22 Javascript If Match Regex
Performs a regular expression (regex) pattern matching and returns: true if a match exists.; false if a match doesn't exist.; MongoDB uses Perl compatible regular expressions (i.e. "PCRE" ) version 8.41 with UTF-8 support. RegExr is an online tool to learn, build, & test Regular Expressions (RegEx / RegExp). Supports JavaScript & PHP/PCRE RegEx. Results update in real-time as you type. Roll over a match or expression for details.
Regular Expressions In Javascript Guide To Regular Expressions
In this article we'll cover various methods that work with regexps in-depth. str.match(regexp) The method str.match(regexp) finds matches for regexp in the string str.. It has 3 modes: If the regexp doesn't have flag g, then it returns the first match as an array with capturing groups and properties index (position of the match), input (input string, equals str):
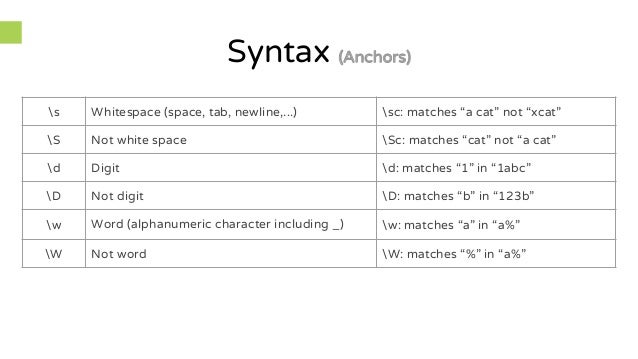
Javascript if match regex. JavaScript RegExp - [a-z], [a-z] matches any character from lowercase a through lowercase z. Regular Expression to Given a list of strings (words or other characters), only return the strings that do not match. If you need to know if a string matches a regular expression RegExp , use RegExp.test () . If you only want the first match found, you might want to use RegExp.exec () instead. If you want to obtain capture groups and the global flag is set, you need to use RegExp.exec () or String.prototype.matchAll () instead.
I want to use JavaScript (can be with jQuery) to do some client-side validation to check whether a string matches the regex: ^([a-z0-9]{5,})$ Ideally it would be an expression that returned true or false. I'm a JavaScript newbie, does match() do what I need? It seems to check whether part of a string matches a regex, not the whole thing. If you use exec() or match() and if the match succeeds, these methods return an array and update properties of the associated regular expression object and also of the predefined regular expression object, RegExp. If the match fails, the exec() method returns null (which coerces to false). javascript regex example match. javascript by POG on Apr 07 2020 Donate Comment. 11. //Declare Reg using slash let reg = /abc/ //Declare using class, useful for buil a RegExp from a variable reg = new RegExp ('abc') //Option you must know: i -> Not case sensitive, g -> match all the string let str = 'Abc abc abc' str.match (/abc/) //Array (1 ...
In JavaScript, you can use regular expressions with RegExp () methods: test () and exec (). There are also some string methods that allow you to pass RegEx as its parameter. They are: match (), replace (), search (), and split (). Executes a search for a match in a string and returns an array of information. RegExp.prototype.test () This method is used to test whether a match has been found or not. It accepts a string which we have to test against regular expression and returns true or false depending upon if the match is found or not. If a match is found, the returned Match object's Value property contains the substring from input that matches the regular expression pattern. If no match is found, its value is String.Empty. This method returns the first substring found in input that matches the regular expression pattern.
RegExp Object. A regular expression is an object that describes a pattern of characters. Regular expressions are used to perform pattern-matching and "search-and-replace" functions on text. 18/8/2020 · Javascript regex match JavaScript match () method searches a string for a match versus a regular expression, and returns the matches, as the array. To use a javascript regex match, use a string match () method. The string.match () is an inbuilt function in JavaScript used to search a string for a match against any regular expression. If the match is found, then this will return the match as an array. Parameters: Here the parameter is "regExp" (i.e. regular expression) which will compare with the given string.
The match () method searches a string for a match against a regular expression, and returns the matches, as an Array object. Read more about regular expressions in our RegExp Tutorial and our RegExp Object Reference. Note: If the regular expression does not include the g modifier (to perform a global search), the match () method will return only ... String.prototype.match () String.prototype.match () is a method in JavaScript that returns an array of results matching a string against a regular expression. Returned results depend a lot on using the global (g) flag. If a g flag is used we will get all results but without capturing groups. Use regular expressions (RegEx) to determine the strength of a password in JavaScript by checking if certain alpha numeric or special characters exist. ... mainly for use in pattern matching with strings, or string matching. RegEx is nice because you can accomplish a whole lot with very little. In this case we are going to check various aspects ...
Regular Expressions (also called RegEx or RegExp) are a powerful way to analyze text. With RegEx, you can match strings at points that match specific characters (for example, JavaScript) or patterns (for example, NumberStringSymbol - 3a&). The .replace method is used on strings in JavaScript to replace parts of function getAllMatches(regex, text) { if (regex.constructor !== RegExp) { throw new Error('not RegExp'); } var res = []; var match = null; if (regex.global) { while (match = regex.exec(text)) { res.push(match); } } else { if (match = regex.exec(text)) { res.push(match); } } return res; } // Example: var regex = /abc|def|ghi/g; var res = getAllMatches(regex, 'abcdefghi'); res.forEach(function (item) { console.log(item[0]); }); RegExpオブジェクトを使用する方法. 今度は、RegExpオブジェクトを利用したmatchメソッドの使い方を見ていきましょう!RegExpオブジェクトは、JavaScriptの標準で用意されている正規表現を扱うのに便利なクラスになります。 RegExpの基本構文は以下の通りです。
The RegExp object is known as regular expression, it is used to specify the pattern of characters. Regular expressions are patterns used to match character combinations in strings. This is used for the pattern matching and also very useful for search and replace the text Regular expression tester with syntax highlighting, explanation, cheat sheet for PHP/PCRE, Python, GO, JavaScript, Java. Features a regex quiz & library. regex101: build, test, and debug regex The enhanced regex engine includes an additional flag to allow Java syntax to be used in JavaScript regular expressions. Regular expressions with the additional flag work in all places that expect a regular expression, such as String.prototype.split and String.prototype.replace.
4/1/2021 · If there's a match, the.match () method will return an array with the match. We'll go into more detail about this in a bit. If there isn't a match, the.match () method will return null. Some of you might have already noticed this, but if you look at the example above,.match () is only matching the first occurrence of the word "are". Javascript Regex Exercise. I have put the example above on a website that lets you experiment with regular expressions and what they match. Open the following link in a new tab: Regex Exercise on Regex101 . You will see the regular expression for our email, and a test string with the 3 lines from above. 4/4/2020 · Javascript RegExp¶ Regex or Regular expressions are patterns used for matching the character combinations in strings. Regex are objects in JavaScript. Patterns are used with RegEx exec and test methods, and the match, replace, search, and split methods of String. The test() method executes the search for a match between a regex and a specified string.
Parentheses contents in the match Parentheses are numbered from left to right. The search engine memorizes the content matched by each of them and allows to get it in the result. The method str.match (regexp), if regexp has no flag g, looks for the first match and returns it as an array: Defining Regular Expressions. In JavaScript, regular expressions are represented by RegExp object, which is a native JavaScript object like String, Array, and so on. There are two ways of creating a new RegExp object — one is using the literal syntax, and the other is using the RegExp () constructor. The rule of thumb is that simple regular expressions are simple to read and write, while complex regular expressions can quickly turn into a mess if you don't deeply grasp the basics. How does a Regular Expression look like. In JavaScript, a regular expression is an object, which can be defined in two ways.
Now and then lookaheads in JavaScript regular expressions cross my way, and I have to admit that I never had to use them, but now the counterpart lookbehinds are going to be in the language, too, so I decided to read some documentation and finally learn what these regex lookaheads and lookbehind are.
Python Regex Re Match Re Search Re Findall With Example
Javascript Regular Expression Word Boundaries
Regular Expression In Javascript
Loggly Derived Field Regex Matching May Require A Newline In
Cloudflare Denial Of Service Dos Blackout The Case For
Beginning To Use Regex In Javascript By Cristina Murillo
Javascript Regular Expression How To Create Amp Write Them In
Regular Expression Tutorial Learn How To Use Regular
How To Match Anything Up Until This Sequence Of Characters
The Regex Table Variable In Google Tag Manager Simo Ahava S
Regex To Test If Strings Exist In A String To Match
Using Regular Expressions And Groovy For Testing In Jmeter
Regular Expression In Javascript
The Essentials Of Regular Expressions By Sgwethan Towards
Javascript Regular Expression Ignore Case Exception Invalid
What Is Regex Regular Expression Pattern How To Use It In
8 Regular Expressions You Should Know
Python Regex Re Match Re Search Re Findall With Example
Javascript Regex Match Example How To Use Js Replace On A
Find And Replace Text Using Regular Expressions Pycharm
Playing With Regexp Named Capture Groups In Node 10
0 Response to "22 Javascript If Match Regex"
Post a Comment