32 Javascript Post Form Data To Api
In the following example, we use the FileReader API to access binary data and then build the multi-part form data request by hand: < form id = " theForm " > < p > < label for = " theText " > text data: </ label > < input id = " theText " name = " myText " value = " Some text data " type = " text " > </ p > < p > < label for = " theFile " > file data: </ label > < input id = " theFile " name = " myFile " type = " file " > </ p > < button > Send Me! </ button > </ form > To follow along, create a file named form.js in the same folder as the HTML form. Let's see. Grabbing form fields from the event target. First of all we register an event listener for the submit event on the form to stop the default behaviour (they send data to the back-end).
How To Get Form Data On Submit In Reactjs
To send data to the REST API server using JavaScript/AJAX, you must send an HTTP POST request and include the POST data in the request's body. You also need to provide the Content-Type: application/json and Content-Length request headers. Below is an example of a REST API POST request to a ReqBin REST API endpoint.
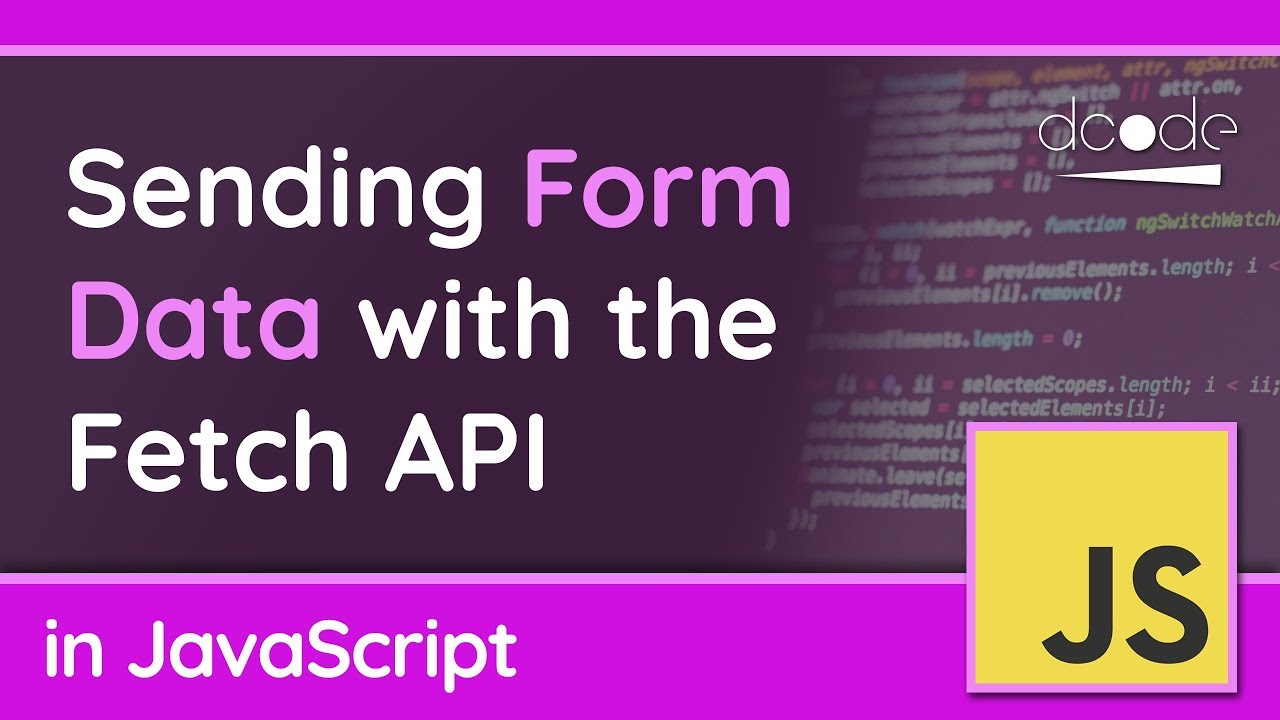
Javascript post form data to api. Sep 14, 2020 - This blog post was inspired by a question that I received from one of my new subscribers, Stéphanie. She had built a static front end with Eleventy, and an API with Express and a MongoDB database. Stéphanie wanted to know how she could have the JavaScript in her front end send data from a form on ... 19/7/2021 · To send a POST request using vanilla JavaScript, you must use an XMLHttpRequest object to interact with the server and provide the correct Content-Type request header for the POST message body data. In this JavaScript POST request example, the Content-Type: application/json header indicates that the body of the POST message contains JSON, and the Accept: application/json … formData.append (name, value) - add a form field with the given name and value, formData.append (name, blob, fileName) - add a field as if it were <input type="file">, the third argument fileName sets file name (not form field name), as it were a name of the file in user's filesystem,
Mar 23, 2020 - I just completely redesigned my APIs & JavaScript pocket guide with modern ES6 approaches, including Promises and fetch(). This is an excerpt. (The examples below use the JSON Placeholder API.) Specifying the HTTP method with fetch() The fetch() method accepts an optional second argument: an ... Note: For this form, I have not implemented client side validation. Here, I am reading the form values, using jQuery.and posting form values to Web API for saving on the database. The output of the Application is: After saving the data on the Server, you will get a message like: Download the source code of this Application here.. 21/8/2019 · Using Fetch to Post Data. JavaScript Fetch API provides a simple interface for fetching resources. It is the newest standard for handling network requests in the browser. The biggest advantage of Fetch over XMLHttpRequest (XHR) is that the former uses promises that make working with requests and responses far easier.
23 Apr 2021 — An HTML form on a web page is nothing more than a convenient user-friendly way to configure an HTTP request to send data to a server. This ...On the server side: retrieving the data · special case: sending files · Security issues XMLHttpRequest () is a JavaScript function that made it possible to fetch data from APIs that returned XML data. XMLHttpRequest gave us the option to fetch XML data from the backend without reloading the entire page. This function has grown from its initial days of being XML only. Now it supports other data formats like JSON and plaintext. It is commonly used to send data from or to server. Nowadays it is widely used in API integration because of its advantages and simplicity. In this example we are going to use AJAX (Asynchronus JavaScript And XML), to send data in background. We are using PHP for the backend.
It's a common task for JavaScript developers to send GET and POST requests to retrieve or submit data. There are libraries like Axios that help you do that with beautiful syntax. However, you can achieve the same result with a very similar syntax with Fetch API, which is supported in all modern browsers. Table of Contents hide Your form data was being appended to the POSTrequest, but it was being appended in a format that reqres.inignores. It seems reqres.inonly processes a request body if it's a valid JSON string. When you send a request with fetch that has a body set to new FormData(), your browser adds the Content-Type: multipart/form-dataheader to the request. version added: 1.0 jQuery.post ( url [, data ] [, success ] [, dataType ] ) A string containing the URL to which the request is sent. A plain object or string that is sent to the server with the request. A callback function that is executed if the request succeeds.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Jan 28, 2021 - The JavaScript Fetch API provides a utility to make AJAX requests. This post will show how ES6 syntax can be used with Typescript and the Fetch API to submit an HTML form. Using the Fetch API in conjunction with other Web API's a post request can be sent, containing FormData Objects in the ...
We should modify the javascript file to handle the newly added Save Draft button click and when a user click on this button we have to read the form data and post form data to the controller. So I added some jquery methods to post form data. First we serialize the form data and post form data to the controller as an Ajax post. custom script file Summary: in this tutorial, you will learn about JavaScript form API: accessing the form, getting values of the elements, validating form data, and submitting the form. Form basics. To create a form in HTML, you use the <form> element: < The first step is to attach an event handler the <form> to capture the submit event: const form = document.querySelector('#signup-form'); form.addEventListener('submit', () => { }); The next step is to create and send an actual POST request. If you are already familiar with jQuery, sending a POST request is quite similar to the $.post () method.
Available in Chrome 42+ | View on GitHub | Browse Samples · This sample shows how the Fetch API can make POST requests Just like its name suggests it's designed for holding forms data i.e you can use it with JavaScript to build an object that corresponds to an HTML form. It's mostly useful when you need to send form data to RESTful API endpoints, for example to upload single or multiple files using the XMLHttpRequest interface, the fetch() API or Axios. Handling JSON request bodies in an Express based API. If your API is built with Express you'll want to configure your routes to be able to accept JSON request bodies. You can use the body-parser middleware to handle this for you. It can be configured to parse the JSON request body for POST/PUT/PATCH requests and it will then add it as an object under a body property in the request object.
Feb 23, 2020 - A practical guide to learning how to use native JavaScript Fetch API to get and post data from a server. Jan 02, 2019 - FormData API: Provides a JavaScript interface to easily construct a set of key/value pairs representing form fields and their values, which can then be easily sent using ajax. It uses the same format a form would use if the encoding type were set to “multipart/form-data". In this video we look at sending form data (POST) with the Fetch API. Fetch makes it super easy to do this and is great for making asynchronous, mobile frien...
Apr 17, 2018 - I´m currently new into this whole API and JavaScript thing. I need some help as i am googling this issue the whole day. I am trying to link a html form and a JS button to salesforce via an API. That's what our sample API expects when receiving data, but it may differ in your APIs. Finally when doing .send(), make sure to include the form data there so it gets sent to the API. Sending JSON data with vanilla JavaScript. Sending JSON data just means sending a string, and telling the API what the string represents. The main change in the front-end is to remove the default POST behavior and replace it with a JavaScript handler that will submit the post data asynchronously and update the user interface.
The valid methods are: An HTTP Request consists of five parts. REST API in JavaScript. GET for getting data from the server. POST for creating data on the server. PUT for updating data on the server. PATCH for partially updating data on the server. DELETE for deleting data on the server. 2. May 15, 2020 - Let’s say you have a form. When it’s submitted, you want to get the values of all of the fields and submit them to an API. How do you easily get the values of all of the fields? Today, we’re going to look at an easy, native way to do that: FormData(). Obj of data to send in future like a dummyDb const data = { username: 'example' }; //POST request with body equal on data in JSON format ...
"Oh yeah, man. Alright, come on. Looks good to me." I recently performed a technical exercise wherein I had to upload files to a particular platform using their API.You often learn a lot when ... 15/6/2012 · HTML forms use either GET or POST to send data to the server. The method attribute of the form element gives the HTTP method: <form action="api/values" method="post"> The default method is GET. If the form uses GET, the form data is encoded in the URI as a query string. If the form uses POST, the form data is placed in the request body. Warning: When using FormData to submit POST requests using XMLHttpRequest or the Fetch_API with the multipart/form-data Content-Type (e.g. when uploading Files and Blobs to the server), do not explicitly set the Content-Type header on the request. Doing so will prevent the browser from being able to set the Content-Type header with the boundary expression it will use to delimit form fields in ...
For each of these actions, JAAS API provides a corresponding endpoint. Browse APIs In order to demonstrate the entire CRUD functionality in JavaScript, we will complete the following steps: Make a POST request for the API used to create the object. We will save object id which was received in the answer. 31 answersDynamically create <input> s in a form and submit it. /** * sends a request to the specified url from a form. this will change the window location. The Fetch API provides a JavaScript interface for accessing and manipulating parts of the HTTP pipeline, such as requests and responses. It also provides a global fetch() method that provides an easy, logical way to fetch resources asynchronously across the network.
22 Aug 2021 — The Fetch API provides a JavaScript interface for accessing and ... with a value of application/x-www-form-urlencoded , multipart/form-data ... Nov 09, 2017 - Browse other questions tagged javascript ajax fetch-api or ask your own question. ... React native 422 Unprocessable Entity error returned from server when using POST Fetch call with content type: application/x-www-form-urlencoded ... Why is any(True for ... if cond) much faster than any(cond for ...)? How to ... In this lesson you can learn post json data in React Js to API with the help of an example. API has created in CodeIgniter and MySQL. Bootstrap also installed in this tutorial. You can learn how to Integrate Bootstrap with React Js. Create src/AddProduct.js file and create stateful component AddProduct .
Jun 22, 2021 - The server reads form data and the file, as if it were a regular form submission. ... FormData objects are used to capture HTML form and submit it using fetch or another network method. We can either create new FormData(form) from an HTML form, or create an object without a form at all, and then append ... Since this is not an article on APIs, I will put the discussion to "REST" and will come right on the topic which is how Node.JS server handles user data using GET or POST methods. GET and POST Methods - Transfer Form Data to Server. The two most widely used methods to transfer user input data to the server are GET and POST methods.
Javascript Fetch Api Tutorial With Js Fetch Post And Header
Accepting Raw Request Body Content In Asp Net Core Api
Get And Post Requests Using Python Geeksforgeeks
Node Js Rest Api To Add Edit And Delete Record From Mysql
How To Handle The Post Request Body In Node Js Without Using
React Js How To Post Form Data To Api With React Js Youtube
Sending Html Form Data In Asp Net Web Api Form Urlencoded
How To Send Formdata Objects With Ajax Requests In Jquery
Post Form Data To Api Using Axios In Vuejs 5 Balloons
Read Html Form Data Using Get And Post Method In Node Js By
Simple Custom Post Request Datatables Forums
How To Submit Ajax Forms With Jquery Digitalocean
Save Web Form Data To Spreadsheets And Google Sheets
Fetch Api Post Form Data Javascript Code Example
Rest Up Consuming A Multipart Form Data Rest Method With
React File Upload Proper And Easy Way With Nodejs
Sending Form Data Post With The Fetch Api In Javascript
Building Requests Postman Learning Center
Tools Qa How To Make A Post Request In Postman
Using Fetch To Post Json Data Ultimate Courses
How To Post Upload Formdata Multipart Form Data With
How To Post Data When You Have Javascript Object Using
Sending An Array As Form Data Help Postman
Building Requests Postman Learning Center
Building Requests Postman Learning Center
How To Post A Multi Part Form Data From Android To Web Api
Api Tutorial For Beginners With Google Sheets And Apps Script
Forms Without Servers Handling Form Submissions With Lambda
Javascript Send Http Get Post Request And Read Json Response
0 Response to "32 Javascript Post Form Data To Api"
Post a Comment