28 Javascript Push Object Into Object
So, how can you add an array element into a JSON Object in JavaScript? This is done by using the JavaScript native methods .parse()and .stringify() We want to do this following: Parse the JSON object to create a native JavaScript Object; Push new array element into the object using .push() Use stringify() to convert it back to its original format. The only native Javascript function to convert a string into an object is JSON.parse (). For example, var parsed = JSON.parse (' {"foo":"bar"}'). To convert strings of other formats, it has to be done manually. That covers the basics, but let us walk through a few more examples in this guide - Read on! ⓘ I have included a zip file with all ...
How To Find Unique Values By Property In An Array Of Objects
Use the object.assign () Method to Append Elements to Objects in JavaScript The object.assign () method will copy all properties defined in an object to another object, i.e., it copies all properties from one or more sources to the target objects. By doing this, we are appending an element to the object.
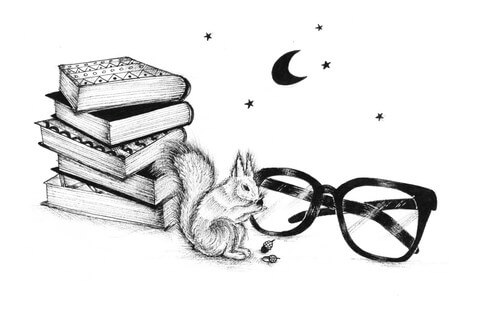
Javascript push object into object. So we get a plain object with same key/values as the map. Set. A Set is a special type collection - "set of values" (without keys), where each value may occur only once. Its main methods are: new Set(iterable) - creates the set, and if an iterable object is provided (usually an array), copies values from it into the set. The Object.assign () method only copies enumerable and own properties from a source object to a target object. It uses [ [Get]] on the source and [ [Set]] on the target, so it will invoke getters and setters. Therefore it assigns properties, versus copying or defining new properties. In Javascript, push () is a method that helps in adding one or more than one elements to an array's end. That is, length of the array will be changed on using this push () method. Moreover, it has certain other features. This method is deliberately generic. This method can be used on arrays that resembles objects.
12/7/2019 · In order to push an array into the object in JavaScript, we need to utilize the push () function. With the help of Array push function this task is so much easy to achieve. push () function: The array push () function adds one or more values to the end of the array and returns the new length. This method changes the length of the array. The reason is the code -. var activeItem = { itemId : 0, itemName: '', itemAmount : 0, itemPrice : 0 } You see, this line creates and initialises the object activeItem. Nowhere else you have instantiated a new one. And thus every time you are modifying it, it is modifying the same item and inserting the same item. The task is to convert an Object {} to an Array [] of key-value pairs using JavaScript. Introduction: Objects, in JavaScript, is it's most important data-type and forms the building blocks for modern JavaScript. These objects are quite different from JavaScript's primitive data-types(Number, String, Boolean, null, undefined and symbol).
The destructuring assignment is a cool feature that came along with ES6. Destructuring is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables. That is, we can extract data from arrays and objects and assign them to variables. Why is So, we are going to push an object (maybe empty object) into that array. myArray.push({}), or myArray.push({""}). This will push an empty object into myArray which will have an index number 0, so your exact object is now myArray[0] Then push property and value into that like this: myArray[0].property = value; //in your case: myArray[0]["01 ... From our class, we can create object instances — objects that contain the data and functionality defined in the class. From our Person class, we can now create some actual people: When an object instance is created from a class, the class's constructor function is run to create it. This process of creating an object instance from a class is called instantiation — the object instance is ...
Objects does not support push property, but you can save it as well using the index as key, var tempData = {}; for ( var index in data ) { if ( data[index].Status == "Valid" ) { tempData[index] = data; } } data = tempData; Spread syntax can be used when all elements from an object or array need to be included in a list of some kind. In the above example, the defined function takes x, y, and z as arguments and returns the sum of these values. An array value is also defined. When we invoke the function, we pass it all the values in the array using the spread syntax ... person[0] will access the first item in the array. If you want to push something onto that array, it would be: person.push() Your array contains an object as it's first item, so I would assume you'd want to do something like this if you were going to push to the array:
Summary. Objects are assigned and copied by reference. In other words, a variable stores not the "object value", but a "reference" (address in memory) for the value. So copying such a variable or passing it as a function argument copies that reference, not the object itself. All operations via copied references (like adding/removing ... The push () method adds new items to the end of an array. push () changes the length of the array and returns the new length. Tip: To add items at the beginning of an array, use unshift (). The push method appends values to an array.. push is intentionally generic. This method can be used with call() or apply() on objects resembling arrays. The push method relies on a length property to determine where to start inserting the given values. If the length property cannot be converted into a number, the index used is 0. This includes the possibility of length being nonexistent, in ...
proto. The object which should be the prototype of the newly-created object. propertiesObject Optional. If specified and not undefined, an object whose enumerable own properties (that is, those properties defined upon itself and not enumerable properties along its prototype chain) specify property descriptors to be added to the newly-created object, with the corresponding property names. 22/4/2018 · JavaScript does not make a copy of the object, it makes the new variable (or array item) contain the exact same object you assigned. To illustrate this take a look at this code: var example = { message: "Hello" }; var example2 = example; example. message = "world"; console. log ( example2 ); JavaScript Push object into array with key. object. array. key. javascript. IvanLomakin posted Aug 4 1 min read. In this article, let's have a look at how to push object into array with key through example. The example is as follows:
javascript array push object. How to push an array of the object into array. Let's take a simple example of this, suppose we have one an arrayObj and it contains two object. If you want to add one more object into the arrayObj. So you can see the below example. stuff is an object and push is a method of an array. So you cannot use stuff.push(..). Lets say you define stuff as an array stuff = []; then you can call push method on it. This works because the object[key/value] is well formed. stuff.push( {'name':$(this).attr('checked')} ); Whereas this will not work because the object is not well formed. Introduction. An object in JavaScript is a data type that is composed of a collection of names or keys and values, represented in name:value pairs.The name:value pairs can consist of properties that may contain any data type — including strings, numbers, and Booleans — as well as methods, which are functions contained within an object.. Objects in JavaScript are standalone entities that ...
Method 1: push () method of Array. The push () method is used to add one or multiple elements to the end of an array. It returns the new length of the array formed. An object can be inserted by passing the object as a parameter to this method. The object is hence added to the end of the array. In this article, I'll show you the basics of working with object arrays in JavaScript. If you ever worked with a JSON structure, you've worked with JavaScript objects. Quite literally. JSON stands for JavaScript Object Notation. Creating an object is as simple as this: How To Check If An Object Is Empty In JavaScript; Understanding Object.keys Method In JavaScript; JavaScript Ternary Operator; How To Reverse A String In JavaScript; JavaScript, Array Contains; JavaScript Array Includes Method; How To Return Data From JavaScript Promise; How To Push JSON Object Into An Array Using JavaScript; How To Create JSON ...
Create a new object. Setup a variable to count the number of loops (since objects don’t have a native index). Loop through the original object. If the index variable equals the position you want to insert the new key/value pair into, push that to the new object. Push the old key/value pairs into the new object. In the above program, the splice () method is used to add an object to an array. The splice () method adds and/or removes an item. The first argument represents the index where you want to insert an item. The second argument represents the number of items to be removed (here, 0). The third argument represents the element that you want to add to ... Return value: This method returns the new length of the array after inserting the arguments into the array. Below examples illustrate the JavaScript Array push () method: Example 1: In this example the function push () adds the numbers to the end of the array. Example 2: In this example the function push () adds the objects to the end of the array.
Respond To Change With Object Observe Web Google Developers
Comprehensive Guide To Javascript Design Patterns Toptal
Javascript Array Push How To Add Element In Array
Object In Java Class In Java Javatpoint
How To Push Object To An Array Php Code Example
How To Check If Object Is Empty In Javascript Samanthaming Com
Immutability In Javascript React And Immutable Js By Y
Angularjs Push Values Object Into Array First Index
5 Way To Append Item To Array In Javascript Samanthaming Com
Javascript Object Convert An Object Into A List Of Pairs
How To Add Object Inside Object In Javascript Code Example
Javascript Tracking Key Value Pairs Using Hashmaps By
Push Object Key Into Array Javascript
How To Use Javascript Collections Map And Set
How To Add An Object To An Array In Javascript Geeksforgeeks
How Do I Correctly Clone A Javascript Object Stack Overflow
Angularjs Push Object Into Array First Index Angularjs
Push Data Into Javascript Object Is Not Working Stack Overflow
What Is Center Of Mass Article Khan Academy
How To Insert A File Into A Word Document 7 Steps With
Array Push Objects Javascript Code Example
What Is Nullreferenceexception Object Reference Not Set To
Push In Javascript How Push Works In Javascript
How To Get The Current Url And Pathname In React Suraj Sharma
Pop Push Shift And Unshift Array Methods In Javascript
Data Structures Objects And Arrays Eloquent Javascript
The Json Query Function To Extract Objects From Json Data
0 Response to "28 Javascript Push Object Into Object"
Post a Comment