30 Javascript Map Return Value
The callback function () is called on each array element, and the map () method always passes the current element, the index of the current element, and the whole array object to it. The this argument will be used inside the callback function. By default, its value is undefined. For example, here's how to change the this value to the number 80: The return statement stops the execution of a function and returns a value from that function. Read our JavaScript Tutorial to learn all you need to know about functions. Start with the introduction chapter about JavaScript Functions and JavaScript Scope. For more detailed information, see our Function Section on Function Definitions ...
Javascript Map Array Array Map Method Mdn
Map is a data structure in JavaScript which allows storing of [key, value] pairs where any value can be either used as a key or value. The keys and values in the map collection may be of any type and if a value is added to the map collection using a key which already exists in the collection, then the new value replaces the old value.
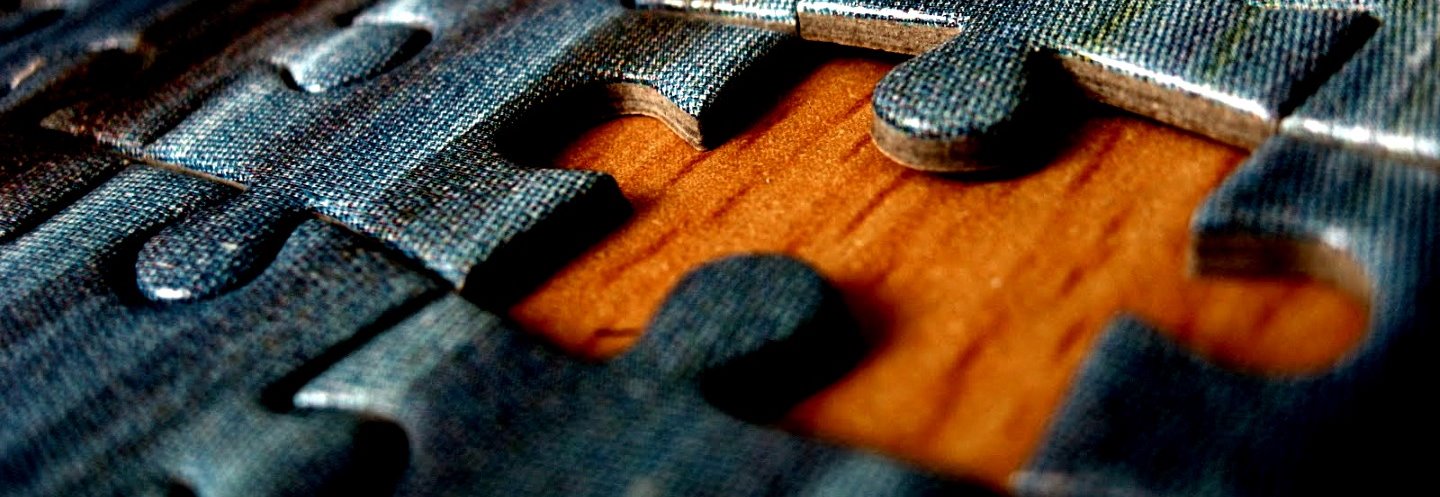
Javascript map return value. Map.prototype.values () The values () method returns a new Iterator object that contains the values for each element in the Map object in insertion order. Sep 04, 2019 - So how does .map() work? Basically is takes 2 arguments, a callback and an optional context (will be considered as this in the callback) which I did not use in the previous example. The callback runs for each value in the array and returns each new value in the resulting array. set (key, value) - sets the value for the key in the map object. It returns the map object itself therefore you can chain this method with other methods. values () returns a new iterator object that contains values for each element in insertion order.
The map () method is used to apply a function on every element in an array. A new array is then returned. Here's what the syntax looks like: let newArr = oldArr.map ( (val, index, arr) => { // return element to new Array }); newArr — the new array that is returned. oldArr — the array to run the map function on. The map () method in JavaScript creates an array by calling a specific function on each element present in the parent array. It is a non-mutating method. Generally map () method is used to iterate over an array and calling function on every element of array. Array iteration methods are like a 'gateway drug'. 1 They get many people hooked on functional programming. Because they're just so darn useful. And most of these array methods are fairly simple to understand. Methods like .map() and .filter() take just one callback argument, and do fairly simple things. But .reduce() seems to give people trouble. It's a bit harder to grasp.
map, filter, reduce, find. Those are 3 really powerful array functions: map returns an array with the same length, filter as the name implies, it returns an array with less items than the original array; reduce returns a single value (or object) find returns the first items in an array that satisfies a condition JavaScript Map values() method. The JavaScript map values() method returns an object of new Map iterator. This object contains the value for each element. It maintains insertion order. Syntax. The values() method is represented by the following syntax: Jan 30, 2020 - The Map() accepts an optional iterable object whose elements are key-value pairs. ... delete(key) – removes an element specified by the key. It returns if the element is in the map, or false if it does not.
The Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value. ... A Map object iterates its elements in insertion order — a for...of loop returns an array of [key, value] for ... The compare () function accepts two arguments a and b. The sort () method will sort elements based on the return value of the compare () function with the following rules: If compare (a,b) is less than zero, the sort () method sorts a to a lower index than b. In other words, a will come first. var new_array = arr.map (function callback (element, index, array) { // Return value for new_array } [, thisArg]) In the callback, only the array element is required. Usually some action is performed on the value and then a new value is returned.
forEach vs map: Return value. forEach and map both iterate elements. The choice of choosing one over another depends on the situation. The basic difference is map always returns a new array with ... Definition and Usage. The map() method creates a new array with the results of calling a function for every array element.. The map() method calls the provided function once for each element in an array, in order.. map() does not execute the function for empty elements. map() does not change the original array. Jun 20, 2018 - In this instance we’ll return v or the current value unchanged. ... Thanks for reading! This has been a brief introduction into JavaScript’s map() function. If you’re ready to finally learn Web Development, check out the The Ultimate Guide to Learning Full Stack Web Development in 6 months.
Jun 03, 2019 - And if you're using arrow functions ... explicit return, and the logging statement: ... Over time this can begin to feel a little cumbersome, especially if you're deep into debugging some issue. However, we can leverage a little JavaScript logic to make debugging map a whole lot easier: ... All we have to do is add the console.log(value) with a || ... Apr 25, 2018 - The iteration of elements in a map object is done in the insertion order and a “for…” loop returns an array of all [key, value] pairs for each iteration. Differences between Objects and Maps in JavaScript Both of these data structures are similar in many ways such as both store values ... If you wish to process a plain array or object, use the jQuery.map () instead. As the return value is a jQuery object, which contains an array, it's very common to call .get () on the result to work with a basic array. The .map () method is particularly useful for getting or setting the value of a collection of elements.
Get first value from javascript Map Map is a new key/value data structure in es6 (and its available in your chrome browser RIGHT NOW). But getting values out of a Map without using a key is complicated a little by having to go through a javascript iterator. Map keys. Just like any value (object, array, string, number) can be used as the value of the key-value entry of a map item, any value can be used as the key, even objects. If you try to get a non-existing key using get() out of a map, it will return undefined. Weird situations you'll almost never find in real life It runs each element through an iteratee function and returns an array with the results. The synchronous version that adds one to each element: const arr = [1, 2, 3]; const syncRes = arr.map( (i) => { return i + 1; }); console.log(syncRes); // 2,3,4. An async version needs to do two things. First, it needs to map every item to a Promise with ...
Description. Javascript array map() method creates a new array with the results of calling a provided function on every element in this array.. Syntax. Its syntax is as follows −. array.map(callback[, thisObject]); Parameter Details. callback − Function that produces an element of the new Array from an element of the current one.. thisObject − Object to use as this when executing callback. .map () accepts a callback function as one of its arguments, and an important parameter of that function is the current value of the item being processed by the function. This is a required parameter. With this parameter, you can modify each item in an array and create a new function. The syntax of JavaScript map method is as below : let finalArray = arr.map(callback( currentValue[, index[, array]]), arg) Following are the meanings of these parameters : callback: This is the callback method. It is called for each of the array arr elements. The returned value of this method is added to the final array finalArray.
2 days ago - Each time callbackFn executes, the returned value is added to newArray. The callbackFn function accepts the following arguments: ... The current element being processed in the array. ... The index of the current element being processed in the array. ... The array map was called upon. The Array.prototype.map() method was introduced in ES6 (ECMAScript 2015) for iterating and manipulating elements of an array in one go. This method creates a new array by executing the given function for each element in the array. The Array.map() method accepts a callback function as a parameter that you want to invoke for each item in the array. This function must return a value after ... Dec 12, 2017 - Meanwhile, the map() method will also call a provided function on every element in the array. The difference is that map() utilizes return values and actually returns a new Array of the same size.
new Map () - creates the map. map.set (key, value) - stores the value by the key. map.get (key) - returns the value by the key, undefined if key doesn't exist in map. map.has (key) - returns true if the key exists, false otherwise. With map, you can operate on the element directly, rather than having to index into the array. You don't have to create a new array and push into it. map returns the finished product all in one go, so we can simply assign the return value to a new variable. You do have to remember to include a return statement in your callback. 4 weeks ago - In this article we would be discussing Map object provided by ES6. Map is a collection of elements where each element is stored as a Key, value pair. Map object can hold both objects and primitive values as either key or value. When we iterate over the map object it returns the key,value pair ...
In the case of map, the return value of the callback function is used as the transformed value in our new array. However, forEach doesn't return anything, and if the callback function returns a value, nothing is done with that value.
Map Not Working As Expected On Uninitiated Array Stack Overflow
Javascript Tracking Key Value Pairs Using Hashmaps By
Our Guide To Map Filter And Reduce Functions In Python Udacity
Javascript Fundamental Es6 Syntax Create An Array Of
Javascript Merge Array Of Objects By Key Es6 Reactgo
How To Check If Array Includes A Value In Javascript
Javascript Array Map Method Geeksforgeeks
Map In Javascript Geeksforgeeks
Javascript Array Map Method Geeksforgeeks
Higher Order Function In Javascript Json World
How To Clone An Array In Javascript
How To Use Async Await With Array Map In Javascript Codez Up
Using The Map Function In Javascript And React By Jonathan
Javascript Language Mind Map Element Objects Mind Map
Javascript Map Vs Foreach What S The Difference Between
Exploring Sets And Maps In Javascript Scotch Io
Source Map Support For Javascript Error Analysis Dynatrace
Using Leaflet Instead Of Folium In Streamlit To Return
Javascript Map Vs Foreach What S The Difference Between
Cloud Integration Mapping Your Options Explained And
Map In Javascript Geeksforgeeks
Getting Javascript Properties For Object Maps By Index Or
How To Use Array Map To Render Data In React
Using The Map Function In Javascript And React By Jonathan
Array Map Method In Javascript It Boy Java Script
Javascript Engine Fundamentals Shapes And Inline Caches
0 Response to "30 Javascript Map Return Value"
Post a Comment