27 Javascript Parse Phone Number
Google's libphonenumber is an ultimate phone number formatting and parsing library developed by Google for Android phones. It is written in C++ and Java, and, while it has an official autogenerated javascript port, that port is tightly coupled to Google's closure javascript framework, and, ... This blog post will walk through how to build a phone number input field to process and parse international phone numbers using basic HTML, JavaScript, and the intl-tel-input plugin. Level up your Twilio API skills in TwilioQuest , an educational game for Mac, Windows, and Linux.
Intl Phone Number Input Flutter Package
For projects that support PackageReference, copy this XML node into the project file to reference the package. paket add libphonenumber-csharp --version 8.12.30. The NuGet Team does not provide support for this client. Please contact its maintainers for support. #r "nuget: libphonenumber-csharp, 8.12.30".
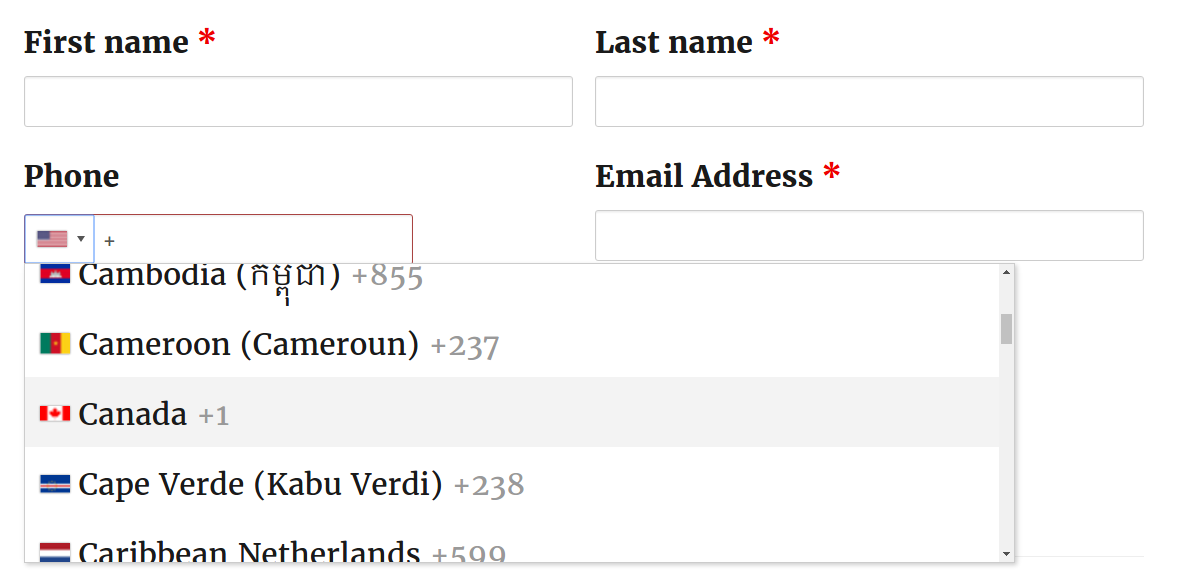
Javascript parse phone number. Every JavaScript number format method may be used on any type of number, including literals, variables, expressions. Methods Used For Numbers There are a few JavaScript number format methods to keep in mind if you want to manipulate and change numeric values. Nov 29, 2020 - Learn how to build phone number input that formats and validates user input from scratch using Javascript. isPossibleNumber - quickly guesses whether a number is a possible phone number by using only the length information, much faster than a full validation. isValidNumber - full validation of a phone number for a region using length and prefix information.Tests whether a phone number matches a valid pattern.
Feb 26, 2020 - Javascript function to validate a phone number. Also discussed a phone number structure, example of valid phone number. 4/4/2012 · This parse solves this by choosing a minimum valid number length (which can change for each number during the parse depending on the type of number detected), and collecting the digits of that number until either a non-number non-punctuation character is found (text or other punctuation) which definitely delimits a number or in the case of a space, if we have enough number to satisfy the ... Google's common Java, C++ and JavaScript library for parsing, formatting, and validating international phone numbers. The Java version is optimized for running on smartphones, and is used by the Android framework since 4.0 (Ice Cream Sandwich).
more easily, Google developed and helps maintain an open source Java, C++, and JavaScript library for "parsing, formatting, and validating international phone numbers", libphonenumber. This is a great thing, but not convenient for C# developers working in ASP.NET or ASP.NET Core who want to do server-side data validation (which is definitely a ... Parse phone number. Hey friends, support level up lunch by signing up with project fi and receive a $20 credit!! This example will show how to parse a phone number from a formatted string using java, google guava, apache commons and google libphonenumber. A quick little background. The 3-3-4 phone number scheme was first developed by AT&T in 1947. A simpler (and smaller) rewrite of Google Android's libphonenumber library in javascript. https://catamphetamine.gitlab.io/libphonenumber-js
The function takes a parsed telephone number as its argument: var tel = phoneUtil.parse (' (0) 20 7925 0918', 'GB'); var type = phoneUtil.getNumberType (tel) The return value is a constant defined... Dec 31, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Aug 04, 2014 - Free source code and tutorials for Software developers and Architects.; Updated: 30 Oct 2019
May 30, 2019 - Learn how to format phone number in javascript or Jquery for any country. Format the phone number with or without country code. Number formatting in JavaScript Jun 26, 2018 2 min read There are many different ways of printing an integer with a comma as a thousands separators in JavaScript. The number from a string in javascript can be extracted into an array of numbers by using the match method. This function takes a regular expression as an argument and extracts the number from the string. Regular expression for extracting a number is (/ (\d+)/). Example 1: This example uses match () function to extract number from string.
Nov 10, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. The Number.parseFloat () method parses an argument and returns a floating point number. If a number cannot be parsed from the argument, it returns NaN. So let's create that first: PhoneNumber phoneNumberProto = phoneUtil.parse (inputPhoneNumber, shortCode); This will be called inside a loop, so the inputPhoneNumber variable will have the phone number from each list, and the shortCode variable will be the short code of the country against which we're running the test.
Dec 02, 2011 - I'm looking to reformat (replace, not validate - there are many references for validating) a phone number for display in Javascript. Here's an example of some of the data: 123 4567890 (123) 456-78... Jan 04, 2021 - How to format a string or number to a US phone number in JavaScript. Solution for formatting an input to a US phone number format in JavaScript. Write a function that accepts a string of numbers or a number and converts it to a US phone number format. What HTML5 or JavaScript functions can retrieve the mobile phone , libphonenumber is an ultimate phone number formatting and parsing library from a simpler and smaller library that would just get the parsing and for phone numbers in text (Google's autogenerated javascript port can't).
: After the second set of 3 digits the phone number can have another optional hyphen (-). E.g (308)-135-or 308-135-or 308135-(\d{4})$/: Finally, the phone number must end with four digits. E.g (308)-135-7895 or 308-135-7895 or 308135-7895 or 3081357895. Reference : http://www.zparacha /phone_number_regex/ Apr 19, 2020 - What you don’t see often is telephone number validation because it's difficult. Not anymore. So here are some Javascript libraries that will help you to add validation to your phone number input fields. Google's common Java, C++ and JavaScript library for parsing, formatting, and validating international phone numbers. - GitHub - google/libphonenumber: Google's common Java, C++ and JavaScript library for parsing, formatting, and validating international phone numbers.
JavaScript is known for its wide assortment of plugins. These plugins allow developers to execute everyday tasks efficiently without having to write the same scripts over and over again. ... The best thing about the library is its ability to automatically parse and format phone numbers in any given input. You can start even with mixed type ... You can create this from a string representing a phone number using the parse function, but you also need to specify the country that the phone number is being dialled from (unless the number is in E.164 format, which is globally unique). Google's libphonenumber is a library that parses, formats, stores and validates international phone numbers. It is used by Android since version 4.0 and is a phenomenal repository of carrier metadata. Although it compiles down to Java, C++ and JS, its JS port is tightly coupled to the Google Closure library.
When the user presses the "Check" button, the script checks the validity of the number. If the number is valid (matches the character sequence specified by the regular expression), the script shows a message thanking the user and confirming the number. If the number is invalid, the script informs the user that the phone number is not valid. A simpler and smaller rewrite of Google Android's libphonenumber library · This is a demo for the new API. See legacy API demo grunt test - downloads PhoneNumberMetadata.xml and prints converted JavaScript to console · If you want to update PhoneNumberMetadata.js, just run: ... Loading these files exposes the following globals: PhoneNumber, PhoneNumberNormalizer and PHONE_NUMBER_META_DATA. ... If the parser is not able ...
If the number is invalid, alert message will be displayed as 'Phone number is not valid. This javaScript code determine whether a user entered phone number in a common format that includes 0999999999, 099-999-999, (099)-999-9999, (099)9999999, 099 999 9999, 099 999-9999, (099) 999-9999, 099.999.9999 and all related combinations Parse Phone Numbers Create a parsePhone function that takes a string as input. If the input cannot be parsed then return null. If the input can be parsed, return an object with the following ... The Number () function converts the object argument to a number that represents the object's value. If the value cannot be converted to a legal number, NaN is returned. Note: If the parameter is a Date object, the Number () function returns the number of milliseconds since midnight January 1, 1970 UTC.
Today I will introduce a simple solution to do international phone number parsing, validation and formatting in Infor Smart Office.The goal is to validate phone numbers entered by users in Infor M3 Programs such as M3 Customer - CRS610/E, or in Infor Customer Lifecycle Management (CLM) Account Details, against international phone number specifications, and to get the resulting phone number ... phone number validation JavaScript JS. Phone number validation in JavaScript is used to make sure that all the number entered in the specified phone number field should be a valid phone number. A Parse.Relation behaves similar to an array of Parse.Object for querying purposes, so any query you can do on an array of objects, you can do on a Parse.Relation. Data Types. So far we've used values with type String, Number, and Parse.Object. Parse also supports Dates and null.
I have a list of phone numbers which are formatted in multiple ways such as: (212)-555-1234 or 212-555-1234 or 2125551234. Using JavaScript, what would be the best way to extract only the area cod... Jul 28, 2021 - JavaScript Library For Parsing, Formatting and Validating Phone Numbers – front.phone ... front.phone is an easy yet useful JavaScript library used for identifying, formatting and validation phone numbers. In order to remove all non-numeric characters from a string, replace() function is used. replace() Function: This functiion searches a string for a specific value, or a RegExp, and returns a new string where the replacement is done. Syntax: string.replace( searchVal, newValue ) Parameters: This function accepts two parameters as mentioned above and described below:
4.2. Validate and Format North American Phone Numbers Problem You want to determine whether a user entered a North American phone number, including the local area code, in a common … - Selection from Regular Expressions Cookbook, 2nd Edition [Book] 1 week ago - Google's libphonenumber is an ultimate phone number formatting and parsing library developed by Google for Android phones. It is written in C++ and Java, and, while it has an official autogenerated javascript port, that port is tightly coupled to Google's closure javascript framework, and, ... The parseInt () function parses a string and returns an integer. The radix parameter is used to specify which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. If the radix parameter is omitted, JavaScript assumes the following:
Parsing the Address Number. According to the Thoroughfare, Landmark, and Postal Address Data Standard document, the Address Number Prefix "is not found in most Complete Address Numbers", so let's start with the Address Number itself. I'll be using JavaScript to execute my tests because it doesn't require any special tools. A javascript library for formatting and manipulating numbers. libphonenumbers is JS port of Google's libphonenumber. libphonenumbers - JavaScript port of Google's libphonenumber library for parsing, formatting, and validating international phone numbers in Node.js.
Js Regex Validate Phone Number Code Example
Download Parse Phone Auth Login Parse Server Users By
Input Type Tel Gt Html Hypertext Markup Language Mdn
Phone Hacking Script Javascript Code Example
Troy Hunt The Facebook Phone Numbers Are Now Searchable In
Github Jackocnr Intl Tel Input A Javascript Plugin For
Phone Number Fields Better Wordpress Form Builder
Fresh Installation Unable To Set Invalid Phone Number
The Current State Of Telephone Links Css Tricks
Working With Phone Numbers In Javascript Sitepoint
Libphonenumber Javascript Phone Number Parsing And
Friendly Format For Phone Numbers User Experience Stack
How To Format A Phone Number In Human Readable Using
Json Format Example Json Table Function Ibm Developer
A Beautiful Text Field To Format Phone Numbers Made With Vuejs
Auto Format Phone Number Based On Country With Javascript
Lightweight Jquery Input Mask Plugin For Phone Numbers Free
How To Build International Phone Number Input In Html And
International Telephone Input With Vue
Phone Number Formatting In Android Stack Overflow
Building A Phone Input Field In Javascript From Scratch By
How To Validate Phone Number With Javascript
How To Extract Email Addresses Phone Numbers And Links From
How To Find Patterns Of Text In Javascript By Anh Dang
How To Build International Phone Number Input In Html And
How To Change Phone Number Format In Input As You Type
0 Response to "27 Javascript Parse Phone Number"
Post a Comment