31 Get Number From String Javascript Regex
The number from a string in javascript can be extracted into an array of numbers by using the match method. This function takes a regular expression as an argument and extracts the number from the string. Regular expression for extracting a number is (/ (\d+)/). Example 1: This example uses match () function to extract number from string. Capturing groups. A part of a pattern can be enclosed in parentheses (...). This is called a "capturing group". That has two effects: It allows to get a part of the match as a separate item in the result array. If we put a quantifier after the parentheses, it applies to the parentheses as a whole.
How To Check If A String Contains At Least One Number Using
Free online regular expression matches extractor. Just enter your string and regular expression and this utility will automatically extract all string fragments that match to the given regex. There are no intrusive ads, popups or nonsense, just an awesome regex matcher. Load a string, get regex matches. Created for developers by developers from ...
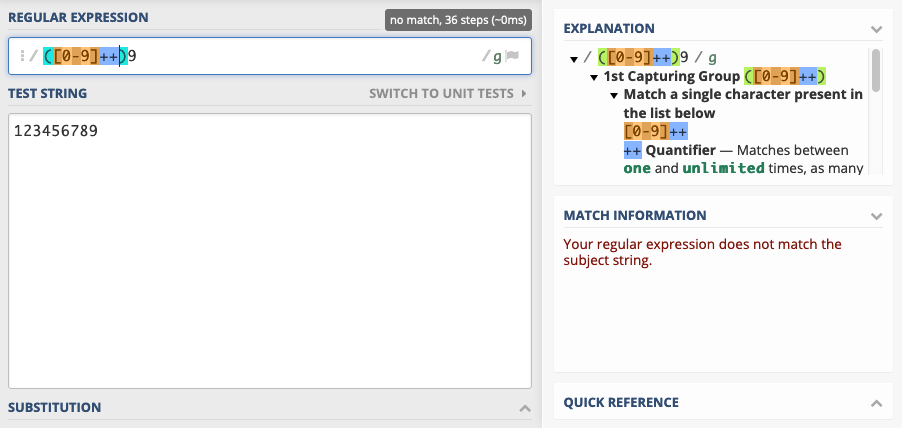
Get number from string javascript regex. May 11, 2015 - Suppose I have a string like - "you can enter maximum 500 choices". I need to extract 500 from the string. The main problem is the String may vary like "you can enter maximum 12500 choices". So ho... javascript create a function that counts the number of syllables a word has. each syllable is separated with a dash -. ... JavaScript function that generates all combinations of a string. Oct 08, 2014 - Hello all, I need help with some simple programming. I need a script to extract numbers out of a string. For eg, this string contains the following fields: $TOTAL (variable) = US$2.40 (string) I need to remove the US$ so that when i submit the form it only passes 2.40 into the output.
Jun 26, 2020 - Write a function that accepts an array of 10 integers (between 0 and 9), that returns a string of those numbers in the form of a phone number. ... Write a javascript program to find roots of quadratic equation. Match string not containing string Url checker with or without http:// or https:// Check if a string only contains numbers Only letters and numbers Match elements of a url Match an email address date format (yyyy-mm-dd) Validate an ip address Url Validation Regex | Regular Expression - Taha ... Get code examples like "regular expression extract number from string javascript" instantly right from your google search results with the Grepper Chrome Extension.
Here's the decomposition of the regex /<(.*)>/: / and / are mandatory to define a regex < and > simply matches the two < and > in your string parenthesis "capture" what you're looking for. Here, they get the mail address inside..* : . means "any character", and * means "any number of times. Phone number extraction with regular expressions. 26. Extract numbers from a string-Javascript. 2. Javascript RegEx match URLs for tokens extraction. 3. Extracting JSON from string using regex. 6. Named placeholders filling and extraction in Java. 5. Parsing numbers and ranges from a string in Javascript. You can always load the string into jQuery and get the attributes: $ ('<tag value="20.434" value1="-12.334" />').attr ('value') $ ('<tag value="20.434" value1="-12.334" />').attr ('value1') In your case regex is probably the better route.
To check for all numbers in a field. To get a string contains only numbers (0-9) we use a regular expression (/^ [0-9]+$/) which allows only numbers. Next, the match () method of the string object is used to match the said regular expression against the input value. Here is the complete web document. HTML Code. How to get numeric value from string JavaScript? Simply use a regular expression in the replace function to get numbers from the string in JS. Regular expression for extracting a number is (/ (\d+)/). string.replace (/ [^0-9]/g, ''); Also, we should use Regex.exec as much as possible instead of String.match for finding single matches. To declare a TypeScript module, use the namespace keyword instead of module . JavaScript_Dots
Occasionally you will need to extract numbers from a string. In this tutorial we show a pretty simple technique using a regular expression with the String ma... Nov 21, 2019 - You can match numbers in the given string using either of the following regular expressions −“\\d+” Or, ([0-9]+)Example 1import java.util.Scanner; impor ... Regex using javascript to return just numbers, I guess you want to get number(s) from the string. In which case, you can use the following: // Returns an array of numbers located in the string The number from a string in javascript can be extracted into an array of numbers by using the match method.
Regex.Split can extract numbers from strings. You get all the numbers that are found in a string. string input = "There are 4 numbers in this string: 40, 30, and 10."; Regex or Regular expressions are patterns used for matching the character combinations in strings. Regex are objects in JavaScript. Patterns are used with RegEx exec and test methods, and the match, replace, search, and split methods of String. Trying to get number of numbers in a string using regex. 1. get phone number from Field of type Phone without changing type to Text. Related. 7. Unable to use multiline with pattern. 0. ... Problem with get all the link from String Regular Expression. 5. Find if a word from a list exist in a string. 0.
Being able to parse strings and extract information from it is a key skill that every tester should have. This is particularly useful when testing APIs and you need to parse a JSON or XML response. The following Java Regular Expression examples focus on extracting numbers or digits from a String. Extract All Numbers from a String import java ... This guide teaches the basics of manipulating data using JavaScript in the browser, or in node.js. Specifically, demonstrating tasks that are geared around preparing data for further analysis and visualization. This guide will demonstrate some basic techniques and how to implement them using ... The [0-9] expression is used to find any character between the brackets. The digits inside the brackets can be any numbers or span of numbers from 0 to 9. Tip: Use the [^0-9] expression to find any character that is NOT a digit.
Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec() and test() methods of RegExp, and with the match(), matchAll(), replace(), replaceAll(), search(), and split() methods of String. This chapter describes JavaScript regular expressions. I would appreciate a combo with each for a multple coincidences inside a string. ie the string 12a55 report the last number, not all. - m3nda Feb 25 '15 at 0:14 1 @YevgeniyAfanasyev it should not. JavaScript comes with handy string methods. RegEx support in JavaScript string methods is helpful in a lot of use-cases. For example, if you want to remove all numbers from a string: JavaScript has your back! Node.js Series Overview
Regular expression for extracting a number is (/(\d+)/). There are many ways you can extract or get numbers of a string in JavaScript. One of the simplest ways of extracting numbers from a given string in JavaScript is using Regex or Regular Expressions. Let us assume I have a string value, ... Jun 09, 2021 - Regular expressions, abbreviated as regex, or sometimes regexp, are one of those concepts that you probably know is really powerful and useful. But they can be daunting, especially for beginning programmers. It doesn't have to be this way. JavaScript includes several helpful methods that make ... One of the simplest ways of extracting numbers from a given string in JavaScript is using Regex or Regular Expressions. Let us assume I have a string value, a name, suffixed with some random numbers. var tName = 'arun_4874'; I want to extract the number 4874 from the above string and there are two simple methods to this.
4 weeks ago - When the regexp parameter is a string or a number, it is implicitly converted to a RegExp by using new RegExp(regexp). If it is a positive number with a positive sign, RegExp() will ignore the positive sign. const str1 = "NaN means not a number. Infinity contains -Infinity and +Infinity in JavaScript... String.match(regexp) is supposed to return an array with the matches. In my browser it doesn't (Safari on Mac returns only the full match, not the groups), but Regexp.exec(string) works. ... Javascript extract unknown number in string-1. Javascript Get all number from array. 0. Skip the same string value. 0. Use Regular Expression to Get Strings Between Parentheses with JavaScript. We can use the match method to get the substrings in a string that match the given regex pattern. We create the regExp regex that matches anything between parentheses. The g flag indicates we search for all substrings that matches the given pattern.
26. Consider the following string in javascript: var string="border-radius:90px 20px 30px 40px"; I want to extract the 4 numbers from that string and store them in an array called numbers.To do that I developed the following code: var numbers=string.split ("border-radius:"); numbers=numbers [1].split ("px"); This code is working fine but I was ... javascript extract number from string . Method str.replace (regexp, replacement) that replaces all matches with regexp in str allows to use parentheses contents in the replacement string. Let's start with the real-life-sounding, contrived scenario of wanting to extract all numbers from a JavaScript string. Ran a wrong sed command. At a first glance they may remind you of wildcards; however ... Found a tool which allows you to edit regular expression visually: JavaScript Regular Expression Parser & Visualizer. Update: Here's another one with which you can even debugger regexp: Online regex tester and debugger. Update: Another one: RegExr. Update: Regexper and Regex Pal.
Regex Tutorial. The term Regex stands for Regular expression. The regex or regexp or regular expression is a sequence of different characters which describe the particular search pattern. It is also referred/called as a Rational expression. It is mainly used for searching and manipulating text strings. The regexp.exec (str) method returns a match for regexp in the string str. Unlike previous methods, it's called on a regexp, not on a string. It behaves differently depending on whether the regexp has flag g. If there's no g, then regexp.exec (str) returns the first match exactly as str.match (regexp). Jul 26, 2009 - If I have a string like "something12" or "something102", how would I use a regex in javascript to return just the number parts?
Regular Expressions (also called RegEx or RegExp) are a powerful way to analyze text. With RegEx, you can match strings at points that match specific characters (for example, JavaScript) or patterns (for example, NumberStringSymbol - 3a&). The .replace method is used on strings in JavaScript to replace parts of string with characters. It is ...
Everything You Need To Know About Regular Expressions By
Everything You Need To Know About Regular Expressions By
How Javascript Works Regular Expressions Regexp By
Basic Regex In Javascript For Beginners Dev Community
8 Regular Expressions You Should Know
Javascript Extract Number From String Regex Amp Replace
Introduction To The Use Of 10 Regular Expressions In
A Guide To Javascript Regular Expressions
Basic Regex In Javascript For Beginners Dev Community
How To Remove Numbers From A String Learn Uipath
How To Get Numbers From A String In Javascript
Regular Expressions Eloquent Javascript
How To Find Patterns Of Text In Javascript By Anh Dang
Javascript Regex Match Example How To Use Js Replace On A
How To Compare String And Number In Javascript Code Example
Javascript Validation With Regular Expression Count Number
Javascript Split Examples Dot Net Perls
Javascript Regex Match Example How To Use Js Replace On A
Built In Way In Javascript To Check If A String Is A Valid
Javascript Regex To Prepend String To Variable Names Jupyter
Javascript Regex Match Check If String Matches Regex
Regular Expression Tutorial Learn How To Use Regular
Github Mikemcl Bignumber Js A Javascript Library For
Everything You Need To Know About Regular Expressions By
Regex Tricks Change Strings To Formatted Numbers 231webdev
How Javascript Works Regular Expressions Regexp By
Everything You Need To Know About Regular Expressions By
Playing With Regexp Named Capture Groups In Node 10
How To Get Decimal Portion Of A Number Using Javascript
0 Response to "31 Get Number From String Javascript Regex"
Post a Comment