22 Validate Password Regex Javascript
Recently one of my Twitter followers asked me how they might validate password strength using regular expressions (RegEx) in their code. Regular expressions via Wikipedia: A sequence of characters that forms a search pattern, mainly for use in pattern matching with strings, or string matching. The regular expressions class. Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. Regex is also denoted as RegExp. They can be constructed using: The Regular Expression literal where the pattern is enclosed between two slashes.
Validator Js How To Validate Alphanumeric Password In
The Password strength validation in JavaScript and jQuery will be performed using Regular Expressions (Regex). TAGs: JavaScript, jQuery, Regular Expressions Here Mudassar Ahmed Khan has explained how to implement Password Strength validation for Password TextBox with examples in JavaScript and jQuery.
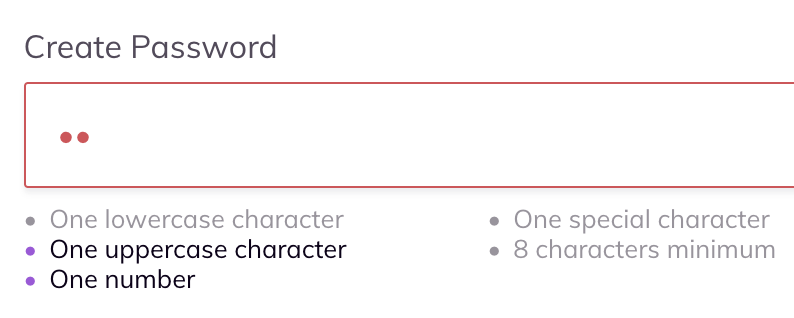
Validate password regex javascript. Using JavaScript to validate passwords in a web browser can be very beneficial for your users, but make sure to also implement your validation routine on the server. If you don't, it won't work for users who disable JavaScript or use custom scripts to circumvent your client-side validation. function validatePassword() { var p = document.getElementById('newPassword').value, errors = []; if (p.length < 8) { errors.push("Your password must be at least 8 characters"); } if (p.search(/[a-z]/i) < 0) { errors.push("Your password must contain at least one letter."); } if (p.search(/[0-9]/) < 0) { errors.push("Your password must contain at least one digit."); } if (errors.length > 0) { … Java regex validate number; java regex pattern validate 12 hours format; java regex validate alphanumeric; java regex pattern validate image file extension; java regex pattern validate ip address; Java regex validate alphabets; Java regular expression metacharacters; java regex pattern validate hex code; JavaScript math sqrt() method; java ...
Regular Expressions Password validation regex A password containing at least 1 uppercase, 1 lowercase, 1 digit, 1 special character and have a length of at least of 10 As the characters/digits can be anywhere within the string, we require lookaheads Regular expressions don't have an AND operator, so it's pretty hard to write a regex that matches valid passwords, when validity is defined by something AND something else AND something else... As you can see the function validate () checks if the entered string contains characters that does NOT (notice the ^ symbol) match the numbers 0 to 9 including white spaces and special characters. Since I've seen tons of password validation help requests on regexadvice (where I hang out from time to time), I've written up a more general-purpose JavaScript password validation function. It's reasonably straightforward, and covers the validation requirements I've most frequently encountered. Plus, if it doesn't handle your exact needs, its functionality can be augmented by…
Using regex to validate a password strength can be quite a handy trick where password requirements keep getting stricter. A typical scenario for a complex password in this day and age would be a password with a minimum of 8 characters, including uppercase, lowercase and special characters. Confirm password validation in JavaScript. In this chapter, we will discuss password validation using JavaScript. We need to validate a password every time whenever a user creates an account on any website or app. So, we have to verify a valid password as well as put the confirm password validation. Password Validation Modification at User Registration. Open RegisterController.php which is located at App > Http > Controllers > Auth Directory. Under the validator() method. Modify the password validation rule to add the regex rule as well.
A software engineer provides a tutorial article on how to use the JavaScript language to write RegEx patterns that can help test the strength of our passwords. ... validate password strength using ... The \s metacharacter is used to find a whitespace character. A whitespace character can be: A space character. A tab character. A carriage return character. A new line character. A vertical tab character. A form feed character. Given a password, the task is to validate the password with the help of Regular Expression. A password is considered valid if all the following constraints are satisfied: It contains at least 8 characters and at most 20 characters. It contains at least one digit.
26/2/2020 · Here we validate various type of password structure through JavaScript codes and regular expression. Check a password between 7 to 16 characters which contain only characters, numeric digit s and underscore and first character must be a letter. Check a password between 6 to 20 characters which contain at least one numeric digit, one uppercase and one lowercase letter. Using Regex (Regular Expression), you can easily validate the password strength in PHP. One of the most popular ways of validating email in JavaScript is by using regular expressions. The password is getting updated if we have all the characters as alphabets. JavaScript uses regular expressions to describe a pattern of characters. How to Build a Password Validator with JavaScript. Published on March 13, 2015. Enforcing secure passwords is an essential part on any login system. The first step in this process is ensuring that users select a password which matches a set of criteria. ... Most of the methods use regular expressions to test the password, which means altering a ...
Given an email id and the task is to validate the email id is valid or not. The validation of email is done with the help of Regular Expressions. Approach 1: RegExp - It checks for the valid characters in the Email-Id (like, numbers, alphabets, few special characters.) Validate an ip address Url Validation Regex | Regular Expression - Taha match whole word Match or Validate phone number nginx test Match html tag Extract String Between Two STRINGS Blocking site with unblocked games Find Substring within a string that begins and ends with paranthesis Empty String Match dates (M/D/YY, M/D/YYY, MM/DD/YY, MM/DD/YYYY) JavaScript RegExp Email validation code, username validation, Number validation, URL validation, JavaScript regex strong password validation
Also The email address must start with any character. Javascript(JS) Email Validation without Regex and with Regex Examples. Email validation in JavaScript on button click. The domain name contains are Letters, Digits, Dots and Hyphens. Email Validation in JavaScript - Step by Step Example with source code. * Password validation RegEx for JavaScript * * Passwords must be * - At least 8 characters long, max length anything * - Include at least 1 lowercase letter * - 1 capital letter * - 1 number * - 1 special character => !@#$%^&* * * @author Harish Chaudhari <harishchaudhari > * */ var str = "password"; // your password field's value goes here / ^ (? =. * [\d]) (? =. Use the password input type: Instead of <input type="text">, use <input type="password"> characters in a password field which are masked (shown as asterisks or circles) snow that the contents of that field need to be secured. The password won't appear on the screen as you type and most browsers also won't 'remember' the values entered in ...
4. Regular Expressions/REGEX Regular expressions are patterns to match the original combination of literals passed in the field. In password validation, using JavaScript's regular expression plays an essential role in identifying the string and whether it is in the given format or not. Regular expressions are JavaScript objects. Confirm password with form validation in JavaScript Code examples Form validation is a process to validate that user is submitting the data according to correct format or not. Most of the time it happens that user enter the password during registration and he thinks that he enter the password as he thinks but unfortunately due to the wrong ... Learn how to validate a password that requires at least one lowercase letter, one uppercase letter and one number. Here's the regular expression we end up with in the video. It uses a "lookahead". Here's an article on lookaheads (and lookbehinds) in regular expressions.
3/4/2018 · Because the password input type obscures the text typed, you should let the user confirm that they haven't made a mistake. The simplest way to do this is to have the password entered twice, and then check that they are identical. Another method is to display what they've entered as part of a 'confirmation page'. JavaScript Learn JavaScript ... Learn how to create a password validation form with CSS and JavaScript. ... Note: We use the pattern attribute (with a regular expression) inside the password field to set a restriction for submitting the form: it must contain 8 or more characters that are of at least one number, ... RegExr is an online tool to learn, build, & test Regular Expressions (RegEx / RegExp). Supports JavaScript & PHP/PCRE RegEx. Results update in real-time as you type. Roll over a match or expression for details. Validate patterns with suites of Tests. Save & share expressions with others.
2/3/2010 · 1. You can make your own regular expression for javascript validations; (/^ (?=.*\d) //should contain at least one digit (?=.* [a-z]) //should contain at least one lower case (?=.* [A-Z]) //should contain at least one upper case [a-zA-Z0-9] {8,} //should contain at least 8 from the mentioned characters $/) Example:- /^ (?=.*\d) (?=.* ...
React 17 Form Validation Tutorial With Example Positronx Io
How Javascript Works Regular Expressions Regexp By
Question How To Do Multiple Checks For Password Validation
Javascript Ip Address Validation W3resource
10 Best Password Strength Checkers In Javascript 2021 Update
Validate Email Amp Password Regular Expressions Coding In Flow
How To Check Password Validation Dynamically Using Jquery
Javascript Validation On Name Code Example
How To Perform Email Validation In Javascript Edureka
Password Validation Using Regular Expressions And Html5
How To Create A Password Validation Form
Ui For Password Validation Codemyui
Confirm Password Validation In Javascript Javatpoint
How To Give Password Validation In Extjs In Runtime Stack
Password Amp Password Confirmation Validation In Laravel 6
Javascript Validation In Asp Net Website Asp Net C Net Vb
The Javascript Ecosystem 3 Dynamic Input Validation Using
Php Form Validation With Example Codingstatus
Password Validation With Vanilla Js And Bootstrap 4
Use Regex Lookaheads To Validate A Password In Javascript Echo
Strong Password Validation Codeproject
0 Response to "22 Validate Password Regex Javascript"
Post a Comment