33 Javascript Immediately Invoked Function
What is IIFE and how to use it (with code example) ? Javascript iife pattern .Javascript iife namespaceWHat is Function Expressionself-executing anonymous ... You can create an Immediately Invoked Function Expression (IIFE for short). These are essentially self-executing anonymous functions. They have access to the surrounding scope, but the function itself and any internal variables will be inaccessible from outside.
Javascript Tutorial Immediately Invoked Function Expression
An immediately invoked function expression (or IIFE, pronounced "iffy", IPA /ˈɪf.i/) is a programming language idiom which produces a lexical scope using function scoping. It was popular in JavaScript as a method to support modular programming before the introduction of more standardized ...
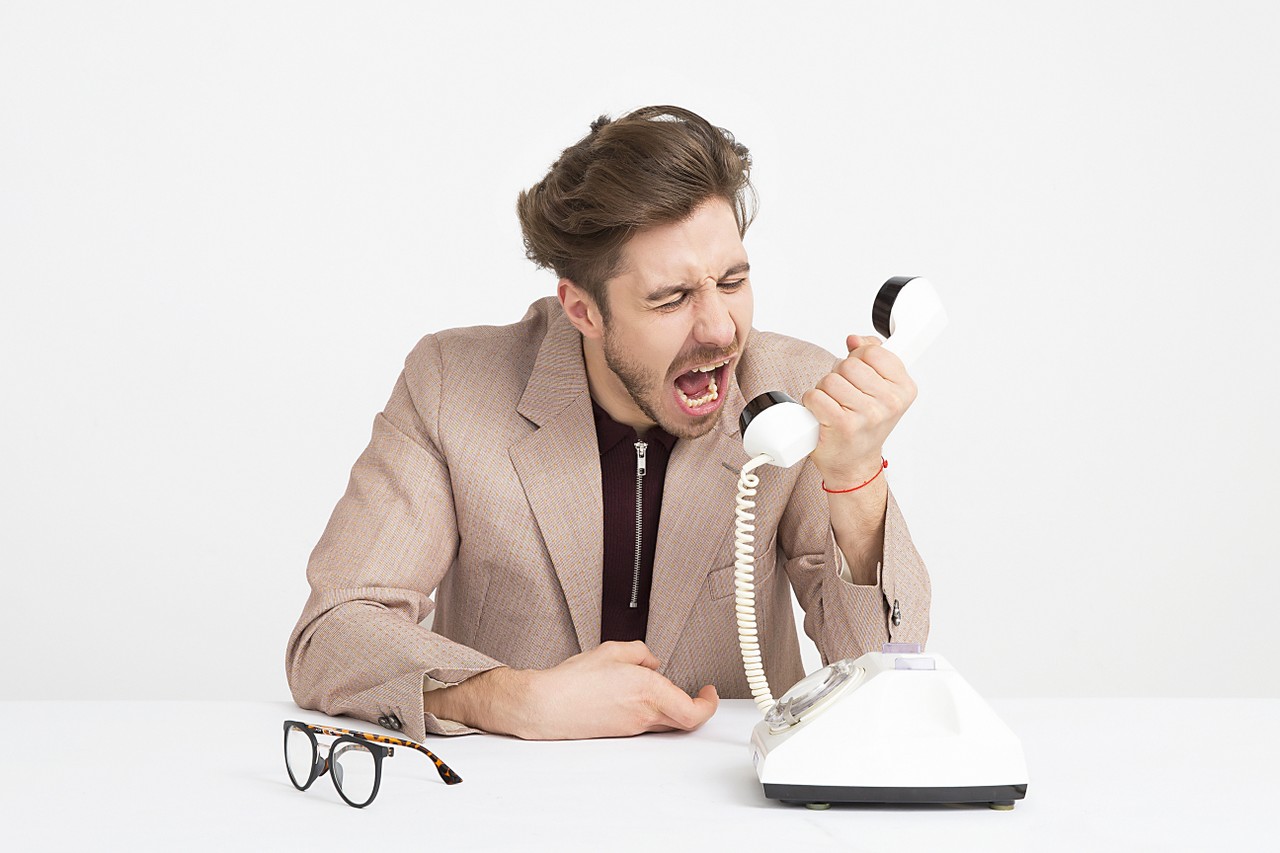
Javascript immediately invoked function. then the above if would fail, comparing car to 🤔.. This has since been fixed in ES5, but was possible before that. If car was actually undefined, there was no way to find out now.. Except using this technique: we wrap all our code in an IIFE (Immediately-invoked Function Expression) and we pass one parameter to the function definition, without adding it in the invocation phase. Now JavaScript provides a variety of methods to define and execute Functions, there are named functions, anonymous functions and then there are Functions that are executed as soon as they are mounted, these functions are known as Immediately Invoked Function Expressions or IIFEs. Let us dive deeper to know more about IIFEs. Immediately-Invoked Function Expressions (IIFE), pronounced "iffy", are a common JavaScript pattern that executes a function instantly after it's defined. Developers primarily use this pattern to ensure variables are only accessible within the scope of the defined function. In this article, you will first learn about function expressions.
Immediately-invoked Function Expression (IIFE) This is a technique that was used a lot in the ES5 days to implement the "module" design pattern (before this was natively supported). The idea is to "wrap" your module in a function that is immediately executed. An IIFE (Immediately Invoked Function Expression) is a JavaScript function that runs as soon as it is defined. The name IIFE is promoted by Ben Alman in his blog. (function () { statements })(); It is a design pattern which is also known as a Self-Executing Anonymous Function and contains two major parts: Sep 10, 2020 - Understanding functions inside out and then learning how to exploit them to write modern, clean JavaScript code is a critical skill for becoming a JavaScript ninja. One of the often used coding…
The code inside a function is executed when the function is invoked. It is common to use the term "call a function" instead of "invoke a function". It is also common to say "call upon a function", "start a function", or "execute a function". In this tutorial, we will use invoke, because a JavaScript function can be invoked without being called. ES6 Immediately Invoked Function Expression with What is ES6, History of ES6, ES6 Versions, ES6 Loops, Environment Setup, ES6 Syntax, ES6 Operators, ES6 Variables, ES6 Functions, ES6 Cookies, ES6 Strings, ES6 Math etc. Immediately Invoked Function Expressions (IIFE) in JavaScript. May 27, 2020. An immediately invoked function expression (IIFE for short) is a JavaScript design pattern that declares an anonymous function and immediately executes it. // Prints "Hello, World!" (function() { console.log ('Hello, World!');
The immediate function, WHICH offers a heightened level of functionality, is an often overlooked feature of JavaScript. An immediate function is one that executes as soon as it is defined. Creating an immediate function is simple: you add the open/close parentheses after the closing curly bracket, and then wrap the entire function in parentheses. JavaScript Immediately-invoked Function Expressions (IIFE) An Immediately-invoked Function Expression is a way to execute functions immediately, as soon as they are created. IIFEs are very useful because they don't pollute the global object, and they are a simple way to isolate variables declarations Today, I will explain you how to use javascript immediately invoked function.An IIFE can be used for avoiding the variable hoisting from within the blocks and they don't pollute the global object. The following syntax represents how to define an immediately invoked function expression:
JavaScript Immediately-invoked Function Expressions (IIFE) An Immediately-invoked Function Expression that runs as soon as it defined. An IIFE can be used for avoiding the variable hoisting from within the blocks and they don't pollute the global object. The following syntax represents how to define an immediately invoked function expression: 1. In JavaScript, an Immediately Invoked Function Expression (IIFE) is a JavaScript function expression that executes as soon as it defined. (function iifeFunction () { console.log ('Hello World'); }) (); This function will print to the console 'Hello World' right after we define it. Writing IIFE is similar to write a regular function, but ... The Immediately-Invoked Function Expression (IIFE) in JavaScript is a way to execute functions immediately as soon as they are created. In other words, IIFE is a function expression that immediately invokes after the function definition is complete automatically. The parenthesis () plays an important role in the IIFE pattern.
Apr 28, 2021 - In above example when javascript engine execute above code it will create global execution context when it sees code and create function object in memory for IIFE. And when it reaches on line 46 due to which function is Invoked a new execution context is created on the fly and so greet variable ... In JavaScript, every function, when invoked, creates a new execution context. Because variables and functions defined within a function may only be accessed inside, but not outside, that context, invoking a function provides a very easy way to create privacy. Immediately-invoked Function Expressions (IIFE) IIFE or Immediately-invoked Function Expressions are functions that we execute immediately after we define them. IIFE was used to isolate the variables and stop polluting the global object. But with the introduction of block scope, modules, let & const statements IIFE is rarely needed.
JavaScript IIFE stands for an immediately invoked function expression. It defined as a function expression and executed immediately after creation. It is a design pattern which is also known as a Self-Executing Anonymous Function The pattern is called an immediately invoked function expression, or IIFE (pronounced "iffy"). In JavaScript functions can be created either through a function declaration or a function expression. A function declaration is the "normal" way of creating a named function. // Named function declaration function myFunction () { /* logic here */ } Feb 02, 2015 - This is called IIFE (Immediately Invoked Function Expression). One of the famous JavaScript design patterns, it is the heart and soul of the modern day Module pattern. As the name suggests it executes immediately after it is created. This pattern creates an isolated or private scope of execution.
You need to invoke it manually. The most widely accepted way to tell the parser to expect a function expression is just to wrap it in parens, because in JavaScript, parens can't contain statements. At this point, when the parser encounters the function keyword, it knows to parse it as a function expression and not a function declaration. Jul 11, 2017 - Maybe you’ve seen the syntax before, but why in the heck does an Immediately-Invoked Function Expression (IIFE — pronounced ‘iffy’) look like it does? Why is it useful? Before we can learn why an… Immediately Invoked. To make it immediately invoked, we append () to the function expression. (function () { statements }) (); Enter fullscreen mode. Exit fullscreen mode. We basically have a function defined inside parentheses, and then we append () to execute that function. -The first is the anonymous function with lexical scope enclosed ...
Mar 24, 2021 - Learn what an Immediately Invoked Function Expression (IIFE) is in five minutes or less! Immediately Invoked Function Expression - IIFE. Immediately Invoked Function Expression (IIFE) is one of the most popular design patterns in JavaScript. It pronounces like iify. IIFE has been used since long by JavaScript community but it had misleading term "self-executing anonymous function". Ben Alman gave it appropriate name "Immediately Invoked Function Expression" An immediately invoked function expression (or IIFE, pronounced "iffy", IPA /ˈɪf.i/) is a JavaScript programming language idiom which produces a lexical scope using JavaScript's function scoping.
Self invoking function. The function body will be invoked immediately. You can still refer to the function x inside the function body. So when you want to execute something immediately, and then you may want to iterate it, you can just reference to it directly. (function x() { console.log(this); // window console.log(x); // function x() {} })(); A JavaScript immediately invoked function expression is a function defined as an expression and executed immediately after creation. The following shows the syntax of defining an immediately invoked function expression: (function() { //... An immediately-invoked function expression (or IIFE, pronounced 'iffy' for short) is a Javascript pattern to create a private scope within the current scope. This new scope may or may not be persistent (a closure). The technique uses an anonymous function inside parentheses to convert it from a declaration to an expression, which is executed ...
Aug 29, 2012 - That is the reason Ben Alman gave self-invoking functions a new name: Immediately Invoked Function Expression (IIFE). It is recommended to use the term IIFE since it’s semantically correct and more clear. ... Please enable JavaScript to view the comments powered by Disqus. Scope of variable in immediately invoke function In general rule, the variable defined within JavaScript functions has function scope. In this example, we have defined two functions and the "name" variable is defined in the first function. We are then trying to access it from the second function.
Javascript Iife Immediately Invoked Function Expression
Javascript Syntax For Immediately Invoked Function Expression
Types Of Functions In Javascript By Abhiburman Medium
Javascript Immediately Invoked Function Expression Iife
Iife In Javascript Immediately Invoked Function Expressions
Javascript Wisdom On Twitter Iife 39 S Immediately Invoked
How To Using Es6 Arrow Function To Realize Immediately
Javascript What The Heck Is An Immediately Invoked Function
Iife S Will Change The Way You Use React Hooks By Brian
Advanced Javascript Immediately Invoke Function In Javascript
Javascript Advance Iife Immediate Invoke Function Expression Tamil Njan Tutorial 3
Understanding Immediately Invoked Function Expressions Part
Javascript Iife Immediately Invoked Function Expression
Iife In Javascript What Are Immediately Invoked Function
Javascript Immediately Invoked Function Expression Example
Proful Sadangi On Twitter Javascript Iife Immediately
3 Iife Immediately Invoked Function Expression Javascript Avancado
Immediately Invoked Function Expression Iife By Kevin Lai
Js When To Use An Iife I Wrote An Article About Iife Js
Es6 Immediately Invoked Function Expression
Beginner Javascript 10 Immediate Invoked Function
Hey Guy S Let S Start With A Starter Javascript Startup
Iife In Javascript Immediately Invoked Function Expressions
Javascript What The Heck Is An Immediately Invoked Function
Iife Immediately Invoked Function Expression In Javascript
How To Prevent Overriding Using Immediately Invoked Function
Javascript Iife How Iife Work In Javascript With
Understanding Javascript Iifes
Javascript Immediately Invoked Function Expression Iife Tutorial
Javascript Iife Immediately Invoked Function Expressions
Iife Imediately Invoked Function Expression
0 Response to "33 Javascript Immediately Invoked Function"
Post a Comment