30 How To Check Not Null In Javascript
The value null represents the object value or variable value is don't have any value ("No value"). There is no isNull function in JavaScript script to find without value objects. However you can build your own isNull () function with some logics. Examples of Check null in JavaScript At the end we see that even though null and undefined are considered equal, they are not the same identity (equal without type conversion).As discussed, this is because they are of different types behind the scenes: null being an object and undefined being an undefined type. With that out of the way we can start to understand why trying to access a property of null or undefined may fail.
Count Cells If Not Blank Non Empty Cells In Google Sheets
You can easily check if a variable Is Null or Not Null in JavaScript by applying simple if-else condition to the given variable. There are two ways to check if a variable is null or not. First I will discuss the wrong way that seems to be right to you at first, then we will discuss the correct way to check if a variable is null or not.
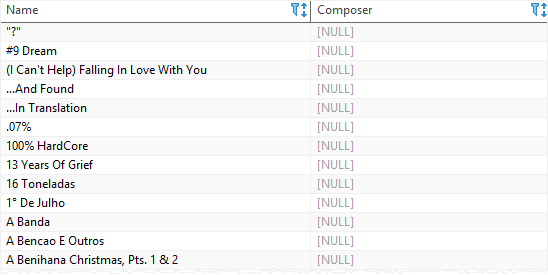
How to check not null in javascript. Feb 09, 2017 - Evaluate whether a variable is not null. In other instances, you may want to run code when a value is not null. The "!" operator tells the compiler to run the statements if the result is false. In this statement, JavaScript runs statements if myVal is not null: Using === operator we will check the string is empty or not. If empty then it will return "Empty String" and if the string is not empty it will return "Not Empty String" Nov 08, 2011 - The double-not operator !! will remove more than just null. It will convert NaN and undefined into false as well. And that's why your array filters correctly when using the double not-operator !!. ... Use !== as != will get you into a world of nontransitive JavaScript truth table weirdness. ... Unless you want to specifically check ...
Aug 17, 2020 - You can see that if you check null === undefined, it returns false. That means, if we strictly check both values, then they are not the same. So, if you are explicitly checking null value, then use the triple equality operator(===). It is the recommended operator to check null values in JavaScript. Because of this interesting property, ... way of checking whether a given value is "nothing" (null/undefined) or "something" (anything else). In addition to jQuery, you can find examples of this convention in Underscore.and Less. Indeed, JSHint, one of the more popular JavaScript linting tools, ... In this example, you will learn to write a JavaScript program that will check if a variable is undefined or null.
How to Check for Empty/Undefined/Null String in JavaScript In JavaScript , one of the everyday tasks while validating data is to ensure that a variable , meant to be string , obtains a valid value. Null: It is the intentional absence of the value. It is one of the primitive values of JavaScript. Undefined: It means the value does not exist in the compiler. It is the global object. You can see refer to "==" vs "===" article. It means null is equal to undefined but not identical. When we define a variable to undefined then we are ... There are 7 falsy values in JavaScript - false, 0, 0n, '', null, undefined and NaN. A nullish value consists of either null or undefined. This post will provide several alternatives to check for nullish values in JavaScript. To check for null variables, you can use a strict equality operator (===) to compare the variable with null.
Approach 1: We can implement coalescing in pre-ES6 JavaScript by looping through the arguments and checking which of the arguments is equal to NULL. We then return the argument that is not NULL immediately. Approach 2: We can use the ES6 alternative to the above method to achieve a similar effect. Using Rest Parameters in ES6, we collect all ... To check for null SPECIFICALLYyou would use this: if (variable === null) This test will ONLYpass for nulland will not pass for "", undefined, false, 0, or NaN. Additionally, I've provided absolute checks for each "false-like" value (one that would return true for !variable). Here's a Code Recipe to check if an object is empty or not. For newer browsers, you can use plain vanilla JS and use the new "Object.keys" 🍦 But for older browser support, you can install the Lodash library and use their "isEmpty" method 🤖 const empty = {}; Object.keys(empty).length === 0 && empty.constructor === Object _.isEmpty(empty)
JavaScript checking null | Using equality operator. Posted July 13, 2020. May 15, 2021. by Rohit. In JS null value means no value or absence of a value. Checking null in Javascript is easy, you can use the equality operator == or strict equality operator === (also called identity operator). February 3, 2021 June 25, 2021 amine.kouis 0 Comments check if value is null javascript, check if variable is undefined javascript, how to check undefined in jquery, javascript check for null or empty string, javascript check if not undefined, javascript check if variable is defined, javascript check undefined or null or empty, jquery check if ... O ne way to check for null in JavaScript is to check if a value is loosely equal to null using the double equality == operator: As shown above, null is only loosely equal to itself and undefined, not to the other falsy values shown.
So you don't have to use comparison ... or !== to check for null values. ... Undefined is also a primitive value in JavaScript. A variable or an object has an undefined value when no value is assigned before using it. So you can say that undefined means lack of value or unknown value. ... In the above example, we have not assigned any ... Nov 19, 2020 - That is because a variable that ... not null. Unfortunately, typeof returns "object" when called on a null value, because of a historical bug in JavaScript that will never be fixed. That means checking for null cannt be performed using typeof. ... “null is a falsy value (i.e. it evaluates to false if coerced ... To check for null values in JavaScript, you need to check the variable value with null. Use the JavaScript strict equality operator to achieve this.
the Correct Way to Check Variable Is Not Null if (myVar !== null) {...} The above code is the right way to check if a variable is null because it will be triggered only in one condition - the variable is actually null. For example, if you wanted to check if the fax number field on the Account record is empty, first open the form and find the field name: Get the field name: Now if we add the following script to the loading of the account form: JavaScript for impatient programmers (ES2021 edition) Please support this book: buy it or donate ... Many programming languages have one “non-value” called null. It indicates that a variable does not currently point to an object – for example, when it hasn’t been initialized yet.
Apr 10, 2017 - Connect and share knowledge within a single location that is structured and easy to search. ... Is there any check if a value is not null and not empty string in Javascript? I'm using the following one: Arunkumar Gudelli. I am One among a million Software engineers of India. I write beautiful markup.I make the Web useful. The value null is written with a literal: null.null is not an identifier for a property of the global object, like undefined can be. Instead, null expresses a lack of identification, indicating that a variable points to no object.In APIs, null is often retrieved in a place where an object can be expected but no object is relevant.
Since 2015, JavaScript has supported default values that get filled in when you don't supply a value for the argument or property in question. Those defaults don't work for null values. That is... May 05, 2020 - Write a function that checks whether a person can watch an MA15+ rated movie javascript One of the following two conditions is required for admittance: checkbox Type 'Event' is not assignable to type 'boolean'. Nov 01, 2018 - With default parameters, undefined ... while null does not. ... Thanks to Tim Branyen for the code inspiration. ... Thanks for reading! If you’re ready to finally learn Web Development, check out: The Ultimate Guide to Learning Full Stack Web Development in 6 months. If you’re working towards becoming a better JavaScript Developer, ...
Sep 29, 2020 - To check if a value is not null, you use the strict inequality operator (!==): ... JavaScript null has the following features. javascript check if not null . javascript by Rey on May 05 2020 Comment . 6. Add a Grepper Answer . Javascript answers related to "check if not null js" check if variable is undefined or null jquery; javascript check for null variables; javascript check if is nan; JavaScript check if is Nan; javascript check if null ... In javascript, we can check if an object is empty or not by using. JSON.stringify. Object.keys (ECMA 5+) Object.entries (ECMA 7+) And if you are using any third party libraries like jquery, lodash, Underscore etc you can use their existing methods for checking javascript empty object.
If the array has elements in it, the code within the if block will not run. Here's the third way to check whether or not an array is empty using .length. .length example three. By combining the use of the length property and the logical "not" operator in JavaScript, the "!" symbol, we can check if an array is empty or not. Check if JavaScript array is empty, null or undefined in 4 ways June 21, 2020 It is very easy to check if JavaScript array or object is empty but might need additional checks if you want to also check for null or undefined. Null and undefined can be treated in the same way if (typeof data === "undefined") will return true if the data is undefined (or null), so if (typeof data !== "undefined") will return true if the data has been defined (so is not null), then you can check if it is an empty string,
Jan 07, 2021 - There’s a long-standing bug in JavaScript meaning that every check for an object requires a check for null: typeof null === "object". ... null using the triple equality operator (=== or !==), also known as the strict equality operator, to be sure that the value in question is definitely not null: ... Dec 06, 2010 - I know that below are the two ways in JavaScript to check whether a variable is not null, but I’m confused which is the best practice to use. ... Possible duplicate of How do you check for an empty string in JavaScript? – T.Todua Dec 4 '18 at 9:32 input can not be null javascript; javascript get input value not null; JavaScript empty field; js if empty validation; check is not empty in js; javascript check empty input; javascript validation for blank text field; check field number and not empty javascript; javascript check if input field is null; empty input check in js; check empty field js
Turns out typoef null being 'object' was a mistake in the early JavaScript implementation. Do not use typeof operator to detect a null value. As mentioned previously, use the strict equality operator myVar === null. If you'd like to check whether a variable contains an object using typeof operator, you have to check againts null too: Answer: Use the equality operator ( ==) In JavaScript if a variable has been declared, but has not been assigned a value, is automatically assigned the value undefined. Therefore, if you try to display the value of such variable, the word "undefined" will be displayed. Whereas, the null is a special assignment value, which can be assigned to a ...
Typeerror Document Getelementbyid Is Null
Python Pandas Isnull And Notnull Geeksforgeeks
How To Check If A String Is Empty Undefined Null In Javascript
Javascript Check If Empty Code Example
Show 0 If Value Has No Data Tableau Edureka Community
How To Check For An Undefined Or Null Variable In Javascript
How To Check Null In Java With Pictures Wikihow
How To Check An Object Is Empty Using Javascript Geeksforgeeks
Why Is Null An Object And What S The Difference Between Null
How To Check For Null In Javascript By Dr Derek Austin
How Can I Check For An Empty Undefined Null String In
Null Propagation Operator In Javascript
How To Replace A Value If Null Or Undefined In Javascript
Javascript String Null Or Empty Check
How To Check An Empty Undefined Null String In Javascript Quora
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
Javascript Null Undefined And Nan Example
How To Check Empty Undefined Null String In Javascript
A Brief History Of Null And Undefined In Javascript By
Mysql Is Null Amp Is Not Null Tutorial With Examples
Javascript Lesson 4 Null And Undefined Type In Javascript
How To Check For An Object In Javascript Object Null Check
Handling Null In T Sql Codeproject
Ms Excel How To Use The Isblank Function Ws
Javarevisited Jsp How To Check If Arraylist Is Empty Using
Null And Undefined In Javascript Understanding Different
How To Check For Null In Javascript By Dr Derek Austin
0 Response to "30 How To Check Not Null In Javascript"
Post a Comment