24 Create Dynamic Table In Javascript
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. In this tutorial, we will learn how to create a dynamic table that dynamically adds a row on clicking on the Add Row button. Prerequisite. Basic knowledge of Html, CSS and JavaScript are required to fully understand the content of this post. Html. First, we have to create the initial table that you see when you first load the page using Html
Dynamically Creating An Html Table With Javascript Stack
Build a Dynamic Table with Sorting | HTML, CSS & JavaScript Frontend Mini Projects | Dylan IsraelThank you to our sponsor: https://www.DevMountain MY CO...
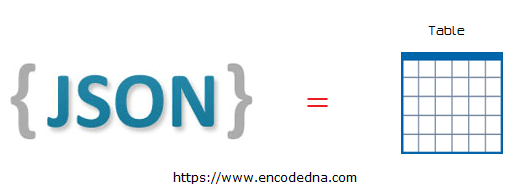
Create dynamic table in javascript. Use JavaScript to build a dynamic html table using loops. This video discusses the structure of HTML tables and explains how to use the for loop to create th... 26/9/2020 · This tutorial will teach you how to create a dynamic HTML table through the use of Javascript and Document Object Model (DOM) manipulation. The number of table rows and cell content will vary depending on your data. In this tutorial, I will be creating a scoreboard for a Javascript video game and the data will be coming from a fetch request. In addition, you will learn how you can dynamically create a table in JavaScript using createElement () Method. Note: You can also use jQuery to convert data from a JSON file to an HTML table, and using this process you can create a simple CRUD application using either jQuery or JavaScript.
Next, we create some new cell elements for the new row. Finally we set the HTML (value) of these cells to the elements in the array and age. innerHTML does this. Doing this will create a new row in the table; making it a nice dynamic table.. You can also delete rows but I will leave this for another post. Yep, this is the "alternate" way to generate an HTML table. The basic mechanics of looping through an array remains, but we now create the table with HTML objects: Create a new HTML table - table = document.createElement("table"); Add a new row to the table - row = table.insertRow(); Add cells to the row - cell = row.insertCell(); 2. I'm trying to dynamically create a table of input fields (two per field) that I will then send off to a Python script. Here's the expected behaviour: User chooses "manual entry," specifies rows, columns, of table, clicks button. Table is printed with two input fields per cell (e.g.: A (i,j), B (i,j) ), user fills in all fields, hits button.
ModernTable is a simple modern JavaScript ES6 library that fetches JSON data in an HTML table that renders nicely inside a Bootstrap 4 tag. display json data in html using javascript dynamically, create dynamic table from json object in javascript, jquery dynamically create table from json, create table from json data First a dynamic HTML Table is created using JavaScript createElement method. Adding the Header Row The Header Row will be built using the first element of the Array as it contains the Header column text values. JavaScript: Create a user defined table, accepting rows and columns Last update on February 26 2020 08:08:58 (UTC/GMT +8 hours) JavaScript DOM: Exercise-7 with Solution. Write a JavaScript function that creates a table, accept row, column numbers from the user, and input row-column number as content (e.g. Row-0 Column-0) of a cell.
JSON is lightweight and language independent and that is why it's commonly used with jQuery Ajax for transferring data. Here, in this article I'll show you how to convert JSON data to an HTML table dynamically using JavaScript. In addition, you will learn how you can dynamically create a table in JavaScript using Create Element() Method. Now that we have covered all the basics of JavaScript, we will use its implementation in this tutorial in some good JavaScript Problems along with a brief use of HTML. In this tutorial, we will create a dynamic table using JavaScript.Along with that, we will be implementing the JavaScript snippet inside an HTML body. I'm trying to create a table in javascript and put a header on it. I tried to incorporate the answer from this SO question but perhaps I didn't include it in the right place. The body of the table works perfectly, but the header appends as a bunch of text to the top instead of a nicely formatted header. Here is the code:
In line 18, we have used the <h2> tag for writing the heading Create dynamic table from ArrayList using JavaScript. In <h2> tag, we have styled using the text-align:left to place the heading on the left side of the HTML page. In line 20, we used the <table> tag to pass the ArrayList values later using the id='fetch' attribute. Tabulator is a lightweight jQuery UI plugin designed to make building complex interactive tables a doddle, using only a few lines of JavaScript you can turn almost any data source into a... To remove rows in the table, I’ll add dynamically created buttons (using JavaScript) in each row of the table. For data entry, I’ll create and add textboxes in each cell, dynamically. The second button will submit the data in the table. The first method createTable () in the script creates the table.
If you want to create dynamic editable rows in a table using html and javascript, below example will helps you. In the below code first I have created a button and then created table in which I have added 2 columns, as the output is shown ate the end of this tutorial. when we need an editable row in my html application we just click on AddRow ... With document.createElement() method you can create a specified HTML element dynamically in JavaScript. Once created, you can insert (or add) the element to your web page, or add it to a pre-defined element or a dynamically created element. In fact, you can create an entire form dynamically using this method. Today we will learn how to create dynamic tables using jquery ajax using data from server side in the form of json object. The good thing will be that all would be done without having a postback. What we will learn: Some basic HTML structure for table; Jquery ajax syntax; Creation of WebMethod for ajax call; Using JSON object; What we need to ...
Dynamically creating a HTML table with JavaScript. The following example attempts to demonstrate how a html <table> can be dynamically created with JavaScript. <!DOCTYPE html> <html> <head> <meta content="text/html;charset=utf-8" http-equiv="Content-Type"> <title>Create a html table with java script</title> </head> <script ... This JavaScript tutorial has two goals in mind. The first goal is to show you how to generate a dynamic HTML table, rows, and cells. The second the goal is to generate a random number within a range of numbers and storing the values within the dynamically generated table. Generating random numbers is a common question found on many forum sites. JavaScript is a dynamic programming language which can be applied to HTML documents to improve interactive behavior of websites. Dynamic tables created using JavaScript lets you organize your data in rows and columns which makes it easy to access and store the data. Let us see how to create a dynamic table using JavaScript.
This article will be helpful to create a dynamic table and dynamic grid using javascript and. html. Shrink Copy Code. I have used DOM for the same purpose. The code is given below. Javascript: function addRowToTable () { var tbl = document.getElementById ( 'tblSample' ); var lastRow = tbl.rows.length; // if there's no header row in the table ... Now we'll insert rows, cells and checkboxes dynamically using JavaScript. First we'll select the table: Create sample data object: Create a new function addRows: We've created the tbody tag and assigned it an id, now its time to insert rows inside the tbody tag. We can use three methods to insert a table row: JavaScript allows you to create tables in dynamic way by adding rows and cells using HTML 5 table API. In this article, we will create two different table using table API and JavaScript. dynamic table using rows dynamic table using rows and cells.
createCaption () Creates an empty <caption> element and adds it to the table. createTFoot () Creates an empty <tfoot> element and adds it to the table. createTHead () Creates an empty <thead> element and adds it to the table. deleteCaption () Removes the first <caption> element from the table. 25/2/2019 · function generateTableHead (table, data) {let thead = table. createTHead (); let row = thead. insertRow (); for (let key of data) {let th = document. createElement ("th"); let text = document. createTextNode (key); th. appendChild (text); row. appendChild (th);}} let table = document. querySelector ("table"); let data = Object. keys (mountains [0]); generateTableHead (table, data); 22/6/2017 · 1. how to add border into my table which table is dynamically generated..here is the code.. var table = document.createElement ('table'); for (var i = 1; i < 4; i++) { var tr = document.createElement ('tr'); var td1 = document.createElement ('td'); var td2 = document.createElement ('td'); var text1 = document.createTextNode ('Text1'); var ...
The basic steps to create the table in sample1.html are: Get the body object (first item of the document object). Create all the elements. Finally, append each child according to the table structure (as in the above figure). The following source code is a commented version for the sample1.html.
A Vue Js Server Side Component To Create Dynamic Tables
Editable Html Table Using Javascript Jquery With Add Edit
How To Bind Javascript Array To Dynamic Html Table Stack
Larachat Articles Create Dynamic Data Table Component With
Programmers Sample Guide Dynamically Generate Html Table
Java Script Can T Retrieve Data From Dynamically Created
How To Create Customized Dynamic Table In React With
Add Edit And Delete Data In An Html Table Using Javascript
Convert Json Data Dynamically To Html Table Using Javascript
Programmers Sample Guide Dynamically Generate Html Table
Javascript Dom Tutorial Dom Manipulation And Create A
Easily Create A Form And A Dynamic Table In Vue Js Laptrinhx
Dynamic Table Creation In Pure Javascript Gridtablejs Css
Programmers Sample Guide Dynamically Generate Html Table
Creating A Dynamic Html Table With Javascript By Daniel
How To Add Tables In Wordpress Posts And Pages No Html Required
Easily Create A Form And A Dynamic Table In Vue Js
How To Create Dynamic Html Table With Javascript And Dom
For Loop In Html Table Using Javascript Javascript Loop
Handling Dynamic Web Tables Using Selenium Webdriver
How To Use Javascript Ajax Response To Create Table
Add Edit And Delete Data In An Html Table Using Javascript
Dynamically Add Or Remove Table Rows In Javascript And Save
0 Response to "24 Create Dynamic Table In Javascript"
Post a Comment