32 Javascript Assign Function To Variable
To demonstrate JavaScript's Date, let's create a variable and assign the current date to it. This article is being written on Wednesday, October 18th in London (GMT), so that is the current date, time, and timezone that is represented below. Jul 22, 2013 - I'm not a Javascript expert, so take this with a grain of salt. I think in some cases people might do it for style and same thing could be achieved by simply writing "function sum() {...}" However, assigning a function to a variable is a very powerful technique in functional programming.
Extract Functions Arguments Using Destructure In Javascript
Jul 20, 2021 - There is a distinction between the function name and the variable the function is assigned to. The function name cannot be changed, while the variable the function is assigned to can be reassigned. The function name can be used only within the function's body.
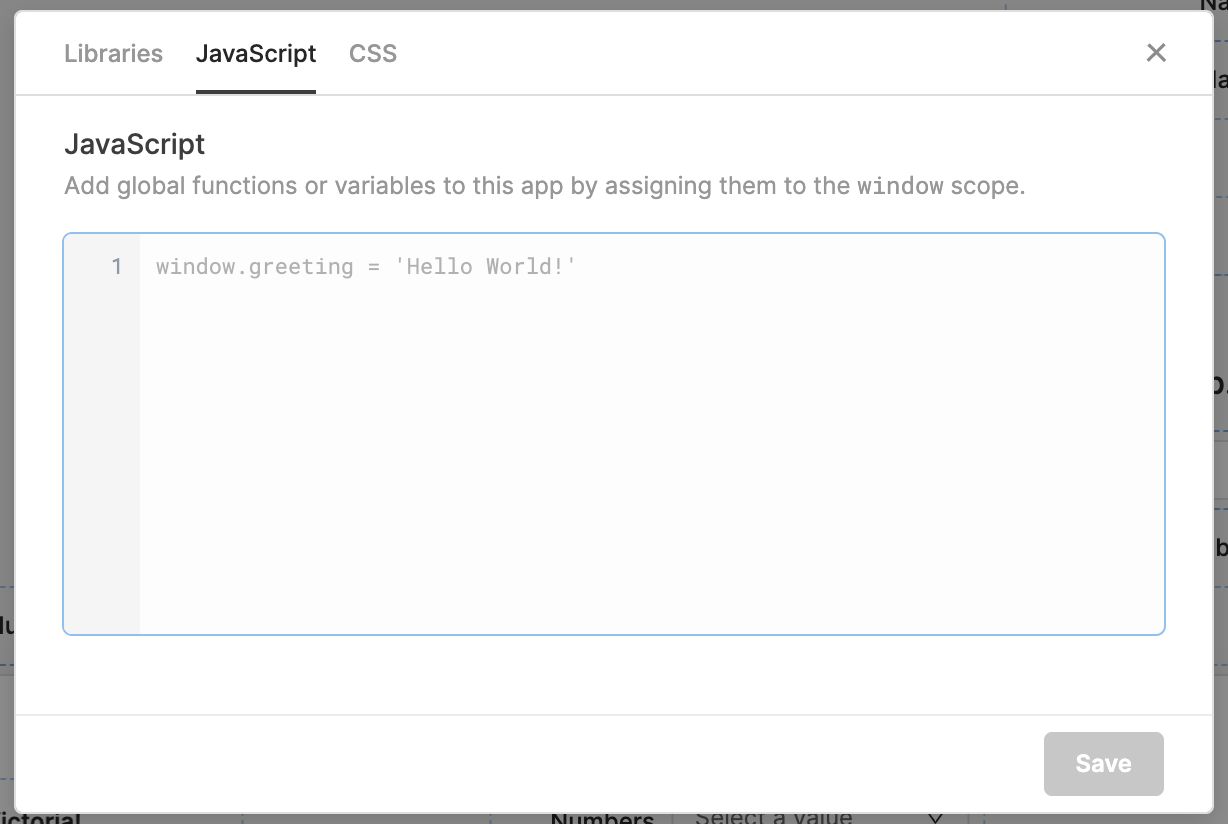
Javascript assign function to variable. The new local variable does not modify the global variable, but if we want to access the global value from within the function we'd need to use the global window object. For me, this code would be much easier to read if we'd used a global namespace for our global variable and rewrite our function to use block scope:- In Python, we can assign a function to a variable. And using that variable we can call the function as many as times we want. Thereby, increasing code reusability. Simply assign a function to the desired variable but without () i.e. just with the name of the function. If the variable is assigned with function along with the brackets (), None ... A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses ()
In JavaScript, a variable can be declared either in the global scope or the local scope. Global Variables. Variables declared out of any function are called global variables. They can be accessed anywhere in the JavaScript code, even inside any function. Local Variables. Variables declared inside the function are called local variables to that ... I am trying to assign an ajax response to a variable. How would I go about doing this? @Swoodend is right. You don't want to do this. It's a horrible idea, and you're having a hard time getting it to work because it's the worst way to work with AJAX requests. All JavaScript variables must be identified with unique names. These unique names are called identifiers. Identifiers can be short names (like x and y) or more descriptive names (age, sum, totalVolume). The general rules for constructing names for variables (unique identifiers) are: Names can contain letters, digits, underscores, and dollar signs.
You don't need to assign a function reference to a variable in order to invoke it. This example, building off example 5, will call getHashingFunction and then immediately invoke the returned function and pass its return value to hashedValue. // Example 6 var hashedValue = getHashingFunction ( 'sha1' ) ( 'Fred' ); JavaScript has first-class functions, that is, create a function and assign it to a variable just like you create a string or number and assign it to a variable. Here, the fn variable is assigned to a function. The reason for this concept is functions are objects in JavaScript; fn is pointing ... Mar 13, 2017 - To assign a function to a variable you have to use just the name, such as: ... As a counter-example you invoke it in this next line of code and pass to g not the function be the result of its execution: ... Not the answer you're looking for? Browse other questions tagged javascript or ask your ...
Sep 24, 2017 - I know that in the code above, we passed a function to a variable. fn=baz, if I change it to fn=baz() IDE will give me a type error: fn is not a function. So I assume in js we do not assign functions to variables, we assign the output of the functions into a variable. A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses () ... When you run JavaScript in a Node.Js application, elements in a Node.JS Stack actually executes the JavaScript: ... Javascript Make your console talk! ... Learn how to use Js export ... Save returned value from a function to a variable · java2s | © Demo Source and Support. All rights reserved
Nov 23, 2011 - JavaScript has first-class functions, that is, create a function and assign it to a variable just like you create a string or number and assign it to a variable. Here, the fn variable is assigned to a function. The reason for this concept is functions are objects in JavaScript; fn is pointing ... Sep 16, 2020 - In the parsing phase, The JavaScript engine moves all variable declarations to the top of the file if the variables are global, or to the top of a function if the variables are declared in the function. In the execution phase, the JavaScript engine assigns values to variables and execute the code. This form of function is immutable, ... a new assignment at any time. To learn more about this, search, ‘function declaration vs function expression’ and keep an eye out for the term, ‘hoisting’ so you get an idea how that fits into this picture. Aside: name is reserved word in JavaScript and we cannot ...
So when you log or display the contents of the variable, the function has not yet run and the content has not yet been set. So even though JavaScript actually works with a single-thread model, you've landed in the asynchronous problem zone. Jul 20, 2021 - The var statement declares a function-scoped or globally-scoped variable, optionally initializing it to a value. ... Variable name. It can be any legal identifier. ... Initial value of the variable. It can be any legal expression. Default value is undefined. Alternatively, the Destructuring Assignment ... In JavaScript, you can assign a function in a variable using the concept of anonymous functions. Anonymous, as the name suggests, allows creating a function without any names identifier. It can be used as an argument to other functions. They are called using the variable name −
Here ID attribute is "Element1" with the text value "GetElementById1". Then variables are created to assign the getElementById() value but here some string text called "PROFITLOOPS" is created and assigned to replace the original text. Then with the help of document.write() function, the output of the s variable will be printed. In this example, the anonymous function has no name between the function keyword and parentheses (). Because we need to call the anonymous function later, we assign the function to the show variable. Using anonymous functions as arguments of other functions. We often use anonymous functions as arguments of other functions. For example: Among the Object constructor methods, there is a method Object.assign () which is used to copy the values and properties from one or more source objects to a target object. It invokes getters and setters since it uses both [ [Get]] on the source and [ [Set]] on the target. It returns the target object which has properties and values copied from ...
11/9/2020 · Use return statement to assign function to variables. Following is the code −Example Live Demo<!DOCTYPE html> <html lang=en> <head> <meta charset=UTF-8> ... × 2 days ago - Before ES2015, JavaScript variables were solely declared using the var keyword. ... The above code corresponds to variable x, variable y, and variable y. We are declaring these variables. Notice we are not assigning a value to these variables just yet. We are only saying they should exist. A function is an object type in ... name you assign to the function becomes a case-sensitive identifier for that object. As a result, you cannot use a JavaScript-reserved keyword as a function name, nor should you use a function name that is also an identifier for one of your other global entities, such as variables or (in IE ...
Assignment (=) The simple assignment operator (=) is used to assign a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables When you assign a function object to another variable JavaScript does not create a new copy of the function. Instead it makes the new variable reference the same function object as original. It is just that two variables having different names are accessing the same underlying function object. Hoisting is JavaScript's default behavior of moving declarations to the top of the current scope. Hoisting applies to variable declarations and to function declarations. Because of this, JavaScript functions can be called before they are declared: myFunction ( 5 ); function myFunction (y) {. return y * y; }
In the code snippet below, the variable magneto is a compound value (Array object), thus it is assigned in variable (function argument) x with assign-by-reference. Inside factorial () a recursive call is being made using the variable that holds the function: factorial (n - 1). It is possible to use a function expression and assign it to a regular variable, e.g. const factorial = function (n) {...}. But the function declaration function factorial (n) is compact (no need for const and =). JavaScript Variable: Declare, Assign a Value with Example. By James Hartman. Updated on August 27, 2021. Variables are used to store values (name = "John") or expressions (sum = x + y). Declare Variables in JavaScript. Before using a variable, you first need to declare it. You have to use the keyword var to declare a variable like this:
Local variables are deleted when the function is completed. Global Scope: Global variables can be accessed from inside and outside the function. They are deleted when the browser window is closed but is available to other pages loaded on the same window. There are two ways to declare a variable globally: Declare a variable outside the functions ... 20/5/2016 · The declaration can be assigned to a variable, which can then be passed as a parameter to another function. Thus, a JavaScript Function is a JavaScript Variable until it is executed (evaluated). The Object.assign () method copies all enumerable own properties from one or more source objects to a target object. It returns the modified target object.
In other words, a function declaration declares a new variable, creates a function object, and assigns it to the variable. ... The constructor Function() evaluates JavaScript code stored in strings. For example, the following code is equivalent to the previous example: Can't Pass an Input Value Into a JavaScript Variable. Imagine the following scenario - you have a simple input and a button. When a user types into the input and presses the button, the text from the input should be logged to the console. Here's what you have so far: But when you load the page you see Uncaught ReferenceError: test is not ... Functions are one of the fundamental building blocks in JavaScript. A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output.
Variable Assignment. Variable assignment is one of the first things you learn in coding. For example, this is how we would assign the number 3 to the variable bears: const bears = 3; A common ... Variable assignment is a concept that many developers find confusing. In this post I will try to explain how JavaScript treats variable assignments and argument passing. Key to this is understanding the difference between primitive values and objects. In JavaScript there are five types of primitive values - undefined, null, boolean, string and ... A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). The parentheses may include parameter names separated by commas: (parameter1, parameter2, ...) The code to be executed, by the function ...
Working With Javascript In Visual Studio Code
Understanding Scope In Javascript Scotch Io
Javascript Nested Functions 4 Examples Of Javascript Nested
Quick Tip How To Declare Variables In Javascript Sitepoint
Apache Jmeter User S Manual Functions And Variables
Reput Replit Js Assignment 3 Introduction To Control Chegg Com
Chapter 6 Return Values Getting Data From Functions Get
Declaring A Variable Inside A Function In Javascript Stack
Javascript Variables A To Z Guide For A Newbie In
Assignment With A Returned Value Freecodecamp Basic Javascript
Chapter 16 Variables Scopes Environments And Closures
Preloaded Javascript And Libraries
Hoisting In Javascript Javascript S Another Beautiful Part
Var Functionname Function Vs Function Functionname
Storing The Information You Need Variables Learn Web
Ssis Expression Tasks Vs Evaluating Variables As Expressions
Understanding Variables Scope And Hoisting In Javascript
Javascript Assign Function To Variable Reference Value
Javascript Variables A To Z Guide For A Newbie In
Declaring A Variable Inside A Function In Javascript Stack
First Class Function In Javascript
4 Ways To Swap Variables In Javascript
Chapter 5 Arguments Passing Data To Functions Get
4 Ways To Convert String To Character Array In Javascript
Print All Variables Defined Javascript Code Example
Advanced Concepts In Javascript Higher Order Functions Hof
Mastering This In Javascript Callbacks And Bind Apply
How To Use Object Destructuring In Javascript
Function Hoisting Amp Hoisting Interview Questions
0 Response to "32 Javascript Assign Function To Variable"
Post a Comment