24 Javascript If Property Exists
The if/else statement is a part of JavaScript's "Conditional" Statements, which are used to perform different actions based on different conditions. In JavaScript we have the following conditional statements: Use if to specify a block of code to be executed, if a specified condition is true As you can see, with jQuery, it is even simpler to check if an element exists or not. If the length attribute of an element object is 0, then it does not exist. If you run the snippet above, you should see an alert dialog saying "Element exists!" Hopefully, you found this guide to be useful!
The JavaScript interpreter looks for a property in an object, if not found, it looks for the property in the prototype chain. If this scenario didn't make any sense to you, you need to look up Prototypal Inheritance. The reliable way of checking if a property exists in an object or not is using the hasOwnProperty() method. This method check if ...
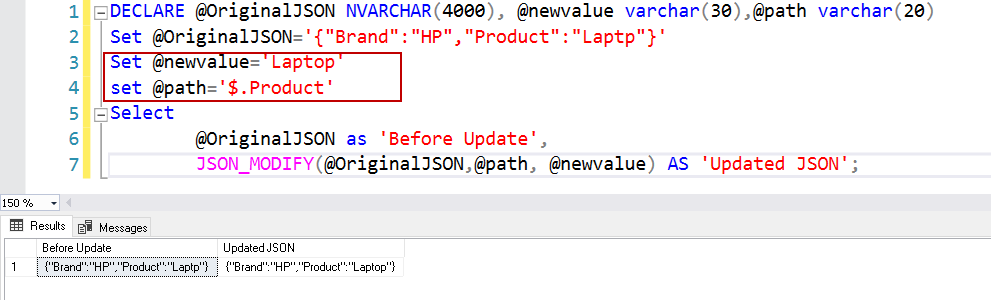
Javascript if property exists. Fortunately, JavaScript offers a bunch of ways to determine if the object has a specific property: obj.prop !== undefined: compare against undefined directly. typeof obj.prop !== 'undefined': verify the property value type. obj.hasOwnProperty ('prop'): verify whether the object has an own property. The in operator is another way to check the presence of a property in an object in JavaScript. It returns true if the property exists in an object. Otherwise, it returns false. Let us use the in operator to look for the cake property in the food object: Last Updated : 29 May, 2019. Given a JSON Object, the task is to check whether a key exists in Object or not using JavaScript. We're going to discuss few methods. hasOwnProperty () This method returns a boolean denoting whether the object has the defined property as its own property (as opposed to inheriting it). Syntax:
This is a type-safety check in JavaScript, and TypeScript benefits from that. However, there are some cases where TypeScript at the time of this writing needs a little bit more assistance from us. Let's assume you have a JavaScript object where you don't know if a certain property exists. The object might be any or unknown. In JavaScript ... 14/6/2012 · Several ways to check if an object property exists. const dog = { name: "Spot" } if (dog.name) console.log("Yay 1"); // Prints. if (dog.sex) console.log("Yay 2"); // Doesn't print. if ("name" in dog) console.log("Yay 3"); // Prints. if ("sex" in dog) console.log("Yay 4"); // Doesn't print. 10/1/2021 · The common ways to check if a property exists in an object are: The easiest is to use the hasOwnProperty() function – var exist = OBJECT.hasOwnProperty("PROPERTY"); Extract the keys from the object, then use the includes() function to check. var keys = Object.keys(OBJECT); var exist = keys.includes("PROPERTY");
You can use the JavaScript some () method to find out if a JavaScript array contains an object. This method tests whether at least one element in the array passes the test implemented by the provided function. Here's an example that demonstrates how it works: A Javascript object has normally the hasOwnProperty native method. The hasOwnProperty method returns a boolean indicating whether the object has the specified property as first parameter. Unlike the in operator, this method does not check down the object's prototype chain. To check if an attribute with a specified name exists, you use the hasAttribute () method: const result = element.hasAttribute (name); Code language: JavaScript (javascript) It returns true if the attribute with the specified name exists; otherwise false. The following example checks if the title attribute of the anchor element exists:
JavaScript Program to Check if a Key Exists in an Object JavaScript Program to Check if a Key Exists in an Object In this example, you will learn to write a JavaScript program that checks if a key exists in an object. To understand this example, you should have the knowledge of the following JavaScript programming topics: 1/9/2021 · The easiest way to check if an object property exists but is undefined in JavaScript is to make use of the Object.prototype.hasOwnProperty method which will let you find properties in an object. There are mainly 3 ways to check if the property exists. The first way is to invoke object.hasOwnProperty (propName). The method returns true if the propName exists inside object, and false otherwise. hasOwnProperty () searches only within the own properties of the object.
Published May 31, 2020. Given a JavaScript object, you can check if a property key exists inside its properties using the in operator. Say you have a car object: const car = { color: 'blue' } We can check if the color property exists using this statement, that results to true: 'color' in car. We can use this in a conditional: JavaScript objects are included in all aspects of the language, so you need to learn them as soon as you start to study it. Objects are created with figure brackets {…} and should have a list of properties. Property is known as a "key: value", in which key or property name is a string and value can be whatever. The some () method takes a callback function, which gets executed once for every element in the array until it does not return a true value. The some () method returns true if the user is present in the array else it returns false. You can use the some () method to check if an object is in the array.
Use the hasOwnProperty()method to check if an property exists in the own properties of an object. Use the inoperator to check if a property exists in both own properties and inherited properties of an object. 13/12/2020 · If we wanted to check if, for example, the name property with a specific value exists in the objects array, we could do it in the following ways: Using some() Introduced in ES5, the some() method returns a boolean value. It tests whether at least one element in the array satisfies the test condition (which is implemented by the provided function). When used with function calls, it returns undefined if the given function does not exist. This results in shorter and simpler expressions when accessing chained properties when the possibility exists that a reference may be missing.
So today I would like to list out the ways to check if the property exists in an object. 1) Using Object method hasOwnProperty () The most common solution would be to use hasOwnProperty () which is one of the common object methods. This method returns a boolean indicating whether the object has the specified property. Property 'fromPromise' does not exist on type 'typeof Observable'. rxjs 6 how to check if an element exists in an array of objects js javascript check if variable is object 9/8/2019 · Since JavaScript allows you to create dynamic objects, you have to be careful and check if an object’s property actually exists. There are at least 2 ways to do this. hasOwnProperty. hasOwnProperty...
36 How To Check If Property Exists In Object Javascript Written By Roger B Welker. Tuesday, August 31, 2021 Add Comment Edit. Method 1: Using the prop method: The input can be accessed and its property can be set by using the prop method Uploading Files Using Formidable In A Node Js Application. Unlike the in operator, hasOwnProperty does not check for a property in the object's prototype chain. If an object is an Array, this method can check whether an index exists. If the specified property exists in the object or its prototype chain, the in operator returns true. check if property exists javascript and overwrite it; javascript has property; check if attribute exists javascript object; typescript "array.hasOwnProperty( pos )" grpc; ajs get hasOwnProperty values; isproperty javascript; javascript does object have property; javascript element exists in object; nodejs check if object attribute is set
The hasOwnProperty () method returns true if the specified property is a direct property of the object — even if the value is null or undefined. The method returns false if the property is inherited, or has not been declared at all. That would be naive! The above code (to the new developer or non-double-banger) might say "If toddObject.favouriteDrink exists, do something".But no, because (I'll say it again…) this casts values, the value is null and falsy - even though the property exists. It's generally not a good idea in this case to use it for checking if a property exists incase it has a falsy value to begin ...
Properties Supported Data Types Mixpanel Help Center
5 Things You Need To Know About The Delete Operator In
4 Ways To Check If The Property Exists In Javascript Object
Sifat On Twitter Optional Chaining In Javascript
Javascript How To Check If A Property Exists In An Object
Here Are The New Built In Methods And Functions In Javascript
5 Ways To Check If Property Exists In Javascript Object
How To Check If A Key Exists In A Javascript Object
The Principles Of Object Oriented Javascript By Việt Hung Cao
How To Check If A Key Exists In An Object In Javascript
Javascript Array Contains A Step By Step Guide Career Karma
How To Access Properties Of Office Js Objects That Don T
Here Are The New Built In Methods And Functions In Javascript
How To Check If A Property Exists On A Javascript Object By
Modifying Json Data Using Json Modify In Sql Server
Debug Node Js Apps Using Visual Studio Code
How Do I Check If An Object Has A Specific Property In
2 Ways To Check If Value Exists In Javascript Object
Why Property Does Not Exist On Type In Object Destructuring
Javascript How To Check If Object Property Exists Code Example
How To Use Object Destructuring In Javascript
0 Response to "24 Javascript If Property Exists"
Post a Comment