35 How To Access Css Class In Javascript
JavaScript access CSS class by its name? Ask Question Asked 8 years, 10 months ago. Active 11 months ago. Viewed 9k times 6 1. I have to access CSS class by name, and the code below works. However if I try hui["myclass"] or hui[".myclass"] instead of hui[0] it cannot find it. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http ... You do this using CSSOM, which is a rather different API, even though you first get access to it from the DOM. Unlike the DOM, CSS rules don't have ids or class names that can be searched for cleanly and quickly, so we have to search through the list of stylesheets and style rules.
1 week ago - The getElementsByClassName method of Document interface returns an array-like object of all child elements which have all of the given class name(s).
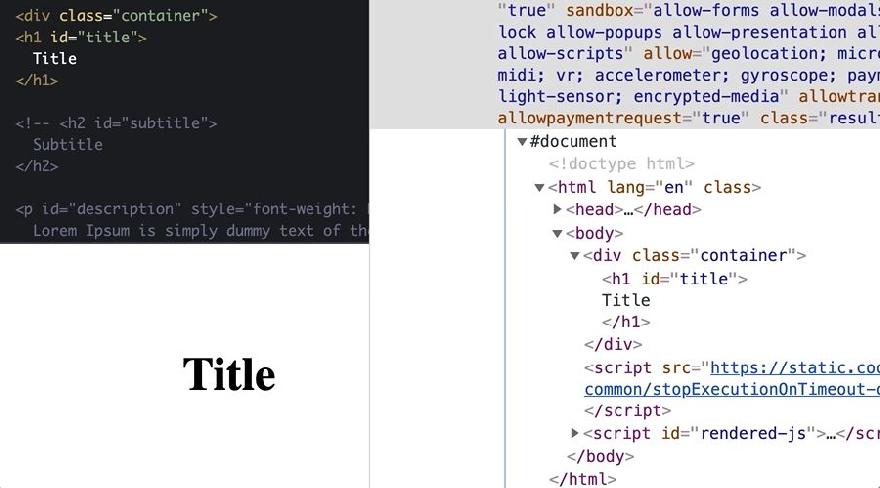
How to access css class in javascript. This gives us all the CSS rules for all the stylesheets. We want to discard some of those, so let's move on. Step 4: Discard any rules that aren't basic style rules. CSS rules come in different types. CSS specs define each of the types with a constant name and integer. The most common type of rule is the CSSStyleRule. Dec 17, 2015 - For class selectors, jQuery uses JavaScript's native getElementsByClassName() function if the browser supports it. ... Finds the element with the class "myClass". class is a reserved word in JavaScript, so in order to access the element's class, you use element.className. You can append strings to className if you want to add a class to an element, or you could just overwrite className and assign it a whole new class. Check out the element class names example. Summary
Change Variables With JavaScript. CSS variables have access to the DOM, which means that you can change them with JavaScript. Here is an example of how you can create a script to display and change the --blue variable from the example used in the previous pages. For now, do not worry if you are not familiar with JavaScript. Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics. So, the statement $( "p" ).css( "color", "red !important" ) does not turn the color of all paragraphs in the page to red as of jQuery 3.6.0. Do not depend on that not working, though, as a future version of jQuery may add support for such declarations. It's strongly advised to use classes ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. To do that, first we create a class and assign it to HTML elements on which we want to apply CSS property. We can use className and classList property in JavaScript. Approach: The className property used to add a class in JavaScript. It overwrites existing classes of the selected elements. If we don't want to overwrite then we have to add a ... Oct 24, 2017 - I have to access CSS class by name, and the code below works. However if I try hui["myclass"] or hui[".myclass"] instead of hui[0] it cannot find it.
The CSS file is used to describe how HTML elements will be displayed. There are various ways to add CSS file in the HTML document. JavaScript can also be used to load a CSS file in the HTML document. Approach: Use document.getElementsByTagName() method to get HTML head element. Create new link element using createElement('link') method. Aug 07, 2019 - Say you want to fetch the value of a CSS property in a web page, one that is set using a stylesheet. How can you do so? Definition and Usage. The .class selector selects elements with a specific class attribute. To select elements with a specific class, write a period (.) character, followed by the name of the class. You can also specify that only specific HTML elements should be affected by a class.
Setting a CSS Variable's Value. To set the value of a CSS variable using JavaScript, you use setProperty on documentElement 's style property: document. documentElement. style .setProperty('--my-variable-name', 'pink'); You'll immediately see the new value applied everywhere the variable is used. Another benefit in using a framework is that you can more easily add or remove class names rather than simply replace the entire class attribute's value (e.g. to remove "myclass" from an element with a class attribute of "myclass myotherclass" you'd otherwise have to replace the attribute value with "myotherclass" or do something funky like split the string into an array and remove the entry ... In CSS, selectors are patterns used to select the element(s) you want to style, but as you can tell from the title above, selectors are also useful in javascript and below are some examples on how to use them. Basics Using a selector in javascript Use the .querySelector method
JAVASCRIPT. js accessing css vars. Line by line here: 4 — grab footer element. 5 — grab NodeList of all inputs on the page. 7 — input CHANGE EventListener. 8 — input MOUSEMOVE ... The classList property allows greater performance and functionality to alter your HTML elements and their CSS classes within JavaScript. For additional reading, check out the article How To Modify Attributes, Classes, and Styles in the DOM and the ebook Understanding the DOM — Document Object Model . Summary: in this tutorial, you will learn how to use the JavaScript className property to manipulate CSS classes of an element. The className is the property of an element. It returns a space-separated list of CSS classes of the element: Suppose that you have the following ul element: The following code shows the classes of […]
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. May 17, 2018 - In this tutorial, we learned how to access, modify, and remove attributes on an HTML element in the DOM using plain JavaScript. We also learned how to add, remove, toggle, and replace CSS classes on an element, and how to edit inline CSS styles. For additional reading, check out the documentation ... CSS Object Model (CSSOM) InsertRule() According to MDN: The CSS Object Model is a set of APIs allowing the manipulation of CSS from JavaScript. It is much like the DOM, but for the CSS rather than the HTML. It allows users to read and modify [the] CSS style dynamically. The CSSStyleSheet.insertRule() method inserts a new CSS rule to a ...
Multiple classes can be applied to a single element in HTML and they can be styled using CSS. In this article, we will stick to only two classes. But the concepts used in assigning two classes can be extended to multiple classes as well. Assigning classes to an element in HTML: The names of the classes can be written within the "class ... Aug 28, 2020 - This is a tutorial on how to add a CSS class to a HTML element using JavaScript. Alternatively, you can also use the getPropertyValue() method on the styles object to access a CSS property. It accepts the actual name of the CSS property and not the one used for JavaScript: const fontWeight = styles. getPropertyValue ('font-weight'); // 700. Note: The value 700 is equivalent to bold for the CSS property font-weight.
And then, simply add the class to the element using JavaScript: const btn = document. querySelector ('.btn'); // add CSS class btn. classList. add ('btn'); Take a look at this guide to learn more about setting CSS styles using vanilla JavaScript. The purpose of these tutorials is not to discourage the use of CSS classes. May 06, 2021 - Learn more about How to Add/Remove CSS Classes Using JavaScript from DevelopIntelligence. Your trusted developer training partner. Get a customized quote today: (877) 629-5631. Say you want to fetch the value of a CSS property in a web page, one that is set using a stylesheet. The style property of an element does not return it, because it only lists CSS properties defined in inline styles, or dynamically.
Since class is a reserved word in JavaScript, the name className is used for this property instead of class. This property is supported by all modern and old browsers, including Internet Explorer. Using classList Property. There is even a better way to manipulate CSS classes in JavaScript, thanks to the classList property. The class global attribute is a space-separated list of the case-sensitive classes of the element. Classes allow CSS and Javascript to select and access specific elements via the class selectors or functions like the DOM method document.getElementsByClassName. HTML CSS JAVASCRIPT SQL PYTHON PHP BOOTSTRAP HOW TO W3.CSS JAVA JQUERY C++ C# R React Kotlin ... The getElementsByClassName() method returns a collection of all elements in the document with the specified class name, as an HTMLCollection object. The HTMLCollection object represents a collection of nodes. The nodes can be accessed by index numbers.
A computed style value is the value after all CSS rules and CSS inheritance is applied, as the result of the CSS cascade. It can look like height:1em or font-size:125% . A resolved style value is the one finally applied to the element. This article outlines some CSS and JavaScript best practices that should be considered to ensure even complex content is as accessible as possible. Basic computer literacy, a basic understanding of HTML, CSS, and JavaScript, and understanding of what accessibility is. To gain familiarity with using CSS and JavaScript appropriately in your web ... Adding a CSS class to an element using JavaScript. Now, let's add the CSS class "newClass" to the DIV element "intro". For the purpose of this example, I have added a delay using the setTimeout() method so that you can see the style changing: //Delay the JS execution by 5 seconds //by using setTimeout setTimeout(function(){ //Add the ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The style property only retrieves inlined CSS values while getComputedStyle style retrieves computed CSS values. If this lesson has helped you, might enjoy Learn JavaScript , where you'll learn how to build anything you want from scratch. In a nutshell, to style elements directly using JavaScript, the first step is to access the element. Our handy querySelector method from earlier is quite helpful here. The second step is just to find the CSS property you care about and give it a value. Remember, many values in CSS are actually strings.
The querySelector () is a method of the Element interface. The querySelector () allows you to find the first element that matches one or more CSS selectors. You can call the querySelector () method on the document or any HTML element. The following illustrates the syntax of the querySelector () method: In this syntax, the selector is a CSS ... The following table defines the first browser version with full support for Classes in JavaScript: Chrome 49. Edge 12. Firefox 45. Safari 9. Opera 36. Mar, 2016.
Assigning An Id Or Class To An Element Wordpress Websites
View And Change Css Chrome Developers
How To Hide Elements On Specific Wordpress Pages
Add Css Class Javascript Code Change Class On Click In
Using Css Modules With React A Quick Overview Of What Is Css
View Webpage Source Html Css And Javascript In Google Chrome
Edit Css Content Of Before From Javascript Stack Overflow
How To Get Css Values In Javascript Zell Liew
Cannot Access Custom Css And Js Files From Server Stack
Css Disable Button Code Example
Javascript Style Attribute How To Implement Css Styles In
How To Get Css Values In Javascript Zell Liew
How To Manage Css Classes With Javascript Creative Bloq
An Introduction To Css In Js Examples Pros And Cons
Angular Global Css Styles Tektutorialshub
Webxperience Javascript Animation 2
Javascript Call Class Method Code Example
Change An Element Class Javascript Geeksforgeeks
The 10 Most Common Bootstrap Mistakes That Developers Make
Examine And Edit Html Firefox Developer Tools Mdn
Extending Ui With Html Javascript And Css Evaluation Guide
How To Remove Unused Css For Leaner Css Files Keycdn
View And Change Css Chrome Developers
Css Class Amp Id Cascading Style Sheets Html
Using Style Setproperty To Keep Css Property Names
Setting Css Styles With Javascript Soshace Soshace
Adding Or Removing Css Class In Lightning Web Component
Intellisense For Css Class Names In Html Visual Studio
Examine And Edit Html Firefox Developer Tools Mdn
Change An Element Class Javascript Geeksforgeeks
Checking To See If An Element Has A Css Pseudo Class In
Setting Css Styles With Javascript Soshace Soshace
How To Create A Shopping Cart Ui Using Css Amp Javascript
0 Response to "35 How To Access Css Class In Javascript"
Post a Comment