23 Javascript Random Integer Between Two Numbers
To create a random number in Javascript, the math.random () function is used. JavaScript math.random () generates a random decimal value between 0 and 1. The function does not take any arguments. To get random numbers higher than 1 simply multiply the result according to the need. Find Your Bootcamp Match. Generate a Random Number Between Two Numbers in JavaScript. If we also want to have a user-defined minimum value, we need to change the equation of Math.random () * max to Math.random () * (max-min)) +min. Using this equation the returned value is a random number between min and max. JavaScript.
Let us see some of the examples to generate random numbers: 1. Use of Math.random () function. We have the Math. random () function in JavaScript to deal with the random numbers. This number always return less than 1 as a result. This will always give the result in the form of a decimal point. 2.
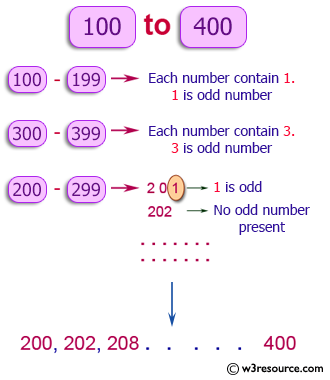
Javascript random integer between two numbers. We can use Math.floor and Math.random () method to generate a random number between two numbers where both minimum and the maximum value is included in the output. This below example shows you a function that is used to generate a random number based on the arguments we passed to it. function randomNumber(min, max) { return Math.floor(Math ... As you can see from the examples above, it might be a good idea to create a proper random function to use for all random integer purposes. This JavaScript function always returns a random number between min (included) and max (excluded): Learn JavaScript - Get Random Between Two Numbers. Example. Returns a random integer between min and max:. function randomBetween(min, max) { return Math.floor(Math.random() * (max - min + 1) + min); }
Generating random whole numbers in JavaScript in a specific range? 3256. What is the difference between call and apply? 2193. How to print a number with commas as thousands separators in JavaScript. 2103. Generate random number between two numbers in JavaScript. 1829. What is the difference between Bower and npm? Hot Network Questions Correct ... If you need to generate a random number between two numbers in JavaScript, then you are in the right place! Here you will learn javascript random number generation. We can easily do this by using a combination of built-in Math functions. Javascript Random Number from Math.random() Let's start by using Math.random() to In JavaScript, you can generate a random number with the Math.random () function. Math.random () returns a random floating-point number ranging from 0 to less than 1 (inclusive of 0 and exclusive of 1) The above program will show an integer output between min (inclusive) to max (inclusive). First, the minimum and maximum values are taken as input ...
In JavaScript, you can use the Math. random () function to generate a pseudo-random floating number between 0 (inclusive) and 1 (exclusive). If you want to get a random number between 0 and 20, just multiply the results of Math.random () by 20: To generate a random whole number, you can use the following Math methods along with Math.random (): How to generate a random number between two numbers in JavaScript The simplest possible way to randomly pick a number between two. Published Oct 20, 2018. Use a combination of Math.floor() ... JavaScript Python React HTML CSS Node.js Linux C Express.js Next.js Vue.js Svelte Deno ES5 to ESNext. We have used the Math.random() method to generate the random values and Math.floor method to round a number downward to its nearest integer. You have to pass the min and max values in the parameter of the function to generate a random number between those values. 2. Get a random number between 0 to n number
function getR(lower, upper) { var percent = (Math.random() * 100); // this will return number between 0-99 because Math.random returns decimal number from 0-0.9929292 something like that //now you have a percentage, use it find out the number between your INTERVAL :upper-lower var num = ((percent * (upper - lower) / 100)); //num will now have a ... How can I generate random whole numbers between two specified variables in JavaScript, e.g. x = 4 and y = 8 would output any of 4, 5, 6, 7, 8? Program 1: Math.random () can be used to get a random number between two values. The returned value is no lower than min and may possibly be equal to min, and it is also less than and not equal to max. For getting a random number between two values the math.random () function can be executed in the following way: <script type="text/javascript">.
If you want to get an integer instead of a float, you should apply the Math.floor () in combination with Math.random (). So, if you need to get a random integer between 1 and 5, you should calculate it as follows: let math = Math .floor ( Math .random () * 5) + 1 ; console .log (math); Javascript math floor and math random method. 9/2/2011 · This function can generate a random integer number between (and including) min and max numbers: function randomNumber(min, max) { if (min > max) { let temp = max; max = min; min = temp; } if (min <= 0) { return Math.floor(Math.random() * (max + Math.abs(min) + 1)) + min; } else { return Math.floor(Math.random() * (max - min + 1)) + min; } } Sep 21, 2020 - All the random values will be generated between those two arguments. In the above example, all the values are generated in floating values. You can also generate the integer values as well. See the following example.
How to return a random number between 0 and 199 with JavaScript? How to return a random number between 1 and 200 with JavaScript? Get a random value between two values in MySQL? Finding the nth palindrome number amongst whole numbers in JavaScript; MySQL update with random number between 1 - 3; How do I convert a float number to a whole number ... JavaScript: get a random number from a specific range - random.js. JavaScript: get a random number from a specific range - random.js ... One modification could be done with ES6 default parameters to allow it to take either one or two args. function random (n, b = 0) {return Math ... I know this isn't recent but any tips on actually getting ... In this post, we will fetch a random integer between two numbers we define. I.E. let's say we want a random number between 1 and 100. We will use methods from the MATH object to achieve this. More specifically the Random and Floor methods.. Let's first see what the random method does:
Apr 16, 2021 - Javascript random numbers are essential for simulating random behavior in computing, but are never truly random and may not be cryptographically secure. Write a JavaScript function to generate a random integer. ... Learn how Grepper helps you improve as a Developer! ... Write a function that accepts an array of 10 integers (between 0 and 9), that returns a string of those numbers in the form of a phone number. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
See the Pen javascript-math-exercise-4 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to convert a decimal number to binary, hexadecimal or octal number. Next: Write a JavaScript function to format a number up to specified decimal places. This article describes how to generate a random number using JavaScript. Method 1: Using Math.random () function: The Math.random () function is used to return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive). This random number can then be scaled according to the desired range. The above snippet will print a random number in the JavaScript console. Now, we know how to print a random number, but what actually we need is, we have to print a random number between two values, so let's write another example: 2. JavaScript to print a random number between two given values:
13/9/2017 · Random number generation is the generation of a sequence of numbers or symbols that cannot be reasonably predicted better than by a random chance, usually through a random-number generator (RNG). Computer random number generators are important in mathematics, cryptography and gambling (on game servers). In this article, we will be generating a random integer between given two numbers using JavaScript. We will be generating a random number in a given range using JavaScript … The Math.random() function returns a floating-point, pseudo-random number in the range 0 to less than 1 (inclusive of 0, but not 1) with approximately uniform distribution over that range — which you can then scale to your desired range. The implementation selects the initial seed to the random number generation algorithm; it cannot be chosen or reset by the user. Aug 28, 2018 - A short guide to .ceil, .floor, and .round. “Using Math.random() in JavaScript” is published by Joe Cardillo.
In this tutorial, we will learn about the JavaScript Math.random() function with the help of examples. The Math.random() function returns a floating-point, pseudo-random number between 0 (inclusive) and 1 (exclusive). ... Example 3: Generate random integer between two numbers You can get a random integer between 1 (and only 1) and 6, you would calculate. Use this Math.floor(Math.random() * 6) + 1 to get random number between.. Aug 24, 2018 - To generate random number between two numbers is so simple you just need to understand how the Math.random() works in Javascript.
Dec 28, 2020 - For weighted random or normal distributed random with JavaScript, read this article instead. ... Return integer between min and max (inclusive) Negative numbers are supported. A Proper Random Function. As you can see from the examples above, it might be a good idea to create a proper random function to use for all random integer purposes. This JavaScript function always returns a random number between min (included) and max (excluded): Oct 09, 2015 - Last Edited at 10/09/15 08:19pm · Report this snippet Tweet
How can I generate random whole numbers between two specified variables in JavaScript, e.g. x = 4 and y = 8 would output any of 4, 5, 6, 7, 8? JavaScript Tip: get a Random Number between two Integers. Teylor Feliz August 24, 2010. Many languages like PHP has a random function which takes two integers as parameters and returns a number in the range of the value of the two integers. In JavaScript, we … Write a JavaScript function to generate a random integer. ... Learn how Grepper helps you improve as a Developer! ... Write a function that accepts an array of 10 integers (between 0 and 9), that returns a string of those numbers in the form of a phone number. ... 14. Write a JavaScript function to find the difference of two ...
Random Integer Between X and Y (Exclusive Y) Let's say you want to generate an integer in a range between X and Y. For example, a number larger than 1024, but no bigger than 49151. Create a new method called between which can be part of your app's utilities. This between method accepts two arguments: min and max.
How To Generate A Random Number Between Two Numbers In
How To Generate A Random Number Between Two Numbers In
Working With Numbers In Javascript
For This Project You Ll Create A Webpage That Hosts Chegg Com
Seeding The Random Number Generator In Javascript Stack
Generate Random Integer Between Two Numbers Using Javascript
Python Exercise Find Numbers Between Two Numbers Where Each
Techniques For Generating A Simple Random Sample Video
Changing Program Behavior With Conditions In Javascript
Generate Random Integer Between Two Numbers Using Javascript
Generate Random Integer Between Two Numbers Using Javascript
Ms Excel How To Use The Rand Function Ws
Generate Random Integer Between Two Numbers Using Javascript
Program To Find Prime Numbers Between Given Interval
React Native Generate Random Number Between 1 To 100 In
Java67 3 Ways To Create Random Numbers In A Range In Java
Javascript Random Number Between 1 And 10 Design Corral
Generate A Random Number In Javascript
Javascript Number Between Design Corral
Generate Random Number In Java Within A Range Without
How To Generate A Random Number Between 1 And 6 In Javascript
Function Challenge How To Javascript Functions Treehouse
0 Response to "23 Javascript Random Integer Between Two Numbers"
Post a Comment