29 Mongodb Javascript Example Client
10/12/2020 · This method is used to select a MongoDB database. Here is the complete example code: connect-mongo-client.js. const MongoClient = require('mongodb'). MongoClient; const url = 'mongodb://127.0.0.1:27017'; MongoClient.connect( url, { useNewUrlParser: true, useUnifiedTopology: true }, (err, client) => { if ( err) { return console.log( err); } const db ... Export MongoDB data to CSV file using fs. For this method, we need json2csv module. The module has Parser class that we can use parse () method to get the CSV formated data as a string. Then fs writeFile () function helps us to write the string to CSV file. Install with the command: npm install json2csv.
Caching For Performance With Amazon Documentdb And Amazon
The MongoDB shell is an interactive JavaScript shell. As such, it provides the capability to use JavaScript code directly in the shell or executed as a standalone JavaScript file. Subsequent hours that deal with using the shell to access the database and create and manipulate collections and documents provide examples that are written in ...
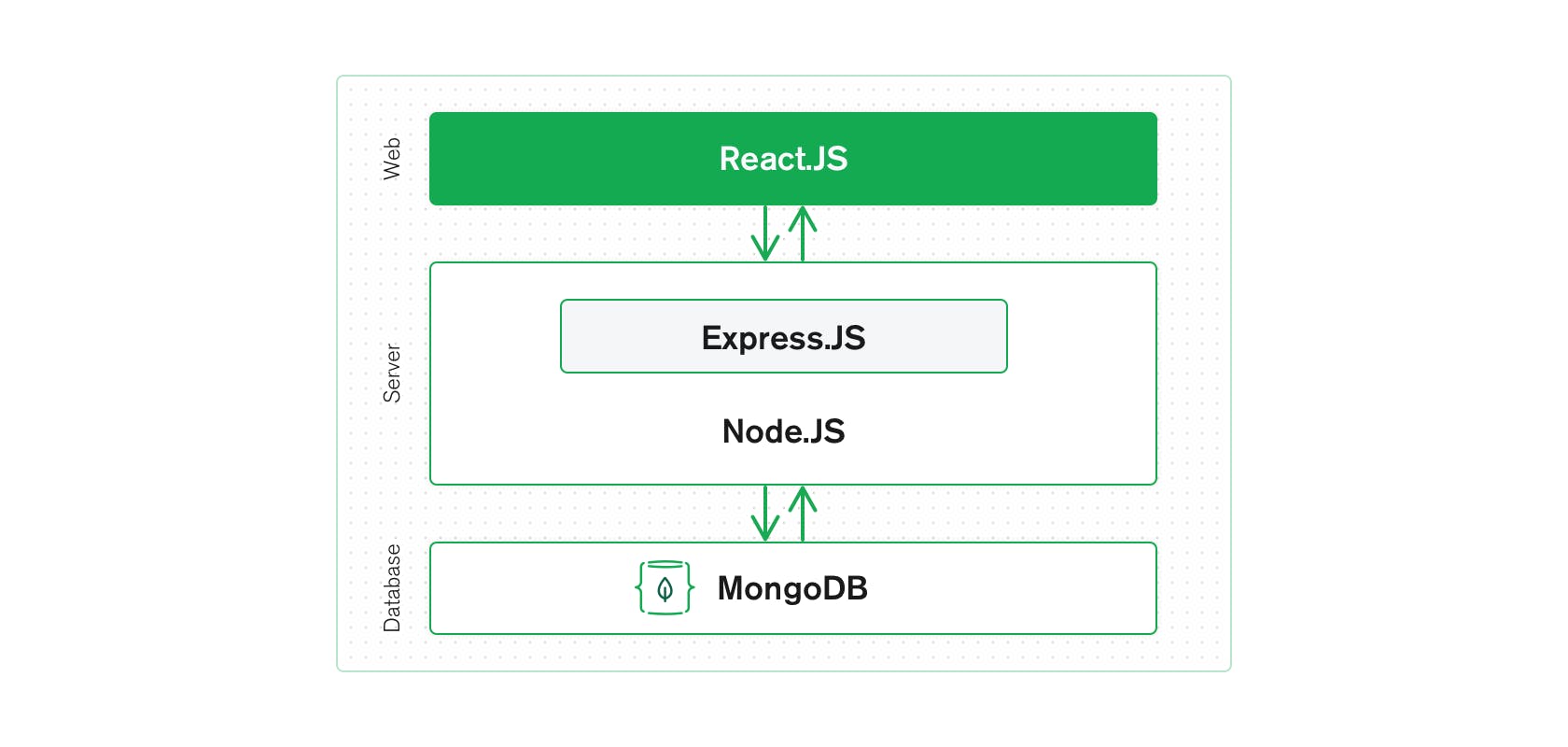
Mongodb javascript example client. JavaScript MongoClient.connect.ObjectId - 1 examples found. These are the top rated real world JavaScript examples of MongoDB.MongoClient.connect.ObjectId extracted from open source projects. You can rate examples to help us improve the quality of examples. MongoClient. Best JavaScript code snippets using mongodb. MongoClient.connect (Showing top 15 results out of 2,466) origin: parse-community / parse-server. _connect () { if (! this ._connectionPromise) { this ._connectionPromise = _mongodb.MongoClient. connect ( this ._databaseURI, this ._mongoOptions).then (client => { this ._client = client; Insert a Document¶. You can insert a single document into a collection using the insertOne() method on a MongoCollection object. To insert a document, construct a Document object that contains the fields and values that you want to store. If you call the insertOne() method on a collection that does not exist yet, the server automatically creates it for you.
36 Mongodb Javascript Example Client Written By Roger B Welker. Friday, August 20, 2021 Add Comment Edit. Mongodb javascript example client. Express Tutorial Part 3 Using A Database With Mongoose. Github Didactics Vue Mongodb Nodejs Example A Single Page. Building A Simple Crud App With Node Express And Mongodb. A client-side representation of a MongoDB cluster. Instances can represent either a standalone MongoDB instance, a replica set, or a sharded cluster. Instance of this class are responsible for maintaining an up-to-date state of the cluster, and possibly cache resources related to this, including background threads for monitoring, and connection ... Java MongoDB Connectivity. To connect with MongoDB database, Java project includes the following steps. Here, we are creating a maven project and providing dependency for the mongodb-driver. Follow the Steps: 1) Create a Maven Project 2) Add Dependecy to POM file // pom.xml
Best JavaScript code snippets using mongodb.MongoClient (Showing top 15 results out of 2,682) Write less, code more. _connect () { if (! this ._connectionPromise) { this ._connectionPromise = _mongodb. MongoClient .connect ( this ._databaseURI, this ._mongoOptions).then (client => { this ._client = client; return client.db (client.s… Check out my udemy Introduction to Database Engineering coursehttps://husseinnasser /courses Learn the fundamentals of database systems to understand and... This is a short tutorial and an example app which reads data from a MongoDB database. The app is using MongoDB, Express.js and Node.js. I'm using Apple macOS High Sierra 10.13.4 (17E199), Node.js…
7/7/2020 · const MongoClient = require('mongodb').MongoClient; const url = 'mongodb://localhost:27017'; MongoClient.connect(url, { useNewUrlParser: true }, (err, client) => { if (err) throw err; const db = client.db("testdb"); let collection = db.collection('cars'); let query = { name: 'Volkswagen' } collection.findOne(query).then(doc => { console.log(doc); }).catch((err) => { console.log(err); … Find One. To select data from a collection in MongoDB, we can use the findOne() method. The findOne() method returns the first occurrence in the selection.. The first parameter of the findOne() method is a query object. In this example we use an empty query object, which selects all documents in a collection (but returns only the first document). The official MongoDB Node.js driver allows Node.js applications to connect to MongoDB and work with data. The driver features an asynchronous API which allows you to access method return values through Promises or specify callbacks to access them when communicating with MongoDB. These docs are for version 3.6 of the MongoDB Node.js driver.
For MongoDB, this tutorial uses Azure Cosmos DB. Cosmos DB supports MongoDB client connections. Create a resource group. A resource group is a logical container into which Azure resources, such as web apps, databases, and storage accounts, are deployed and managed. For example, you can choose to delete the entire resource group in one simple ... Node.js MongoDB Tutorial with Examples. Mostly all modern-day web applications have some sort of data storage system at the backend. For example, if you take the case of a web shopping application, data such as the price of an item would be stored in the database. The Node js framework can work with databases with both relational (such as ... We're over halfway through the Quick Start with MongoDB and Node.js series. We began by walking through how to connect to MongoDB and perform each of the CRUD (Create, Read, Update, and Delete) operations.Then we jumped into more advanced topics like the aggregation framework.. The code we write today will use the same structure as the code we built in the first post in the series; so, if you ...
Install MongoDB Driver. Let us try to access a MongoDB database with Node.js. To download and install the official MongoDB driver, open the Command Terminal and execute the following: The following code snippets come from the QuickTour.java example code that can be found with the driver source on github. Prerequisites A running MongoDB on localhost using the default port for MongoDB 27017 MongoDB is a database that allows you to store documents with a dynamic structure. These documents are saved inside a collection. Mongoose is a JavaScript library that allows you to define schemas with strongly typed data. Once a schema is defined, Mongoose lets you create a Model based on a specific schema.
Installing it in your Node.js project is as simple as running the following command: npm install mongodb --save. The driver makes connecting to your database, authentication, CRUD operations, and even logging/monitoring as simple as running a command. The driver also supports JavaScript promise/async features. Python MongoClient Examples. See a method and class attribute list by passing the class MongoClient to the dir() Python function. You'll see server_info() and list_database_names() information returned in the list. Get MongoDB server information from MongoClient. The server MongoDB has data to show when you use a client instance in this manner: 7/10/2012 · I understand that MongoDB is a server and runs remotely from the client, but for my example, I simply want to be able to query quickly and easily from client-side in-browser JavaScript: $("#toggle").click(function() { if ($(this).is(":checked") { // add items from mongodb addItems(mongodb.test.find({ age: { $gt: 5 }})); } else { $("#results").hide(); } });
2) Robo 3T. Robo 3T (formerly Robomongo) is the popular free GUI for MongoDB enthusiasts. This lightweight, open-source tool has cross-platform support and also embeds the mongo shell within its interface to provide both shell and GUI-based interaction. It is developed by 3T Software, the team behind Studio 3T, the IDE for MongoDB. There is a GUI client by MongoDB; it is the Compass. It is a desktop application (not browser based). Compass lets a user to connect to a database, and browse the collections in it. You can manage the collections and the documents in it. The GUI h... To connect and use your mongoDB on Azure Cosmos DB with JavaScript and mongodb, use the following procedure. Make sure Node.js and npm are installed. Create a Node.js project in a new folder: mkdir DataDemo && \ cd DataDemo && \ npm init -y && \ npm install mongodb && code . The command: Creates a project folder named DataDemo
If you start from scratch, create a new folder with your terminal and run npm init -y to start up a new Node.js project, and then run the npm install mongodb command.. Connecting to MongoDB. You require the mongodb package and you get the MongoClient object from it. An introduction into MongoDB using Javascript MongoDB is a NoSQL database that stores data in flexible, JSON like documents which means that fields can vary from document to document and data structure can be changed over time. In our modern world data is vast, unstructured, and sometimes unwieldy. 24 rows · In the example above, we set two connection options: poolSize=20 and writeConcern=majority. For more information on connection options, skip to the Connection Options section. The code below shows how you can use the sample connection URI in a client to connect to MongoDB. const { MongoClient } = require ( "mongodb" );
MongoDB Drivers API Documentation. Official Drivers. C. C++
Why Use Node Js A Comprehensive Tutorial With Examples Toptal
Node Js Mongodb User Authentication Amp Authorization With
How To Write A Simple Node Js Mongodb Web Service For An Ios
Mongodb Javascript Tutorial With Examples
Building A Simple Crud App With Node Express And Mongodb
Mongodb The Mongo Shell Amp Basic Commands Bmc Software Blogs
How To Develop A Boilerplate For An Api With Node Js Express
Nodejs Mongodb Tutorial Find Insert Update Delete Records
Creating A Secure Rest Api In Node Js Toptal
Storing And Retrieving Files From Mongodb Using Mean Stack
How To Integrate Mongodb With A Node Application Using
React Redux Http Client Nodejs Restapi Express Mongoose
Node Js Mongodb Tutorial How To Build Crud Application
Integration Of Node Js And Mongodb Simple Example Journaldev
Releases Mongodb Node Mongodb Native Github
Building A Simple Crud App With Node Express And Mongodb
What Is The Mern Stack Introduction Amp Examples Mongodb
Connect A Mongodb Application To Azure Cosmos Db Microsoft Docs
Node Express Angular 7 Graphql And Mongodb Crud Web App
Mean Stack Programmatic Ponderings
Web Development With Mongodb Amp Nodejs Explained Mobilunity
Github Mongo Express Mongo Express Web Based Mongodb Admin
A Beginner Level Introduction To Mongodb With Node Js
Nosqlbooster The Smartest Gui Tool And Ide For Mongodb
Node Js Mongodb Tutorial With Examples
Angular 11 Node Js Mongodb Crud Example With Express
Node Js Application Writing To Mongodb Kafka Streams
Building Resilient Applications With Amazon Documentdb With
0 Response to "29 Mongodb Javascript Example Client"
Post a Comment