the index of item afterthe last one you want to include. An alternative to for and for/in loops isArray.prototype.forEach(). The forEach() runs a function on each indexed element in an array. Starting at index[0] a function will get called on index[0], index[1], index[2], etc… forEach() will let you loop through an array nearly the same way as a for loop:
The JavaScript Array.sort () method is used to sort the array elements in-place and returns the sorted array. This function sorts the elements in string format. It will work good for string array but not for numbers. For example: if numbers are sorted as string, than "75" is bigger than "200". Example: The sort() method is used to sort the elements of an array in order and returns the new array. Sorting is nothing but putting all the elements of a list in a certain order. The different type of sort orders are alphabetic, numeric, ascending and descending. The default sort order of the sort() method is alphabetic order for the words and acending order for arrange an array in ascending order javascript; sort array in ascending order javascript without function; javascript sorting from small to largest; arrange javascript code in ascending order; sort by ascending number js; sort table values in ascending order js; how to convert an array in ascending order javascript; sort from lowest to highest js
The compare function compares all the values in the array, two values at a time (a, b). When comparing 40 and 100, the sort () method calls the compare function (40, 100). The function calculates 40 - 100 (a - b), and since the result is negative (-60), the sort function will sort 40 as a value lower than 100. May 22, 2017 - Stack Overflow | The World’s Largest Online Community for Developers Jul 08, 2019 - Arrays in JavaScript come with a built-in function that is used to sort elements in alphabetical order. However, this function does not directly work on arrays of numbers or objects. Instead a custom function, that can be passed to the built-in sort() method to sort objects based on the ...
Apr 28, 2021 - In JavaScript, we can sort the elements of an array easily with a built-in method called the sort( ) function. However, data types (string, number, and so on) can differ from one array to another. This means that using the sort( ) method alone is not always an appropriate solution. In this Nov 26, 2019 - Build your JavaScript skills on a rock-solid foundation. ... If you need to sort an array of objects into a certain order, you might be tempted to reach for a JavaScript library. But before you do, remember that you can do some pretty neat sorting with the native Array.sort function. JavaScript arrays have the sort () method, which sorts the array elements into alphabetical order. The sort () method accepts a function that compares two items of the Array.sort ([comparer]). Watch a video course JavaScript - The Complete Guide (Beginner + Advanced)
sort() will loop through each element of the array, passing 2 of them into the custom compare function on every round. b is the current element, and a is the next element. The whole idea is to compare a and b, then return a number to specify the sort order. Using an ascending sort order as an example: Use.sort ((a,b) => a-b) In order to sort a list in numerical order, the Array.prototype.sort () method needs to be passed a comparison function. To sort the array numerically in ascending order,... Feb 01, 2016 - Using a new ES6 feature called Map. A Map object iterates its elements in insertion order — a for...of loop returns an array of [key, value] for each iteration.
Sort an Array of Objects in JavaScript Summary : in this tutorial, you will learn how to sort an array of objects by the values of the object's properties. To sort an array of objects, you use the sort() method and provide a comparison function that determines the order of objects. The sort () method sorts the elements of an array. The sort order can be either alphabetic or numeric, and either ascending (up) or descending (down). By default, the sort () method sorts the values as strings in alphabetical and ascending order. This works well for strings ("Apple" comes before "Banana"). The reverse () method reverses the order of the elements in an array. Note: this method will change the original array.
Sep 08, 2017 - The JavaScript array sort() is used to sort array items. The order in which items are sorted may be either alphabetic or ascending and descending or ascending. Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: JavaScript has sort function which helps in sorting array and object. 1. Sorting JavaScript array in descending order: A. Sort numbers in an array in descending order: In the below example, we use sort function callback to arrange elements of an array by descending order. var nums = [10,5,6,20,87]; nums.sort(function(a, b){return b-a});
Here are the different JavaScript functions you can use to add elements to an array: # 1 push - Add an element to the end of the array. #2 unshift - Insert an element at the beginning of the array. #3 spread operator - Adding elements to an array using the new ES6 spread operator. #4 concat - This can be used to append an array to ... Arrays in JavaScript can work both as a queue and as a stack. They allow you to add/remove elements both to/from the beginning or the end. In computer science the data structure that allows this, is called deque. ... Fill the array in the reverse order, like arr[1000], arr[999] and so on.
What Are Higher Order Array Methods In Js Weekly Webtips
How To Sort An Array Of Objects By A Property Value In Javascript
Javascript Sort Array Sorting Arrays Of Strings In
Javascript Array Push Adding Elements In Array With
Algodaily Remove Duplicates From Array Description
How To Sort Arrays In Javascript
How To Sort Array In Alphabetical Order Javascript Code Example
Solved Multiple Choice 1 The Javascript Array Object Su
Looping Javascript Arrays Using For Foreach Amp More
The Javascript Array Handbook Js Array Methods Explained
How To Group An Array Of Associative Arrays By Key In Php
Deep Dive Into Javascript Array Sort Method
Making Use Of Built In Higher Order Array Functions In Javascript
How To Reverse An Array In Javascript Samanthaming Com
Javascript Fundamental Es6 Syntax Return 1 If The Array Is
How To Sort An Array Of Objects By A Property Value In Javascript
Javascript Array A Complete Guide For Beginners Dataflair
What Is The Best Way To Publish A List Of Objects To
Sort Array In Descending Order Javascript 3 Ways Code
How To Sort An Array Of Numbers In Javascript How To Sort An Array Of Strings In Javascript
Hacks For Creating Javascript Arrays
Sorting Objects In Javascript E C How To Get Sorted Values
Java67 How To Sort An Array In Descending Order In Java
How To Remove Array Duplicates In Es6 By Samantha Ming
Array Prototype Sort Javascript Mdn
0 Response to "27 How To Order An Array In Javascript"
Post a Comment