25 Javascript Day Of Week Function
JS this Keyword JS Debugging JS ... Calculate current week number in JavaScript Calculate days between two dates in JavaScript JavaScript String trim() JavaScript timer Remove elements from array JavaScript localStorage JavaScript offsetHeight Confirm password validation ... JavaScript Date now () Method. Below is the example of Date now () method. The date.now () method is used to returns the number of milliseconds elapsed since January 1, 1970, 00:00:00 UTC. Since now () is a static method of Date, it will always be used as Date.now (). Parameters: This method does not accepts any parameter.
Calculate Age Using Javascript Javatpoint
12/3/2012 · This will add a getDayOfWeek() function as a prototype to the JavaScript Date class. Date.prototype.getDayOfWeek = function(){ return ["Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday"][ this.getDay() ]; };
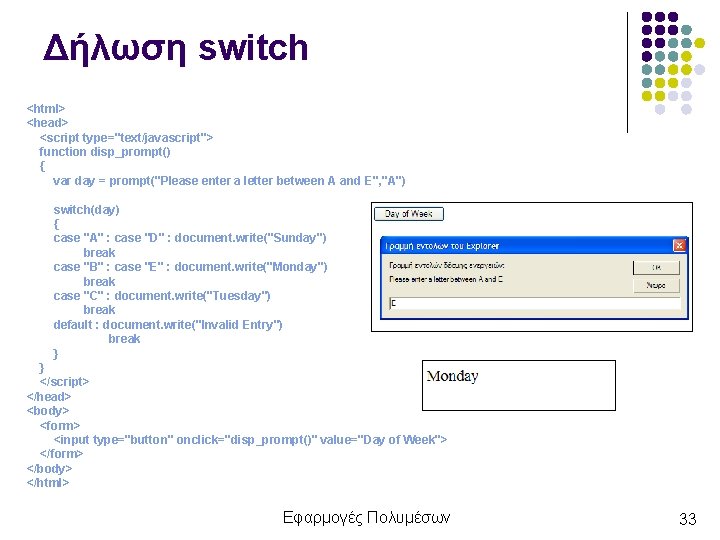
Javascript day of week function. Syntax. The switch expression is evaluated once. The value of the expression is compared with the values of each case. If there is a match, the associated block of code is executed. If there is no match, the default code block is executed. The getDay () method returns the weekday as a number between 0 and 6. Dec 03, 2018 - This is a short JavaScript guide on how to get the full textual representation of the day of the week. i.e. How to get “Monday”, “Tuesday” or “Wednesday” in JavaScript. Custom function. Returns the day of the week for the specified date according to local time. ... Try the following example. ... <html> <head> <title>JavaScript getDay Method</title> </head> <body> <script type = "text/javascript"> var dt = new Date("December 25, 1995 23:15:00"); document.write("getDay() : " ...
2/1/2019 · javascript function returning day of week x num of days later. What I am trying to write is a simple function that takes in a day (D), X number of days and returns the day X days later. Days can be represented by ('Mon', 'Tue', 'Wed', 'Thu','Fri','Sat','Sun'). X can be any int 0 and up. For Example D='Wed', X='2', return 'Fri', D='Sat' and X='5', ... Description The getDay () method is used to get the day of the week of a given date according to local time. The value returned by getDay () method is an integer corresponding to the day of the week: 0 for Sunday, 1 for Monday, 2 for Tuesday, 3 for Wednesday, 4 for Thursday, 5 for Friday, 6 for Saturday. Date and time in JavaScript are represented with the Date object. We can't create "only date" or "only time": Date objects always carry both. Months are counted from zero (yes, January is a zero month). Days of week in getDay () are also counted from zero (that's Sunday).
You have the current day of week, and you want to know how many days to go forward to get to a friday: day offset --- ----- 0 +5 1 +4 2 +3 3 +2 4 +1 5 +0 6 +6 You can use 5 - dayOfWeek to get the right value for most days: Jun 27, 2020 - Day of the week will be returned in the form of integer value ranging from 0 to 6 means 0 for Sunday, 1 for Monday, and so on till 6 for Saturday. ... Program 1: If the date of the month is not given, by default the function considers it as the first date of the month and hence accordingly ... See the Pen JavaScript - Find out the last day of a month-date-ex- 9 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to get difference between two dates in days. Next: Write a JavaScript function to calculate 'yesterday day'.
Despite the JavaScript Date warts, it's straightforward to add day(s) to a date in JavaScript. While it would be very easy to reach for moment.js or another date manipulation library (date-fns, luxon, dayjs) to do something as simple as adding days to a Date in JavaScript, writing a short helper function might just be easier. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. JavaScript Date Reference ... Returns the function that created the Date object's prototype: prototype: ... Method Description; getDate() Returns the day of the month (from 1-31) getDay() Returns the day of the week (from 0-6) getFullYear() Returns the year: getHours() Returns the hour (from 0-23) getMilliseconds() Returns the milliseconds ...
Jul 20, 2021 - The getUTCDay() method returns the day of the week in the specified date according to universal time, where 0 represents Sunday. This is the mistake: var onejan = new Date(this.getFullYear(), 0, 1) That's because, according to ISO, the first week of the year is the one that has the first Thursday. Or, in other words, the week that has Jan 4th in it. This solves the problem: var onejan = new Date(this.getFullYear(), 0, 4) That also means onejan is not the right name for that variable. See the Pen JavaScript - Get the week start date-date-ex-50 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to get time differences in years between two dates. Next: Write a JavaScript function to get the week end date.
Previous: Write a JavaScript function to get time differences in years between two dates. Next: Write a JavaScript function to get the week end date. ... [1, 2] is our initial value. This is the value we start with, and the value of the very first acc. During the first round, acc is [1,2], ... days of the week. Hello. Today is: Saturday, 7th August 2021. And the time is now (according to your computer): On this page, we will deal with the Months and the Days as we use them in JavaScript. We can get the day from the date object as follows: today= new Date () //as no date has been entered, then it is taken as today. The code below adds two new functions to the Date object. Add this to your source code. To get the ISO week number (1-53) of a Date object, use date .getWeek (). To get the corresponding four-digit year, use date .getWeekYear () . // This script is released to the public domain and may be used, modified and // distributed without restrictions.
day: Required. An integer representing the day of a month. Expected values are 1-31, but other values are allowed: 0 will result in the last day of the previous month-1 will result in the day before the last day of the previous month; If the month has 31 days: 32 will result in the first day of the next month; If the month has 30 days: JavaScript exercises, practice and solution: Write a JavaScript function to get a full textual representation of the day of the week Let's see the list of JavaScript date methods with their description. Methods. Description. getDate () It returns the integer value between 1 and 31 that represents the day for the specified date on the basis of local time. getDay () It returns the integer value between 0 and 6 that represents the day of the week on the basis of local time.
Nov 19, 2011 - Otherwise the first 3 days of 2016 would fall out. First days of the month are considered to comprise the first week for that month, no-matter which and how many days there are. If you need the function to work differently, you can tweak it according to your needs. Here's a quick way to get first and last day, for any start day. knowing that: 1 day = 86,400,000 milliseconds. JS dates values are in milliseconds . Recipe: figure out how many days you need to remove to get the your week's start day (multiply by 1 day's worth of milliseconds). In JavaScript, the first day of the week (0) means "Sunday", even if some countries in the world consider the first day of the week to be "Monday" You can use an array of names, and getDay () to return the weekday as a name:
Approach 2: Calculate the days from current date. In this approach, we will use the math function Math.floor() to calculate the days. Follow the below step: Define two date variables, i.e., date1 and date2; Initialize the date1 variables by creating the date objects using new Date(), which will take system date by default. Description. Javascript date getDay() method returns the day of the week for the specified date according to local time. The value returned by getDay() is an integer corresponding to the day of the week: 0 for Sunday, 1 for Monday, 2 for Tuesday, and so on.. Syntax. Its syntax is as follows −. Date.getDay() Return Value. Returns the day of the week for the specified date according to local time. JavaScript Get week number. GitHub Gist: instantly share code, notes, and snippets.
Syntax for How to find day of the week in Javascript How to find day of week. Example: If day is Sunday - returns 0 Monday - returns 1 Tuesday - returns 2 Wednesday - returns 3 Thursday - returns 4 Friday - returns 5 Saturday - returns 6 //Example 1 //Finds Current date in javascript var currDate = new Date(); //Find the day of month alert(currDate.getDay()); //Output: returns day based on current date. See the Pen JavaScript - Get a full textual representation of the day of the week-date-ex-21 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to get a textual representation of a day (three letters, Mon through Sun). See the Pen JavaScript - Get the week end date-date-ex-51 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to get the week start date. Next: Write a JavaScript function to get the month start date.
Custom function. Below, I've created a custom JavaScript function that will return the day of the week in a text format: /** * Function takes in a Date object and returns the day of the week in a text format. */ function getWeekDay(date){ //Create an array containing each day, starting with Sunday. Topic: JavaScript / jQueryPrev|Next · You can use the Date object methods getDate(), getMonth(), and getFullYear() to get the date, month and full-year from a Date object. Let's check out an example: Jul 13, 2011 - get week of the year in JavaScript. GitHub Gist: instantly share code, notes, and snippets.
Overview. Datejs is an open source JavaScript Date library for parsing, formatting and processing. The last official release was Alpha-1 on November 19th, 2007. The project has been mostly dormant since that early release. The getDay() method returns the day of the week for the specified date according to local time, where 0 represents Sunday. For the day of the month, see Date.prototype.getDate() . Syntax Then it is only a matter of looping for each day of the week and push each date into my week's array. But we also need to reconstruct our date. Line 6 "setDate()"
Sometimes we need to calculate the current week number or the week number for a given date. This problem can be solved using the JavaScript programming language. JavaScript offers several date functions, such as getDays (), getMonth (), getTime (), to solve date-related tasks.
Algorithms With Javascript A Day With Javascript Algorithms
Js Get Days Of The Week Stack Overflow
How Do I Get The Day Of Week Given A Date Stack Overflow
Now Getday Ranges Javascript Sitepoint Forums Web
L Script Typetextjavascript Welcome Message Var Name Welcome
Other Excel Functions Date Functions Rand
Demystifying Datetime Manipulation In Javascript Toptal
Inline Week Day Picker Plugin Jquery Weekdays Free Jquery
How To Compare Two Dates In Javascript
Calculating The Day Of The Week On A Glide Date In A Client
Get The Day Of The Week Name And Number In Power Bi Using Dax
Week 5 Vincent S If Else Javascript Exercise Moon Ima
Now Getday Ranges Javascript Sitepoint Forums Web
Javascript Day Of The Week Project Jsbeginners
Use The Getutcday Method To Return The Day Of The Week In
Calendar Toast Ui Make Your Web Delicious
Get The Current Week Using Javascript Without Additional
Node Red Based Custom Full Room Wake Up Light
Mysql Week Function W3resource
Xp Tutorial 3 New Perspectives On Javascript Comprehensive1
Week 5 Vincent S If Else Javascript Exercise Moon Ima
O Xrhsths Ania Kubow Javascriptgames Sto Twitter Package
Devtools Architecture Refresh Migrating To Javascript
0 Response to "25 Javascript Day Of Week Function"
Post a Comment