25 Javascript Check If Variable Is Function
Aug 24, 2019 - javascript create a function that counts the number of syllables a word has. each syllable is separated with a dash -. ... // How to create string with multiple spaces in JavaScript var a = 'something' + '\xa0\xa0\xa0\xa0\xa0\xa0\xa0' + 'something'; The if/else statement is a part of JavaScript's "Conditional" Statements, which are used to perform different actions based on different conditions. In JavaScript we have the following conditional statements: Use if to specify a block of code to be executed, if a specified condition is true
Chapter 12 Conditions Choosing Code To Run Get
In JavaScript, there are two ways to check if a variable is a number : isNaN () - Stands for "is Not a Number", if variable is not a number, it return true, else return false. typeof - If variable is a number, it will returns a string named "number". Note.
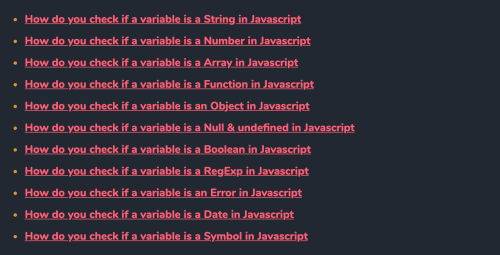
Javascript check if variable is function. # How to check if Variable is an Array in JavaScript Here's a Code Recipe to check whether a variable or value is either an array or not. You can use the Array.isArray() method. Apr 28, 2021 - typeof is a JavaScript keyword that will return the type of a variable when you call it. You can use this to validate function parameters or check if variables are defined. There are other uses as well. The typeof operator is useful because it is an easy way to check Aug 31, 2012 - You’d wrap the snippet around the function you want to check. So say, for example, you have a homepage slider using flexslider or something similar. You might call that function in the global footer or in a JS file that’s shared across pages. Well, if you’re not on the homepage and there is no slider, but you’re calling it in your javascript...
In this example, you will learn to write a JavaScript program that will check if a variable is undefined or null. Tutorials Examples Course Index Explore Programiz ... JavaScript Function and Function Expressions; Example 1: Check undefined or null How to check if a variable is an integer in JavaScript? How to check if a variable is an array in JavaScript? Check if a file exists in Java Check if a File exists in C#; How to check if a key exists in a Python dictionary? Check if MongoDB database exists? How to check if a vector exists in a list in R? How to check if a file exists or not in ... If a function takes a DOM element as a parameter, it can be a string, or a jQuery object , for example. Or why not both? With a small…
16/4/2020 · You can use the typeof operator to check the variable. It returns a string which indicates the type of the unevaluated operand. console .log( typeof '' === 'string' ); console .log( typeof function ( ) {} === 'function' ); console .log( typeof true === 'boolean' ); console .log( typeof Symbol () === 'symbol' ); console .log( typeof { key : 1 } === 'object' ); In JavaScript, checking if a variable is undefined can be a bit tricky since a null variable can pass a check for undefined if not written properly. As a result, this allows for undefined values to slip through and vice versa. Make sure you use strict equality === to check if a value is equal to undefined. Checking the type of a variable can be done by using typeof operator. It directly applies either to a variable name or to a variable.
Watch or Monitor Javascript Variables for Change ... Set the variable, call the function to monitor the variable, then test by pasting a new value into the console. ... Pythagorean Create an Image Zoom Window with Javascript Get String Between Strings with Javascript Immediately Invoke a Javascript Function Check if String Contains Substring ... // program to check if a variable is of function type function testVariable(variable) { if(Object.prototype.toString.call(variable) == '[object Function]') { console.log('The variable is of function type'); } else { console.log('The variable is not of function type'); } } const count = true; const x = function() { console.log('hello') }; testVariable(count); testVariable(x); In JavaScript, a variable can be declared either in the global scope or the local scope. Global Variables. Variables declared out of any function are called global variables. They can be accessed anywhere in the JavaScript code, even inside any function. Local Variables. Variables declared inside the function are called local variables to that ...
This may not make sense at first, but for scripts dealing with multiple frames or windows, and passing objects from one context to another via functions, this will be a valid and strong issue. For instance, you can securely check if a given object is, in fact, an Array using Array.isArray(myObj) javaScript check if variable is a number: isNaN () JavaScript - isNaN Stands for "is Not a Number", if a variable or given value is not a number, it returns true, otherwise it will return false. typeof JavaScript - If a variable or given value is a number, it will return a string named "number". See the article "Determining with absolute accuracy whether or not a JavaScript object is an array" for more details. Given a TypedArray instance, false is always returned. Examples
Similar to the PHP function of the same name, it takes a variable or property and tells you if the value is empty. The definition of empty depends on the value in question. PHP's empty ... Check if a function parameter is defined. A function expecting a number of parameters can be called with only part of them, or even none. In this case, the missing parameters (at the end of the list) are declared but have the value undefined. 15/4/2019 · Variable is of function type Using Strict Equal (===) operator: In JavaScript, ‘===’ Operator is used to check whether two entities are of equal values as well as of equal type provides a boolean result. In this example, we use the ‘===’ operator. This operator, called the Strict Equal operator, checks if the operands are of the same type.
Description: Determines if its argument is callable as a function. What's a parameter? A parameter can also be called an argument. It's the thing passed into a function that you do something with: function test (x) {return x + 1;} test (1); // ==> returns 2 var two = test (1); // this variable is now set to 2. But what if a function can do something without a parameter, or what if the functionality changes based on whether or not a parameter was passed in? Check if an object hasOwnProperty(). An alternative to the plethora of typeof answers, is the use of hasOwnProperty() which of course checks if an object (pretty much everything in JS) has a property i.e. a variable (amongst other things).. The hasOwnProperty() method returns a boolean indicating whether the object has the specified property as own (not inherited) property.
Sep 04, 2020 - This method is best suited for when you know you have a number and would to check if it is a NaN value, not for general number checking. ... The typeof() function is a global function that accepts a variable or value as an argument and returns a string representation of its type. JavaScript has ... I found that when testing native browser functions in IE8, using toString, instanceof, and typeof did not work. Here is a method that works fine in IE8 (as far as I know): function isFn(f){ return !!(f && f.call && f.apply); } //Returns true in IE7/8 isFn(document.getElementById); Alternatively, you can check for native functions using: PHP Array Functions PHP String Functions PHP File System Functions PHP Date/Time Functions PHP Calendar Functions PHP MySQLi Functions PHP Filters PHP Error Levels ... In JavaScript if a variable has been declared, but has not been assigned a value, is automatically assigned the value undefined.
There are 3 ways to create variables in JavaScript: let is block scope, var is function scope, and const is block scope…. medium . If the function was named using the function or class keywords but not assigned to a variable, then check typeof the function's given name. Happy coding! 💻💯🏆💯🙌. The quickest and accurate way to check if a variable is an object is by using the Object.prototype.toString () method. This method is part of Object 's prototype and returns a string representing the object: Object. prototype.toString.call( fruits); Object. prototype.toString.call( user); As you can see above, for objects, the toString ... To check if a particular function name has been defined, you can use the typeof operator: Watch a video course JavaScript - The Complete Guide (Beginner + Advanced) if (typeof myFunctionName === 'function') { myFunctionName (); } In the given case, the typeof operator will return undefined because myFunctionName () has not been defined.
We create two different variables - a function type variable and a string type variable. We create a custom function called isFunction, which checks to see if a JS variable is of type "function" or not. This returns TRUE or FALSE. We then test both of our variables and log the results to the console. An IF statement can be used to compare if the returned value is of the type 'Function'. //javascript check if function-Using object.prototype.toString <script > // Declare a variable and initialize it with an anonymous function var exampleVar = function() { /* A set of statements */ }; // to check a variable is of function type or not function checkFunction(x) { if (Object.prototype.toString.call(x) == '[object … 1 week ago - The typeof operator returns a string indicating the type of the unevaluated operand.
The isNaN () function determines whether a value is an illegal number (Not-a-Number). This function returns true if the value equates to NaN. Otherwise it returns false. This function is different from the Number specific Number.isNaN () method. The global isNaN () function, converts the tested value to a Number, then tests it. The typeof operator We can use the typeof operator to check if a given variable is a string. The typeof operator returns the type of a given variable in string format. Here is an example: PHP Array Functions PHP String ... MySQLi Functions PHP Filters PHP Error Levels ... If you want to check whether a variable has been initialized or defined (i.e. test whether a variable has been declared and assigned a value) you can use the typeof operator. The most important reason of using the typeof operator is that it does ...
Compared to typeof approach, the try/catch is more precise because it determines solely if the variable is not defined, despite being initialized or uninitialized.. 4. Using window.hasOwnProperty(). Finally, to check for the existence of global variables, you can go with a simpler approach. Each global variable is stored as a property on the global object (window in a browser environment ... Jul 20, 2018 - That works in some cases, but… if the variable was assigned to something other than a function—a string, number, or object, for example—you’d the if statement would validate as true and then you’d try to run something that wasn’t a function and get an error.
Writing Tests Postman Learning Center
Variable Guide For Google Tag Manager Simo Ahava S Blog
Thomas Findlay On Twitter This Comparison Is Heavily
The 10 Most Common Mistakes Javascript Developers Make Toptal
Working With Environment Variables In Python
Mastering Check Data Types In Javascript
Javascript Check If Variable Exists Is Defined Initialized
Headphone Screening Test Made For Pavlovia Online
Legacy Method In Solution 2 Challenge Search And Replace
3 Ways To Check If An Object Has A Property In Javascript
Javascript Mcq Multi Choice Questions Javatpoint
How To Check If Google Tag Manager Is Working Testing Gtm
How To Check If An Object Is Empty In Javascript
Angular Unit Testing Why And How To Unit Test Angular
How To Set Php Variable In Javascript With Example Phpcoder
Javascript Typeof How To Check The Type Of A Variable Or
Check If All Values In Array Are True Javascript Code Example
Everything About Javascript Objects By Deepak Gupta
Explaining Value Vs Reference In Javascript By Arnav
Javascript Programming With Visual Studio Code
How To Find Even Numbers In An Array Using Javascript
0 Response to "25 Javascript Check If Variable Is Function"
Post a Comment