28 Javascript Array Of Class Objects
Please note that arr also belongs to the Object class. That's because Array prototypically inherits from Object.. Normally, instanceof examines the prototype chain for the check. We can also set a custom logic in the static method Symbol.hasInstance.. The algorithm of obj instanceof Class works roughly as follows:. If there's a static method Symbol.hasInstance, then just call it: Class ... An Array of Objects is created using the Object class, and we know Object class is the root class of all Classes. We use the Class_Name followed by a square bracket [] then object reference name to create an Array of Objects. Class_Name [ ] objectArrayReference; Alternatively, we can also declare an Array of Objects as :
Arrays of objects don't stay the same all the time. We almost always need to manipulate them. So let's take a look at how we can add objects to an already existing array. Add a new object at the start - Array.unshift. To add an object at the first position, use Array.unshift.
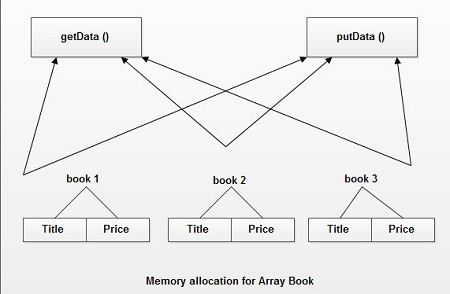
Javascript array of class objects. Feb 27, 2019 - In the above example, create a class Array which contains two properties i.e. length and data, where length will store the length of an array and data is an object which is used to store elements. Rules and Regulations for Implementing. TypeScript Array of Objects, most part of it can be used interchangeably; Inline Types can be used in aliasing much more complicated types and Interfaces are much inclined to basic type structure such as an object or a class, etc. Sep 18, 2018 - Also as a matter of good practises ... object, not push the object in an unrelated array. So yes, avoid unrelated side-effects in constructors – Gigi Sep 18 '18 at 1:13 ... Not the answer you're looking for? Browse other questions tagged javascript class object or ask your own ...
JavaScript is an object-based language based on prototypes, rather than being class-based. Because of this different basis, it can be less apparent how JavaScript allows you to create hierarchies of objects and to have inheritance of properties and their values. An array of objects is created using the 'Object' class. The following statement creates an Array of Objects. Class_name [] objArray; Alternatively, you can also declare an Array of Objects as shown below: Class_nameobjArray[]; Both the above declarations imply that objArray is an array of objects. JavaScript - The Arrays Object. The Array object lets you store multiple values in a single variable. It stores a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
Array class in JavaScript: class Array {. constructor () {. this.length = 0; this.data = {}; } } In the above example, create a class Array which contains two properties i.e. length and data, where length will store the length of an array and data is an object which is used to store elements. Function in Array: There are many functions in array ... Creates an array from an object: includes() Check if an array contains the specified element: indexOf() Search the array for an element and returns its position: isArray() Checks whether an object is an array: join() Joins all elements of an array into a string: keys() Returns a Array Iteration Object, containing the keys of the original array ... Also classes are usually title-cased. Plus as a side note, while it's real that you can change consts properties while maintaining the reference, so you can push objects in an array that's declared as const, it really doesn't add up as self-documenting code, so, if you are going to modify this array, just declare it with "let" from the start.
target is an array-like or iterable object to convert to an array. mapFn is the map function to call on every element of the array; thisArg is the this value when executing the mapFn function. The Array.from() returns a new instance of Array that contains all elements of the target object. JavaScript Array.from() method examples JavaScript lets you create objects and arrays. Objects are similar to classes. The objects are given a name, and then you define the object's properties and property values. An array is an object also, except arrays work with a specific number of values that you can iterate through. JavaScript group an array of objects by key. In this article, I will explain about the groupBy array of object in javascript. I will explain this using one example. Now I am going group using make. For example ' make:'audi' ' to 'audi: { […..]}'. We use to reduce function.
Nov 08, 2011 - For more information about arrays have a look at MDC - Array. ... just out of curiosity, when an array is declared like this, how do you reference it? – david99world May 12 '11 at 9:01 Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The JavaScript Object type has the Object.keys() ... Learn the building blocks of JavaScript programming language like data types, functions, objects, arrays and classes. Use the knowledge from the book to build a small but solid program. Learn more. Post navigation. Previous Post.
Now, let's see how pets_1 looks like as an object. Object is similar to the indexed array, it's often referred to as an Associative Array. So the above indexed array can be rewritten into an object as follows: let pets_2 = { 0 : "cat", 1 : "dog", 2 : "mouse" }; Notice the curly braces — that's the main distinction between an ... Sort an Array of Objects in JavaScript Summary : in this tutorial, you will learn how to sort an array of objects by the values of the object's properties. To sort an array of objects, you use the sort() method and provide a comparison function that determines the order of objects. JavaScript map with an array of objects. JavaScript map method is used to call a function on each element of an array to create a different array based on the outputs of the function. It creates a new array without modifying the elements of the original array.
What Is An Array Of Objects? JAVA ARRAY OF OBJECT, as defined by its name, stores an array of objects. Unlike a traditional array that store values like string, integer, Boolean, etc an array of objects stores OBJECTS. The array elements store the location of the reference variables of the object. The target object is the first argument and is also used as the return value. The following example demonstrates how you can use the Object.assign () method to convert an array to an object: const names = ['Alex', 'Bob', 'Johny', 'Atta']; const obj = Object.assign({}, names); console.log( obj); Take a look at this guide to learn more about the ... Java - Array of Objects You might have already created array of integers, strings, floats, etc., which are primitive and mostly well known datatypes. In this tutorial, we will learn how to create an Array of user defined datatype or simply called, class objects. You can create an array of objects just like how you create an array of integers.
Walk through the solution to the third project in this JS practice session and see how to create a JavaScript array of object literals. Java object is a top-level, predefined JavaScript object used to access java classes. JavaArray. Javaarray object is an instance of a java array. Can be created by any java method which creates an array or by using newInstance method of the array class. JavaClass. A JavaScript reference to a Java class. But most browsers permit a very narrow class of objects (specifically, the document.all object for any page), in some contexts, to act as if they emulate the value undefined. Loose equality is one such context: null == A and undefined == A evaluate to true if, and only if, A is an object that emulates undefined.
Classes are declared with the class keyword. We will use function expression syntax to initialize a function and class expression syntax to initialize a class. const x = function() {} Copy. const y = class {} Copy. We can access the [ [Prototype]] of an object using the Object.getPrototypeOf () method. Array constructor syntax:var numericArray = new Array(3); A single array can store values of different data types. An array elements (values) can be accessed using zero based index (key). e.g. array[0]. An array index must be numeric. Array includes length property and various methods to operate on array objects. The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. Description. Arrays are list-like objects whose prototype has methods to perform traversal and mutation operations. Neither the length of a JavaScript array nor the types of its elements are fixed.
JavaScript is more than just strings and numbers. JavaScript lets you create objects and arrays. Objects are similar to classes. The objects are given a name, and then you define the object's properties and property values. An array is an object also, except arrays work with a specific number of values that you can iterate through. JavaScript - The Arrays Object, The Array object lets you store multiple values in a single variable. It stores a fixed-size sequential collection of elements of the same type. An array is use Objects. Object in JavaScript is a unique entity which contains property and methods. Most of the times, variables or arrays are not sufficient to simulate real-life situations, and to deal with such situations JavaScript allows you to create objects. Creating an Object:-A JavaScript object can be created like below-
JavaScript has powerful and flexible object-oriented capabilities with strong support for objects, arrays, hashes and classes. Objects are the foundation of the object-oriented capabilities of JavaScript. They may be dynamically created and are automatically garbage collected. Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: I have an array of unnamed objects, which contain an array of named objects, and I need to get the object where "name" is "string 1" Javascript Array Methods The Javascript Array Class Is A A Set is a collection of unique values
Any changes made to this property affects all instances of that particular object type. Classes. The concept of classes was introduced in JavaScript in ES6 (ECMA2015). In the object-oriented programming paradigm, a class is a blueprint for creating objects with properties and methods while encapsulating the implementation details from the user. Jul 20, 2021 - You might want to return Array objects in your derived array class MyArray. The species pattern lets you override default constructors. For example, when using methods such as map() that returns the default constructor, you want these methods to return a parent Array object, instead of the MyArray ... Oct 03, 2011 - I thought it might be the comments ... book on javascript that I'm using, as thats why I'd put the comments in there in the first place, they are now gone, unlike the error Would the error have anything to do with my event listener that creates my array of objects?...
JavaScript: Arrays vs Objects. September 05, 2016. When learning JavaScript, we all stumble across the phrase "Arrays are just Objects in JavaScript". Today, we're going to put that statement under the microscope. let groceries = ["apples"] console.log(typeof groceries) Looking at the code it is quite obvious that an array is of type object.
3 Ways To Clone Objects In Javascript Samanthaming Com
Rewriting Javascript Converting An Array Of Objects To An
Front End Development Stuff And More Catalin Red
Json Generated By Javascript Code Returns Arrays As Objects
Game Class Constructor Method Solution
Java Reflection Tutorial Create Java Pojo Use Reflection Api
Data Structures Objects And Arrays Eloquent Javascript
Details Of The Object Model Javascript Mdn
Data Structures In Javascript Arrays Hashmaps And Lists
How To Add An Object To An Array In Javascript Geeksforgeeks
The Ultimate Guide To Javascript Prototypal Inheritance
Creating A List Of Objects Inside A Javascript Object Stack
Javascript Array Of Objects Check For Key Stack Overflow
Array Of Objects In C Computer Notes
Abstract Classes In Javascript What Are Abstract Classes
How To Create Array Of Objects In Java Geeksforgeeks
Traverse Array Object Using Javascript Javatpoint
How To Group An Array Of Objects In Javascript By Nikhil
Javascript Lesson 26 Nested Array Object In Javascript
Useful Javascript Array And Object Methods By Robert Cooper
Remove Object From An Array Of Objects In Javascript
Javascript Group An Array Of Objects By Key By Edison
How To Create Array Of Objects In Java
Constructor In Javascript Two Main Types Of Constructor In
0 Response to "28 Javascript Array Of Class Objects"
Post a Comment