34 Javascript Rotate Array 90 Degrees
Javascript rotate array 90 degrees Rotate an 2D matrix 90 degree clockwise without create another, Rotate a 2D array (n x n matrix) 90 degree close-wise without creating a new array. Q. Program to left rotate the elements of an array. Explanation. In this program, we need to rotate the elements of an array towards the left by the specified number of times. In the left rotation, each element of the array will be shifted to its left by one position and the first element of the array will be added to end of the list.
Measuring Size Of Objects In An Image With Opencv Pyimagesearch
To rotate 90 degrees clockwise, we need to first transpose and then reverse our matrix, which is how we got the one-line rotate function at the top of this section.
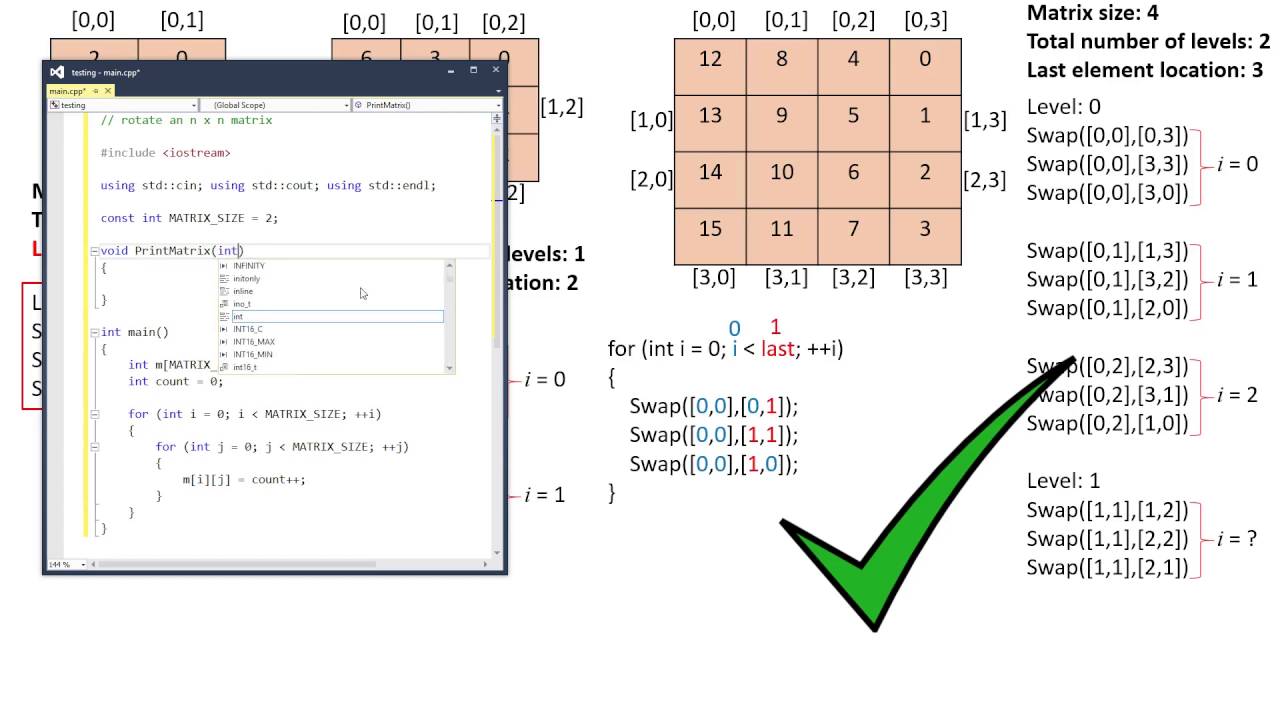
Javascript rotate array 90 degrees. lets say you got array 11 12 21 22 then you could convert that to 21 11 22 12 or 22 21 12 11 or 12 22 11 21 by rotations with multiples of 90 degrees and get as result another 2d array of the same dimensions. All other rotations could not give an ... I needed to rotate a non-square matrix in my program to transpose some spreadsheet data and decided to make a function to rotate 90, 180, or 270 degrees. It doesn't rotate the matrix in place, but instead generates a copy and returns that. I want to go for processing efficiency, let me know what I can do better: 2d-array-rotation. A collection of functions to rotate the values within a given two-dimensional array (i.e. an Array of Arrays) clockwise by 90, 180 or 270 degrees.Potentially useful for -- say -- manipulating game pieces or sprites, among other possible applications.
Now, we can place each element in its original location and shift all the elements around it to adjust as that would be too costly and most likely will time out on larger input arrays. Show Hint 3 One line of thought is based on reversing the array (or parts of it) to obtain the desired result. 2-Dimensional Matrix Rotation in JavaScript. Gaurav Subedi. Jul 11, 2020 · 3 min read. Photo by Markus Spiske on Unsplash. Recently, I came across a problem to rotate a 2 dimensional matrix 90 ... Add to List You are given an n x n 2D matrix representing an image, rotate the image by 90 degrees (clockwise). You have to rotate the image in-place, which means you have to modify the input 2D matrix directly. DO NOT allocate another 2D matrix and do the rotation.
It seems an easy task but it's not… and around the web you can only find examples building a new array, but I wanted to transform the array itself. So I am sharing you these two functions to rotate by 90 degrees clockwise and counter clockwise any two dimensional array with the same number of rows and columns. Definition and Usage. The rotate () method rotates the current drawing. Note: The rotation will only affect drawings made AFTER the rotation is done. JavaScript syntax: context .rotate ( angle ); The steps are: Rotate matrix by 90 degrees Reverse order of entire matrix, flip upside down. Bottom row is now on top and so on.
Approach: The approach is similar to Inplace rotate square matrix by 90 degrees | Set 1. The only thing that is different is to print the elements of the cycle in a clockwise direction i.e. An N x N matrix will have floor (N/2) square cycles. For example, a 3 X 3 matrix will have 1 cycle. Array.from() has an optional parameter mapFn, which allows you to execute a map() function on each element of the array being created. More clearly, Array.from(obj, mapFn, thisArg The rot90() function is used to rotate an array by 90 degrees in the plane specified by axes. Rotation direction is from the first towards the second axis. To rotate by 90 degrees any of the units can be used with their corresponding values. 90 degrees would equal to 100 gradient or 0.25 turns. Applying this property to the required element would rotate it by 90 degrees. Rotate an N × N matrix 90 degrees clockwise, Rotate an N × N matrix 90 degrees clockwise.
Rotations of 90, -90 and 180 degrees are simple transformations which can be performed as long as you know how many rows and columns are in your 2D array; To rotate any vector by 90 degrees, swap the axes and negate the Y axis. For -90 degree, swap the axes and negate the X axis. For 180 degrees, negate both axes without swapping. To rotate a matrix we will follow the steps of how we would rotate a square plane. There is N/2 squares or cycles in a matrix of size N. Process a square one at a time. Run a loop to traverse the matrix a cycle at a time, i.e loop from 0 to N/2 - 1. Traverse half the size of the matrix and in each loop rotate the element by updating them in ... The rotate () is a method of the 2D drawing context. The rotate () method allows you to rotate a drawing object on the canvas. Here is the syntax of the rotate () method: The rotate () method accepts a rotation angle in radians. If the angle is positive, the rotation is clockwise. In case the angle is negative, the rotation is counterclockwise.
numpy.rot90 () function The rot90 () function is used to rotate an array by 90 degrees in the plane specified by axes. Rotation direction is from the first towards the second axis. Hello, i'm struggling to find an algorithm that will rotate a matrix (multidimensional array) 90 degrees clockwise. I cant use any functions (transcope etc), Basically i need to write the code on my own. Any tips? ... Rotate a 3 * 3 matrix 90 degrees with one click with javascript. Rotate image 90 degree in picture box. Given a square matrix, turn it by 90 degrees in anti-clockwise direction without using any extra space.
Rotate the image by 90 degrees (clockwise). You have to rotate the image in-place, which means you have to modify the input 2D matrix directly. DO NOT allocate another 2D matrix and do the rotation. For example, if you were given the 2D array Rotate the elements in an array in JavaScript, Type-safe, generic version which mutates the array: Array.prototype.rotate = ( function () { // save references to array functions to make lookup faster var push Rotate an array of n elements to the right by k steps. For example, with n = 7 and k = 3, the array [1,2,3,4,5,6,7] is rotated to [5,6,7 ... 1/3/2013 · Rotating a two dimensional m x n matrix. Those looking for Rotating a two dimensional matrix (a more general case) here is how to do it. example: Original Matrix: [ [1,2,3], [4,5,6], [7,8,9] ] Rotated at 90 degrees: [ [7,4,1] [8,5,2] [9,6,3] ] This is done in following way:
A rotation by 90 degrees can be accomplished by two reflections at a 45 degree angle so if you take the transpose of the matrix and then multiply it by the permutation matrix with all ones on the minor diagonal and all zeros everywhere else you will get a clockwise rotation by 90 degrees. For my example here, I'll use the rotate() method with the transform property inside my JavaScript code to rotate an image. The method rotate() takes a parameter in form of an angle, like, 90deg or 180deg etc (no spaces between 90deg). The deg denotes the degree. The value with the method rotate() will rotate an element in clock wise. The above solution to How to Rotate a 2D Matrix by 90 Degrees in Java simply uses the same formula (i.e. the item at [i] [j] will simply go at item [j] [M-i-1]), but for all 4 corners of the square at once, to simply do the rotation in place.
To rotate the image, you can select the element using document.querySelector ('#img') and then append the.style.transform property to the element. The rotate property accepts the circular angle parameter measured in 360 degrees. The following JavaScript code will rotate the image by 90 degrees: Javascript rotate 2d array. Rotate Matrix Rotating An Array Using C Articles And Information On C Javascript 2d Array Code Example How To Rotate A Two Dimensional Array By 90 Degrees Clockwise How To Animate Following The Mouse In Jquery How To Animate Working With Matrices Apple Developer Documentation C++ Program Rotate the given image by 90 degrees Image : An image can be represented as a 2D matrix which can be stored in a buffer. So, the matrix contains it's base address.
Given an image represented by an NxN matrix, where each pixel in the image is 4 bytes, write a method to rotate the image by 90 degrees clockwise. For simplicity I assumed a matrix of strings for my example so [ ["a", "a", "a", "a"], ["b", "b", "b", "b"], ["c", "c", "c", "c"], ["d", "d", "d", "d"]]
Rotate Matrix Clockwise Python Javascript Es6 58 Bytes
Unity Rotate Object By 90 Degree
Matrix Rotation In C By 90 Degree Clockwise Amp Anti Clockwise
Javascript Problem Solvers Rotate Image Matrix By Austin
Rotating Shapes Center 0 0 Video Khan Academy
Rotate An 2d Matrix 90 Degree Clockwise Without Create
2 Dimensional Matrix Rotation In Javascript By Gaurav
Bootstrap 90 Degree Text Code Example
Cracking The Top Amazon Coding Interview Questions
Not An Easy Algorithm Rotating An Array Three Ways Dev
Rotate Image Leet Code Solution Gyanblog
Matrix Math For The Web Web Apis Mdn
Let S Rotate A Matrix Clockwise Javascript Beginners By
Rotating The Display By 90 Degrees How To Lvgl Forum
How To Calculate Rotation Angle From Rectangle Points
Image Rotation Augmentation Keras Imagedatagenerator
How To Rotate Videos In Premiere Pro In 3 Easy Steps Motion
Javascript Rotate 2d Matrix 90 Degrees Clockwise Top
Interview Questions How To Rotate A Matrix In Javascript
Transposing And Reversing How To Rotate A 2d Matrix 90
Rotate An 2d Matrix 90 Degree Clockwise Without Create
Code Less Do More With Webgl Libraries Ibm Developer
Moving Rotating And Scaling Drawing And Transforming
Javascript Problem Solvers Rotate Image Matrix By Austin
Solved Rotate Split Polylines Python Arcpy Esri Community
180 Degree Rotation Transformation Matrix
3d Rotations And Euler Angles In Python Meccanismo Complesso
How To Rotate A Text Box Review And Comment
Rotation In R3 Around The X Axis Video Khan Academy
Github Graemeboy Matrix Rotate Rotate A 2d Javascript
0 Response to "34 Javascript Rotate Array 90 Degrees"
Post a Comment