23 Javascript Replace All Characters
You could also pass a regular expression (regex) inside the replace() method to replace the string.. Example 2: Replace Character of a String Using RegEx // program to replace a character of a string const string = 'Mr Red has a red house and a red car'; // regex expression const regex = /red/g; // replace the characters const newText = string.replace(regex, 'blue'); // display the result ... String.prototype.replace () The replace () method returns a new string with some or all matches of a pattern replaced by a replacement. The pattern can be a string or a RegExp, and the replacement can be a string or a function to be called for each match. If pattern is a string, only the first occurrence will be replaced.
Find And Replace Characters In A String In Excel Vba
That's because the replace function returns a new string with some or all matches of a pattern replaced by a replacement.. If you need to swap characters, you can use a regex in your case (but this is not the best implementation):function symbExchange(line) { var tmp = line[0]; var str = line.replace(new RegExp('^' + line[0]), line[line.length-1]); var str2 = str.replace(new RegExp(str[str ...
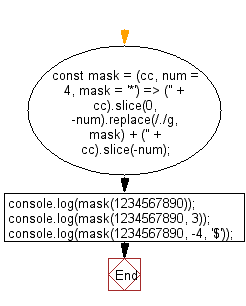
Javascript replace all characters. 21/1/2010 · newStr = str.replace (/ [^a-z0-9]/gi, '_'); or. newStr = str.replace (/ [^a-zA-Z0-9]/g, '_'); This is going to replace all the character that are not letter or numbers to ('_'). Simple change the underscore value for whatever you want to replace it. Share. Normally JavaScript's String replace () function only replaces the first instance it finds in a string: app.js. const myMessage = 'this is the sentence to end all sentences'; const newMessage = myMessage.replace('sentence', 'message'); console.log(newMessage); Copy. In this example, only the first instance of sentence was replaced. Javascript is a language, This is the most popular language. The function will replace all the special characters with empty where whitespaces, comma, and dots are ignored.
However, the replace() will only replace the first occurrence of the specified character. To replace all the occurrence you can use the global ( g ) modifier. The following example will show you how to replace all underscore ( _ ) character in a string with hyphen ( - ). The .replace method is used on strings in JavaScript to replace parts of Regular Expressions (also called RegEx or RegExp) are a powerful way to analyze text. With RegEx, you can match strings at points that match specific characters (for example, JavaScript) or patterns (for example, NumberStringSymbol - 3a&). 24/7/2020 · To make the method replace() replace all occurrences of the pattern you have to enable the global flag on the regular expression: Append g after at the end of regular expression literal: /search/g; Or when using a regular expression constructor, add 'g' to the second argument: new RegExp('search', 'g') Let’s replace all occurrences of ' ' with '-':
Welcome to a quick tutorial on how to replace characters in a string in Javascript. Need to check and replace certain characters in Javascript? There are actually a number of ways to do it: var replaced = STRING.replace ("FROM", "TO"); var replaced = STRING.replaceAll ("FROM", "TO"); var replaced = STRING.replace (/FROM/g, "TO"); Example 1: Replace All Instances Of a Character Using Regex // program to replace all instances of a character in a string const string = 'Learning JavaScript Program'; const result = string.replace(/a/g, "A"); console.log(result); Output. LeArning JAvAScript ProgrAm. In the above example, the RegEx is used with the replace() method to replace all the instances of a character in a string. The replace () method searches a string for a specified value, or a regular expression, and returns a new string where the specified values are replaced. Note: If you are replacing a value (and not a regular expression ), only the first instance of the value will be replaced. To replace all …
Well, there isn't any JavaScript replace all method available out of the box but a JavaScript replace all regex is available. To search and replace all the occurrences of a string in JavaScript using the string.replace() method, you'll have to pass the search string as a regular expression . Removing all special characters in JavaScript To remove the accents and other special characters like /?!(), just use the same formula above, only replace everything but letters and numbers. const str = 'ÁÉÍÓÚáéíóúâêîôûàèìòùÇç/., [email protected] #$%&_-12345'; const parsed = str.normalize('NFD').replace(/([\u0300-\u036f]|[^0-9a-zA-Z])/g, ''); console.log(parsed); You can write a negated character class with ^ to include the period,., and the set of digits, 0-9: var z = x.replace (/ [^0-9.]/g, ''); z is then "100.00".
JavaScript regex - How to replace special characters? Javascript Web Development Front End Technology Object Oriented Programming. To replace special characters, use replace () in JavaScript. The syntax is as follows −. anyVariableName.replace (/ (^\anySymbol)|,/g, ''); 7/7/2020 · Javascript replace() method replaces only the first occurrence of the string. To replace all occurrences of a string in Javascript, use the below methods. Javascript string replace method with regular expression; Javascript split and join method; Javascript indexOf method; Take a look at the below example of Javascript Replace() method The replaceAll () method returns a new string with all matches of a pattern replaced by a replacement. The pattern can be a string or a RegExp, and the replacement can be a string or a function to be called for each match. The original string is left unchanged.
Replace multiple characters in string using split () and join () You can do modifications on a string using JavaScript replace method. replace method is an inbuilt function in JavaScript which is used to change particular character, word, space, comma, or special char in Strings. You can use the JavaScript replace () method in combination with the regular expression to find and replace all occurrences of a word or substring inside any string. Let's check out an example to understand how this method basically works:
In JavaScript, replacing a single occurrence of a character in a string is simple and easy but, if you want to r eplace all the occurrences of a particular character, it becomes a bit tricky.In this article, we will first see how to replace a single occurrence and then we will try to replace all the occurrences of a character in a string. String.replace (): Replace All Appearances. String replacements are a common task in app development. JavaScript has powerful string methods and you intentionally think of string.replace () when reading the headline. Good catch, but there's a trick to replacing all appearances when using it. Read on to get the details! The replace () method in JavaScript searches a string for a specified value or a regular expression and returns a new string with some or all matched occurrences replaced. The replace () method accepts two parameters: const newStr = string.replace( substr | regexp, newSubstr |function); The first parameter can be a string or a regular expression.
Introduced in ES12, the replaceAll () method returns a new string with all matches replaced by the specified replacement. Similar to String.prototype.replace (), replaceAll () allows a string or a regular expression to find matches. Description. In JavaScript, replace () is a string method that is used to replace occurrences of a specified string or regular expression with a replacement string. Because the replace () method is a method of the String object, it must be invoked through a particular instance of the String class. The above code will only replace the first occurrence of "MooTools" -- not every occurrence like PHP's str_replace () would. To replace every occurrence of a string in JavaScript, you must provide the replace () method a regular expression with a global modifier as the first parameter: var replaced = 'The MooTools JavaScript library is is great.
30/4/2019 · In order to remove all non-numeric characters from a string, replace () function is used. replace () Function: This functiion searches a string for a specific value, or a RegExp, and returns a new string where the replacement is done. 2/2/2021 · Y ou can use the replace() method in JavaScript to replace the occurrence of a character in a string. However, the replace() method will only replace the first occurrence of the specified character. To replace all occurrences, you can use the global modifier (g). The following example will show you how to replace all underscores (_) in a string with hyphens (-). Example :
Notepad Simple Trick To Replace Multiple Spaces With
Find And Replace Text And Multi Caret Selection Visual
How To Replace Characters In A String In Javascript Simple
Excel Formula Find And Replace Multiple Values Exceljet
Replace Function Javascript Code Example
Regular Expression To Replace Special Characters From String
How To Replace All After Before Specific Character Or Space
Javascript Fundamental Es6 Syntax Replace All But The Last
Replace All Special Characters In Javascript
Find And Replace In Text Files With Ultraedit
3 Ways To Replace All String Occurrences In Javascript
How To Find And Replace Special Characters In Microsoft Word
Python Program To Replace Characters In A String
Javascript Regular Expression To Replace Escape Special Characters From String
Github Zipavlin Toascii Js Replace Non Ascii Characters
Html Special Characters Javascript Decode Code Example
Everything You Need To Know About Regular Expressions By
Javascript Replace A Step By Step Guide Career Karma
Replace All Characters With Specific Character In Javascript
Javascript Replace All Instances Of A String Scotch Io
Javascript Replace All Occurrences Of String Code Example
Javascript Replace Multiple Characters Design Corral
0 Response to "23 Javascript Replace All Characters"
Post a Comment