22 How To Catch Error In Javascript
PHP - how to catch errors using set_error_handler Python selenium - print browser log (including javascript errors) with phantomjs Using JSLint on command line on Ubuntu linux - quick start guide It's not enough to throw exceptions in JavaScript. You need to throw exceptions effectively. Here's how to create a human-readable—and diagnosable—exception object.
Javascript Try Catch How Does Try Catch Work In Javascript
When a problem arises and is then handled, an "exception is thrown" by the Javascript interpreter. Javascript generates an object containing the details about it, which is what the (error) is...
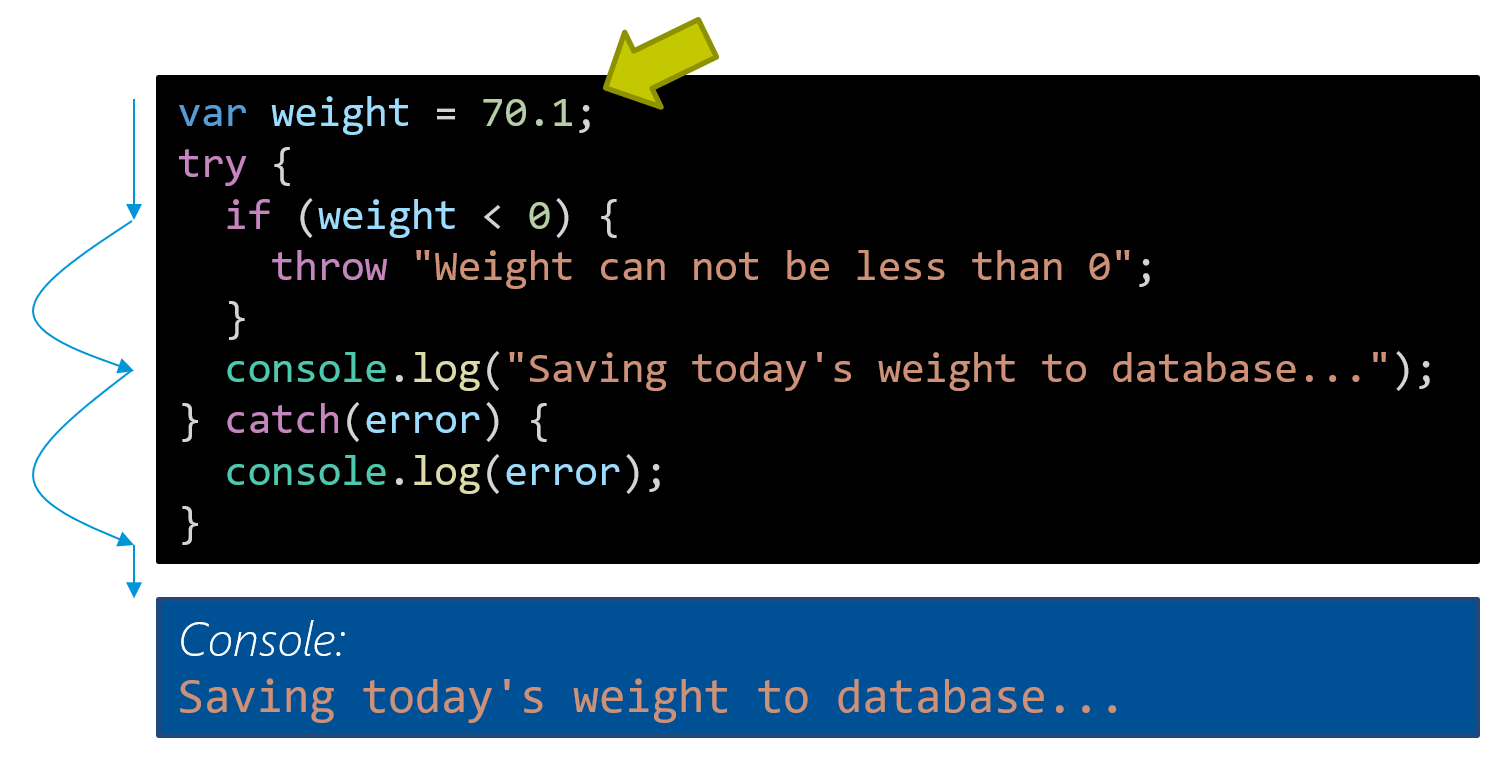
How to catch error in javascript. 1 week ago - You can throw exceptions using the throw statement and handle them using the try...catch statements. ... Just about any object can be thrown in JavaScript. Nevertheless, not all thrown objects are created equal. While it is common to throw numbers or strings as errors, it is frequently more ... 3 weeks ago - If you don't have control over the original errors that are thrown, one option is to catch them and throw new Error objects that have more specific messages. The original error should be passed to the new Error in the constructor option parameter (cause property), as this ensures that the original ... The eval () function is used to evaluate expressions in JavaScript. RangeError - This error occurs when a value is tried to pass when it is outside the range. ReferenceError - This error is thrown when a variable that is not declared is referenced. SyntaxError - This error occurs when the syntax of the code is wrong.
23/1/2020 · JavaScript Error handling, "try..catch" Even the greatest programmers can have errors in the scripts. Generally, errors can occur as a result of mistakes, unexpected user input, and a thousand other reasons. But, hopefully, there exists a try..catch syntax construct, allowing to catch errors, so the script can do something more reasonable. try…catch only handles errors encountered at runtime, and invalid JavaScript will error-out at parse-time so your program won't run at all. Instead, use a linter like ESLint to catch those errors directly when authoring your code. At the end we see that even though null and undefined are considered equal, they are not the same identity (equal without type conversion).As discussed, this is because they are of different types behind the scenes: null being an object and undefined being an undefined type. With that out of the way we can start to understand why trying to access a property of null or undefined may fail.
5/11/2015 · If you reject the promise, then you can catch the error, if you resolve the promise, that becomes the return value of the function. async function helloWorld { return new Promise(function(resolve, reject){ reject('error') }); } try { await helloWorld(); } catch (e) { console.log('Error occurred', e); } How to Throw Errors From Async Functions in JavaScript: catch me if you can Async functions and async methods do not throw errors in the strict sense. Async functions and async methods always return a Promise, either resolved or rejected. You must attach then () and catch (), no matter what. Aug 18, 2020 - Error handling in the asynchronous world is distinct from its synchronous counterpart. Let's see some examples. ... In the beginning of your explorations with JavaScript, after learning about try/catch/finally, you might be tempted to put it around any block of code.
In this article, I'll describe 3 different patterns for handling errors in run(): try/catch, Golang-style, and catch() on the function call. I'll also explain why you rarely need anything but catch() with async functions. The catch method is used for error handling in promise composition. Since it returns a Promise, it can be chained in the same way as its sister method, then (). 30/12/2020 · A try / catch block is basically used to handle errors in JavaScript. You use this when you don't want an error in your script to break your code. While this might look like something you can easily do with an if statement , try/catch gives you a lot of benefits beyond what an if/else statement can do, some of which you will see below.
Jan 25, 2021 - In the example above we use try...catch to handle incorrect data. But is it possible that another unexpected error occurs within the try {...} block? Like a programming error (variable is not defined) or something else, not just this “incorrect data” thing. try { //lines of code in javascript which might cause an error/exception} catch (e){ //lines of code need to be executed when there is an exception} Let's understand these details using the following example, where we are wrapping the possible errors in a try-catch block: The latest versions of JavaScript added exception handling capabilities. JavaScript implements the try...catch...finally construct as well as the throw operator to handle exceptions. You can catch programmer-generated and runtime exceptions, but you cannot catch JavaScript syntax errors. Here is the try...catch...finally block syntax −
So you have two ways to throw errors, and two ways to catch errors. This is more complex than we'd like, but at least each way of catching errors will catch both ways of throwing them, so the complexity here isn't fully as bad as it could have been. Errors thrown in a different call stack. There's more troubly to be had though. The variable named in parentheses after the word catch is the name given to the exception value inside this block. ¶ Note that the function lastElementPlusTen completely ignores the possibility that lastElement might go wrong. This is the big advantage of exceptions ― error-handling code ... JavaScript catches adddlert as an error, and executes the catch code to handle it. JavaScript try and catch The try statement allows you to define a block of code to be tested for errors while it is being executed. The catch statement allows you to define a block of code to be executed, if an error occurs in the try block.
Try and Catch In JavaScript, the try statement allows you to add a block of code that will be tested for errors when it is executed. If an error does occur in the try block, the corresponding catch statement will be executed. You can think of the try and catch statements as a pair. How to catch all JavaScript errors and send them to server? 08, Jun 20. Catch and Throw Exception In Ruby. 10, Oct 19. Difference between try-catch and if-else statements in PHP. 09, Jul 20. Scala | Try-Catch Exceptions. 12, Apr 19. Generating Errors using HTTP-errors module in Node.js. The Throw Statement. The throw statement is used to generate user-defined exceptions. During runtime, when a throw statement is encountered, execution of the current function will stop and control will be passed to the first catch clause in the call stack. If there is no catch clause, the program will terminate. Check out this example showing how to use a throw statement:
The JavaScript engine tracks such rejections and generates a global error in that case. You can see it in the console if you run the example above. In the browser we can catch such errors using the event unhandledrejection: Jan 07, 2019 - Sometimes it is necessary to execute ... is an Error or not. You can use the third, optional block finally for that. Often, it is the same as just having a line after the try … catch statement, but sometimes it can be useful. ... Asynchronity, one topic you always have to consider when working with JavaScript... The try/catch/finally statement handles some or all of the errors that may occur in a block of code, while still running code. Errors can be coding errors made by the programmer, errors due to wrong input, and other unforeseeable things. The try statement allows you to define a block of code to be tested for errors while it is being executed.
Apr 26, 2019 - Handling errors well can be tricky. How Error() historically worked in JavaScript hasn’t made this easier, but using the Error class introduced in ES6 can be helpful 8/6/2020 · All JavaScript errors can be caught by this browser event onerror. It is one of the easiest ways to log client-side errors and report them to your servers. A function is assigned to the window.onerror as such: Syntax: window.onerror = function (msg, source, lineNo, columnNo, error) { // function to execute error handling } If any statement within the try -block (or in a function called from within the try -block) throws an exception, control is immediately shifted to the catch -block. If no exception is thrown in the try -block, the catch -block is skipped. The finally -block will always execute after the try -block and catch -block (s) have finished executing.
Exception Handling in JavaScript The try-catch. As with many programming languages, the primary method of dealing with exceptions in JavaScript is the try-catch. In a nutshell, the try-catch is a code block that can be used to deal with thrown exceptions without interrupting program execution. Nov 22, 2017 - Learn how to handle JavaScript Errors with Try, Throw, Catch, & Finally ... Udemy Black Friday Sale — Thousands of Web Development & Software Development courses are on sale for only $10 for a limited time! Full details and course recommendations can be found here. ... Errors are inevitable. Unless you want to handle the error in a very generic (simple / catch-all etc) way, don't throw an error.
After the catch block finishes—or if the try block finishes without problems—the program proceeds beneath the entire try/catch statement. In this case, we used the Error constructor to create our exception value. This is a standard JavaScript constructor that creates an object with a message ... A Simple Try Catch Let's start with the simple try...catch example. This works as expected, we call the function thisThrows () which throws a regular error, we catch it, log the error and optionally we run some code in the finally block. No rocket science here. While JavaScript does offer the ability to add conditionals directly within the catch definition, this behavior is considered non-standard, so the more reliable way to implement conditional catch clauses is to use a conditional block within the catch to check and react to the error instance.
23/2/2018 · To catch all JavaScript errors, use onerror () method. The onerror event handler was the first feature to facilitate error handling in JavaScript. The error event is fired on the window object whenever an exception occurs on the page. The onerror event handler provides three pieces of information to identify the exact nature of the error − Apr 01, 2016 - Okay, so you’ve done your job – you’ve plugged into window.onerror, and you’re additionally wrapping functions in try/catch in order to catch as much error information as possible. There’s just one last step: transmitting the error information to your servers. Apr 04, 2020 - Summary: in this tutorial, you will learn how to handle errors by using the JavaScript try...catch statement. ... In this statement, you place the code that may cause errors in the try block and the code that handles the error in the catch block.
No longer are you forced to settle for what the browser throws in your face in an event of a JavaScript error, but instead can take the matter into your own hands. The try/catch/finally statement of JavaScript lets you dip your toes into error prune territory and "reroute" when a JavaScript ... The function readUser will catch data reading errors that occur inside it, such as ValidationError and SyntaxError, and generate a ReadError instead. The ReadError object will keep the reference to the original error in its cause property. Get Weekly Developer Tips. I send out a short email each friday with code snippets, tools, techniques, and interesting stuff from around the web.
A Guide To Proper Error Handling In Javascript Sitepoint
Proper Throw Syntax In Javascript Stack Overflow
Javascript Errors A Comprehensive Guide To Master Error
Learn How To Handle Javascript Errors With Try Throw Catch
Js Try Catch Get Error Message Code Example
Github Gautamkrishnar Tcso Try Catch Stack Overflow Tcso
Catch Errors In A Javascript Promise Chain With Promise Prototype Catch
Handling Errors In Javascript Using The Try Catch Statement
Finally In Javascript How Does Finally Works In Javascript
Javascript Error Handling Handle Errors In Javascript Try
Rethrowing Errors In Javascript And Node Js
A Mostly Complete Guide To Error Handling In Javascript
Asynchronous Javascript With Promises Amp Async Await In
Learn How To Handle Javascript Errors With Try Throw Catch
Multiple Api Calls Javascript Try Catch And Async Await Code
Try Catch In Javascript How To Handle Errors In Js
How To Implement Error Handling In Sql Server
Async Await Not Working With Then And Catch Stack Overflow
Javascript Errors A Comprehensive Guide To Master Error
0 Response to "22 How To Catch Error In Javascript"
Post a Comment