20 Object Functions In Javascript
Get all functions of an object in JavaScript. Ask Question Asked 9 years, 11 months ago. Active 5 years, 6 months ago. Viewed 17k times 8 3. For example, Math.mymfunc = function (x) { return x+1; } will be treated as a property and when I write. for(var p in Math.__proto__) console.log(p) ... In JavaScript, the thing called this is the object that "owns" the code. The value of this, when used in an object, is the object itself. In a constructor function this does not have a value. It is a substitute for the new object.
Cheat Sheet Javascript Object Functions Part 1
A JavaScript function is a block of code designed to perform a particular task. A JavaScript function is executed when "something" invokes it (calls it).
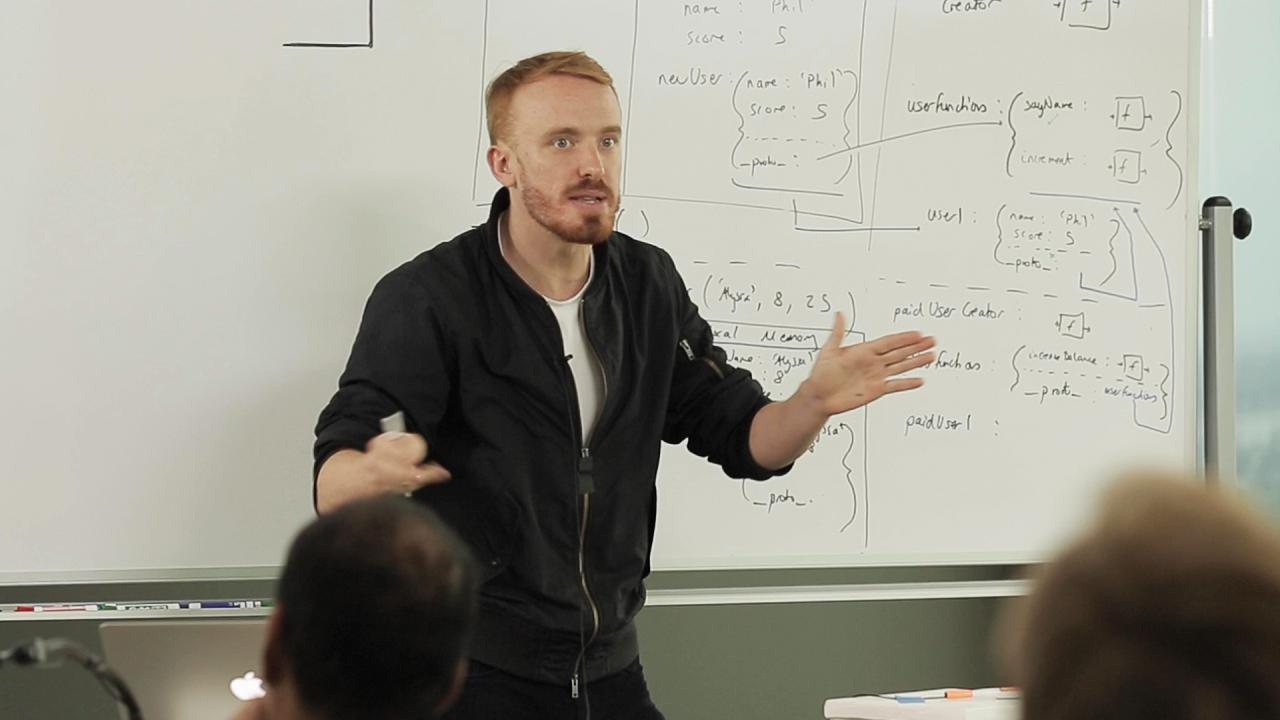
Object functions in javascript. The characteristics of an Object are called as Property, in Object Oriented Programming and the actions are called methods. An Object is an instance of a class. Objects are everywhere in JavaScript almost every element is an Object whether it is a function,arrays and string. Note: A Method in javascript is a property of an object whose value is ... Above, p1 and p2 are the names of objects. Objects can be declared same as variables using var or let keywords. The p1 object is created using the object literal syntax (a short form of creating objects) with a property named name.The p2 object is created by calling the Object() constructor function with the new keyword. The p2.name = "Steve"; attach a property name to p2 object with a string ... JavaScript Objects • JavaScript is an object-based language • It supports for object -oriented programming but not at the same level as other languages (ES6: introduced class – still lacks private property) • Objects are represented as property-value pair • The property values can be data or functions (methods)
JavaScript function basics. A JavaScript (Node.js) function is an exported function that executes when triggered (triggers are configured in function.json). The first argument passed to every function is a context object, which is used for receiving and sending binding data, logging, and communicating with the runtime. Folder structure In JavaScript, functions are objects. You can work with functions as if they were objects. For example, you can assign functions to variables, to array elements, and to other objects. They can also be passed around as arguments to other functions or be returned from those functions. The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. Description Arrays are list-like objects whose prototype has methods to perform traversal and mutation operations.
JavaScript object literals can contain not only properties, but methods too. Some may automatically think of a JavaScript object as a name / value pair scheme with flat members that are essentially just scalar values. Fortunately, this is not quite the case. An object literal can also include functions among its members. One of the best parts of JavaScript is the way it makes working with objects and functions easy. There are two "original" ways to make an object, a variation on a theme, and a brand new way ... To understand object methods, I first need to take a closer look at JavaScript functions. JavaScript Functions Are First Class. In many programming languages, functions and objects are usually considered two different things. In JavaScript, this distinction is blurred—a JavaScript function is really an object with executable code associated ...
The Object class represents one of JavaScript's data types. It is used to store various keyed collections and more complex entities. Objects can be created using the Object() constructor or the object initializer / literal syntax. Accessing Object Methods. You access an object method with the following syntax: objectName.methodName () You will typically describe fullName () as a method of the person object, and fullName as a property. The fullName property will execute (as a function) when it is invoked with (). The JavaScript Math object allows you to perform mathematical tasks on numbers.
JS Functions Are Objects # JavaScript Functions are Objects! Yes, in JS world a function is considered an object, and to explain the reason why we will have to learn about types. Types in JavaScript are categorized by: Almost everything in JavaScript is an object. An object is a collection of key-value properties. An object can be created with either object literal syntax or object constructor function syntax. Whenever a new function is created in JavaScript, the JavaScript engine automatically attaches a prototype property to it. Changes made to this ... The object whose class is Object seems quite different from the usual class instance object, because it acts like an associative array or list: it can be created by simple object literals (a list of keys and properties), like this: let obj={A:'a',B:'b'}; and because it looks very like this same literal notation when displayed in the Developer Tools Console pane and when it is converted to a ...
First, every object in Javascript inherits from the Object object. //these do the same thing var foo = new Object(); var bar = {}; Second, functions ARE objects in Javascript. Specifically, they're a Function object. The Function object inherits from the Object object. Checkout the Function constructor Returns a number indicating the Unicode value of the character at the given index. 3. concat () Combines the text of two strings and returns a new string. 4. indexOf () Returns the index within the calling String object of the first occurrence of the specified value, or -1 if not found. 5. In JavaScript, functions are first-class objects, because they can have properties and methods just like any other object. What distinguishes them from other objects is that functions can be called. In brief, they are Function objects.
Functions that are stored in object properties are called "methods". Methods allow objects to "act" like object.doSomething (). Methods can reference the object as this. The value of this is defined at run-time. Function() Creates a new Function object. Calling the constructor directly can create functions dynamically but suffers from security and similar (but far less significant) performance issues to Global_Objects/eval. However, unlike eval, the Function constructor creates functions that execute in the global scope only. A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output.
The concept of objects in JavaScript can be understood with real life, tangible objects. In JavaScript, an object is a standalone entity, with properties and type. Compare it with a cup, for example. A cup is an object, with properties. A cup has a color, a design, weight, a material it is made of, etc. The same way, JavaScript objects can have properties, which define their characteristics. JavaScript objects are containers for named values called properties. The generator function in JavaScript returns a Generator object. Its syntax is similar to function expression, function declaration or method declaration, just that it requires a star character *. The generator function can be declared in the following forms:
As demonstrated in the chapter Function binding functions in JavaScript have a dynamic this. It depends on the context of the call. It depends on the context of the call. So if an object method is passed around and called in another context, this won't be a reference to its object any more. In JavaScript, everything that is not a primitive is an object. This includes functions, which are basically a special type of object that can be "called" with the () syntax. JavaScript provides a number of built-in functions that have various purposes. Two such functions happen to be called Object and Function. 26/2/2020 · JavaScript function objects are used to define a piece of JavaScript code. This code can be called within a JavaScript code as and when required. Can be created by function constructor. Code defined by a function can be called by function (). Javascript Function Objects Property. Name.
Seeing Javascript Objects As Functions Totally Changed The
Proto Vs Prototype In Javascript Stack Overflow
Object Oriented Javascript For Beginners Learn Web
Everything About Javascript Objects By Deepak Gupta
How To Use Object Destructuring In Javascript
Array Object Properties And Methods In Javascript
Learning Javascript With Object Graphs Part Ii How To
Frequently Misunderstood Javascript Concepts
Learn Functions Are Objects Amp Functions The Hard Parts Of
Mastering This In Javascript Callbacks And Bind Apply
What Is The Arguments Object In A Javascript Function
Javascript Strings Find Out Different Methods Of String
Advanced Javascript Objects And Functions D Z Notes Beta
Javascript Functions Objects Prototypes
0 Response to "20 Object Functions In Javascript"
Post a Comment