26 Get All Properties Of Object Javascript
Here, we will look at objects, properties and methods in JavaScript, touching on the prototype chain and "this". One of the best parts of JavaScript is the way it makes working with objects ... Summary: in this tutorial, you will learn about the JavaScript object's properties and their attributes such as configurable, enumerable, writable, get, set, and value. Object Property types. JavaScript specifies characteristics of properties of objects via internal attributes surrounded by the two pair of square brackets e.g., [[Enumerable ...
Javascript Array Distinct Ever Wanted To Get Distinct
Aug 20, 2020 - Get code examples like "javascript list all properties of object" instantly right from your google search results with the Grepper Chrome Extension.
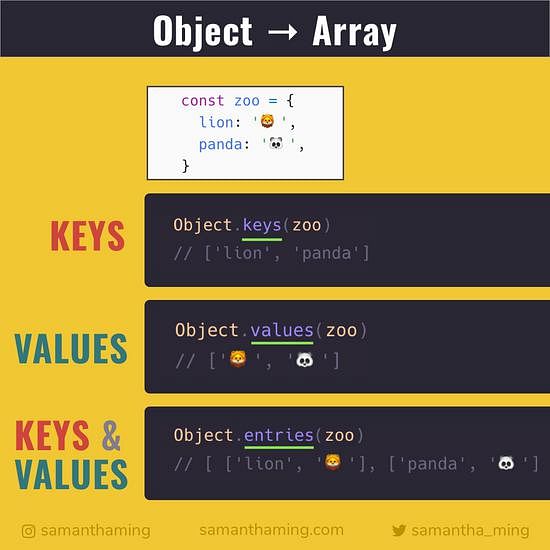
Get all properties of object javascript. To get the symbols of an object to enumerate over, use Object.getOwnPropertySymbols (this function is why Symbol can't be used to make private properties). The new Reflect API from ECMAScript 2015 provides Reflect.ownKeys , which returns a list of property names (including non-enumerable ones) and symbols. Depending on the object type, not all of a object's properties are displayed when you run the "Get-" cmdlet and display the output. If the output uses Format-Table , trying to squeeze all of the properties into the table could make the output noisy and unreadable, so it makes sense that some properties are excluded. In the HTML DOM, the Element object represents an HTML element, like P, DIV, A, TABLE, or any other HTML element. Properties and Methods The following properties and methods can be used on all HTML elements:
To get the symbols of an object to enumerate over, use Object.getOwnPropertySymbols (this function is why Symbol can't be used to make private properties). The new Reflect API from ECMAScript 2015 provides Reflect.ownKeys , which returns a list of property names (including non-enumerable ones) and symbols. Reflecting over JavaScript object properties, or more commonly object maps, is something that I always forget and have to look up over and over again, so I'm writing it down in hopes I can remember and have an easy place to look it up. Iterating over a JavaScript Object. JavaScript provides a bunch of good ways to access object properties. The dot property accessor syntax object.property works nicely when you know the variable ahead of time. When the property name is dynamic or is not a valid identifier, a better alternative is square brackets property accessor: object[propertyName].
Object.keys() returns an array whose elements are strings corresponding to the enumerable properties found directly upon object.The ordering of the properties is the same as that given by looping over the properties of the object manually. 1 week ago - All objects in JavaScript inherit from at least one other object. The object being inherited from is known as the prototype, and the inherited properties can be found in the prototype object of the constructor. See Inheritance and the prototype chain for more information. The attributes collection is iterable and has all the attributes of the element (standard and non-standard) as objects with name and value properties. Property-attribute synchronization. When a standard attribute changes, the corresponding property is auto-updated, and (with some exceptions) vice versa.
That's all for iterating over object properties in JavaScript. We looked at four different methods to accomplish this task. for...in is still a good option if you want to support old browsers. Otherwise, just use any of the latest methods: Object.keys(), Object.values() or Object.entries(). ️ Like this article? Follow me on Twitter and LinkedIn. we can print all enumerable properties either own or inherited of cat object using javascript for..in loop. 3. 1. for (var prop in cat) {. 2. console.log(prop); 3. } using the for..in loop, all ... To get all own properties of an object in JavaScript, you can use the Object.getOwnPropertyNames () method. This method returns an array containing all the names of the enumerable and non-enumerable own properties found directly on the object passed in as an argument. The Object.getOwnPropertyNames () method does not look for the inherited ...
See the Pen javascript-object-exercise-1 by w3resource (@w3resource) on CodePen. Contribute your code and comments through Disqus. Previous: javascript Object Eexercises. Next: Write a JavaScript program to delete the rollno property from the following object. Also print the object before or after deleting the property. Nearly all objects in JavaScript are instances of Object; a typical object inherits properties (including methods) from Object.prototype, although these properties may be shadowed (a.k.a. overridden). However, an Object may be deliberately created for which this is not true (e.g. by ... Object.keys() is used for returning enumerable properties of an array like object with random key ordering. Syntax: Object.keys(obj) Parameters Used: obj : It is the object whose enumerable properties are to be returned. Return Value: Object.keys() returns an array of strings that represent all the enumerable properties of the given object.
JavaScript | Object Properties. Object properties are defined as a simple association between name and value. All properties have a name and value is one of the attributes linked with the property, which defines the access granted to the property. Properties refer to the collection of values which are associated with the JavaScript object. Kinds of properties JavaScript has three different kinds of properties: named data properties, named accessor properties and internal properties. Named data properties ("properties") "Normal" properties of objects map string names to values. For example, the following object obj has a data property whose name is the string "prop" and ... Sep 29, 2020 - Have you ever wanted to check what properties and methods are available for a particular HTML element or a Javascript object? The dir() method displays an interactive list of the properties and…
Jan 21, 2021 - Summary: in this tutorial, you will learn about the JavaScript object’s properties and their attributes such as configurable, enumerable, writable, get, set, and value. ... JavaScript specifies characteristics of properties of objects via internal attributes surrounded by the two pair of ... To detect all the property values of object without knowing the key can be done in a number of ways depending on browsers. The majority of browsers support ECMAScript 5 (ES5). Let's see what methods can be used for getting the property value based on different specifications. In the above program, the property value of key a is extracted from each object of an array. Initially, the extractedValue array is empty. The for loop is used to iterate through all the elements of an array. During each iteration, the value of property a is pushed to the extractedValue array.
With this notation, you'll never run into Cannot read property 'name' of undefined.You basically check if user exists, if not, you create an empty object on the fly. This way, the next level key will always be accessed from an object that exists or an empty object, but never from undefined.. Unfortunately, you cannot access nested arrays with this trick The Object.keys() method returns an array of a given object's own enumerable property names, iterated in the same order that a normal loop would. Jul 27, 2021 - Just like a for..in loop, these methods ignore properties that use Symbol(...) as keys. Usually that’s convenient. But if we want symbolic keys too, then there’s a separate method Object.getOwnPropertySymbols that returns an array of only symbolic keys. Also, there exist a method Reflect.ownKeys(obj) that returns all ...
All JavaScript dictionaries are objects, so getting the properties of a JavaScript object is the same as getting the keys of a JavaScript dictionary. There are a few ways to get the keys of an ... JavaScript objects inherit the properties of their prototype. The delete keyword does not delete inherited properties, but if you delete a prototype property, it will affect all objects inherited from the prototype. ... Get certified by completing a course today! Here's a very common task: iterating over an object properties, in JavaScript. Published Nov 02, 2019, Last Updated Apr 05, 2020. If you have an object, you can't just iterate it using map(), forEach() or a for..of loop. You will get errors:
Object.keys(hero) returns the list ['name', 'city'], which, as expected, are the keys of hero object. 1.1 Keys in practice: detect if object is empty. If you'd like to quickly check if an object is empty (has no own properties), then a good approach is to check whether the keys list is empty. JavaScript object is a standalone entity that holds multiple values in terms of properties and methods. Object property stores a literal value and method represents function. An object can be created using object literal or object constructor syntax. Object literal: var person = { firstName: "James", lastName: "Bond", age: 25, getFullName ... 3 weeks ago - A protip by steveniseki about jquery and javascript.
This means that PHP only needs ... does the property-name to offset mapping and uses a memory-efficient C-array in the individual objects." However, if you call `get_object_vars()` on an object like this, then PHP WILL build a hashtable for the individual object. If you have a large quantity of objects, and you call `get_object_vars()` on all of them, then ... Aug 25, 2020 - A quick guide to learn how to get all own properties of an object in JavaScript. Object.getOwnPropertyNames() returns an array whose elements are strings corresponding to the enumerable and non-enumerable properties found directly in a given object obj.The ordering of the enumerable properties in the array is consistent with the ordering exposed by a for...in loop (or by Object.keys()) over the properties of the object.According to ES6, the integer keys of the object (both ...
The Array.prototype.filter () method returns a new array with all elements that satisfy the condition in the provided callback function. Therefore, you can use this method to filter an array of objects by a specific property's value, for example, in the following way: If a match is not found then the Array.prototype.filter () method will return ... We can use the Object.getOwnPropertyNames() function to get all the property names linked to an object.. Then we can filter the resulting array, to only include that property name if it's a function. We determine if it's a function by using typeof on it.. For example here is how we might create a utility function to do what we need: The Object.entries() method returns an array of a given object's own enumerable string-keyed property [key, value] pairs. This is the same as iterating with a for...in loop, except that a for...in loop enumerates properties in the prototype chain as well).
The property order is the same as in the case of looping over the properties of the object manually. The hasOwnProperty() Method¶ The hasOwnProperty() method returns a boolean value that indicates if the object has the specified property as its own property or not. If the object contains the "key" property, a function is created.
Console Utilities Api Reference Chrome Developers
Java Properties File How To Read Config Properties Values In
Object In Javascript Free Software Tutorials
Properties The Java Tutorials Gt Essential Java Classes
Get Aduser Powershell Command Tutorial To List Active
3 Ways To Clone Objects In Javascript Samanthaming Com
How To Access Object Properties In Javascript In Three Ways
Hiding Properties In Javascript Sometimes You Want To
Diving Deeper In Javascripts Objects By Arfat Salman Bits
How To Get All Property Values Of A Javascript Object
Javascript Fundamental Es6 Syntax Iterate Over All Own
Javascript Object Retrieve All The Values Of An Object S
Javascript For Each Property In Object Property Walls
Everything About Javascript Objects By Deepak Gupta
How Can I Remove Properties From Javascript Objects O Reilly
Different Ways To Search For Objects In Sql Databases
Object Create In Javascript The Object Create Method Is One
Converting Object To An Array Samanthaming Com
Javascript Object Get All Properties With Same Value Code Example
Best Trick To Get All Properties Of Any Javascript Object
How Can I Display A Javascript Object Stack Overflow
0 Response to "26 Get All Properties Of Object Javascript"
Post a Comment